Recruiting the right PHP developers can be challenging, especially with the rapidly evolving landscape of web development. By asking the right PHP interview questions, you ensure that you are hiring proficient candidates who can contribute meaningfully to your projects.
This blog post provides a comprehensive list of PHP interview questions tailored to different experience levels and focus areas. From initiating the interview to understanding technical definitions and situational questions, we cover all bases to help you evaluate your candidates effectively.
By leveraging these questions, you can streamline your interview process and identify top talent for your team. For enhanced accuracy, consider using our PHP online test before conducting interviews.
Table of contents
10 PHP interview questions to initiate the interview
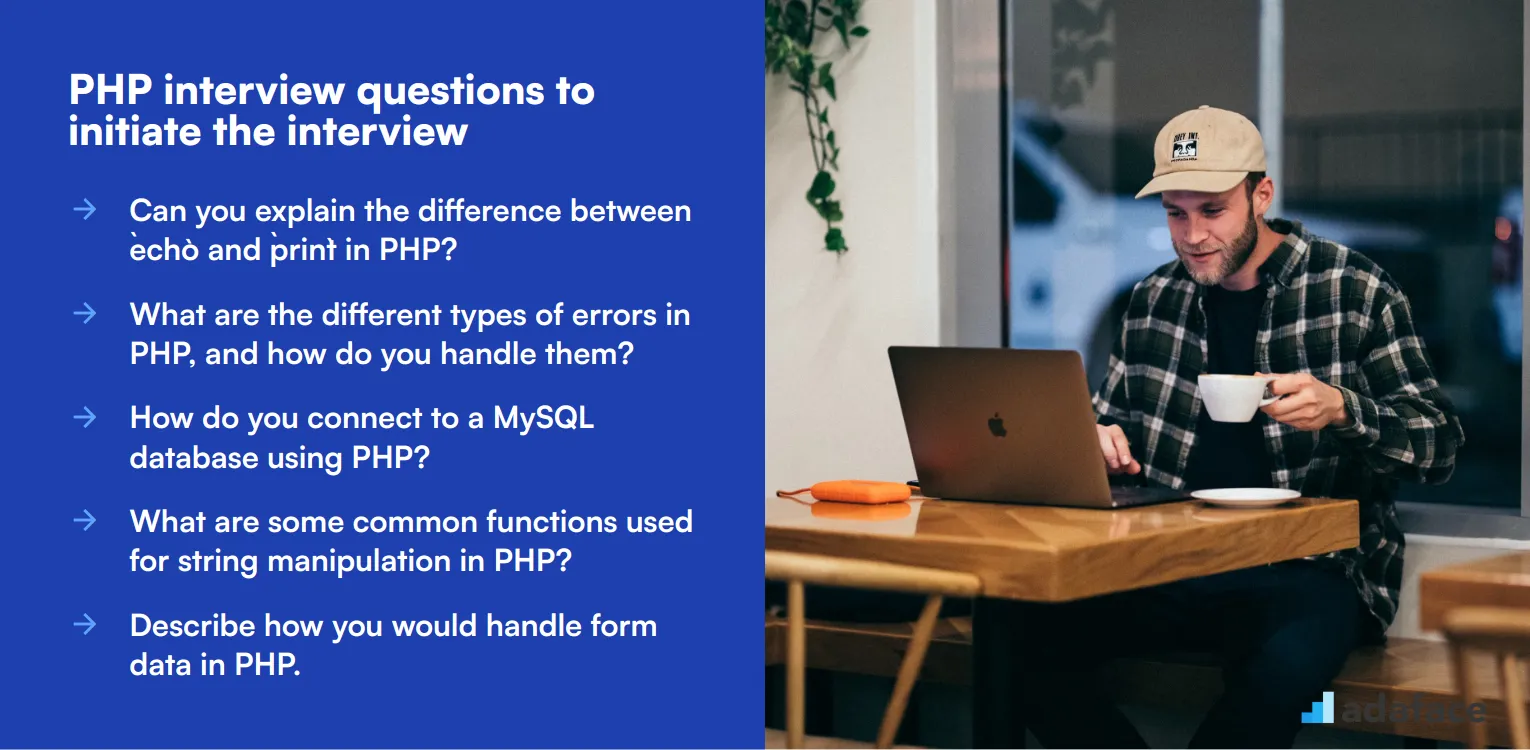
To determine whether your applicants have the right skills to succeed as a PHP developer, use these interview questions to initiate the conversation. These questions will help you assess fundamental knowledge and practical understanding, ensuring you hire the best fit for your team. For more insights on what to look for, check out this PHP developer job description.
- Can you explain the difference between `echo` and `print` in PHP?
- What are the different types of errors in PHP, and how do you handle them?
- How do you connect to a MySQL database using PHP?
- What are some common functions used for string manipulation in PHP?
- Describe how you would handle form data in PHP.
- Can you explain the concept of sessions and cookies in PHP?
- What is the purpose of the `composer.json` file in a PHP project?
- How do you implement error handling in PHP?
- Describe the Model-View-Controller (MVC) architecture and how it is implemented in PHP.
- What are traits in PHP, and how are they used?
