Hiring the right Angular developer can be a challenge for recruiters and hiring managers. To avoid potential missteps, asking the right questions during interviews is essential to identifying strong candidates.
In this blog post, we provide a comprehensive list of Angular interview questions to help you assess candidates across different skill levels. From basic questions for junior developers to more advanced queries for mid-tier candidates, we've got you covered.
By using these questions, you can identify the right talent with the skills your team needs. Additionally, consider using our Angular online test to pre-screen candidates before interviews.
Table of contents
10 basic Angular interview questions and answers to assess candidates
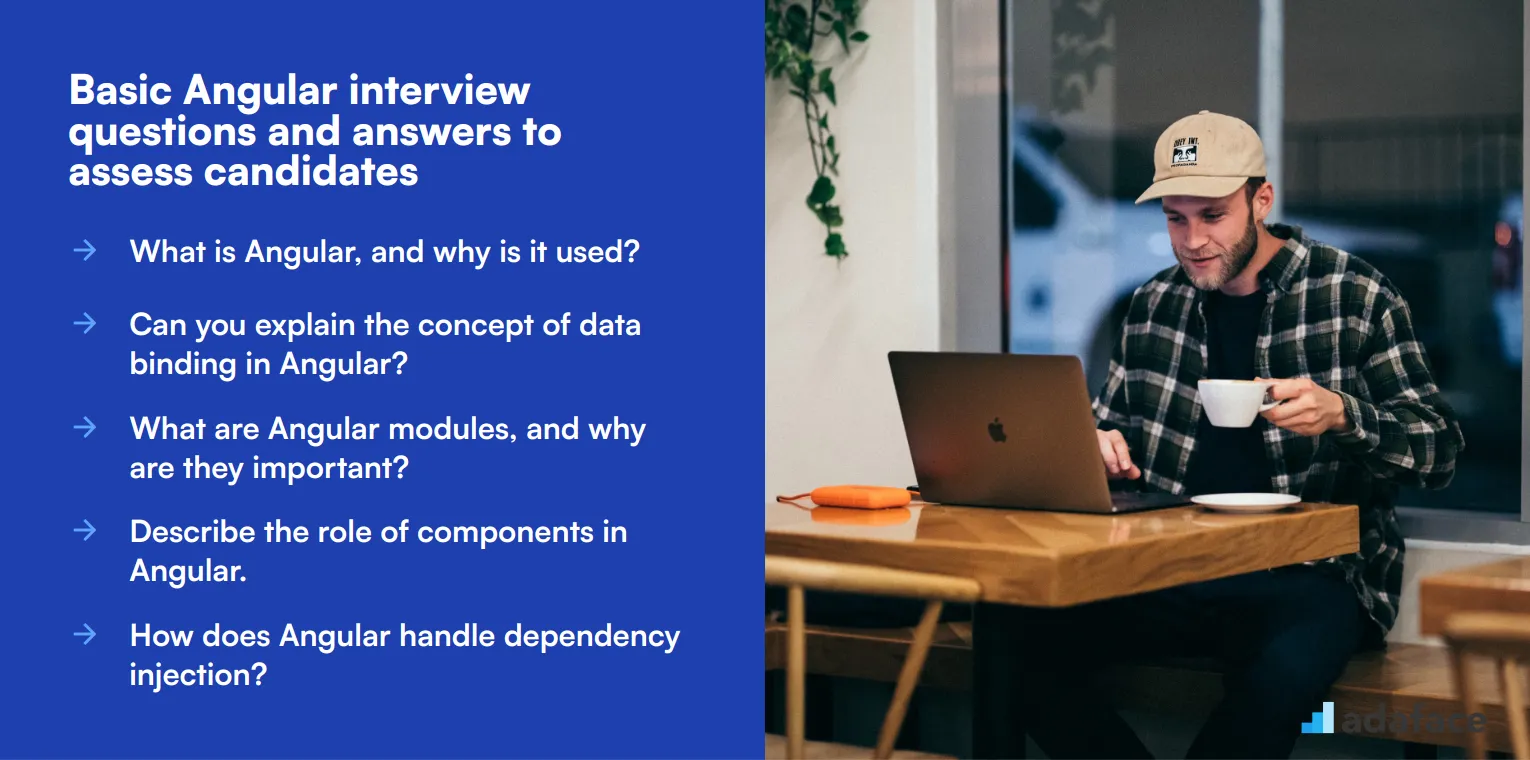
To identify if your candidates have a firm grasp of basic Angular concepts, use this list of questions. These questions are designed to be straightforward and effective in assessing their foundational knowledge during interviews.
1. What is Angular, and why is it used?
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. It is developed and maintained by Google.
Angular is used for developing dynamic web applications because it provides a robust structure, allows for the development of highly responsive applications, and has extensive capabilities for handling complex data binding and dependency injection.
Look for answers that show a clear understanding of Angular's purpose and its benefits in creating dynamic, efficient web applications. Follow up with questions about the candidate's experience with Angular in previous projects.
2. Can you explain the concept of data binding in Angular?
Data binding in Angular refers to the synchronization of data between the model and the view. There are four types of data binding: interpolation, property binding, event binding, and two-way binding.
Interpolation and property binding are used to transfer data from the component to the view, while event binding is used to transfer data from the view to the component. Two-way binding allows for the synchronization of data in both directions.
Candidates should demonstrate an understanding of these concepts and provide examples of how they have implemented data binding in their projects. Follow up with specific scenarios to see how they handle data binding in real-world applications.
3. What are Angular modules, and why are they important?
Angular modules are containers that help organize an application into cohesive blocks of functionality. Each Angular application has at least one module, the root module, which is used to bootstrap the application.
Modules are important because they help manage the dependencies, improve code organization, and allow for lazy loading, which can improve the application's performance by loading modules only when needed.
An ideal response should reflect a candidate's ability to structure an Angular application efficiently using modules. Look for their experience in creating and managing Angular modules in their past projects.
4. Describe the role of components in Angular.
Components are the building blocks of an Angular application. A component controls a part of the screen called a view and consists of three parts: the template, the class, and metadata.
The template defines the HTML view, the class defines the logic and data, and the metadata provides additional information like the selector and styles. Components help in creating a well-structured and maintainable codebase.
