Hiring the right Perl developer can be a challenge, especially when you need to assess their skills accurately. A well-structured interview process with relevant questions is key to identifying top talent and ensuring they have the necessary skills for the role.
This blog post provides a comprehensive list of Perl interview questions, ranging from basic concepts to advanced topics like regex, file handling, and modules. We've organized the questions into different categories to help you assess candidates at various experience levels, from junior developers to seasoned professionals.
By using these questions, you can effectively evaluate a candidate's Perl expertise and problem-solving abilities. Consider complementing your interview process with a Perl skills assessment to get a more complete picture of the candidate's capabilities.
Table of contents
10 basic Perl interview questions and answers to assess applicants
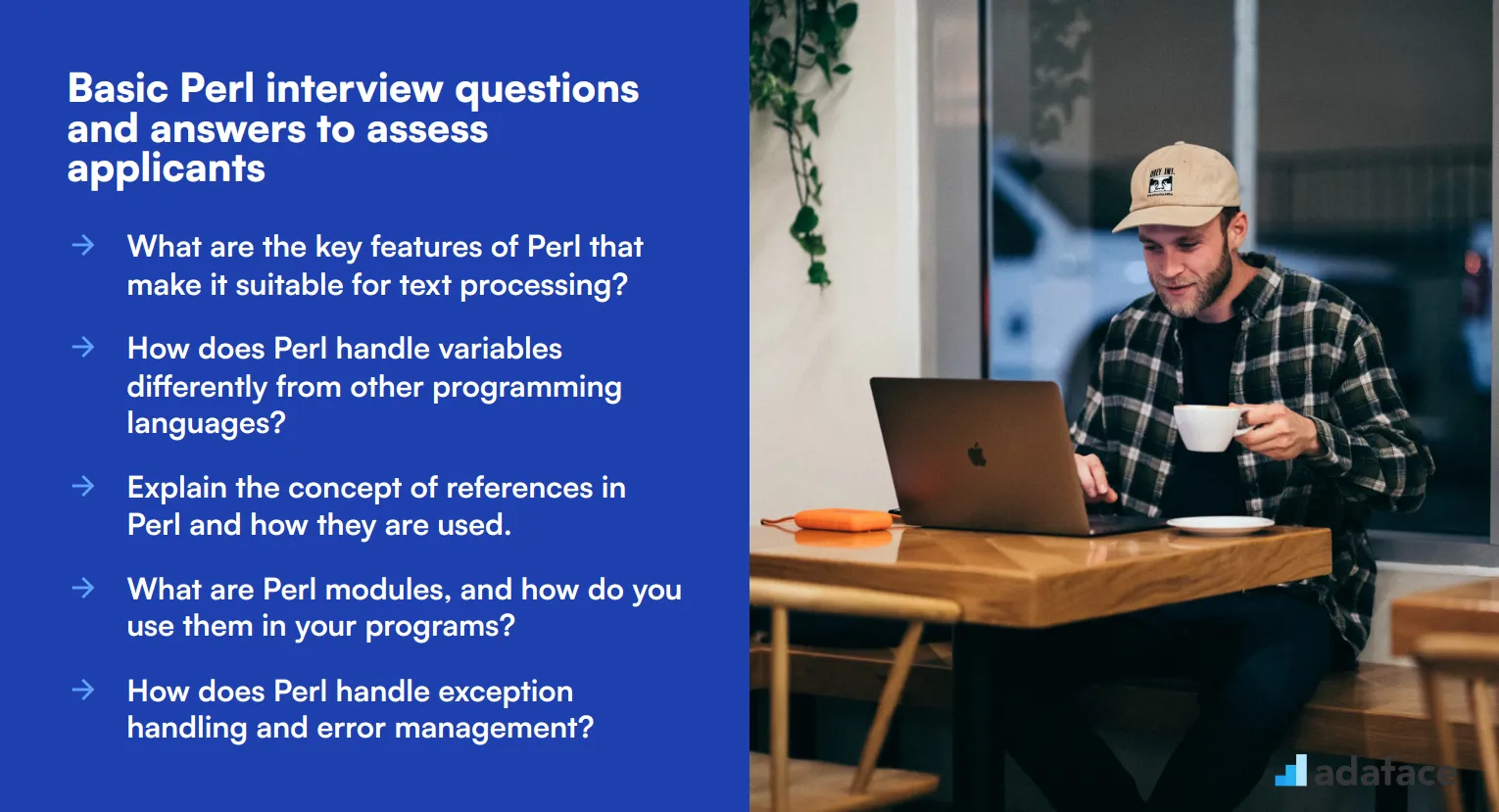
When interviewing Perl developers, it's crucial to assess their foundational knowledge and problem-solving skills. These 10 basic Perl interview questions will help you gauge candidates' understanding of core concepts and their ability to apply them. Use these questions as a starting point to evaluate potential hires and identify those who can contribute effectively to your software development projects.
1. What are the key features of Perl that make it suitable for text processing?
Perl's strengths in text processing stem from several key features:
- Powerful regular expressions: Perl has built-in support for complex pattern matching and text manipulation.
- Flexible data structures: Hashes and arrays in Perl make it easy to store and manipulate text data.
- Built-in functions: Perl offers numerous functions for string manipulation, such as split, join, and substr.
- Automatic type conversion: Perl can seamlessly convert between strings and numbers, simplifying text operations.
Look for candidates who can explain these features and provide examples of how they've used them in real-world scenarios. Strong applicants will also mention Perl's efficiency in handling large text files and its ability to process data line-by-line.
2. How does Perl handle variables differently from other programming languages?
Perl's approach to variables is unique in several ways:
- Dynamic typing: Perl doesn't require explicit type declarations for variables.
- Sigils: Variables are prefixed with symbols ($ for scalars, @ for arrays, % for hashes) that indicate their type.
- Context sensitivity: The same variable can behave differently depending on whether it's used in a scalar or list context.
- Automatic scope: Variables are lexically scoped by default when using 'my' keyword.
A strong candidate should be able to explain these concepts clearly and discuss how they contribute to Perl's flexibility. Look for answers that demonstrate an understanding of how Perl's variable handling affects code readability and maintenance.
3. Explain the concept of references in Perl and how they are used.
References in Perl are similar to pointers in other languages. They allow indirect access to data structures and enable the creation of complex data structures like arrays of arrays or hashes of hashes. Key points about references include:
- Creating references: Use the backslash operator () to create a reference to a variable or data structure.