9 PHP interview questions and answers to evaluate junior developers
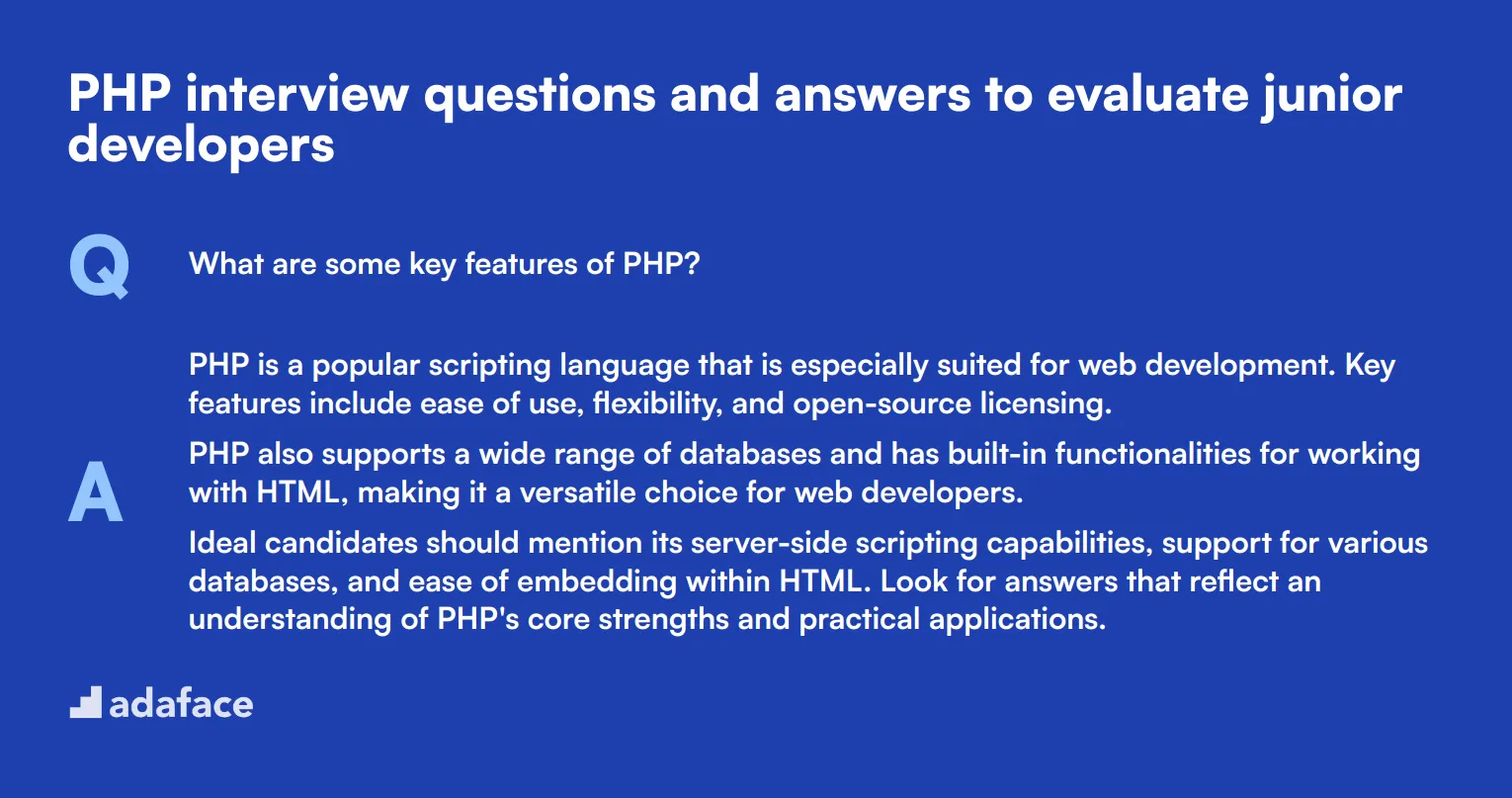
If you're looking to evaluate junior PHP developers, these 9 interview questions will help you gauge both their understanding of fundamental concepts and their problem-solving abilities. Use this list during your interviews to identify candidates with the right skills and mindset for your team.
1. What are some key features of PHP?
PHP is a popular scripting language that is especially suited for web development. Key features include ease of use, flexibility, and open-source licensing.
PHP also supports a wide range of databases and has built-in functionalities for working with HTML, making it a versatile choice for web developers.
Ideal candidates should mention its server-side scripting capabilities, support for various databases, and ease of embedding within HTML. Look for answers that reflect an understanding of PHP's core strengths and practical applications.
2. How do you secure a PHP application?
Securing a PHP application involves a variety of practices, such as input validation, using prepared statements to prevent SQL injection, and implementing proper error handling.
Additional measures include using HTTPS for secure data transmission and regularly updating PHP and its dependencies to protect against known vulnerabilities.
Look for candidates who mention multiple layers of security practices and show an understanding of common threats and how to mitigate them. Follow up by asking for examples from their past experience.
3. Can you explain the purpose and use of PHP frameworks?
PHP frameworks provide a structured and efficient way to build web applications. They offer reusable code and components, reducing development time and improving code maintainability.
Popular PHP frameworks like Laravel and Symfony come with built-in tools for tasks like routing, authentication, and caching, which help standardize development processes.
Ideal candidates should highlight the benefits of using frameworks, such as faster development and better code organization. They should also mention specific frameworks they have experience with and the projects they used them in.
4. What are some common methods for debugging PHP code?
Common methods for debugging PHP code include using error logs, employing debugging tools like Xdebug, and inserting var_dump
or print_r
statements to inspect variables.
Another effective method is to use integrated development environments (IDEs) with built-in debugging functionalities, which can help trace and resolve issues more efficiently.
Candidates should demonstrate familiarity with different debugging techniques and tools. Look for responses that highlight their methodical approach to identifying and fixing bugs.
5. How do you manage dependencies in a PHP project?
In PHP projects, dependencies are usually managed using Composer, a dependency management tool that allows developers to declare the libraries their project depends on.
Composer automatically handles the installation and updates of those libraries, ensuring that the correct versions are used, which simplifies project maintenance.
Look for candidates who mention Composer and can discuss their experience with it. Follow up by asking how they handle version conflicts and keep dependencies up to date.
6. What is the difference between include() and require() in PHP?
Both include()
and require()
functions are used to include files in PHP scripts. The key difference is how they handle errors.
include()
will emit a warning if the file cannot be found but will continue executing the script, whereas require()
will produce a fatal error and halt the script execution.
Ideal candidates should clearly explain the difference and suggest scenarios where one might be more appropriate than the other. Look for practical examples from their experience.
7. How do you handle file uploads in PHP?
Handling file uploads in PHP involves using the $_FILES
superglobal array to retrieve file information and then moving the uploaded file to a designated directory using the move_uploaded_file()
function.
It's essential to validate the file type and size to ensure security and prevent malicious files from being uploaded.
Candidates should describe a clear process for handling file uploads and emphasize the importance of validation and security measures. Look for detailed explanations and examples from past projects.
8. Can you explain the concept of namespaces in PHP?
Namespaces in PHP are a way to encapsulate items such as classes, interfaces, functions, and constants. This helps avoid name collisions between different libraries or parts of a project.
Namespaces allow developers to organize code better and make it easier to maintain, especially in large applications with multiple components.