Candidates should discuss their experience in creating and managing components, emphasizing the importance of breaking down the application into smaller, reusable parts. Look for examples of complex components they've built or managed.
5. How does Angular handle dependency injection?
Dependency injection in Angular is a design pattern that allows a class to receive its dependencies from external sources rather than creating them itself. Angular provides a built-in dependency injection framework to help manage and inject dependencies.
This framework allows for better code modularity, testability, and easier maintenance. Services and providers are often used in conjunction with dependency injection to manage shared data and logic across the application.
Look for a candidate's understanding of dependency injection and their experience in using it to manage dependencies in their applications. Follow up with questions about specific services or providers they've implemented.
6. Can you explain what Angular services are and how they are used?
Angular services are singleton objects that are used to share data and logic across components. They are typically used to encapsulate business logic, data access, and other reusable functions.
Services are injected into components using Angular's dependency injection system, which allows for easy sharing and management of data and functionality across the application.
An ideal response should include the candidate's experience in creating and using services, along with examples of how they've used services to manage state, perform API calls, or share data between components.
7. What is Angular CLI, and how does it help in Angular development?
Angular CLI (Command Line Interface) is a powerful tool that helps automate the development process. It provides commands for creating, building, testing, and deploying Angular applications.
Using Angular CLI, developers can generate components, services, and modules quickly, ensuring consistent project structure and following best practices. It also helps in managing dependencies and configurations.
Candidates should highlight their proficiency with Angular CLI and how it has streamlined their development workflow. Look for specific commands they frequently use and how they manage their projects using the CLI.
8. How do you implement routing in an Angular application?
Routing in Angular is implemented using the Angular Router module. It allows developers to define routes, which map URLs to components, enabling navigation within the application without reloading the page.
To set up routing, developers need to import the RouterModule and define route configurations. They can use routerLink directives in the template to navigate between routes and handle route parameters for dynamic routing.
Look for the candidate's experience in setting up and managing routing in their applications. They should be able to explain route guards, lazy loading, and handling not-found routes. Follow up with specific scenarios they've managed using routing.
9. What are Angular directives, and how are they different from components?
Angular directives are special markers in the DOM that tell Angular to do something to that element or its children. They are used to extend HTML's capabilities by adding behavior to elements.
There are three types of directives: components, structural directives (like *ngIf
and *ngFor
), and attribute directives (like ngClass
and ngStyle
). Components are a type of directive with a template, while other directives only modify the DOM behavior.
Candidates should explain their experience using and creating custom directives to enhance their applications' functionality. Look for examples of how they've used structural or attribute directives in their projects.
10. How do you handle forms in Angular applications?
Angular provides two ways to handle forms: template-driven forms and reactive forms. Template-driven forms are easy to set up and use directives to bind data to the form, while reactive forms provide more control and are highly scalable.
Reactive forms are created using the FormBuilder
service and allow for dynamic manipulation of form controls, validation, and handling complex form requirements. Template-driven forms are more suitable for simple scenarios.
Candidates should discuss their experience with both types of forms and explain scenarios where they've used each approach. Look for their understanding of form validation, error handling, and dynamic form creation.
20 Angular interview questions to ask junior developers
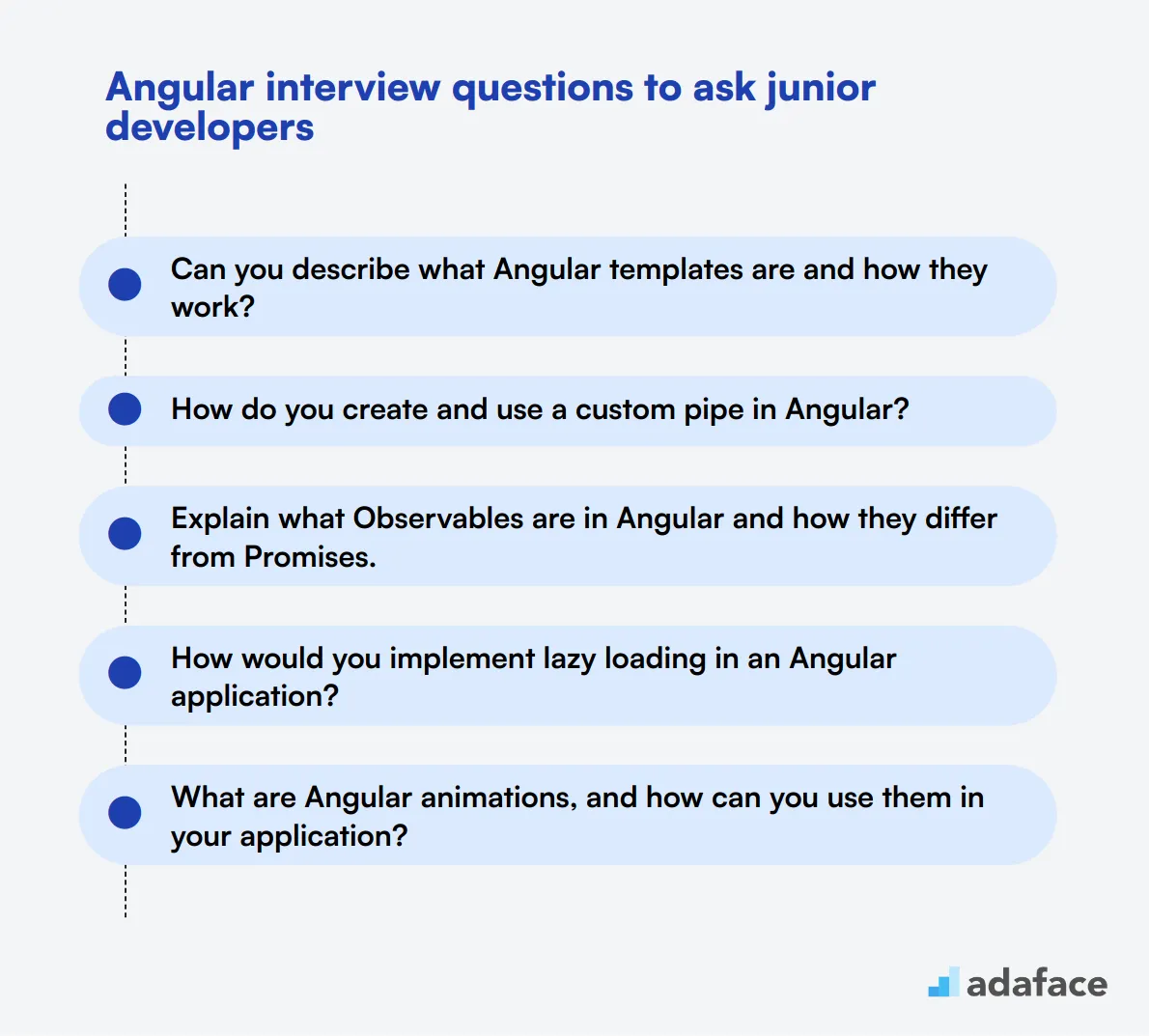
To evaluate whether your candidates possess the right skills and understanding for junior Angular developer roles, consider using these targeted questions. This list will help you identify their grasp of essential concepts and their ability to apply them in real-world scenarios. For more detailed job descriptions, check out this AngularJS developer job description.