- Dereferencing: Access the original data using the appropriate sigil and curly braces (e.g., ${$ref} for scalar references).
- Reference counting: Perl automatically manages memory through reference counting.
An ideal candidate should be able to explain how references can be used to pass complex data structures to subroutines efficiently or to create recursive data structures. Look for examples that demonstrate practical applications of references in real-world scenarios.
4. What are Perl modules, and how do you use them in your programs?
Perl modules are reusable packages of code that extend Perl's functionality. They encapsulate related subroutines and variables, promoting code organization and reusability. Key points about modules include:
- Creating modules: Modules are typically created as .pm files and use the 'package' keyword.
- Using modules: The 'use' statement imports a module into a Perl script.
- CPAN: The Comprehensive Perl Archive Network is a vast repository of open-source Perl modules.
- Core modules: Perl comes with a set of standard modules included in its distribution.
Look for candidates who can explain how they've used both core and third-party modules in their projects. Strong answers will include examples of how modules have helped them solve specific problems or improve code maintainability.
5. How does Perl handle exception handling and error management?
Perl provides several mechanisms for exception handling and error management:
- die and warn functions: 'die' terminates the program with an error message, while 'warn' prints a warning without stopping execution.
- eval block: Used for trapping exceptions in a block of code.
- $@ variable: Contains the error message from the last eval execution.
- Try::Tiny module: Provides a more robust exception handling mechanism similar to try-catch in other languages.
A strong candidate should be able to explain these concepts and discuss best practices for error handling in Perl. Look for answers that demonstrate an understanding of how to write robust, fault-tolerant Perl code and how to effectively debug and troubleshoot issues.
6. What are Perl's strengths and weaknesses compared to other scripting languages?
Perl's strengths include:
- Excellent text processing capabilities
- Large collection of open-source modules (CPAN)
- Flexibility and expressiveness ('There's more than one way to do it')
- Strong support for regular expressions
- Cross-platform compatibility
Weaknesses might include:
- Syntax can be complex and sometimes lead to 'write-only' code
- Performance can be slower compared to compiled languages
- Less popular for web development compared to newer languages
Look for candidates who can provide a balanced view and explain how they leverage Perl's strengths while mitigating its weaknesses in their projects. Strong answers will include specific examples from their experience.
7. How do you optimize Perl code for better performance?
Optimizing Perl code involves several strategies:
- Profiling: Use tools like Devel::NYTProf to identify performance bottlenecks.
- Algorithmic improvements: Choose efficient algorithms and data structures.
- Avoid unnecessary operations: Minimize loops and function calls where possible.
- Use built-in functions: Perl's built-in functions are often faster than custom implementations.
- Leverage modules: Use optimized modules from CPAN for common tasks.
- Compile critical sections: Use inline C or XS for performance-critical parts.
A strong candidate should be able to discuss these techniques and provide examples of how they've optimized Perl code in real projects. Look for answers that demonstrate an understanding of the trade-offs between readability, maintainability, and performance.
8. Explain the concept of context in Perl and how it affects variable behavior.
Context in Perl refers to how a statement or expression is evaluated based on what's expected from it. The two main contexts are scalar and list context. Key points include:
- Scalar context: When a single value is expected (e.g., assignment to a scalar variable).
- List context: When multiple values are expected (e.g., assignment to an array).
- The 'wantarray' function can be used to determine the context in which a subroutine was called.
- Some built-in functions behave differently depending on the context.
An ideal candidate should be able to explain how context affects the behavior of variables and functions, and provide examples of how this can be both powerful and potentially confusing. Look for answers that demonstrate a deep understanding of this unique Perl feature and how it impacts code design and debugging.
9. How do you handle database operations in Perl?
Perl offers robust database handling capabilities, primarily through the DBI (Database Interface) module. Key points to cover:
- DBI module: Provides a consistent interface for database access across different database types.
- Database drivers: Specific drivers (e.g., DBD::MySQL, DBD::Pg) are used for different database systems.
- Connecting to databases: Using DBI->connect() method with appropriate credentials.
- Executing queries: Preparing and executing SQL statements using methods like prepare() and execute().
- Fetching results: Methods like fetchrow_array() or fetchall_arrayref() for retrieving query results.
- Error handling: Checking for and handling database errors appropriately.
Look for candidates who can explain how they've used DBI in real projects, including best practices for security (e.g., using placeholders to prevent SQL injection) and performance optimization (e.g., prepared statements for repeated queries).
10. What are some best practices for writing maintainable Perl code?
Writing maintainable Perl code involves several best practices:
- Use 'use strict' and 'use warnings' to catch potential issues early.
- Follow consistent naming conventions for variables, functions, and modules.
- Write clear, self-documenting code with meaningful variable and function names.
- Use comments judiciously to explain complex logic or non-obvious decisions.
- Modularize code into reusable functions and modules.
- Use version control systems like Git to track changes and collaborate.
- Write and maintain comprehensive unit tests.
- Follow the DRY (Don't Repeat Yourself) principle to avoid code duplication.
A strong candidate should be able to discuss these practices and provide examples of how they've implemented them in their own projects. Look for answers that demonstrate an understanding of the importance of code readability, modularity, and testability in maintaining large Perl codebases over time.
