Conducting effective LINQ interviews can be challenging for recruiters and hiring managers looking to assess a candidate's .NET development skills. By using a curated list of LINQ interview questions, you can gain valuable insights into an applicant's proficiency and problem-solving abilities in this essential technology.
This blog post provides a comprehensive collection of LINQ interview questions tailored for different experience levels and specific aspects of LINQ. From general concepts to advanced topics like deferred execution, we cover a wide range of questions to help you evaluate candidates thoroughly.
By utilizing these questions, you can make more informed hiring decisions and identify top LINQ talent for your team. Consider combining these interview questions with a pre-screening LINQ assessment to streamline your hiring process and ensure you're interviewing the most qualified candidates.
Table of contents
8 general LINQ interview questions and answers to assess applicants
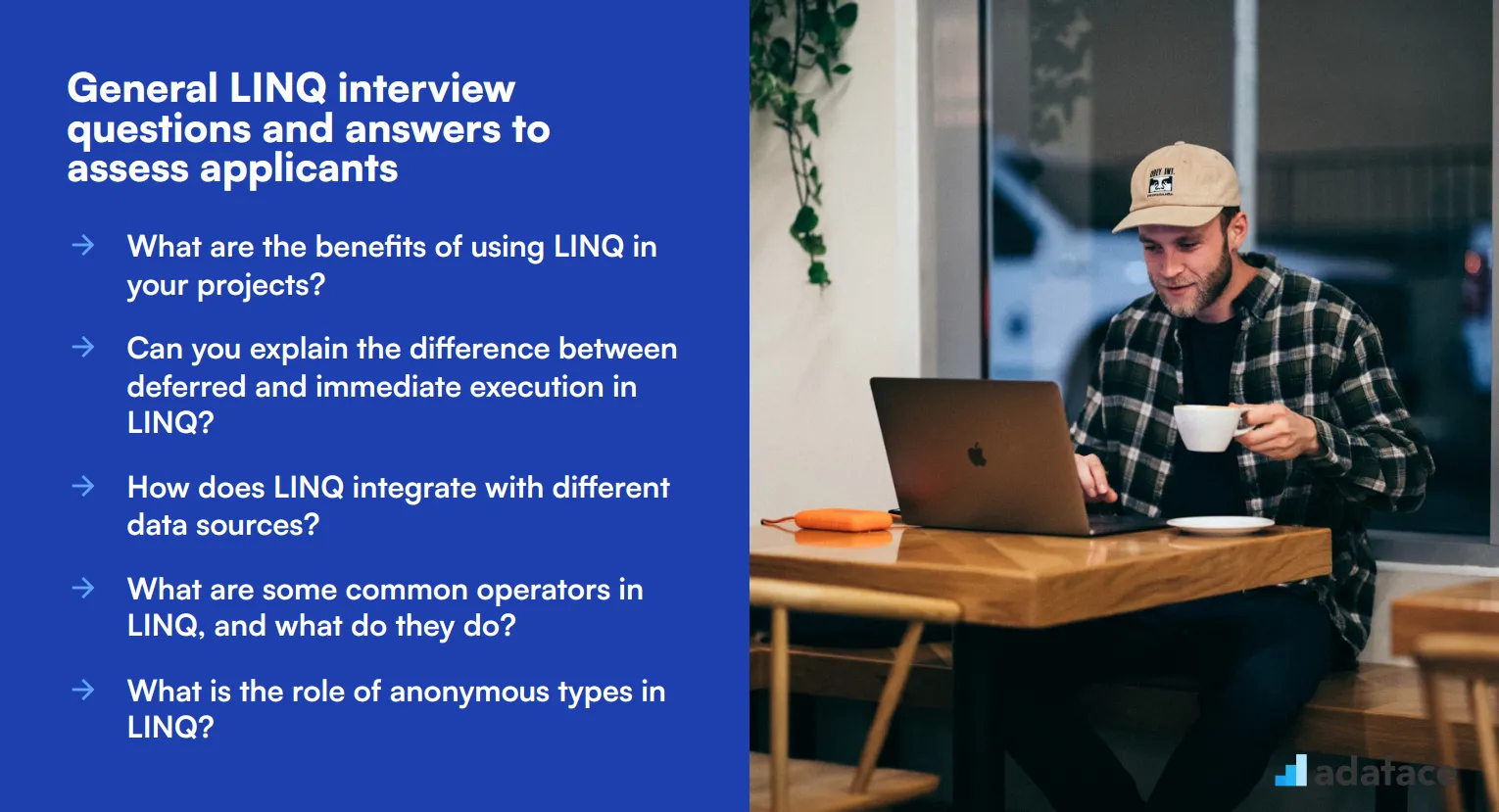
To assess whether applicants have a solid understanding of LINQ, use these general interview questions. These questions are designed to help you gauge their familiarity and comfort with LINQ concepts, which are crucial for any developer working with data in .NET environments.
1. What are the benefits of using LINQ in your projects?
LINQ provides a consistent and readable syntax for querying different data sources, such as databases, XML documents, and collections. This consistency helps reduce the learning curve, making it easier to switch between different types of data.
Another benefit is that LINQ can lead to more maintainable and concise code. By using LINQ, developers can express complex queries in a more readable format compared to traditional loops and conditionals.
Look for candidates who highlight the importance of readability, maintainability, and the reduction of boilerplate code. They should also mention the versatility of LINQ in handling various data sources.
2. Can you explain the difference between deferred and immediate execution in LINQ?
Deferred execution means that the query is not executed when it is defined but when it is enumerated, which allows for more efficient and optimized query processing. Examples of deferred execution methods are Select
, Where
, and Take
.
Immediate execution, on the other hand, means the query is executed and the data is retrieved immediately. Methods like ToList
, ToArray
, and Count
trigger immediate execution.
Candidates should understand how and when to use deferred execution for better performance and when immediate execution is appropriate. Strong answers will include examples of both types and their impact on application performance.
3. How does LINQ integrate with different data sources?
LINQ integrates with various data sources through different LINQ providers. For instance, LINQ to SQL and LINQ to Entities are used for querying databases, LINQ to XML is used for querying XML documents, and LINQ to Objects is used for querying in-memory collections.
These providers translate LINQ queries into the appropriate format for the underlying data source, ensuring that developers can use a unified syntax across different types of data.
Ideal candidates will mention the flexibility and power of LINQ providers and how they streamline querying different data sources. They should also demonstrate an understanding of the specific use cases for each provider.
4. What are some common operators in LINQ, and what do they do?
Common LINQ operators include Select
, Where
, OrderBy
, GroupBy
, Join
, and Aggregate
. Select
is used for projection, Where
is used for filtering, OrderBy
is for sorting, GroupBy
groups elements, Join
combines data from different sources, and Aggregate
performs calculations over a sequence of values.
These operators allow developers to perform a wide range of data manipulation tasks in a concise and readable way.