- Can you describe what Angular templates are and how they work?
- How do you create and use a custom pipe in Angular?
- Explain what Observables are in Angular and how they differ from Promises.
- How would you implement lazy loading in an Angular application?
- What are Angular animations, and how can you use them in your application?
- Can you explain the difference between Angular's ngIf and ngSwitch directives?
- How do you manage state in an Angular application?
- Describe the purpose and usage of Angular lifecycle hooks.
- How do you handle HTTP requests in Angular?
- What is the difference between reactive forms and template-driven forms in Angular?
- How do you use Angular's HttpClient module?
- Can you explain what Angular interceptors are and how they can be used?
- How would you optimize the performance of an Angular application?
- What is Angular's Change Detection mechanism, and how does it work?
- Describe how to use Angular's Router Guards.
- How do you configure environment variables in an Angular project?
- Explain the concept of Angular zones and their significance.
- What are Angular pipes, and how do they help in data transformation?
- How can you handle error management in an Angular application?
- What is the difference between Angular's ViewChild and ContentChild decorators?
10 intermediate Angular interview questions and answers to ask mid-tier developers
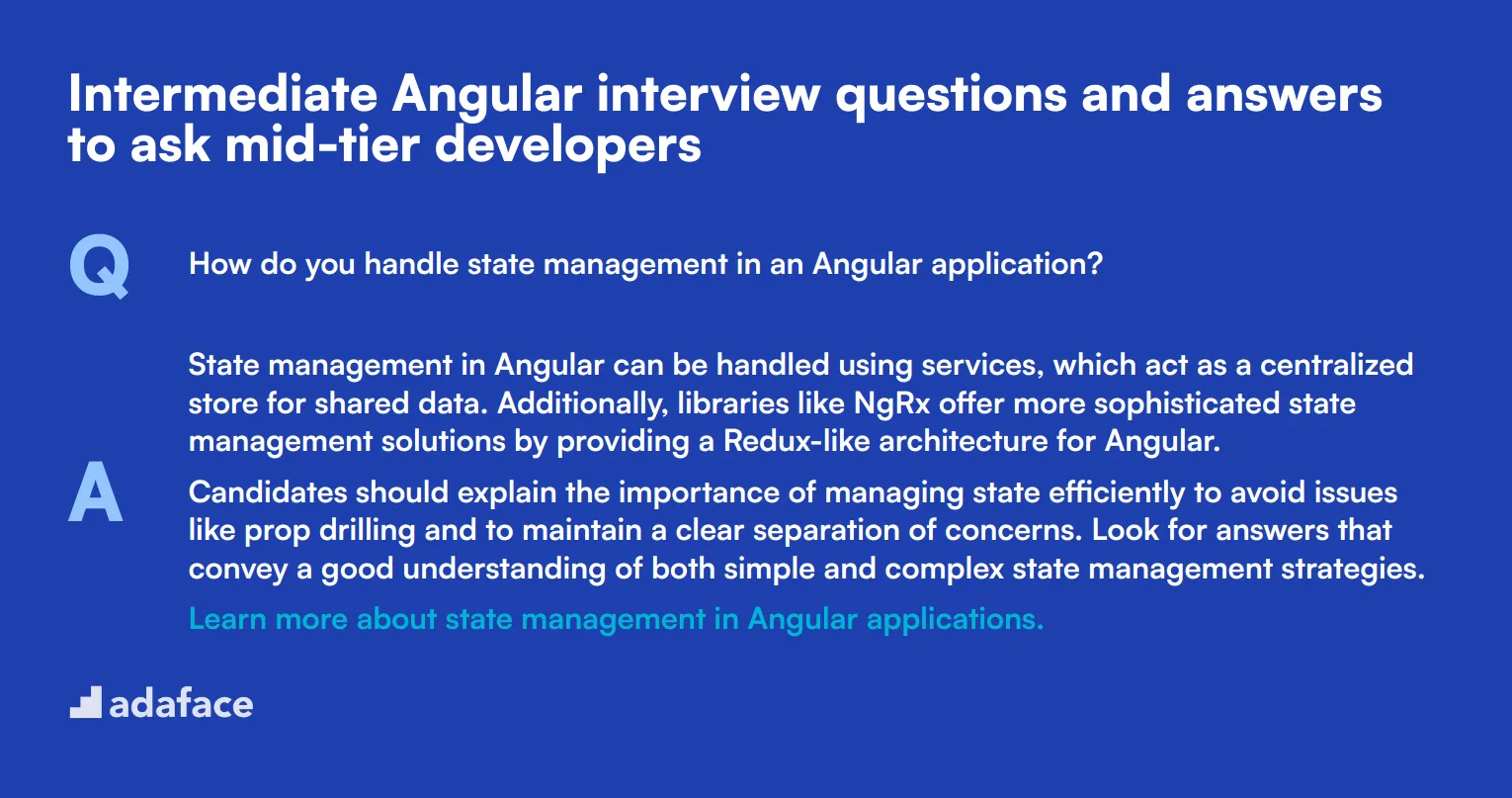
To assess mid-tier Angular developers, you need questions that dig a little deeper into their knowledge. The following list of intermediate Angular interview questions will help you gauge their ability to handle more complex tasks and scenarios in Angular development.