20 Perl interview questions to ask junior developers
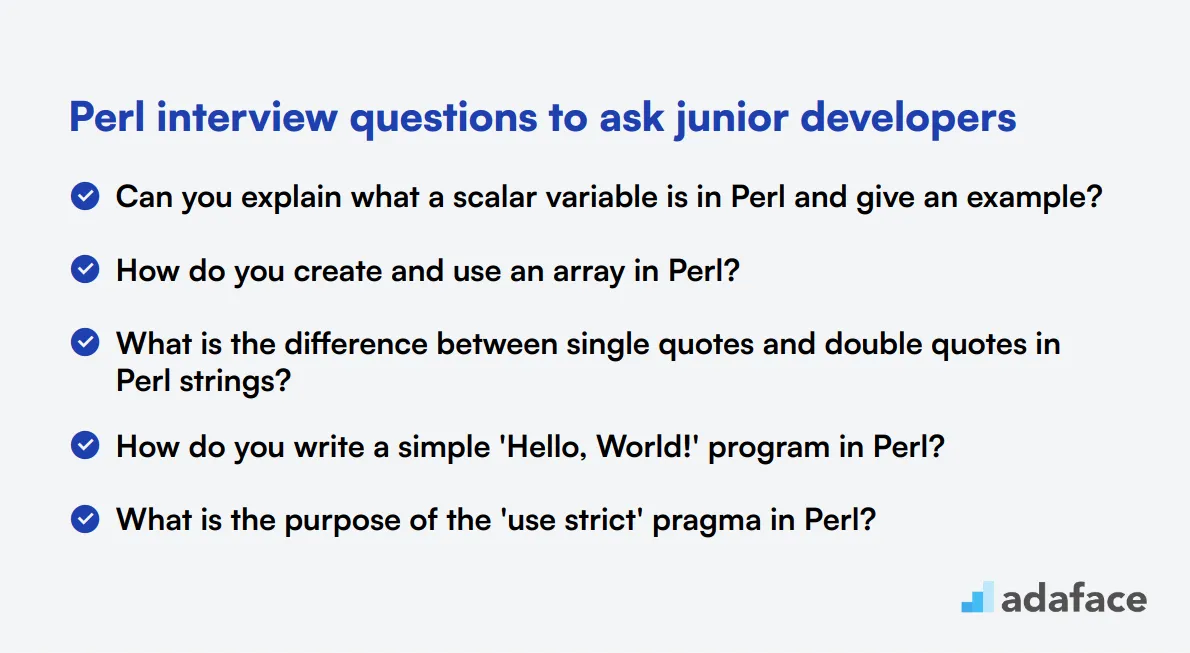
To assess the foundational knowledge of junior Perl developers, use these 20 interview questions. They cover essential concepts and practical skills, helping you identify candidates with a solid grasp of Perl basics and potential for growth.
- Can you explain what a scalar variable is in Perl and give an example?
- How do you create and use an array in Perl?
- What is the difference between single quotes and double quotes in Perl strings?
- How do you write a simple 'Hello, World!' program in Perl?
- What is the purpose of the 'use strict' pragma in Perl?
- How do you read input from the command line in a Perl script?
- Explain the concept of 'chomp' in Perl and when you might use it.
- How do you write a basic for loop in Perl?
- What is the purpose of the '$_' variable in Perl?
- How do you open and read a file in Perl?
- What is a hash in Perl and how is it different from an array?
- How do you define and call a subroutine in Perl?
- What does the '=~' operator do in Perl?
- How do you use regular expressions in Perl?
- What is the difference between 'my' and 'local' variables in Perl?
- How do you concatenate strings in Perl?
- What is the purpose of the 'die' function in Perl?
- How do you check if a file exists using Perl?
- What is the difference between 'shift' and 'pop' in Perl arrays?
- How do you split a string into an array in Perl?
8 Perl interview questions and answers related to regex and file handling
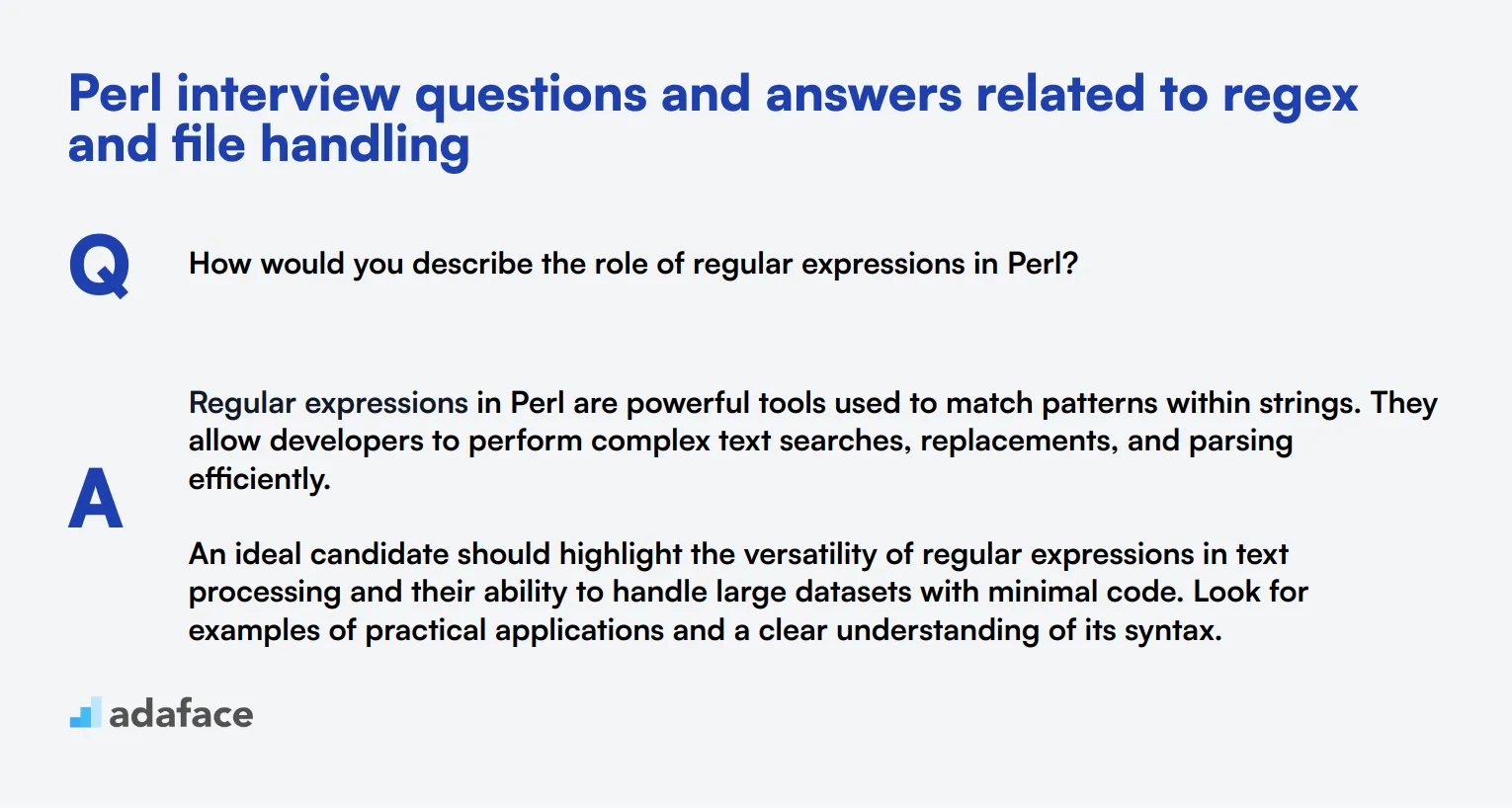
Understanding regex and file handling in Perl is crucial for gauging a candidate’s problem-solving abilities and their efficiency in processing text. Use these questions to discover whether your candidates have the right skills to manage file operations and harness the power of regular expressions in Perl.