Look for candidates who can explain the practical benefits of namespaces and provide examples of how they've used them in their projects. Follow up by asking about specific challenges they faced and how they addressed them.
9. What are the advantages of using Object-Oriented Programming (OOP) in PHP?
Object-Oriented Programming (OOP) in PHP promotes code reusability, modularity, and easier maintenance. By organizing code into classes and objects, developers can create more organized and manageable applications.
OOP also allows for encapsulation, inheritance, and polymorphism, which provide powerful ways to extend and enhance functionality without modifying existing code.
Candidates should discuss the benefits of OOP and provide examples of how they've applied OOP principles in their projects. Look for an understanding of how OOP can improve code quality and development efficiency.
18 intermediate PHP interview questions and answers to ask mid-tier developers.
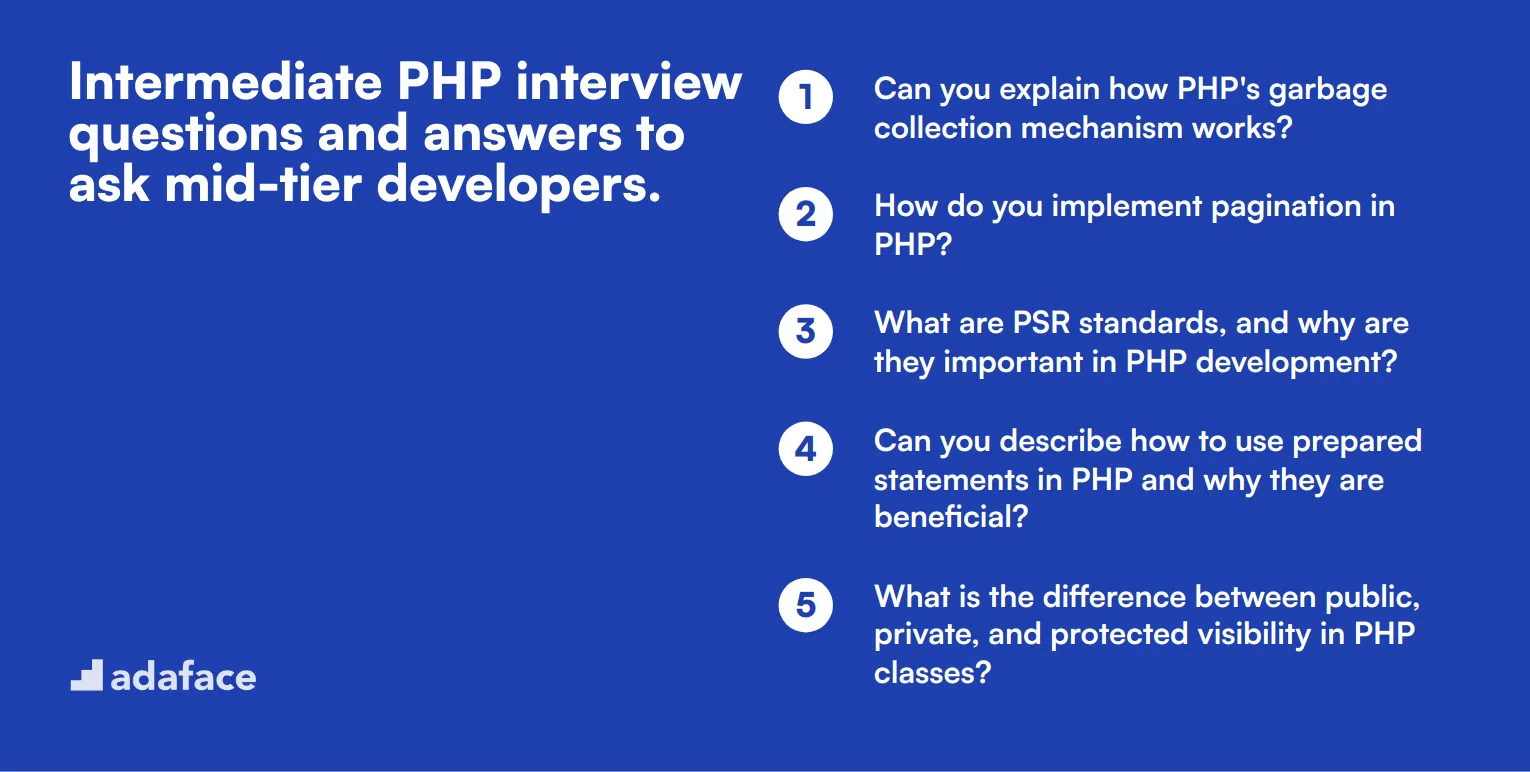
To evaluate if your candidates have the necessary skills for mid-tier PHP development, use these carefully curated PHP interview questions. These questions will help you gauge their technical depth, problem-solving abilities, and real-world application of PHP concepts.
- Can you explain how PHP's garbage collection mechanism works?
- How do you implement pagination in PHP?
- What are PSR standards, and why are they important in PHP development?
- Can you describe how to use prepared statements in PHP and why they are beneficial?
- What is the difference between public, private, and protected visibility in PHP classes?
- How does PHP handle type juggling, and what are some potential pitfalls?
- Explain how to use cURL in PHP to make HTTP requests.
- How do you implement caching in a PHP application?
- Can you describe the difference between GET and POST methods in form submission and when to use each?
- What are anonymous functions (closures) in PHP and how are they used?
- How would you handle large file uploads in PHP?
- Can you explain how to use the PHP Data Objects (PDO) extension for database interactions?
- What are the benefits and drawbacks of using PHP for web development?
- How do you handle and validate user input in PHP?
- What are some common security risks in PHP applications, and how do you mitigate them?
- Can you explain the difference between static and dynamic methods in PHP?
- How do you implement and use interfaces in PHP?
- Can you describe the SPL (Standard PHP Library) and its significance?