1. How do you handle state management in an Angular application?
State management in Angular can be handled using services, which act as a centralized store for shared data. Additionally, libraries like NgRx offer more sophisticated state management solutions by providing a Redux-like architecture for Angular.
Candidates should explain the importance of managing state efficiently to avoid issues like prop drilling and to maintain a clear separation of concerns. Look for answers that convey a good understanding of both simple and complex state management strategies.
Learn more about state management in Angular applications.
2. What are Angular Guards and how do you use them?
Angular Guards are interfaces that allow you to control navigation to and from certain routes. They can be used to protect routes by implementing logic that determines whether a route can be activated or deactivated.
There are several types of guards such as CanActivate
, CanDeactivate
, Resolve
, and CanLoad
. Each serves a different purpose and is used in different scenarios to ensure the right users access the right parts of the application.
Ideal candidates should describe scenarios where guards are necessary, such as preventing unauthorized users from accessing certain routes or ensuring data is fetched before a route is activated.
3. How do you optimize the performance of an Angular application?
Performance optimization in Angular can be achieved through various techniques such as lazy loading, ahead-of-time (AOT) compilation, and using OnPush change detection strategy. Minimizing the number of watchers and efficient use of Angular pipes also play a significant role.
Candidates should discuss the importance of bundle size and how tools like Webpack can be used to analyze and reduce it. They should also mention the use of Angular CLI commands for production builds that optimize code automatically.
Look for candidates who can explain not just the techniques but also the reasoning behind them, demonstrating a deeper understanding of how Angular operates under the hood.
4. Explain the concept of Angular zones and their significance.
Angular zones are a mechanism to keep track of changes in the application and automatically update the view when needed. They play a crucial role in Angular’s change detection mechanism.
Zone.js is the library used by Angular to implement zones. It patches asynchronous APIs like setTimeout
and HTTP requests
to notify Angular about these changes so it can update the view accordingly.
An ideal candidate should describe how zones help in managing asynchronous operations and discuss scenarios where managing zones manually might be required.
5. How do you handle error management in an Angular application?
Error management in Angular involves using try-catch blocks, error interceptors, and global error handlers. Services can be created to centralize error handling logic, making it easier to manage and report errors.
Candidates may also mention using Angular's HttpClient
module's catchError
operator to handle HTTP errors and displaying user-friendly error messages. Logging errors for further analysis is another crucial aspect.
Look for answers that demonstrate a comprehensive approach to error handling, including both user-facing and backend solutions.
6. What is the role of Angular services and how are they implemented?
Angular services are singleton objects that manage shared data and logic across different components. They are implemented using classes and can be injected into components or other services using Angular's Dependency Injection system.
Services help in maintaining a clean architecture by separating business logic from the view layer. They can be configured to be provided at the root level or at a specific module level, depending on the scope required.
An ideal candidate should explain scenarios where services are beneficial, such as data fetching, state management, or encapsulating reusable logic.
7. How do you implement and use Angular pipes?
Angular pipes are used to transform data in templates. Built-in pipes like date
, currency
, and uppercase
handle common data transformations, while custom pipes can be created for more specific needs.
Creating a custom pipe involves implementing the PipeTransform
interface and defining the transformation logic in the transform
method. Custom pipes can then be declared in a module and used across components.
Candidates should discuss scenarios where custom pipes are useful, such as formatting dates in a specific way or transforming data structures for display.
8. How do you implement routing in an Angular application?
Routing in Angular is implemented using the RouterModule
and Routes
array. Routes define the mapping between URL paths and components to be displayed. Angular’s router also supports nested routes and lazy loading for optimizing performance.
Candidates should explain the use of router directives like routerLink
and router-outlet
, as well as router events for tracking navigation changes.
Look for answers that demonstrate a solid understanding of routing configurations and the ability to manage complex routing scenarios efficiently.
9. Explain the concept of dependency injection in Angular.
Dependency Injection (DI) in Angular is a design pattern that allows a class to receive its dependencies from an external source rather than creating them itself. This promotes loose coupling and enhances testability and maintainability.
Angular’s DI framework uses decorators like @Injectable
for defining services and @Inject
for injecting dependencies. The DI system is hierarchical, allowing for different scopes of providers.
Candidates should discuss the benefits of DI, such as improved code modularity and the ability to easily swap out implementations for testing or different environments.
10. What are Angular lifecycle hooks and how are they used?
Angular lifecycle hooks are special methods that provide visibility into key moments in a component's lifecycle, such as creation, change detection, and destruction. Common hooks include ngOnInit
, ngOnChanges
, ngOnDestroy
, and more.
Using these hooks, developers can perform actions like initializing component data, responding to input changes, or cleaning up resources. Each hook serves a specific purpose and is called at a particular point in the component's lifecycle.
An ideal candidate should explain practical use cases for these hooks, such as initializing data in ngOnInit
or unsubscribing from observables in ngOnDestroy
to prevent memory leaks.