1. How would you describe the role of regular expressions in Perl?
Regular expressions in Perl are powerful tools used to match patterns within strings. They allow developers to perform complex text searches, replacements, and parsing efficiently.
An ideal candidate should highlight the versatility of regular expressions in text processing and their ability to handle large datasets with minimal code. Look for examples of practical applications and a clear understanding of its syntax.
2. Can you explain the process of opening, reading, and closing a file in Perl?
Opening, reading, and closing a file in Perl involves a few simple steps. First, you open the file using the appropriate mode (read, write, or append). Then, you read or write to the file as needed. Finally, you close the file to free up system resources.
Candidates should demonstrate a clear, step-by-step understanding of the file handling process. An ideal response will include the importance of error handling and resource management during these operations.
3. How can you search for a specific pattern in a text file using Perl?
To search for a specific pattern in a text file, you can use regular expressions along with file handling techniques. Open the file, read its contents line by line, and apply the regex to each line to find matches.
Look for candidates who can explain the importance of efficient file reading and the use of regex for pattern matching. The ability to provide a clear and logical approach to the problem is key.
4. What are some common file handling errors in Perl and how do you handle them?
Common file handling errors in Perl include issues like being unable to open a file, attempting to read a file that doesn't exist, or failing to close a file properly. These can be handled using error checking mechanisms such as checking the return value of open() and using die() to handle errors gracefully.
Candidates should be able to discuss specific error handling strategies and the importance of robust error management to prevent data loss or corruption. An ideal response will include examples of how they have managed file errors in past projects.
5. How would you extract and manipulate specific data from a text file using Perl?
Extracting and manipulating data from a text file involves reading the file, using regex to identify the required data, and then processing that data accordingly. This could include tasks like formatting, calculations, or storing the data in a different structure.
Candidates should demonstrate a methodical approach to data extraction and manipulation. Look for their ability to explain each step clearly and their use of efficient techniques to handle large datasets.
6. Explain how you can use Perl to search and replace text in a file.
To search and replace text in a file using Perl, you read the file content, apply a regex substitution to change the desired text, and then write the modified content back to the file.
Evaluate the candidate’s understanding of file I/O operations and regex substitution. The ideal answer should highlight the importance of backing up data before performing such operations to prevent accidental data loss.
7. What are some best practices for using regular expressions in Perl for text processing?
Best practices for using regular expressions in Perl include writing clear and maintainable patterns, avoiding overly complex regexes, using non-capturing groups when possible, and testing regex thoroughly to ensure accuracy.
Candidates should emphasize readability, efficiency, and the importance of testing. Look for examples where they have applied these practices in real-world scenarios to solve text processing challenges.
8. How would you handle large files in Perl to ensure efficient processing?
Handling large files in Perl efficiently can involve reading the file in chunks, using memory-mapped files, or employing streaming techniques to process data as it is read.
An ideal candidate should discuss methods to minimize memory usage and improve processing speed. Look for their understanding of the challenges associated with large files and the strategies they use to overcome these challenges.
12 Perl questions related to regex
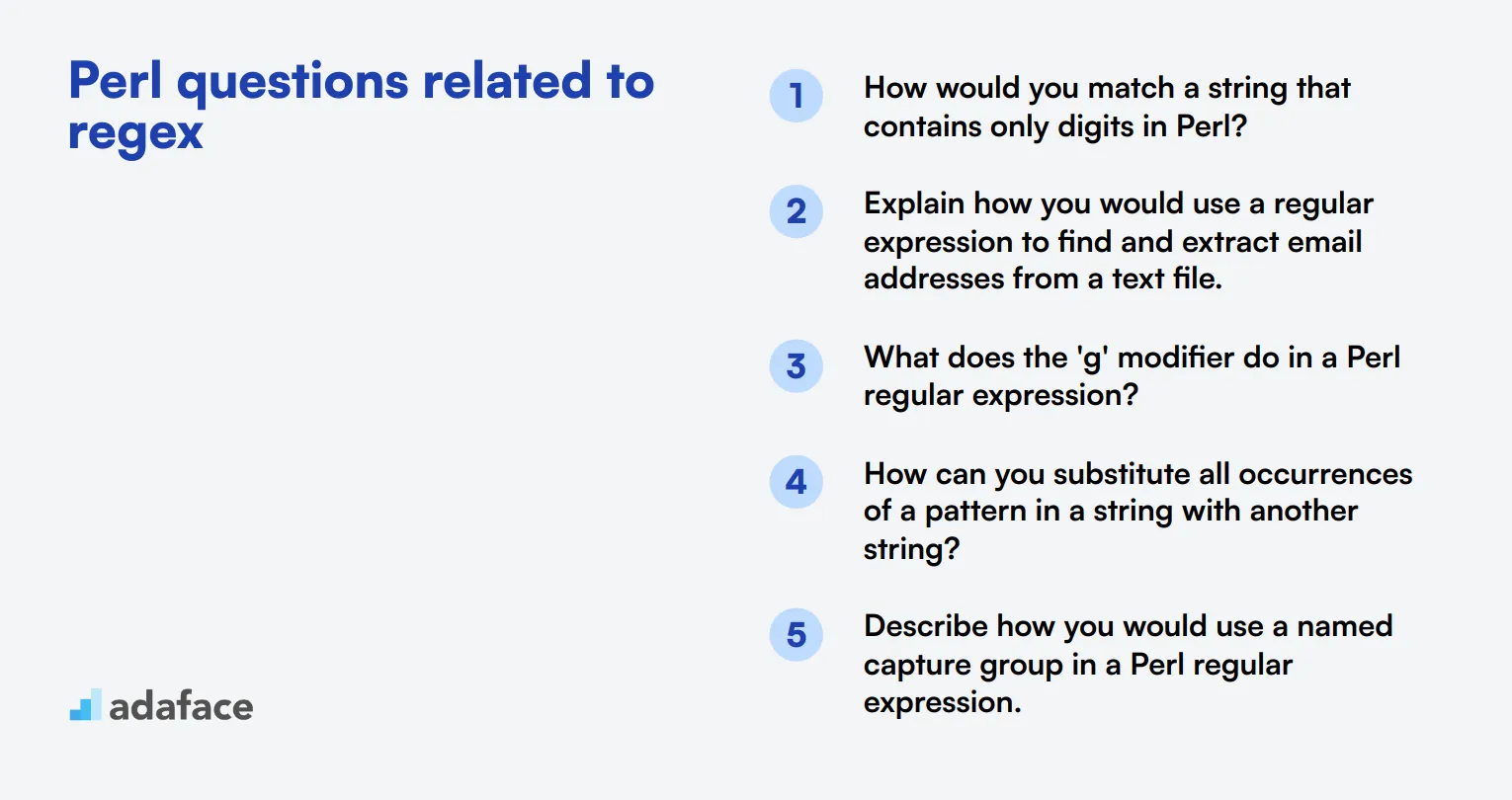
To determine whether your applicants have the right skills to work with Perl's regular expressions, ask them some of these 12 Perl questions related to regex. This list will help you gauge their proficiency in text processing, an essential skill for any Perl Developer.