8 PHP interview questions and answers related to technical definitions
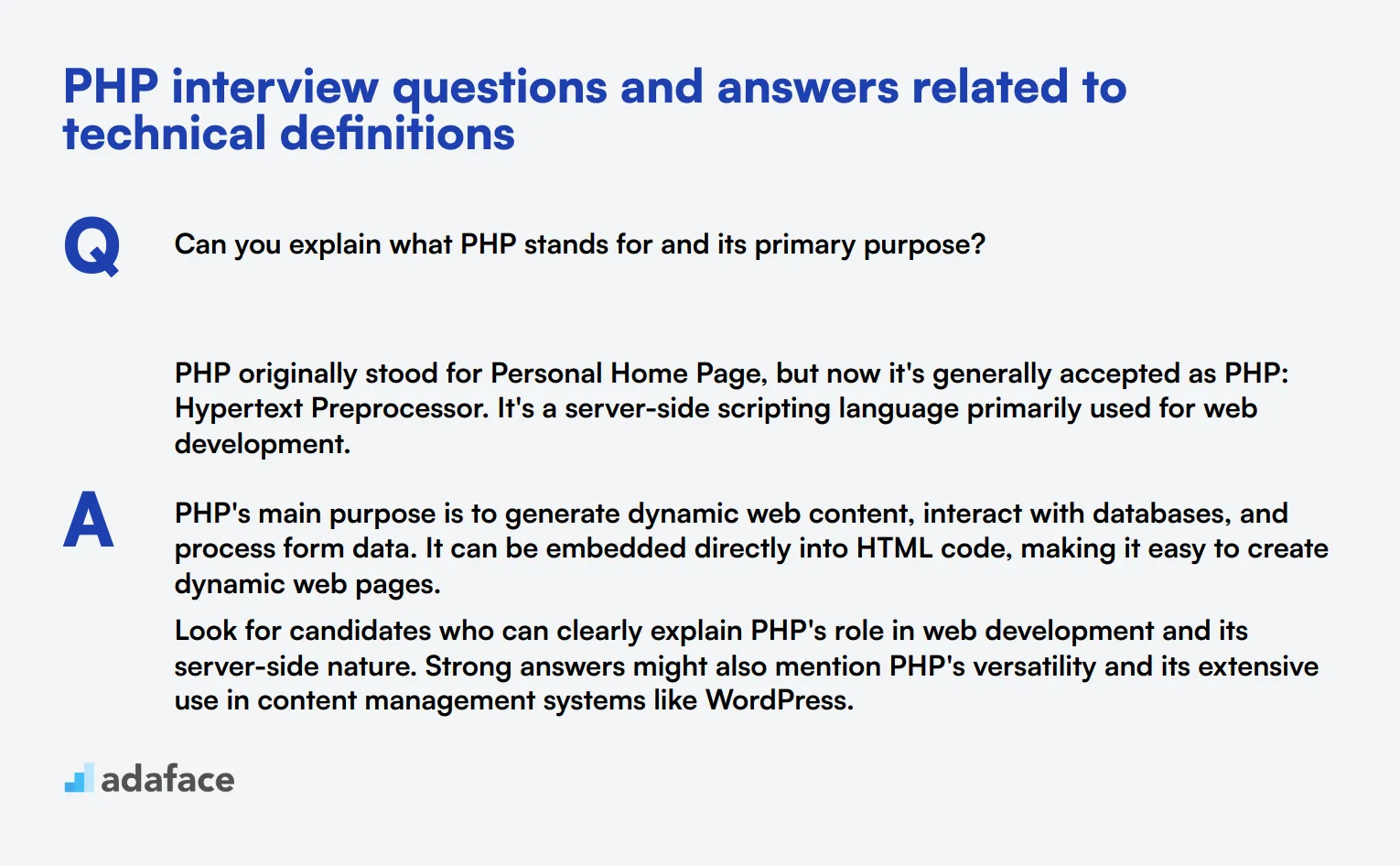
When interviewing for PHP developers, it's crucial to assess their understanding of technical concepts. These questions will help you gauge candidates' knowledge of PHP fundamentals and best practices. Use them to spark discussions and evaluate how well potential hires can explain complex ideas in simple terms – a valuable skill for any PHP developer.
1. Can you explain what PHP stands for and its primary purpose?
PHP originally stood for Personal Home Page, but now it's generally accepted as PHP: Hypertext Preprocessor. It's a server-side scripting language primarily used for web development.
PHP's main purpose is to generate dynamic web content, interact with databases, and process form data. It can be embedded directly into HTML code, making it easy to create dynamic web pages.
Look for candidates who can clearly explain PHP's role in web development and its server-side nature. Strong answers might also mention PHP's versatility and its extensive use in content management systems like WordPress.
2. What is the difference between single-quoted and double-quoted strings in PHP?
In PHP, single-quoted and double-quoted strings have different behaviors when it comes to variable interpolation and escape sequences:
- Single-quoted strings are treated almost literally. Variables and escape sequences are not parsed (except for ' and \).
- Double-quoted strings parse variables and escape sequences. This means you can include variable values directly in the string.
A good candidate should be able to explain this difference clearly and might also mention that double-quoted strings can be slightly slower to process due to the extra parsing involved.
3. Can you explain what a PHP session is and how it works?
A PHP session is a way to store information about a user across multiple pages. It allows developers to create personalized user experiences and maintain state in web applications.
Sessions work by creating a unique session ID for each user when they visit a website. This ID is usually stored in a cookie on the user's browser. On the server, PHP creates a file for each session where data can be stored and retrieved.
Look for candidates who can explain the concept clearly and mention key functions like session_start() and $_SESSION. They might also discuss session security considerations or alternatives like JWT for stateless applications.
4. What is the purpose of the 'finally' block in exception handling?
The 'finally' block in PHP exception handling is used to define a piece of code that will be executed regardless of whether an exception was thrown or caught. It's typically used for cleanup operations that must be performed under all circumstances.
For example, you might use a 'finally' block to close a database connection or file handle, ensuring that resources are properly released even if an error occurs.
A strong candidate should be able to explain that the 'finally' block provides a way to guarantee that certain code will always run, improving resource management and code reliability. They might also mention that 'finally' was introduced in PHP 5.5, showing awareness of language evolution.
5. Can you explain what a PHP trait is and when you might use one?
A trait in PHP is a mechanism for code reuse in single inheritance languages. It allows developers to reuse sets of methods freely in several independent classes living in different class hierarchies.
Traits are particularly useful when you want to include the same functionality in multiple classes without using inheritance. They help solve the limitations of single inheritance and allow for more flexible code organization.