14 Angular interview questions about processes and tasks
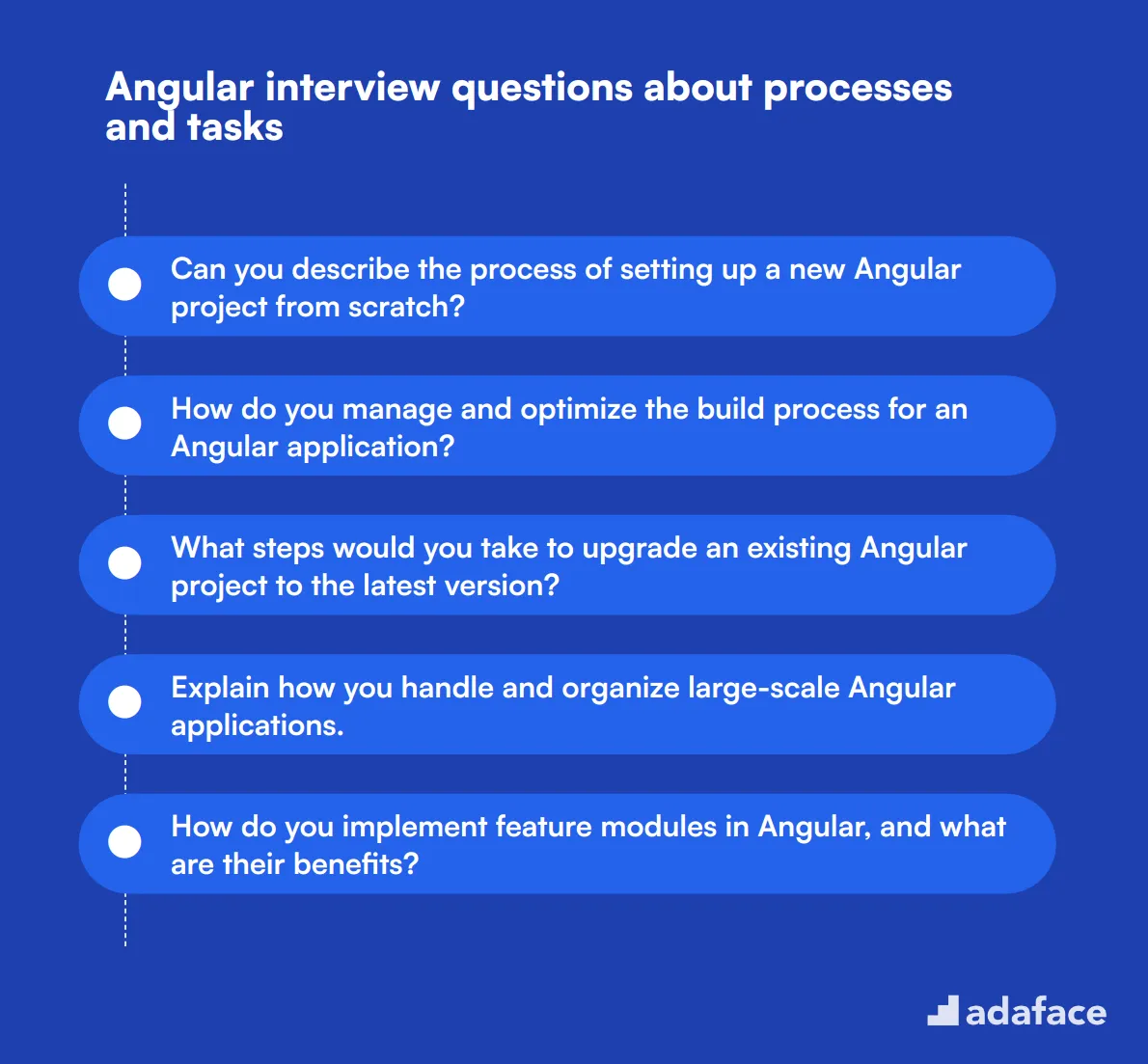
To assess whether your candidates possess the right skills to handle various tasks and processes in Angular development, use these 14 targeted Angular interview questions. They will help you gauge practical knowledge and problem-solving abilities, ensuring you hire the best fit for your team. For more insights, refer to the AngularJS Developer Job Description.
- Can you describe the process of setting up a new Angular project from scratch?
- How do you manage and optimize the build process for an Angular application?
- What steps would you take to upgrade an existing Angular project to the latest version?
- Explain how you handle and organize large-scale Angular applications.
- How do you implement feature modules in Angular, and what are their benefits?
- Can you walk me through the process of creating a reusable Angular library?
- Describe a situation where you had to debug a complex issue in an Angular application. How did you approach it?
- How do you manage different environments (development, staging, production) in an Angular project?
- What strategies do you use for state management in a complex Angular application?
- How do you optimize Angular applications for better performance and faster load times?
- Explain how you would handle user authentication and authorization in an Angular application.
- What are some best practices for testing Angular applications?
- How do you approach the integration of third-party libraries and APIs in an Angular project?
- Describe your process for code review and ensuring code quality in an Angular development team.
9 Angular interview questions and answers related to technical knowledge
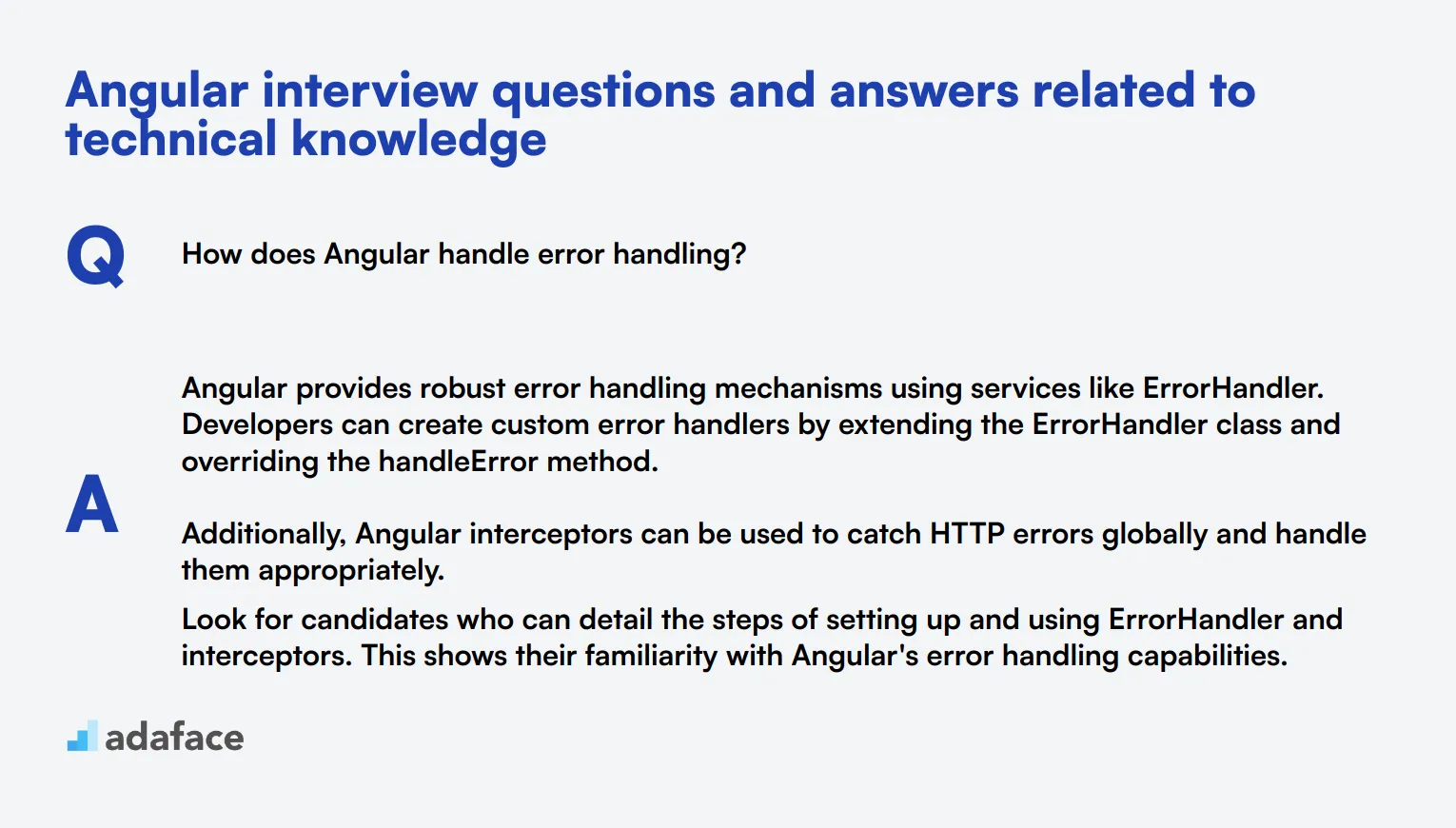
To ensure your candidates possess the right technical knowledge in Angular, use these interview questions. They are designed to test the fundamental concepts and practical understanding essential for any Angular developer role.
1. How does Angular handle error handling?
Angular provides robust error handling mechanisms using services like ErrorHandler. Developers can create custom error handlers by extending the ErrorHandler class and overriding the handleError method.
Additionally, Angular interceptors can be used to catch HTTP errors globally and handle them appropriately.
Look for candidates who can detail the steps of setting up and using ErrorHandler and interceptors. This shows their familiarity with Angular's error handling capabilities.
2. What is the role of Angular Universal, and how do you implement it?
Angular Universal is a technology that allows server-side rendering of Angular applications. This enhances the performance and SEO of web apps by rendering the initial view on the server.
To implement Angular Universal, you need to install the @nguniversal/express-engine package, create an Express server, and configure the server to handle Angular routes.
Ideal candidates should explain the benefits of server-side rendering and provide a high-level overview of the implementation steps, demonstrating their understanding of enhancing web app performance.
3. Can you explain how Angular handles event binding?
In Angular, event binding is used to handle user input in the template and respond to user actions. It involves binding an event, such as a click, to a method in the component using the parentheses syntax.
Event binding helps keep the component logic and template events synchronized, ensuring a responsive user interface.
Candidates should mention the syntax and provide a clear explanation of how event binding works within Angular applications. This showcases their grasp of interactive web design.
4. What is Ahead-of-Time (AOT) compilation in Angular, and why is it important?
Ahead-of-Time (AOT) compilation is a process that compiles Angular templates and components during the build phase, before the browser downloads and runs the code. This results in faster rendering and improved performance.