- How would you match a string that contains only digits in Perl?
- Explain how you would use a regular expression to find and extract email addresses from a text file.
- What does the 'g' modifier do in a Perl regular expression?
- How can you substitute all occurrences of a pattern in a string with another string?
- Describe how you would use a named capture group in a Perl regular expression.
- What is the difference between greedy and non-greedy matching in Perl regex?
- Can you explain the use of the 'm' modifier in Perl regular expressions?
- How would you split a string by whitespace using a Perl regular expression?
- Describe a scenario where you would use lookahead or lookbehind assertions in Perl regex.
- How can you match a literal dot (.) character in a Perl regular expression?
- Explain the use of the '^' and '$' anchors in Perl regex.
- How would you write a Perl regular expression to validate a phone number format?
12 Perl questions related to modules and CPAN
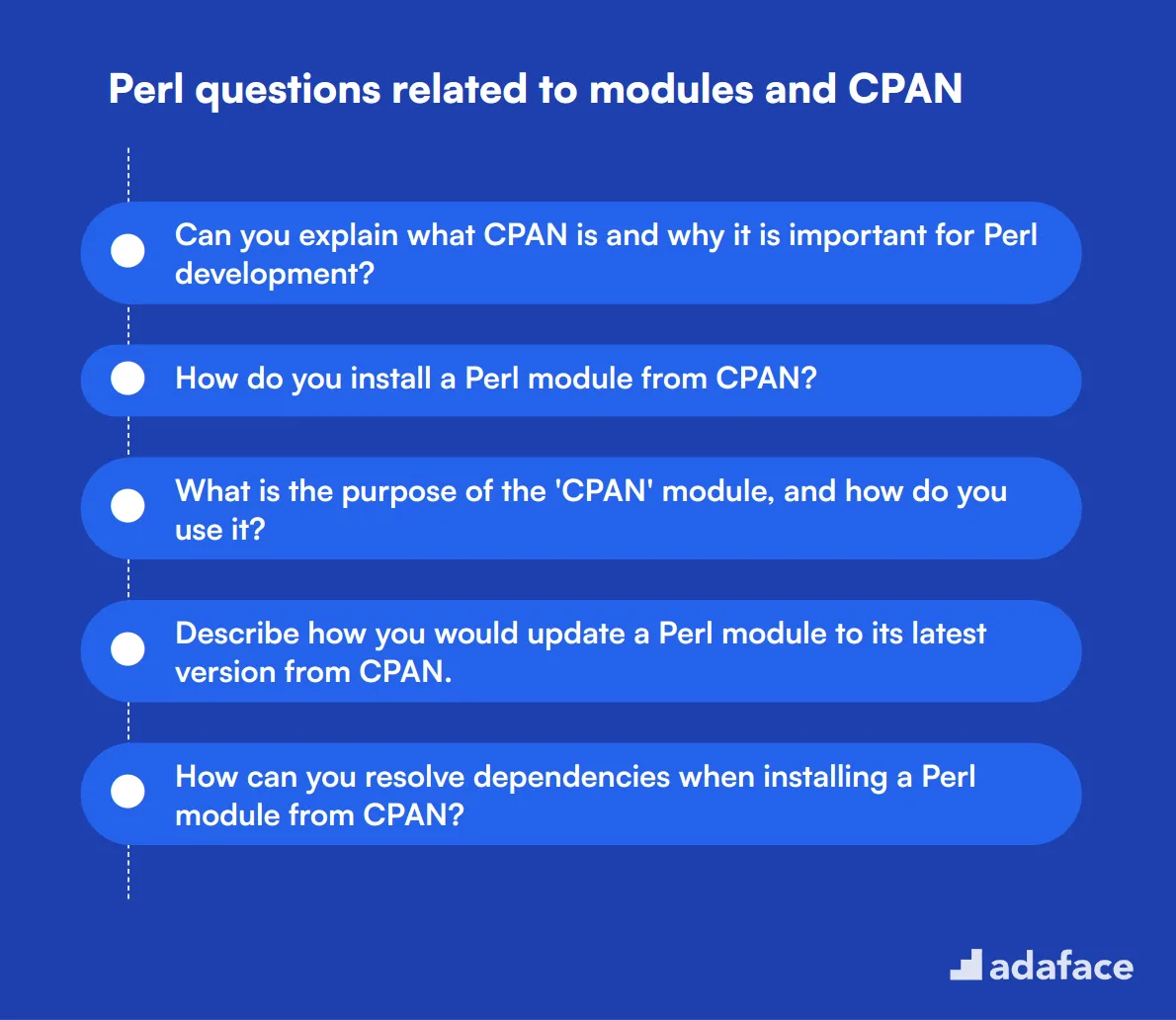
To ensure your candidates have the right expertise in Perl, especially in utilizing modules and the Comprehensive Perl Archive Network (CPAN), ask them some of these targeted interview questions. These questions will help you gauge their practical knowledge and problem-solving skills related to Perl's extensive module ecosystem. For more details, you can refer to this Perl developer job description.
- Can you explain what CPAN is and why it is important for Perl development?
- How do you install a Perl module from CPAN?
- What is the purpose of the 'CPAN' module, and how do you use it?
- Describe how you would update a Perl module to its latest version from CPAN.
- How can you resolve dependencies when installing a Perl module from CPAN?
- What are some commonly used Perl modules that you have worked with, and how have you used them in your projects?
- Explain how you would create your own Perl module and publish it to CPAN.