Look for candidates who can explain traits clearly and provide examples of when they might be useful. Strong answers might discuss how traits differ from interfaces or abstract classes, or mention potential issues like naming conflicts and how to resolve them.
6. What is the difference between `isset()` and `empty()` in PHP?
isset()
and empty()
are both PHP functions used for variable testing, but they have different behaviors:
isset()
checks if a variable is set and is not NULL. It returns true if the variable exists and has any value other than NULL, false otherwise.
empty()
checks if a variable is empty. It returns false if the variable contains a non-empty, non-zero value. It considers "" (empty string), 0, "0", NULL, FALSE, array(), and unset variables as empty.
A good candidate should be able to explain these differences clearly and might also mention that empty()
is generally considered more lenient in its checks. They could also discuss scenarios where one might be preferable over the other.
7. Can you explain what a PHP namespace is and why it's useful?
A namespace in PHP is a way of encapsulating items to avoid name conflicts between libraries, classes, functions, and constants. It's particularly useful in large projects or when using multiple libraries.
Namespaces provide a hierarchical way to organize and group related classes, interfaces, functions, and constants. They help prevent naming collisions and make code more modular and maintainable.
Look for candidates who can clearly explain the concept and its benefits. Strong answers might include examples of how to declare and use namespaces, mention the use of the use
keyword for importing, or discuss how namespaces relate to autoloading in modern PHP development.
8. What is the purpose of the `__construct()` method in PHP classes?
The __construct()
method in PHP is a special method called a constructor. It's automatically called when an object is created from a class. Its primary purpose is to initialize the object's properties or perform any setup the object needs before being used.
Constructors can accept parameters, allowing you to set initial values for object properties at the time of creation. They're a key part of object-oriented programming in PHP, enabling more flexible and reusable code.
A strong candidate should be able to explain the role of constructors clearly and might also mention related concepts like destructors (__destruct()
). They could discuss best practices for constructor use or explain how constructors work with inheritance.
12 PHP interview questions about processes and tasks
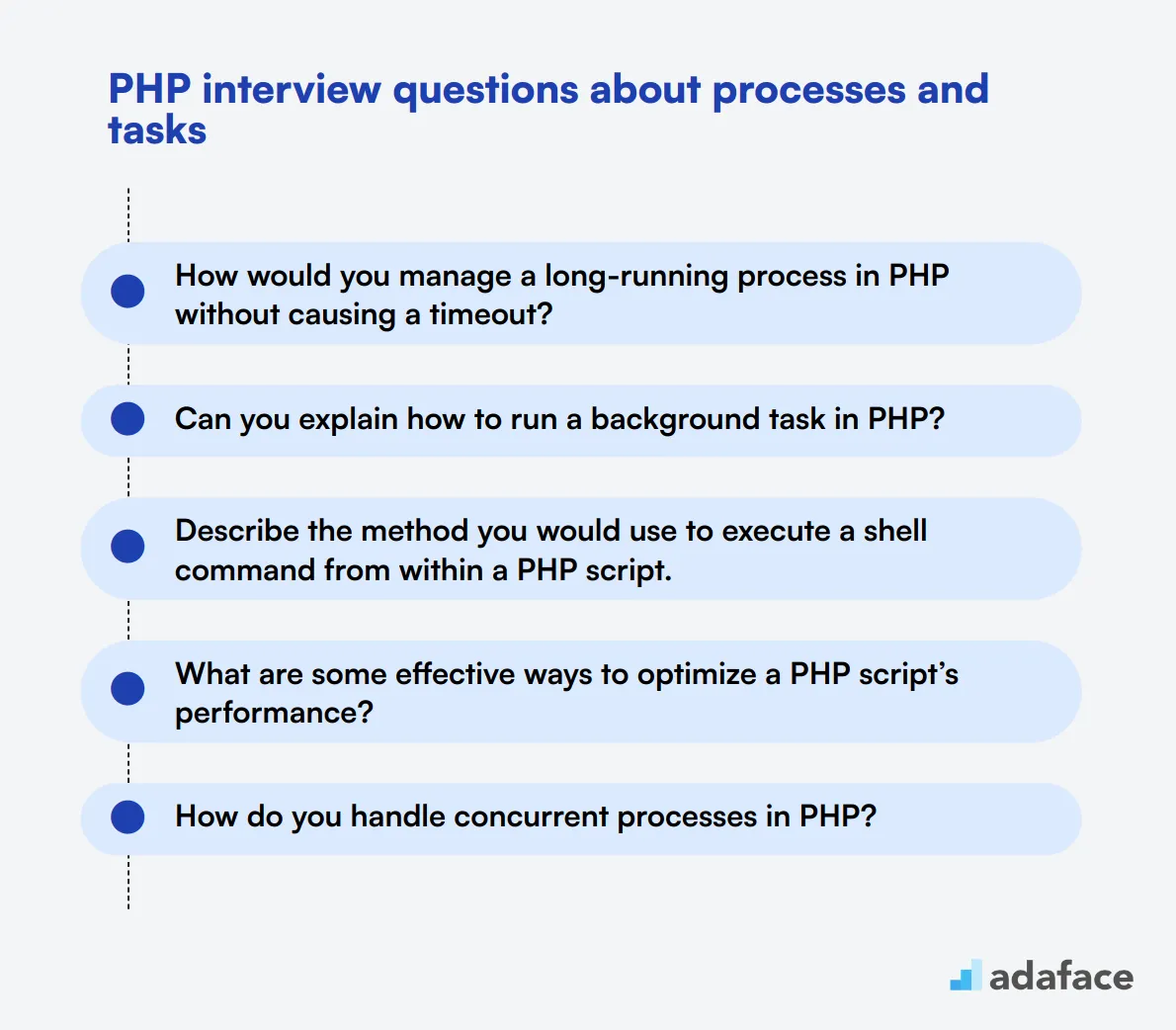
To determine whether your applicants have the right skills to handle processes and tasks with PHP, ask them some of these practical PHP interview questions. These questions are designed to gauge their experience and problem-solving abilities in real-world scenarios, ensuring they can effectively manage the responsibilities of a PHP Developer.
- How would you manage a long-running process in PHP without causing a timeout?
- Can you explain how to run a background task in PHP?
- Describe the method you would use to execute a shell command from within a PHP script.
- What are some effective ways to optimize a PHP script’s performance?