AOT helps in catching template errors early, reducing the payload size, and enhancing the security of the application by eliminating the need to ship the Angular compiler to the client.
An ideal candidate will highlight the benefits of AOT and explain why it is a critical step in optimizing Angular applications, indicating their understanding of performance improvement techniques.
5. How do you manage dependencies in Angular projects?
Dependencies in Angular are managed using the package manager npm (Node Package Manager). Developers can specify dependencies in the package.json file and use commands like npm install to manage and update them.
Angular CLI also plays a significant role in managing dependencies by providing commands to add or remove packages seamlessly.
Look for candidates who can explain the role of npm and Angular CLI in dependency management and describe their process for keeping dependencies updated and secure. This reflects their organizational skills and attention to detail.
6. What is Angular Ivy, and what benefits does it bring?
Angular Ivy is the latest rendering engine for Angular, introduced to improve performance and reduce bundle size. Ivy compiles components more efficiently and provides better debugging capabilities.
Benefits of Angular Ivy include faster compilation times, smaller bundle sizes, improved template type-checking, and enhanced backward compatibility.
Candidates should be able to explain the advantages of Ivy and how it impacts the development lifecycle. This shows their awareness of advancements in the Angular ecosystem.
7. Can you explain the concept of Angular zones and their significance?
Angular zones, managed by the Zone.js library, are a mechanism for detecting and responding to asynchronous operations like HTTP requests and user events. Zones help Angular keep track of changes and update the view accordingly.
By wrapping asynchronous operations, zones ensure that Angular's change detection runs automatically when these operations complete.
Candidates should discuss the importance of zones in maintaining the synchronization between the model and the view, highlighting their understanding of Angular's core change detection mechanism.
8. How do you implement internationalization (i18n) in Angular applications?
Internationalization (i18n) in Angular involves translating the user interface of an application into different languages. Angular provides built-in support for i18n through the Angular i18n library, using translation files and the i18n attribute in templates.
Steps to implement i18n include marking the text for translation, generating translation files, translating the content, and building the application for different locales.
Ideal candidates should outline the process and tools involved in implementing i18n, demonstrating their capability to develop applications for a global audience.
9. What are Angular decorators, and how are they used?
Angular decorators are special declarations that attach metadata to classes, properties, methods, and parameters. They are used to configure and modify Angular components, services, and other entities.
Common decorators include @Component, @Directive, @Injectable, and @Pipe. These decorators help Angular understand how to instantiate and use the decorated elements.
Look for candidates who can explain the purpose and usage of different Angular decorators, showcasing their knowledge of Angular's architecture and how it leverages decorators for dependency injection and component configuration.
Which Angular skills should you evaluate during the interview phase?
While it's impossible to assess every aspect of a candidate's Angular expertise in a single interview, focusing on core skills can provide valuable insights. Here are key Angular skills to evaluate during the interview process.