Candidates should be able to explain these operators and provide examples of how they have used them in previous projects. Look for detailed explanations and practical applications.
5. What is the role of anonymous types in LINQ?
Anonymous types in LINQ provide a way to create a new type without explicitly defining it. This is particularly useful in LINQ queries where you need to project data into a new shape. Anonymous types are often used in the Select
clause to create objects on the fly.
They are defined using the new
keyword followed by an object initializer, making the code more concise and readable.
Ideal candidates will discuss the benefits of using anonymous types, such as reducing the need for creating multiple classes, and provide examples of scenarios where they have effectively used them.
6. How does LINQ improve code readability?
LINQ improves code readability by providing a declarative syntax that clearly expresses the intent of the query. Instead of writing complex loops and conditional statements, developers can use LINQ to write more concise and understandable queries.
The use of method chaining and lambda expressions further enhances readability, making it easier to follow the flow of data manipulation.
Look for candidates who emphasize the importance of readable code and provide examples of how LINQ has helped them simplify complex data operations. They should also mention the benefits of reduced boilerplate code.
7. What are some challenges you might face when using LINQ?
One challenge is performance, especially when working with large data sets. Deferred execution can sometimes lead to unintended multiple enumerations of the data, which can impact performance.
Another challenge is the learning curve associated with LINQ syntax and understanding how different providers translate LINQ queries into underlying data source queries.
Candidates should discuss these potential pitfalls and how they have addressed them in their projects. Look for practical examples and solutions, such as optimizing queries and using profiling tools to identify performance bottlenecks.
8. How do you handle exceptions in LINQ queries?
Exceptions in LINQ queries can be handled using standard exception handling techniques like try-catch
blocks. It's important to anticipate potential errors, such as null reference exceptions or invalid operations, and handle them gracefully.
When working with deferred execution, exceptions may occur at the point of enumeration rather than at the point of query definition, so it's crucial to be aware of this distinction and handle exceptions accordingly.
Strong candidates will demonstrate a clear understanding of exception handling in the context of LINQ and provide examples of how they have implemented robust error handling in their queries.
15 LINQ interview questions to ask junior developers
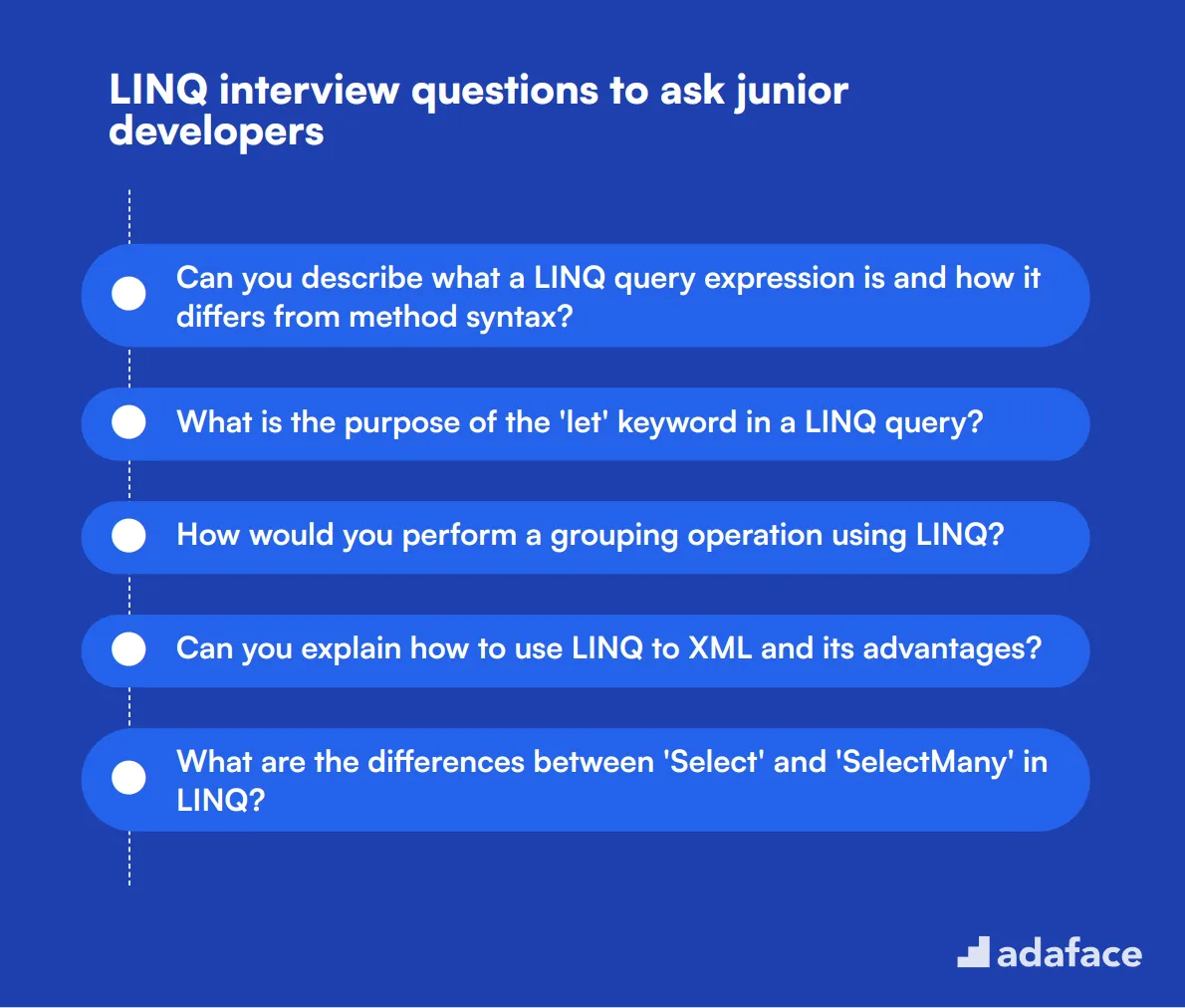
To assess whether your candidates possess essential knowledge of LINQ, consider using these targeted questions. They can help you gauge the applicants' understanding and practical application of LINQ, which is crucial for roles such as a C# developer.
- Can you describe what a LINQ query expression is and how it differs from method syntax?
- What is the purpose of the 'let' keyword in a LINQ query?
- How would you perform a grouping operation using LINQ?
- Can you explain how to use LINQ to XML and its advantages?
- What are the differences between 'Select' and 'SelectMany' in LINQ?
- How do you implement sorting with LINQ? Can you give an example?
- What is the significance of 'FirstOrDefault' and when would you use it?
- How can you combine multiple LINQ queries into one?
- Can you explain what a 'Join' is in LINQ and provide an example of its usage?
- What is the use of 'Distinct' in LINQ, and how does it work?
- How do you filter data in a LINQ query? Can you provide a sample?
- What are 'AsEnumerable' and 'AsQueryable' in LINQ and when should you use them?