- How do you handle concurrent processes in PHP?
- Can you explain how to use cron jobs with PHP?
- Describe how you would monitor and log PHP processes for debugging and performance analysis.
- How do you implement task scheduling in a PHP application?
- What strategies would you use to handle asynchronous tasks in PHP?
- How can you ensure that a PHP script can handle multiple tasks simultaneously?
- Can you describe a method for executing parallel tasks in PHP?
- How would you manage resource-intensive tasks within a PHP application?
10 situational PHP interview questions for hiring top developers
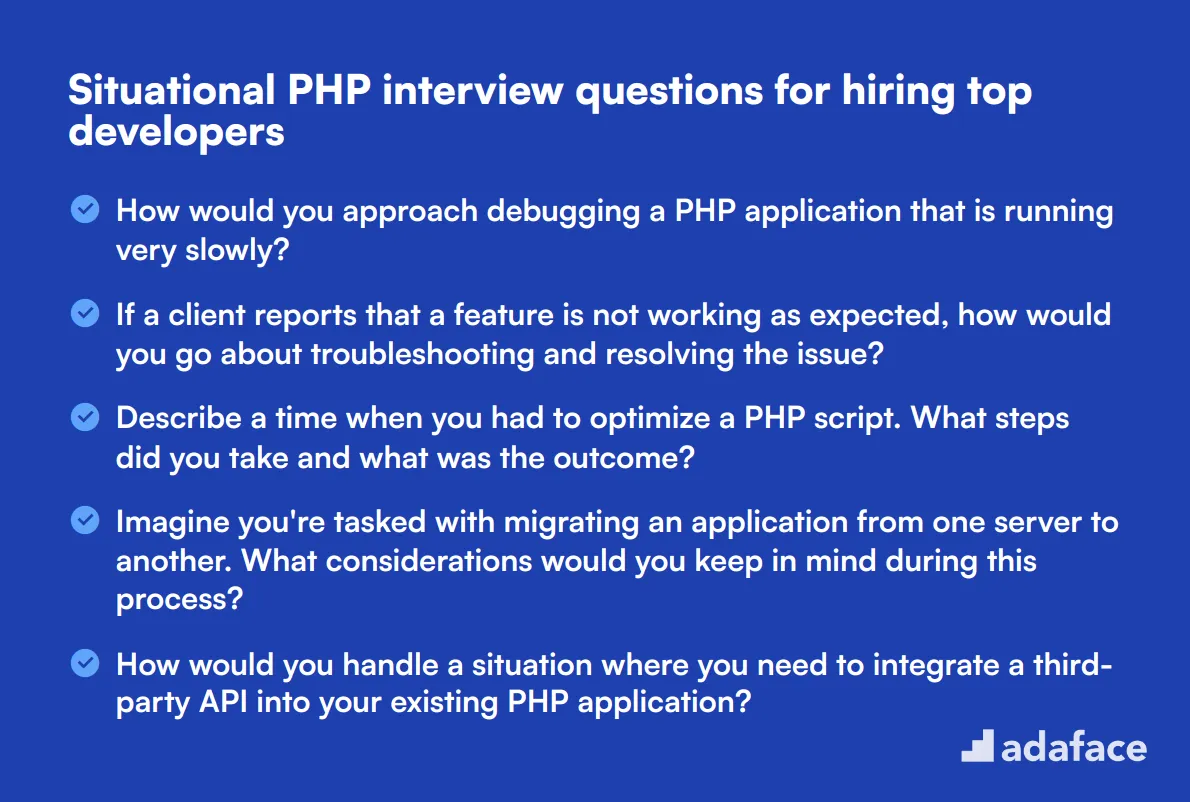
To effectively evaluate a candidate's problem-solving abilities and practical knowledge in PHP, consider using these situational questions. They will help you gauge how well applicants can apply their skills in real-world scenarios, making it easier to identify top talent for your development team. For more detailed insights on the skills required for a PHP developer, check out our job description.
- How would you approach debugging a PHP application that is running very slowly?
- If a client reports that a feature is not working as expected, how would you go about troubleshooting and resolving the issue?
- Describe a time when you had to optimize a PHP script. What steps did you take and what was the outcome?
- Imagine you're tasked with migrating an application from one server to another. What considerations would you keep in mind during this process?
- How would you handle a situation where you need to integrate a third-party API into your existing PHP application?
- What steps would you take if you need to add new functionality to a legacy PHP application without breaking existing features?
- If you discover a security vulnerability in your PHP application, what immediate actions would you take to address it?
- Describe how you would implement a feature that requires real-time data updating in a PHP application.
- If a team member disagrees with your proposed solution to a problem, how would you handle that situation?
- How would you ensure that your PHP application can scale effectively as user demand increases?
Which PHP skills should you evaluate during the interview phase?
During the interview phase, it's important to remember that you can't assess every aspect of a candidate's skill set in a single session. However, specific PHP skills are essential indicators of a developer's capabilities and potential fit within your team. Evaluating these core skills can help you make informed decisions.