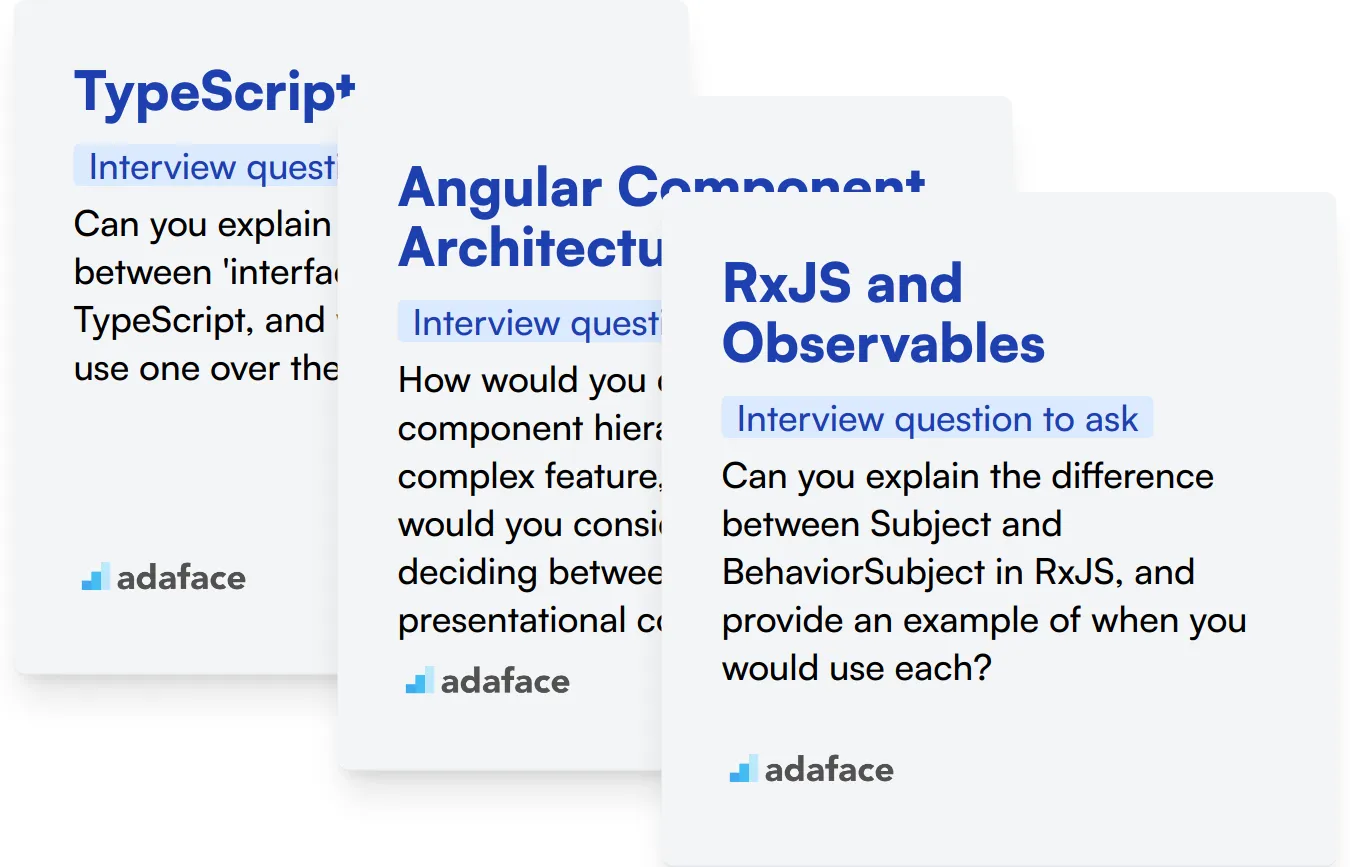
TypeScript
TypeScript is the primary language used in Angular development. It adds static typing to JavaScript, enhancing code quality and maintainability in large-scale applications.
To assess TypeScript proficiency, consider using an online assessment with relevant multiple-choice questions. This can help filter candidates based on their TypeScript knowledge.
During the interview, you can ask targeted questions to gauge the candidate's TypeScript understanding. Here's an example:
Can you explain the difference between 'interface' and 'type' in TypeScript, and when would you use one over the other?
Look for answers that demonstrate understanding of structural typing, declaration merging, and use cases for each. A strong candidate will explain how interfaces are often used for object shapes, while types offer more flexibility for unions and intersections.
Angular Component Architecture
Understanding component architecture is fundamental to building scalable Angular applications. It involves creating reusable, modular components and managing their interactions.
An Angular-specific assessment can help evaluate a candidate's grasp of component architecture through targeted questions.
To further assess this skill during the interview, consider asking:
How would you design a component hierarchy for a complex feature, and what factors would you consider when deciding between smart and presentational components?
Listen for responses that demonstrate knowledge of component communication, state management, and separation of concerns. Strong candidates will discuss data flow, reusability, and maintainability in their component design approach.
RxJS and Observables
RxJS is integral to Angular for handling asynchronous operations and managing data streams. Proficiency in RxJS and Observables is crucial for effective Angular development.
Consider using an RxJS-focused test to evaluate candidates' understanding of reactive programming concepts.
To assess RxJS skills during the interview, you might ask:
Can you explain the difference between Subject and BehaviorSubject in RxJS, and provide an example of when you would use each?
Look for answers that demonstrate understanding of hot vs cold observables, multicast behavior, and state management. Strong candidates will provide clear use cases and explain the implications of using each type in an Angular application.
Hire top Angular developers with skills tests and targeted interview questions
Looking to hire someone with Angular skills? It's important to ensure candidates truly possess the required expertise. Accurate assessment of Angular proficiency is key to building a strong development team.
The most effective way to evaluate Angular skills is through specialized tests. Consider using the Angular online test or Front-end developer test to gauge candidates' abilities objectively.
After administering these tests, you can shortlist the top-performing applicants for interviews. This approach saves time and ensures you're only speaking with candidates who have demonstrated their Angular capabilities.
Ready to streamline your Angular hiring process? Sign up to access our comprehensive test library and assessment tools. For more information on our coding assessments, visit our coding tests page.