- What tools or commands do you use to search for Perl modules on CPAN?
- How do you handle version control for Perl modules in your projects?
- Can you discuss a scenario where you had to troubleshoot an issue with a CPAN module?
- What is 'local::lib' and how does it help in managing Perl modules?
- How do you ensure the security and reliability of Perl modules sourced from CPAN?
10 situational Perl interview questions for hiring top developers
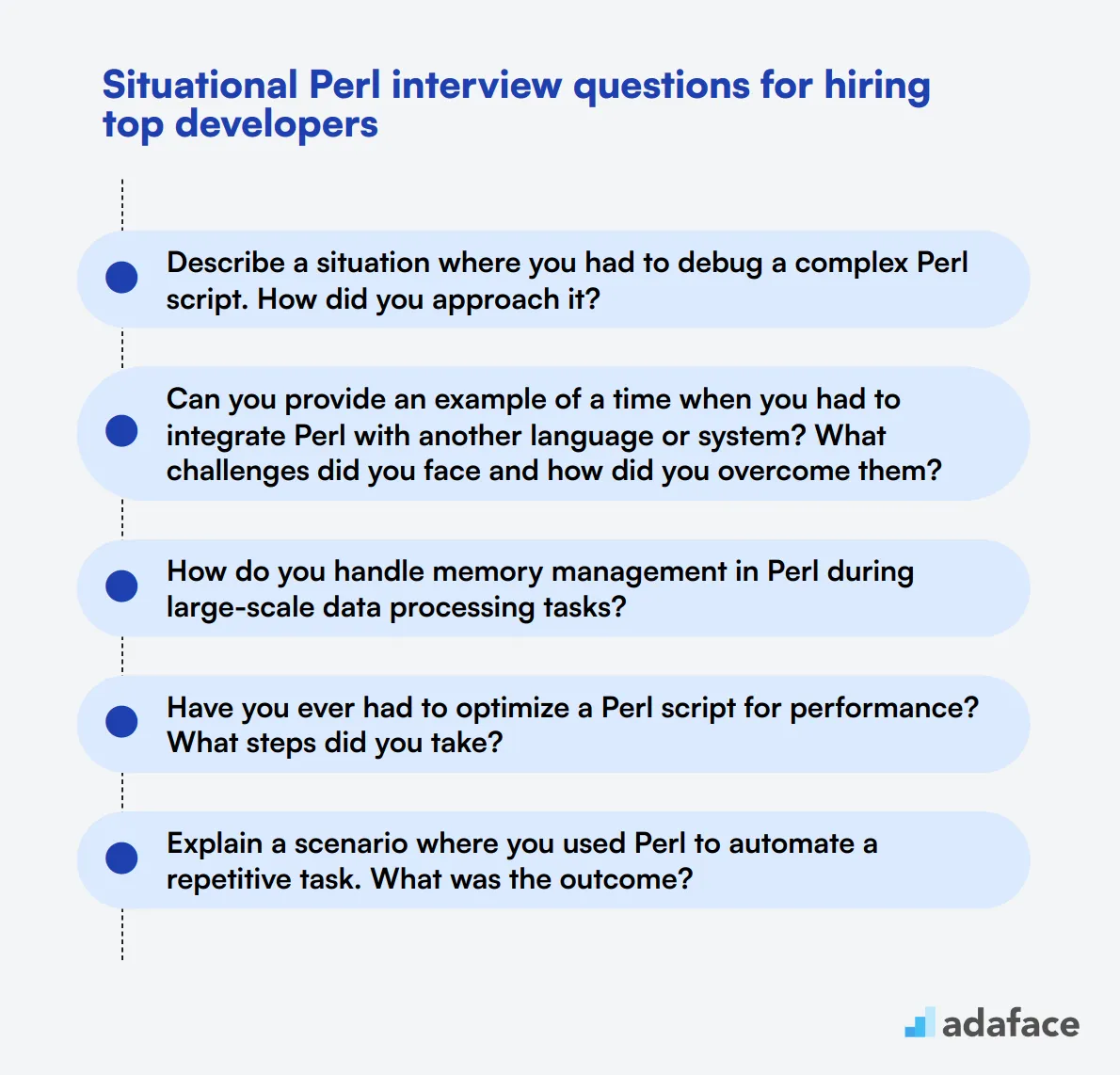
To identify top-tier Perl developers, it's crucial to ask situational questions that reveal their problem-solving skills and practical experience. Use the following questions during your interviews to gain insight into how candidates handle real-world Perl challenges and scenarios.
- Describe a situation where you had to debug a complex Perl script. How did you approach it?
- Can you provide an example of a time when you had to integrate Perl with another language or system? What challenges did you face and how did you overcome them?
- How do you handle memory management in Perl during large-scale data processing tasks?
- Have you ever had to optimize a Perl script for performance? What steps did you take?
- Explain a scenario where you used Perl to automate a repetitive task. What was the outcome?
- Describe a time when you had to refactor existing Perl code. What was your approach and what were the results?
- Can you discuss a challenging bug you encountered in a Perl project and how you resolved it?
- How do you manage dependencies in a Perl project to ensure smooth deployment and maintenance?
- Have you ever had to migrate a Perl application to a newer version? What issues did you encounter and how did you address them?
- Explain how you would go about securing a Perl application from common vulnerabilities.
Which Perl skills should you evaluate during the interview phase?
Assessing a candidate's overall capability in a single interview can be challenging. However, for Perl, there are certain core skills that are important to evaluate to get a fair understanding of their proficiency and fit for the role.