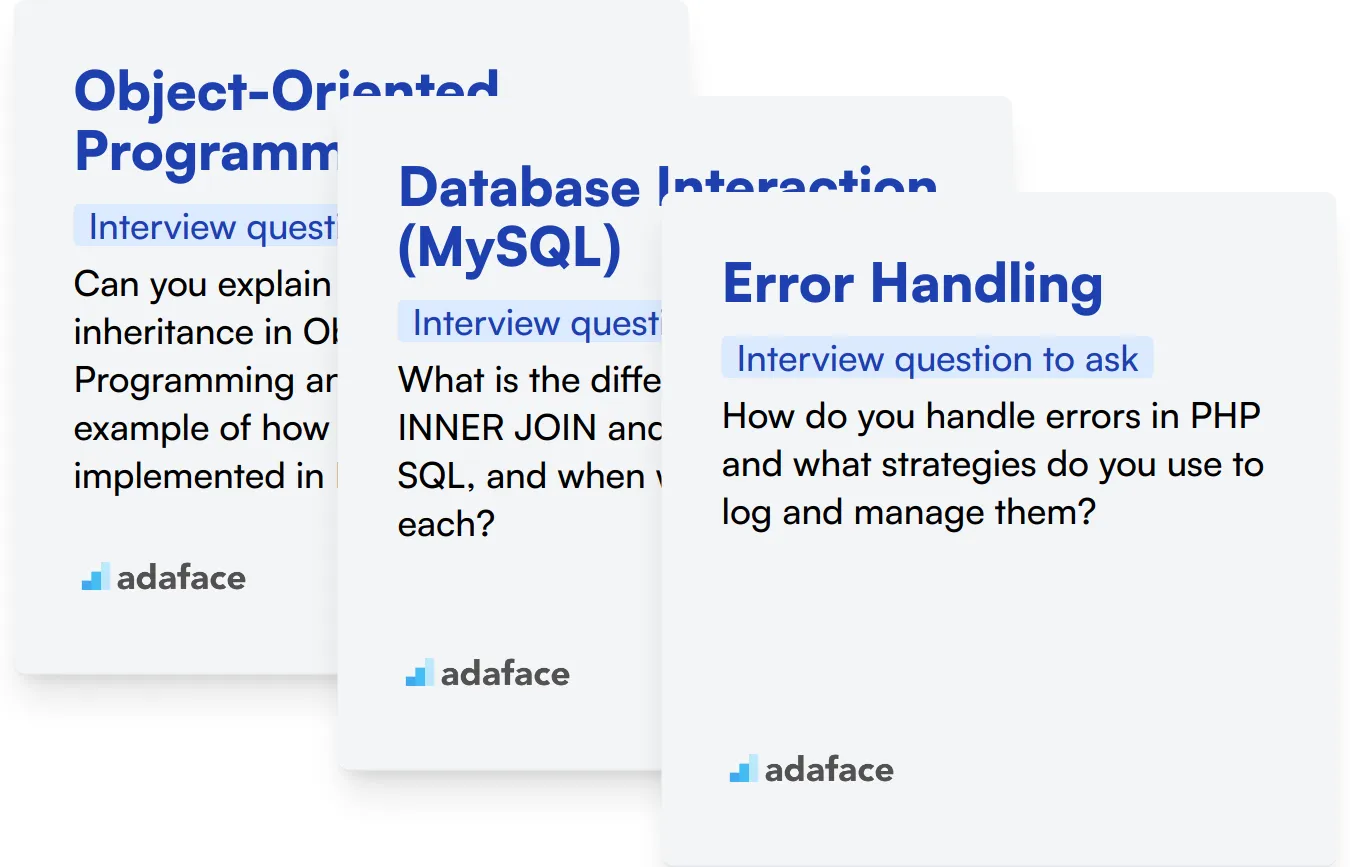
Object-Oriented Programming (OOP)
To assess a candidate's understanding of OOP in PHP, consider using an assessment test that includes relevant MCQs. You can find one available in our library: PHP Online Test.
Additionally, you can ask targeted interview questions to evaluate their grasp of OOP in PHP.
Can you explain the concept of inheritance in Object-Oriented Programming and provide an example of how it can be implemented in PHP?
Look for candidates who can clearly explain inheritance and provide a concise example. Their response should demonstrate an understanding of parent and child classes, as well as the benefits of code reuse and organization that inheritance offers.
Database Interaction (MySQL)
To evaluate a candidate's database skills, consider using an assessment test that includes relevant MCQs. You can check our library for MySQL Online Test.
You can also gauge their knowledge by asking specific questions regarding database interactions.
What is the difference between INNER JOIN and LEFT JOIN in SQL, and when would you use each?
Candidates should demonstrate an understanding of join operations and their use cases. Look for clarity in their explanation of how each join affects the results of a query based on the relationships between tables.
Error Handling
To assess a candidate's error-handling capabilities, consider utilizing an assessment test that covers this topic. You might find a relevant test in our offerings.
In addition to testing, specific questions can help uncover a candidate's approach to error handling.
How do you handle errors in PHP and what strategies do you use to log and manage them?
Look for responses that highlight various error handling methods such as using try/catch blocks, logging errors, and customizing error messages. Candidates should express a clear understanding of best practices in managing errors.
Enhance Your Hiring Strategy with PHP Skills Tests and Targeted Interview Questions
When aiming to hire individuals with PHP skills, it's important to accurately assess whether candidates possess the necessary expertise. Verifying these skills upfront saves time and resources.
The best way to assess PHP competencies is through targeted skills tests. Consider using Adaface's PHP Online Test or the PHP Laravel SQL Test to evaluate candidates effectively.
After candidates complete the skills tests, you can efficiently shortlist the top performers. This allows you to invite only the most promising applicants for interviews, streamlining the hiring process.
To take the next step, visit our Sign Up page to create an account or explore our Online Assessment Platform for more information on how we can assist in optimizing your recruitment process.
PHP & WordPress Developer Online Test
Download PHP interview questions template in multiple formats
PHP Interview Questions FAQs
Look for familiarity with basic PHP concepts, problem-solving abilities, and a keen interest in learning.
Assess their experience with more complex PHP tasks, use of frameworks, and understanding of best practices.
Ask about key PHP terms such as namespaces, traits, and autoloading to gauge their technical knowledge.
They reveal how candidates handle real-world scenarios, showcasing their problem-solving skills and adaptability.
Inquire about their experience with version control, deployment processes, and code optimization techniques.
Skills tests provide a practical assessment of a candidate's abilities, ensuring they meet your technical requirements.