Angular Online Test
Download Angular interview questions template in multiple formats
Angular Interview Questions FAQs
An Angular developer should be proficient in TypeScript, HTML, CSS, and have a strong understanding of Angular framework and its core concepts.
You can evaluate Angular skills by asking questions about basics, processes, tasks, and technical knowledge. Additionally, practical coding tests can be helpful.
Process-related questions help understand the candidate's approach to development, problem-solving, and their ability to work within a team or workflow.
The number of questions can vary, but having a mix of 10-20 questions covering different skill levels and aspects can give a comprehensive understanding of the candidate's abilities.
Yes, including coding tests can provide practical insight into the candidate's coding abilities and how they apply their knowledge in real-world scenarios.
Junior developers can be assessed with basic and foundational questions, as well as through tasks that demonstrate their understanding of core Angular concepts.
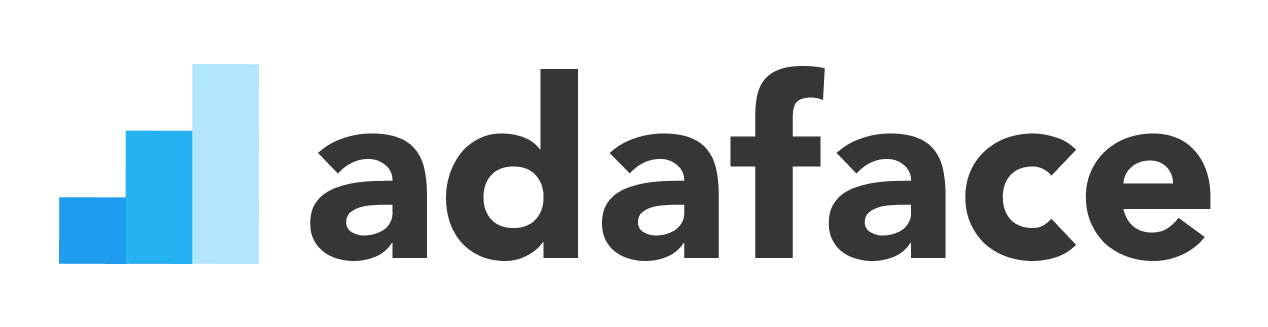
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
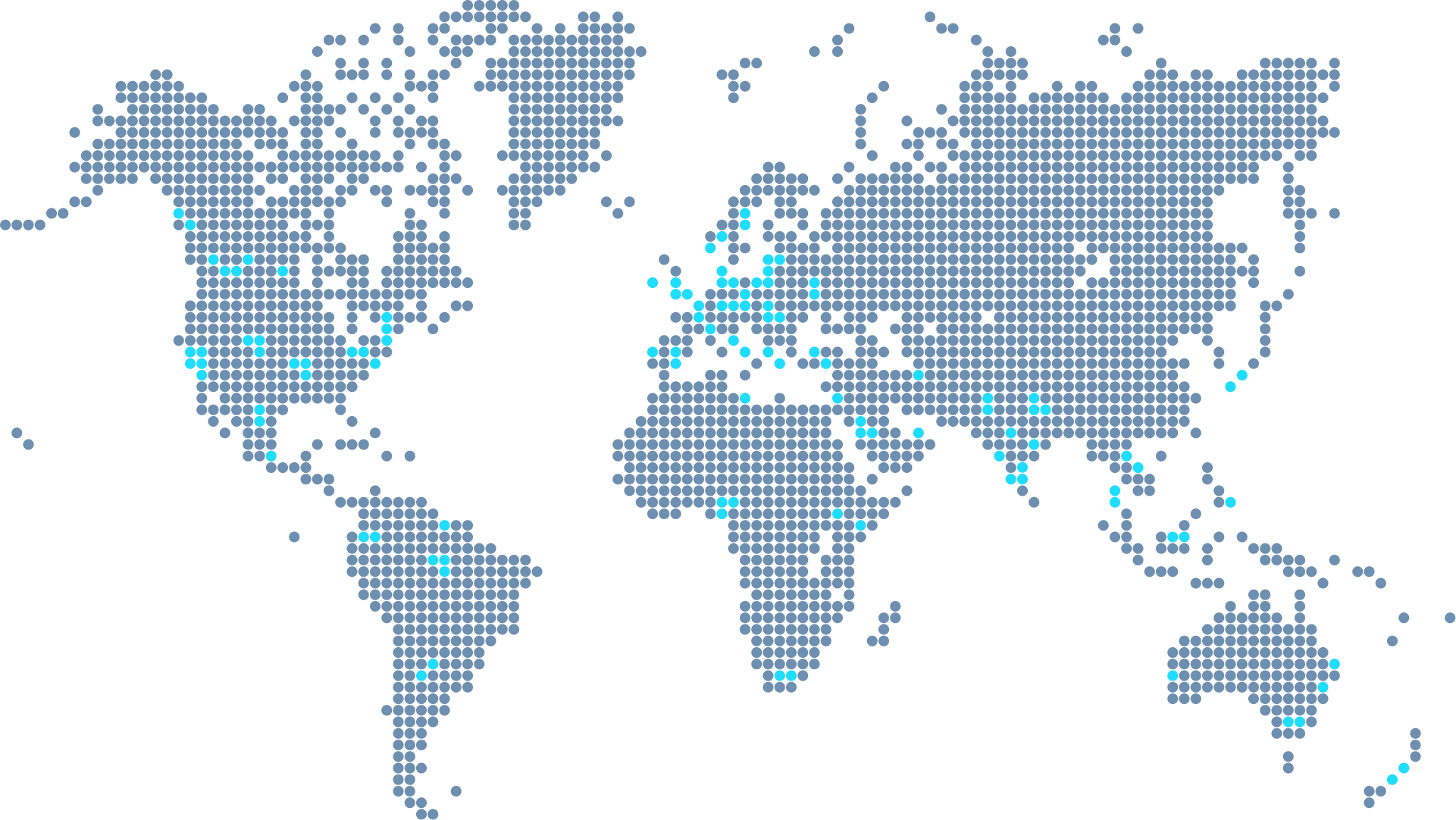