- Can you discuss the difference between 'Any' and 'All' in LINQ?
- How would you convert a LINQ query result to a list or array?
- What strategies would you use to optimize LINQ queries for performance?
10 intermediate LINQ interview questions and answers to ask mid-tier developers.
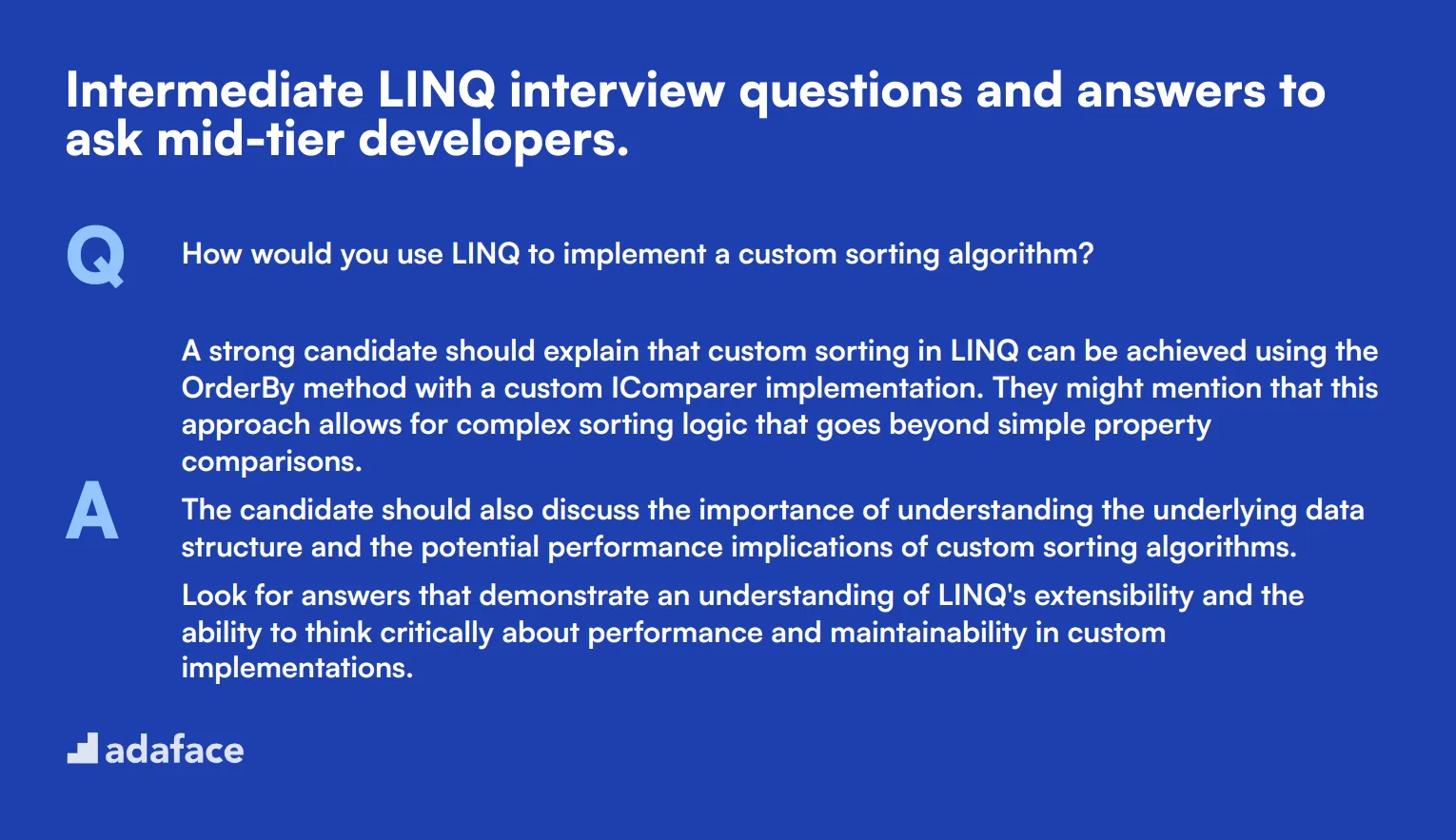
Ready to level up your LINQ interview game? These 10 intermediate questions are perfect for assessing mid-tier developers' understanding of LINQ's nuances. Use them to dig deeper into candidates' knowledge and problem-solving skills, helping you find that perfect fit for your team. Remember, it's not just about the answers—pay attention to their thought process too!
1. How would you use LINQ to implement a custom sorting algorithm?
A strong candidate should explain that custom sorting in LINQ can be achieved using the OrderBy method with a custom IComparer implementation. They might mention that this approach allows for complex sorting logic that goes beyond simple property comparisons.
The candidate should also discuss the importance of understanding the underlying data structure and the potential performance implications of custom sorting algorithms.
Look for answers that demonstrate an understanding of LINQ's extensibility and the ability to think critically about performance and maintainability in custom implementations.
2. Can you explain the concept of lazy evaluation in LINQ and its implications?
Lazy evaluation in LINQ means that query execution is deferred until the results are actually needed. This can lead to improved performance, especially when dealing with large datasets, as only the required elements are processed.
Candidates should mention that lazy evaluation allows for query composition without immediate execution, enabling more efficient operations. They might also discuss potential pitfalls, such as multiple enumeration of the same query leading to repeated execution.
Look for responses that show an understanding of how lazy evaluation impacts query performance and the importance of being mindful of when queries are actually executed in the code.
3. How would you handle null values in a LINQ query without causing exceptions?
Candidates should mention several strategies for handling null values in LINQ queries:
- Using the null-conditional operator (?.) to safely access properties
- Employing the null-coalescing operator (??) to provide default values
- Utilizing the DefaultIfEmpty() method for left outer joins
- Applying Where clauses to filter out null values before further processing
A strong answer would also include a discussion on the importance of null checking in maintaining robust and error-free code, especially when working with data from external sources or databases.
4. Describe a scenario where you would choose to use LINQ to Objects over LINQ to SQL, or vice versa.
A good answer should differentiate between in-memory collections (LINQ to Objects) and database queries (LINQ to SQL):
- LINQ to Objects: Preferred for small to medium-sized in-memory collections, complex operations that benefit from .NET's full feature set, or when working with non-SQL data sources.
- LINQ to SQL: Ideal for large datasets stored in SQL databases, leveraging database engine optimizations, and when needing to minimize memory usage on the application server.
Look for candidates who can articulate the trade-offs between processing data in-memory versus at the database level, showing an understanding of performance considerations and the strengths of each approach.