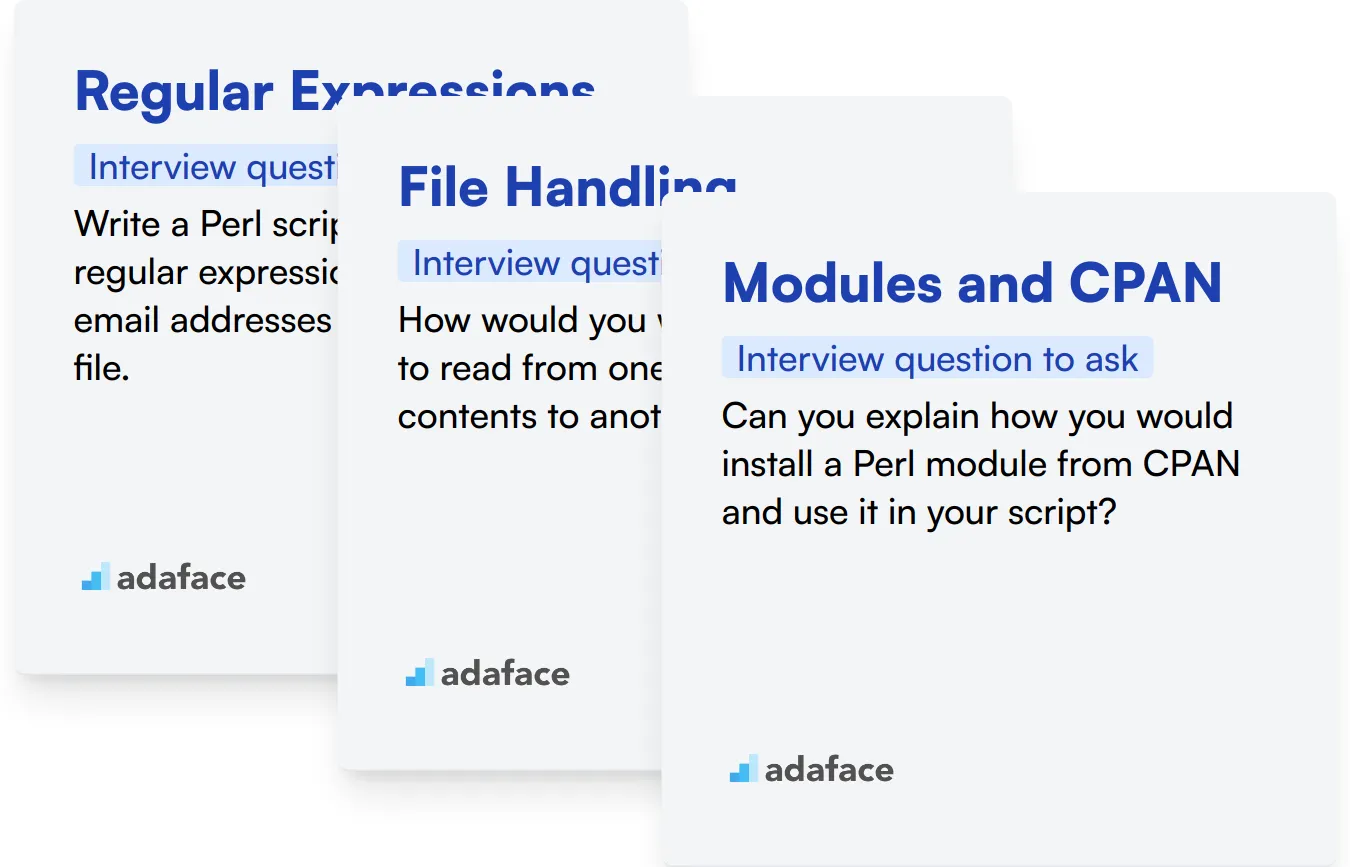
Regular Expressions
Regular expressions are a powerful tool in Perl for pattern matching and text processing. They are integral to many Perl scripts and applications.
To assess this skill, you can use an assessment test that includes relevant MCQs to filter out candidates who have a strong understanding of regular expressions. Our Perl test includes such questions.
You can also ask targeted interview questions specifically designed to judge a candidate's proficiency with regular expressions.
Write a Perl script that uses a regular expression to extract all email addresses from a given text file.
Look for candidates who correctly use or explain the regex pattern for matching email addresses and who can integrate that pattern effectively in a Perl script.
File Handling
File handling is a common task in Perl programming, used in data processing, web development, and more. Understanding file I/O operations is crucial for many Perl applications.
To evaluate this skill, an assessment with relevant MCQs can be beneficial. The Perl test includes questions on file handling.
Interview questions on file handling can help you gauge the applicant's practical understanding and ability to work with files in Perl.
How would you write a Perl script to read from one file and write its contents to another file?
Focus on candidates who demonstrate not only the correct usage of file handles but also error handling and efficient file processing techniques.
Modules and CPAN
Perl's Comprehensive Perl Archive Network (CPAN) is a vast repository of modules that can extend Perl's functionality. Knowing how to use CPAN and select the right modules is a key skill for a Perl developer.
Assessment tests with questions on CPAN and modules can help filter candidates with a good grasp of Perl's module ecosystem. Our Perl test covers this area.
Ask specific questions in the interview to understand their familiarity with using CPAN and integrating modules into their projects.
Can you explain how you would install a Perl module from CPAN and use it in your script?
Look for candidates who can clearly describe the process of installing and using Perl modules, demonstrating practical knowledge of CPAN.
Hire top talent with Perl skills tests and the right interview questions
When hiring for candidates with Perl skills, it's important to ensure they possess the necessary expertise. Accurately assessing their proficiency will help you find the right fit for your team.
One effective method to evaluate these skills is through skill tests. Consider using our Perl online test to gauge candidates' abilities effectively.
After administering the test, you can efficiently shortlist the best applicants. This allows you to focus your interviews on those who truly meet your expectations.
To get started, visit our assessment test library to explore various testing options. You can also sign up to begin assessing candidates today.
Perl Online Test
Download Perl interview questions template in multiple formats
Perl Interview Questions FAQs
The questions cover various skill levels, from basic concepts for juniors to advanced topics for experienced developers.
Tailor the questions to the specific role and use them alongside practical coding tests for a comprehensive assessment.
Yes, there are 20 questions related to regex, covering both general concepts and Perl-specific implementations.
Absolutely. There are 12 questions specifically addressing Perl modules and CPAN usage.
The list includes 10 situational Perl interview questions to assess problem-solving skills in real-world scenarios.