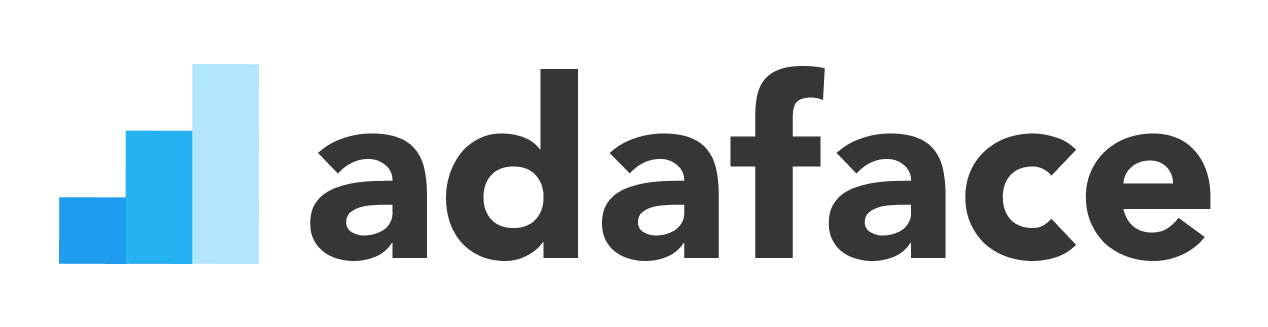
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
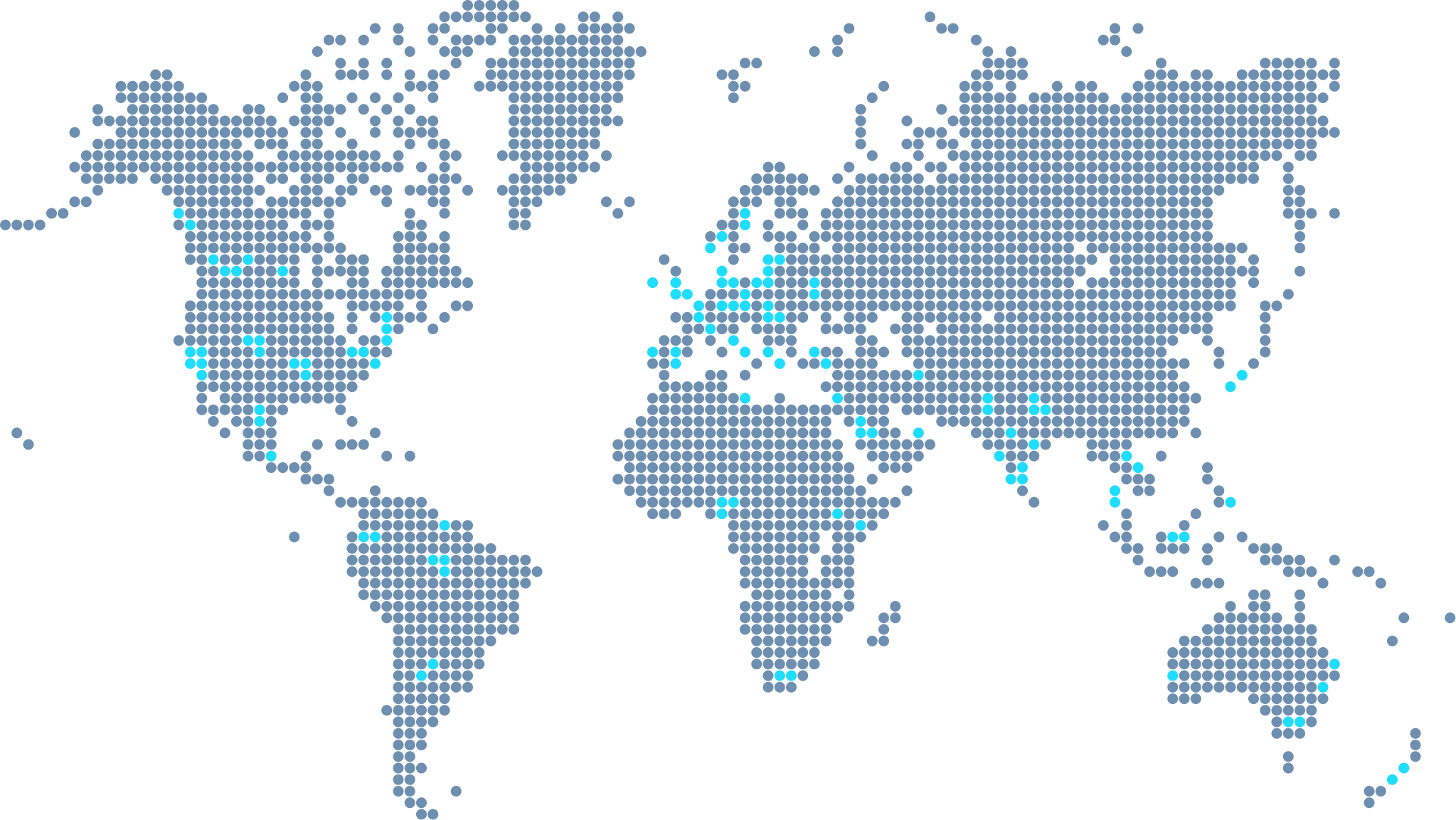
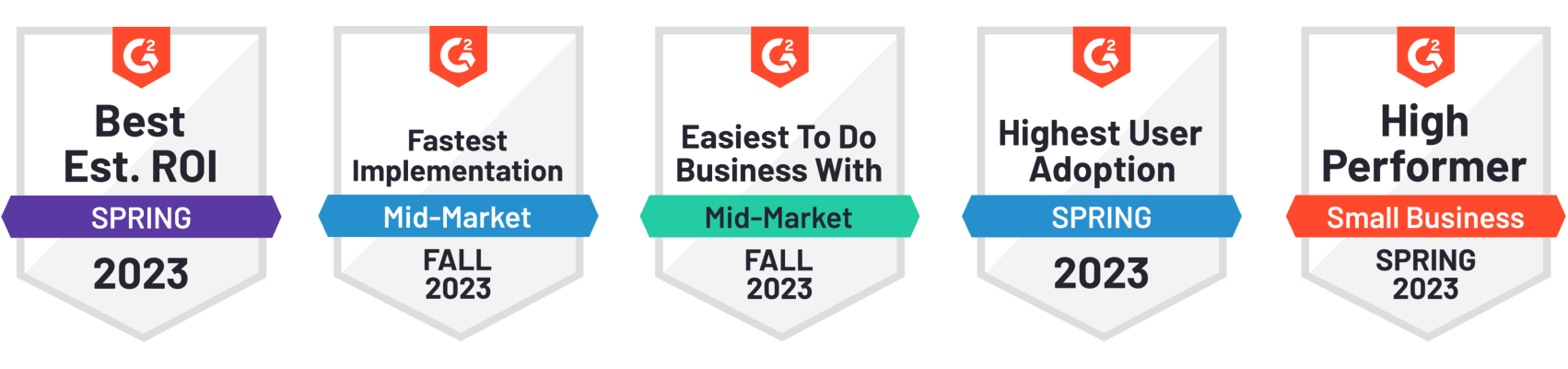