5. How would you optimize a LINQ query that seems to be performing slowly?
Candidates should discuss several optimization strategies:
- Analyzing the generated SQL (for LINQ to SQL) to identify inefficient queries
- Using appropriate indexes on the database side
- Limiting the data retrieved by using Select to choose only necessary fields
- Applying Where clauses early in the query to reduce the dataset
- Considering pagination for large result sets
- Using compiled queries for frequently executed LINQ to SQL queries
A strong answer would also mention the importance of profiling and measuring performance before and after optimizations to ensure real improvements. Look for candidates who demonstrate a systematic approach to performance tuning and an understanding of both LINQ and database optimizations.
6. Explain the difference between IEnumerable and IQueryable in LINQ.
A good answer should cover the following points:
- IEnumerable operates on in-memory collections and executes queries locally
- IQueryable is designed for remote data sources (like databases) and can translate LINQ queries into the data source's native query language (e.g., SQL)
- IQueryable allows for more efficient querying of large datasets by pushing filtering and sorting operations to the data source
- IEnumerable is simpler and more flexible for in-memory operations
Look for candidates who can explain the performance implications of choosing between IEnumerable and IQueryable, especially in scenarios involving large datasets or remote data sources. They should understand when to use each interface for optimal query performance.
7. How would you use LINQ to implement a simple caching mechanism?
A strong answer should outline a basic caching strategy using LINQ:
- Create a dictionary or similar structure to store cached results
- Use LINQ to check if the desired data is in the cache
- If found, return the cached data; if not, perform the query and store the result in the cache
- Implement a mechanism to invalidate or update cached data as needed
Candidates might also discuss considerations like cache expiration, thread safety for concurrent access, and memory management for large caches. Look for answers that demonstrate an understanding of both LINQ's querying capabilities and practical application in improving application performance.
8. Can you explain how you would use LINQ to implement a basic search functionality?
A good answer should cover the following points:
- Using the Where clause to filter data based on search criteria
- Employing string methods like Contains, StartsWith, or EndsWith for text searches
- Utilizing case-insensitive comparisons when appropriate
- Implementing multi-field searches by combining conditions with logical operators
- Considering performance optimizations for large datasets
Look for candidates who can discuss both simple and more advanced search scenarios, such as fuzzy matching or weighted results. They should also be able to explain how to make the search functionality flexible and easily extendable for future requirements.
9. How would you use LINQ to implement a basic audit trail for data changes?
A strong answer should outline a strategy for tracking data changes using LINQ:
- Create a separate audit table or collection to store change records
- Use LINQ to compare the original and modified objects
- Generate audit entries for each changed property
- Store additional metadata like timestamp, user, and operation type
Candidates might also discuss more advanced concepts like using reflection to automate the comparison process or implementing versioning for complex objects. Look for answers that demonstrate an understanding of data integrity, the importance of audit trails in maintaining data history, and how LINQ can be leveraged to simplify the auditing process.
10. Explain how you would use LINQ to implement a basic data validation system.
A good answer should cover the following points:
- Using LINQ to query data and check for specific conditions
- Implementing validation rules as extension methods or separate functions
- Aggregating validation results into a collection of error messages or a validation object
- Considering both simple property validations and more complex cross-property or cross-entity validations
Look for candidates who can discuss how to make the validation system flexible and extensible. They should also be able to explain how LINQ can be used to create expressive and readable validation rules, potentially integrating with existing validation frameworks or patterns used in .NET development.
10 LINQ questions related to query syntax and methods
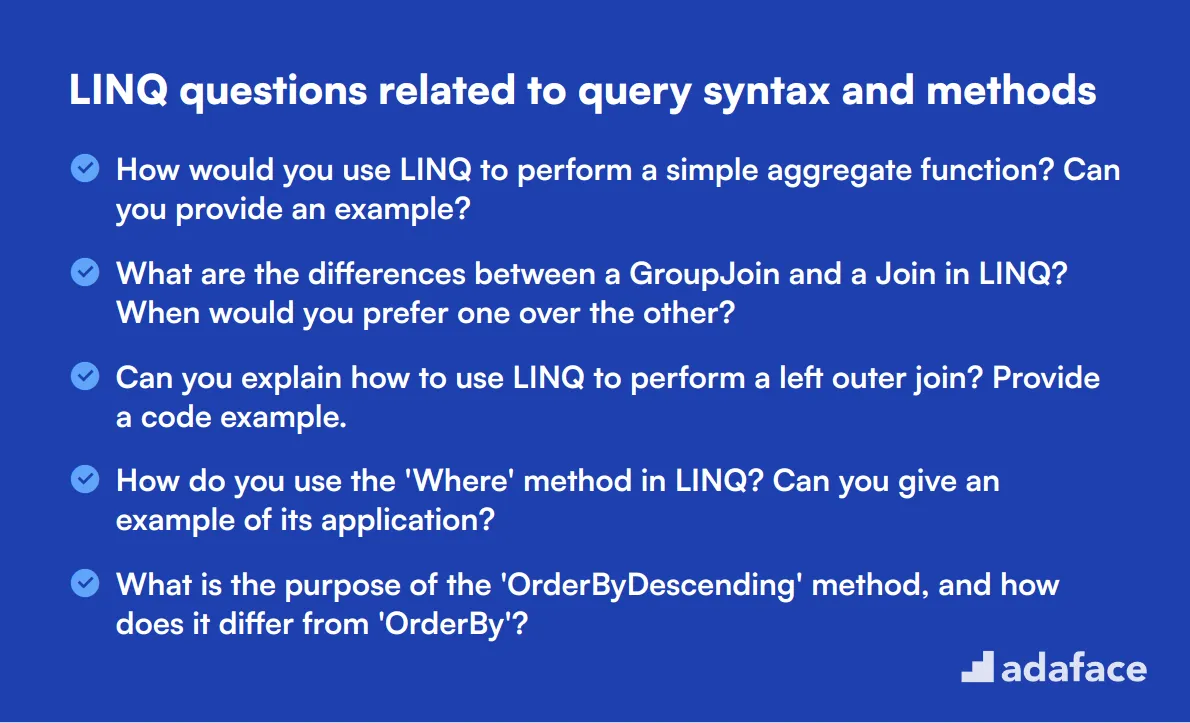
To assess a candidate's practical knowledge of LINQ in .NET applications, consider using this set of questions. They will help you gauge their understanding of query syntax and methods, essential for developers in various roles, such as a software developer.
- How would you use LINQ to perform a simple aggregate function? Can you provide an example?
- What are the differences between a GroupJoin and a Join in LINQ? When would you prefer one over the other?
- Can you explain how to use LINQ to perform a left outer join? Provide a code example.
- How do you use the 'Where' method in LINQ? Can you give an example of its application?
- What is the purpose of the 'OrderByDescending' method, and how does it differ from 'OrderBy'?
- Can you describe how to use the 'Zip' method in LINQ? Provide a use case.
- How can you use LINQ to perform a projection? Can you illustrate with an example?
- What is the significance of the 'Take' and 'Skip' methods in LINQ?
- How would you implement pagination in your LINQ queries?
- Can you explain how to create and use a LINQ expression tree?