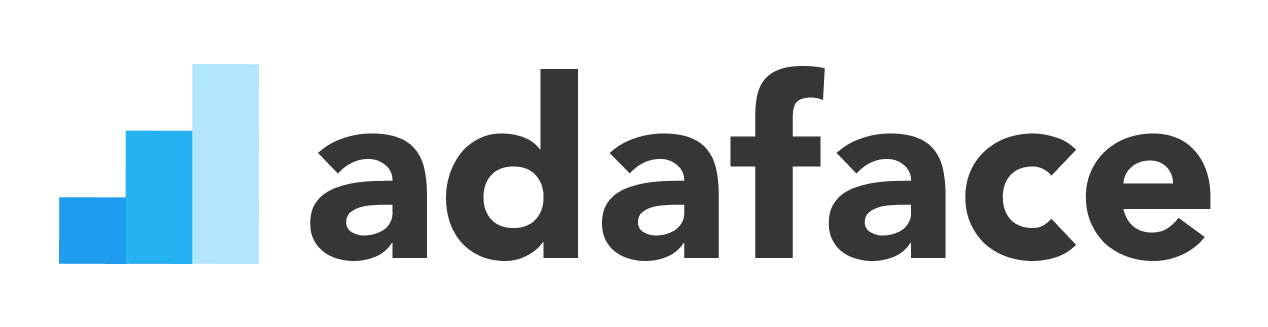
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
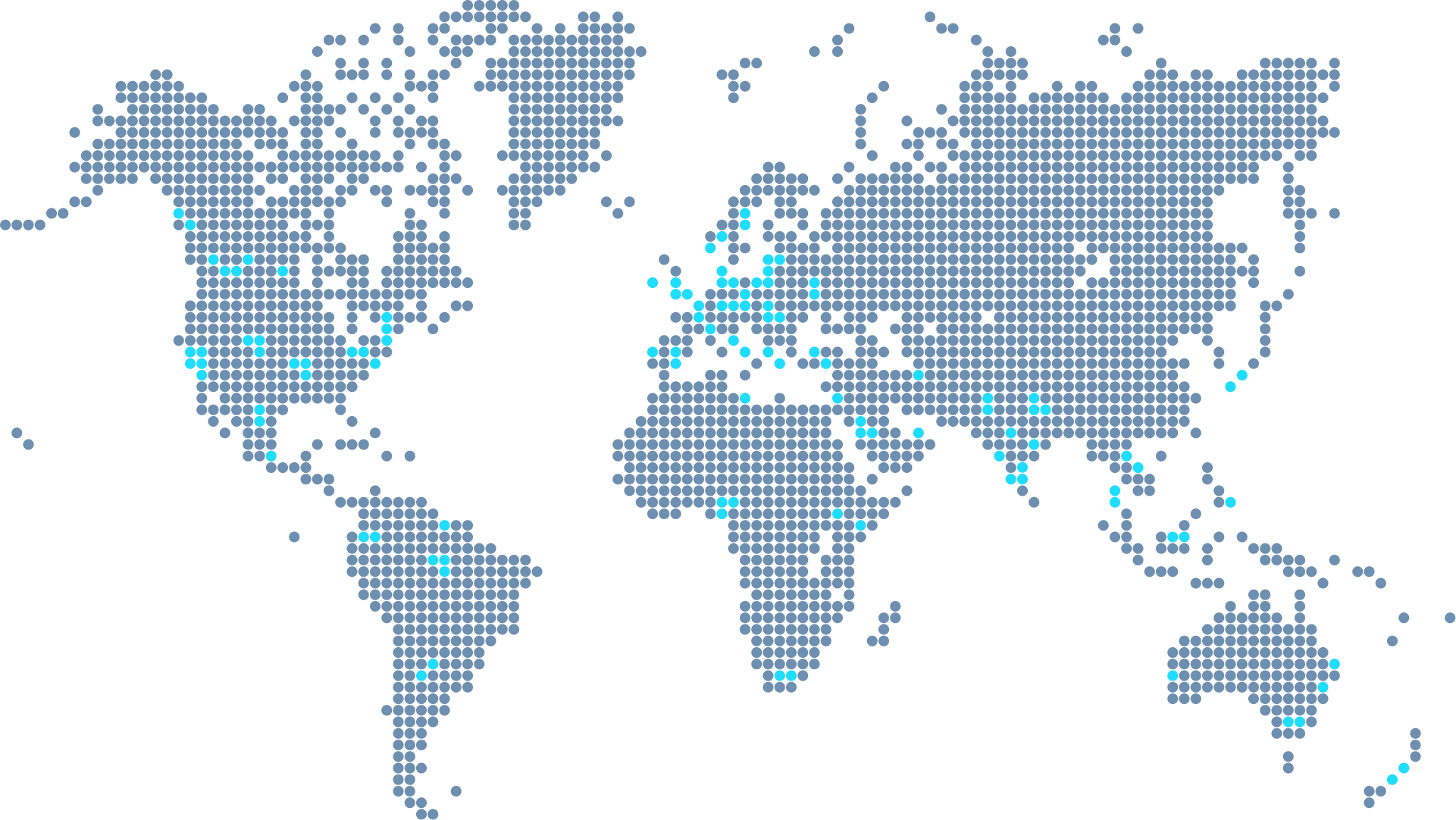
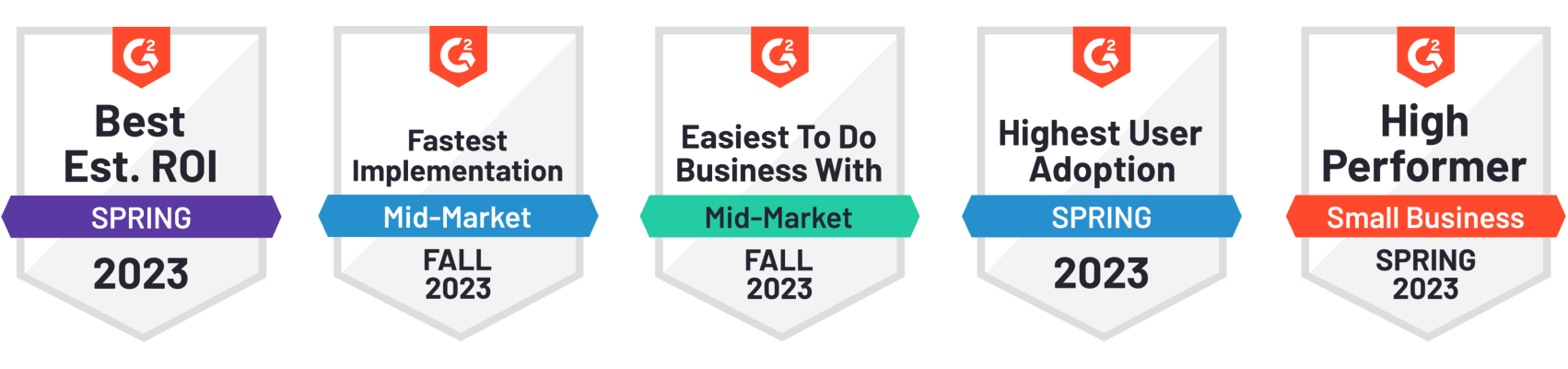