9 LINQ interview questions and answers related to deferred execution
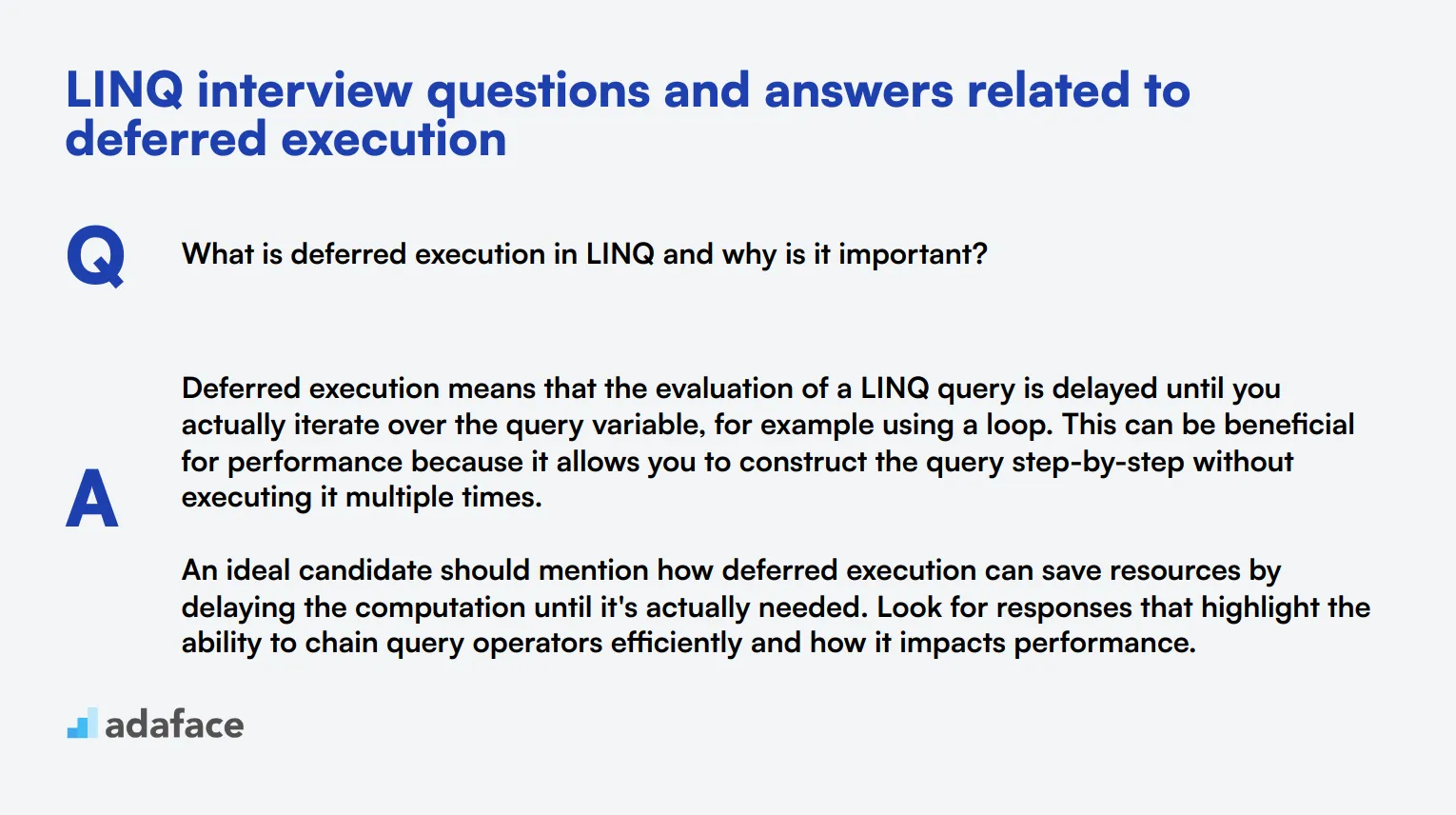
Understanding deferred execution in LINQ can be a game changer for optimizing performance and resource usage in your projects. Use these interview questions to gauge whether your candidates have a solid grasp of this concept and can apply it effectively in real-world scenarios.
1. What is deferred execution in LINQ and why is it important?
Deferred execution means that the evaluation of a LINQ query is delayed until you actually iterate over the query variable, for example using a loop. This can be beneficial for performance because it allows you to construct the query step-by-step without executing it multiple times.
An ideal candidate should mention how deferred execution can save resources by delaying the computation until it's actually needed. Look for responses that highlight the ability to chain query operators efficiently and how it impacts performance.
2. Can you provide an example scenario where deferred execution would be beneficial?
Deferred execution is particularly useful when dealing with large datasets. For instance, if you are filtering and sorting a list of thousands of records, deferred execution ensures that these operations are only performed once you actually need the results.
Look for candidates who can clearly explain how deferred execution reduces unnecessary computations and optimizes resource usage. They should ideally discuss scenarios involving data filtering, transformation, and aggregation.
3. How would you explain the concept of deferred execution to someone unfamiliar with LINQ?
Deferred execution in LINQ means that the query is not run at the point where it is defined but rather when it is iterated over, such as in a loop or by calling a method that forces execution like ToList
. This helps in reducing the immediate overhead and allows for more efficient query composition.
Candidates should keep the explanation simple and relatable, perhaps using an analogy like preparing a shopping list but only going shopping when it's needed. This shows they understand the concept well enough to teach it to someone else.
4. What are some potential pitfalls of deferred execution in LINQ?
One of the pitfalls of deferred execution is that the query might be executed multiple times if iterated multiple times, potentially leading to performance issues. Another issue is that the state of the data source might change between the time the query is defined and when it is executed, leading to unexpected results.
Strong candidates will point out the importance of being mindful about how and when queries are executed. They might suggest using methods like ToList
or ToArray
to force immediate execution when necessary.
5. How can deferred execution impact the debugging process?
Deferred execution can make debugging a bit tricky because the query is not executed when it is defined. This means that any issues related to the data or the query logic will only become apparent at the point of iteration.
Candidates should be able to discuss strategies for mitigating this, such as using immediate execution methods for debugging purposes or carefully checking the query logic. Look for candidates who understand the nuances of debugging deferred execution scenarios.
6. Why might you choose to use deferred execution in a production environment?
Deferred execution is useful in a production environment because it allows for more flexible and efficient data processing. By deferring the execution, you can construct more complex queries without incurring the cost of multiple round-trips to the database or other data sources.
Ideal candidates will discuss how deferred execution can lead to better performance and resource management in production applications. They should also mention the importance of understanding when to force execution to avoid potential pitfalls.
7. Can you explain how deferred execution interacts with memory usage?
Deferred execution can help manage memory usage more effectively by avoiding the immediate allocation of memory for query results. The memory is only used when the results are actually needed, which can be especially beneficial for large datasets.
Look for candidates who can articulate how deferred execution can lead to more efficient memory management and reduced memory footprint. They should ideally provide examples or scenarios where this would be particularly beneficial.
8. What are the advantages of combining deferred execution with lazy loading?
Combining deferred execution with lazy loading can lead to significant performance improvements. Deferred execution ensures that queries are only evaluated when needed, while lazy loading ensures that related data is only loaded when accessed, reducing the initial load time.
Candidates should highlight how these two concepts together can optimize both data retrieval and application performance. Look for explanations that show a deep understanding of how to leverage these techniques in real-world applications.
9. How would you identify if a LINQ query is being executed immediately or deferred?
To determine if a LINQ query is being executed immediately or deferred, you can check if the query is being iterated over or if methods like ToList
, ToArray
, or First
are called, which force immediate execution.
Candidates should be able to identify the signs of immediate versus deferred execution and discuss why it is important to understand this distinction. Look for clarity in their explanation and practical examples.
7 situational LINQ interview questions with answers for hiring top developers
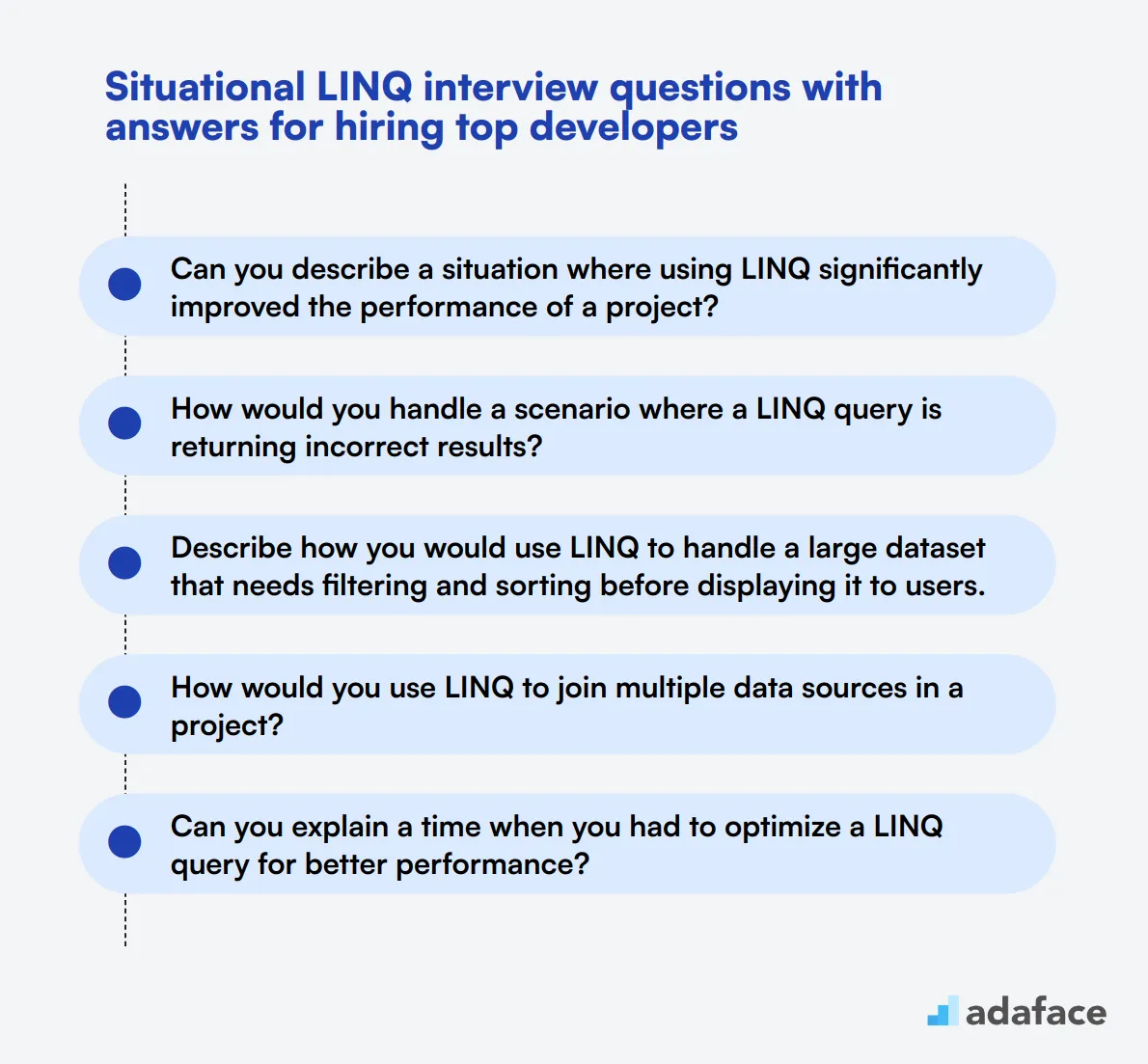
To evaluate candidates' practical understanding of LINQ in real-world scenarios, consider these 7 situational interview questions. These questions are designed to gauge their problem-solving abilities and ensure they can effectively apply LINQ to various challenges they might encounter on the job.
1. Can you describe a situation where using LINQ significantly improved the performance of a project?
Using LINQ can streamline complex queries and operations, leading to more efficient code. For instance, in a project where data from multiple sources needed to be merged and analyzed, LINQ allowed for concise and readable queries, reducing the overall development time and improving performance.
Look for candidates who can provide specific examples, detailing the problem, the LINQ solution they implemented, and the measurable improvements in performance. This showcases their ability to apply LINQ effectively and understand its impact on performance.
2. How would you handle a scenario where a LINQ query is returning incorrect results?
In such a scenario, I would first verify the data sources and the logic of the LINQ query. It’s crucial to ensure that the query accurately reflects the intended operations. Debugging tools and step-by-step examination of the query can help identify where things are going wrong.
A strong candidate will mention methods like breaking down the query into smaller parts, checking for logical errors, and using debugging tools to trace the issue. They should also highlight the importance of testing and validating data at each step.
3. Describe how you would use LINQ to handle a large dataset that needs filtering and sorting before displaying it to users.
When dealing with large datasets, it's important to use efficient queries to filter and sort data. LINQ’s ‘Where’ method can be used for filtering, and ‘OrderBy’ or ‘OrderByDescending’ for sorting. By combining these methods, you can create a query that meets the requirements without loading unnecessary data into memory.
Candidates should demonstrate an understanding of how to structure LINQ queries for performance, mentioning techniques like deferred execution to avoid loading large datasets until necessary. They should also be aware of potential pitfalls like memory usage and query optimization.
4. How would you use LINQ to join multiple data sources in a project?
LINQ provides powerful join operations like ‘Join’ and ‘GroupJoin’ to combine data from different sources. For instance, if you have customer and order datasets, you can join them on a common key, such as customer ID, to analyze customer orders.
Look for candidates who can explain the process and rationale behind using different join methods. They should discuss the importance of matching keys and ensuring data integrity, as well as any challenges they faced and how they overcame them.
5. Can you explain a time when you had to optimize a LINQ query for better performance?
Optimizing LINQ queries often involves reducing the complexity of the query, making sure it executes efficiently. In one project, a query was taking too long to execute because it was processing unnecessary data. By refining the query to fetch only required fields and using methods like ‘AsEnumerable’, we improved performance significantly.
Strong responses should include specific details about the initial problem, the optimization techniques used, and the resultant performance gains. This helps assess the candidate's ability to troubleshoot and enhance LINQ operations.
6. How would you use LINQ to ensure the integrity of data operations in a multi-user environment?
In a multi-user environment, it’s crucial to handle data operations with care to maintain data integrity. LINQ can be combined with transaction management to ensure that operations are atomic, consistent, isolated, and durable (ACID). This might involve using LINQ within a transaction scope to ensure that multiple operations complete successfully before committing changes.
Candidates should highlight their understanding of transactional operations and how LINQ can be used in conjunction with other tools to ensure data integrity. They should also discuss potential challenges, such as handling concurrency issues, and how they addressed them.
7. Discuss how you would use LINQ to implement a dynamic search feature in an application.
A dynamic search feature can be implemented using LINQ by constructing queries based on user input. This involves creating flexible LINQ queries that can adapt to different search criteria without hardcoding the conditions. For example, using predicate builders or dynamic query libraries can help in building such queries.
Look for candidates who can discuss their approach to building dynamic and flexible LINQ queries. They should mention any libraries or tools they used and how they handled complex or changing search criteria efficiently.
Which LINQ skills should you evaluate during the interview phase?
Assessing every aspect of a candidate's skill set in a single interview is impractical. However, for a skill like LINQ, there are key areas that provide a substantial indication of the candidate's proficiency. Here are some core skills to evaluate to ensure the candidate's competence in LINQ.
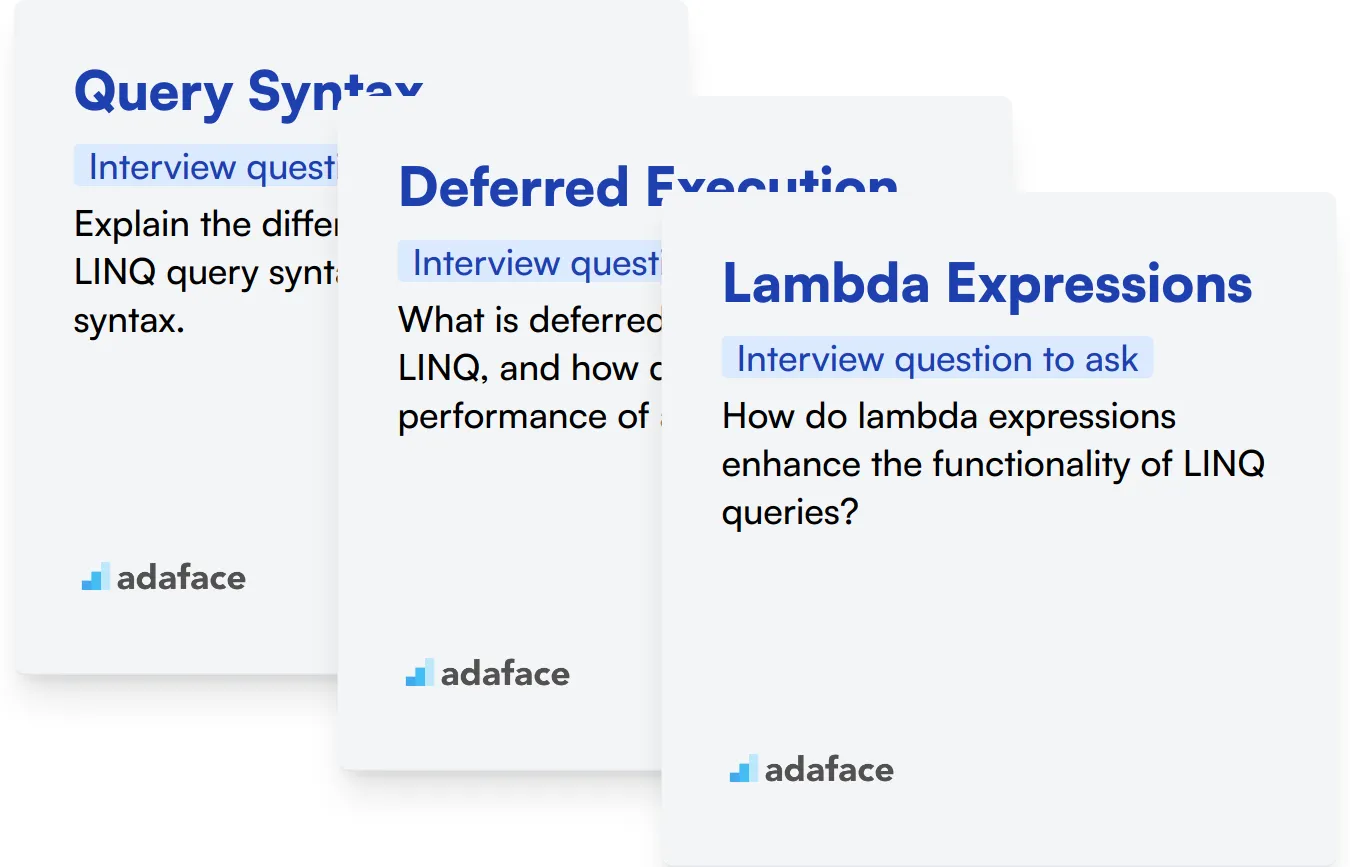
Query Syntax
Understanding LINQ query syntax is essential as it forms the foundation of writing LINQ queries. Proficiency in query syntax allows developers to write more readable and maintainable code, which is crucial for any project.
You can use an assessment test that includes relevant MCQs on query syntax. Our LINQ online test is specifically designed to evaluate this skill effectively.
In addition to assessments, consider asking targeted questions to understand the candidate's grasp of LINQ query syntax. For instance:
Explain the differences between LINQ query syntax and method syntax.
Look for a clear understanding of both syntaxes and their use cases. The candidate should be able to provide examples and discuss scenarios where one might be more advantageous than the other.
Deferred Execution
Deferred execution is a core concept in LINQ that determines when a query is executed. Understanding this concept is crucial as it affects performance and resource management in applications.
You can use an assessment test that includes relevant MCQs on deferred execution. Our LINQ online test covers this topic comprehensively.
To further evaluate this skill, you can ask specific questions during the interview. For example:
What is deferred execution in LINQ, and how does it impact the performance of a query?
Look for an explanation that covers the basics of deferred execution, including how and when LINQ queries are executed. The candidate should also discuss performance implications and provide examples.
Lambda Expressions
Lambda expressions are integral to LINQ as they provide a concise way to represent anonymous methods. Mastery of lambda expressions enables developers to write more expressive and functional code.
You can use an assessment test that includes relevant MCQs on lambda expressions. Our LINQ online test includes questions to assess this skill precisely.
Ask targeted questions during the interview to gauge the candidate's understanding of lambda expressions. For instance:
How do lambda expressions enhance the functionality of LINQ queries?
Evaluate whether the candidate can explain and provide examples of using lambda expressions within LINQ queries. They should be able to illustrate how lambda expressions simplify and enhance code readability and functionality.
Hire the Best LINQ Experts with Adaface
If you are looking to hire someone with LINQ skills, you need to ensure they have the skills accurately. This means thorough assessments are necessary.
The best way to do this is to use skill tests. You can find relevant tests at LINQ Online Test and C# Online Test.
Once you use these tests, you can shortlist the best applicants and call them for interviews. This ensures you are interviewing candidates who already have demonstrated their skills.
To get started, you can sign up at Adaface Dashboard or explore more on our test library.
LINQ Online Test
Download LINQ interview questions template in multiple formats
LINQ Interview Questions FAQs
LINQ (Language Integrated Query) is a set of features in .NET that adds native data querying capabilities to .NET languages like C# and VB.NET.
LINQ simplifies data manipulation, improves code readability, and enhances productivity when working with various data sources in .NET applications.
Use a combination of theoretical questions, practical coding exercises, and situational problems to evaluate their understanding and application of LINQ concepts.
Advanced topics include deferred execution, query optimization, custom LINQ providers, and integrating LINQ with complex data structures or databases.
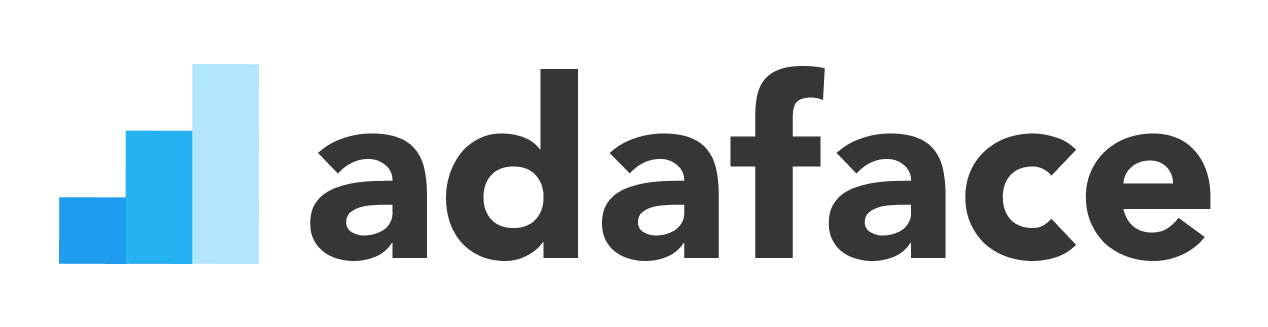
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
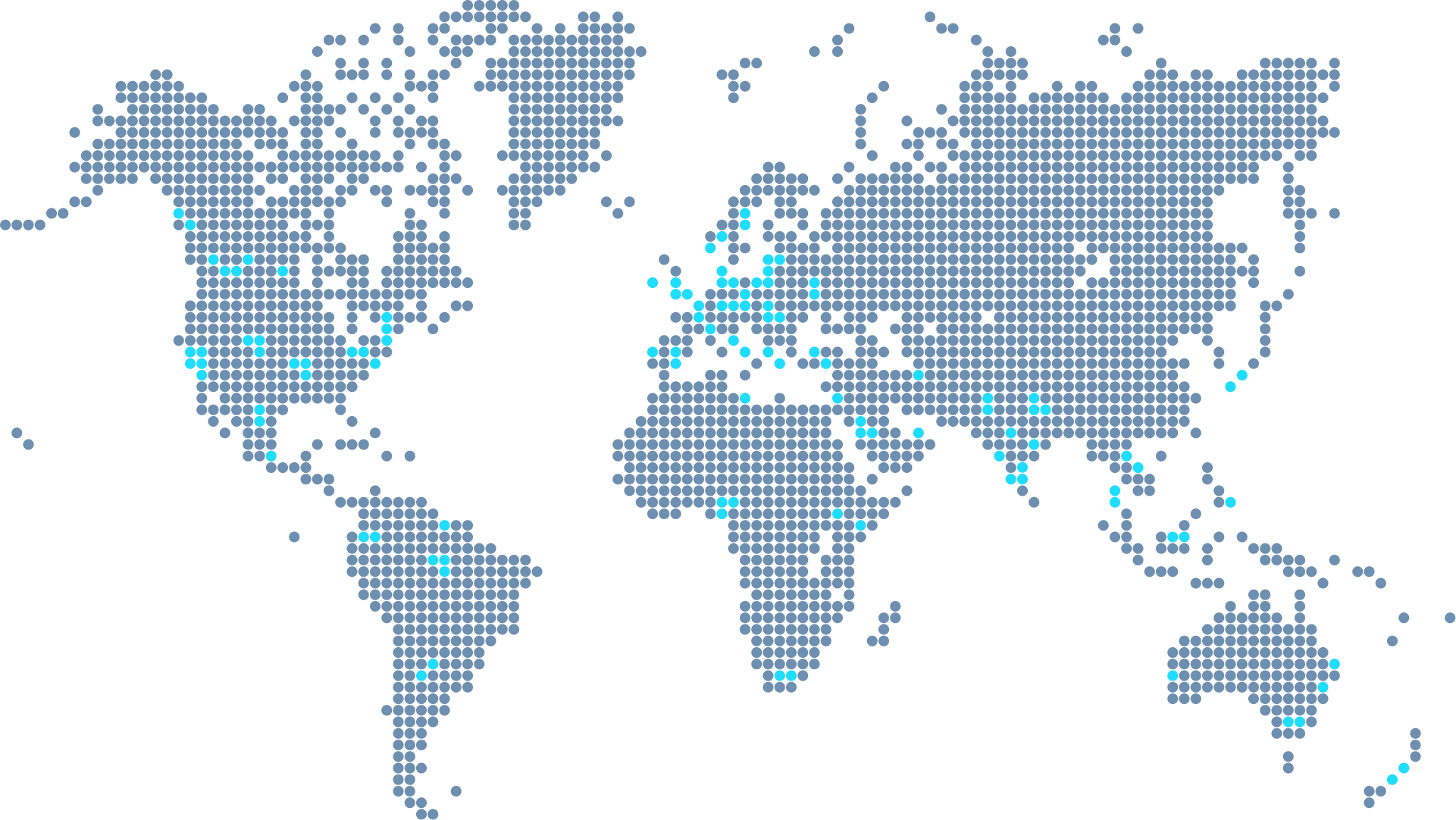
