Recruiting the right database professionals for your team involves not just evaluating technical expertise but also ensuring a productive fit within your existing structure. One crucial aspect of this process is honing in on the specific skills required for SQL developers.
In this blog post, we've compiled a comprehensive set of T-SQL interview questions tailored for different experience levels and focus areas. From basic and junior developer questions to those concerning query optimization and transactions, this guide is structured to help you thoroughly assess your candidates.
By utilizing these curated questions, you can streamline your interview process and make more informed hiring decisions. Additionally, consider integrating the T-SQL skills test from Adaface to identify strong candidates before they even step into the interview room.
Table of contents
15 basic T-SQL interview questions and answers to assess candidates
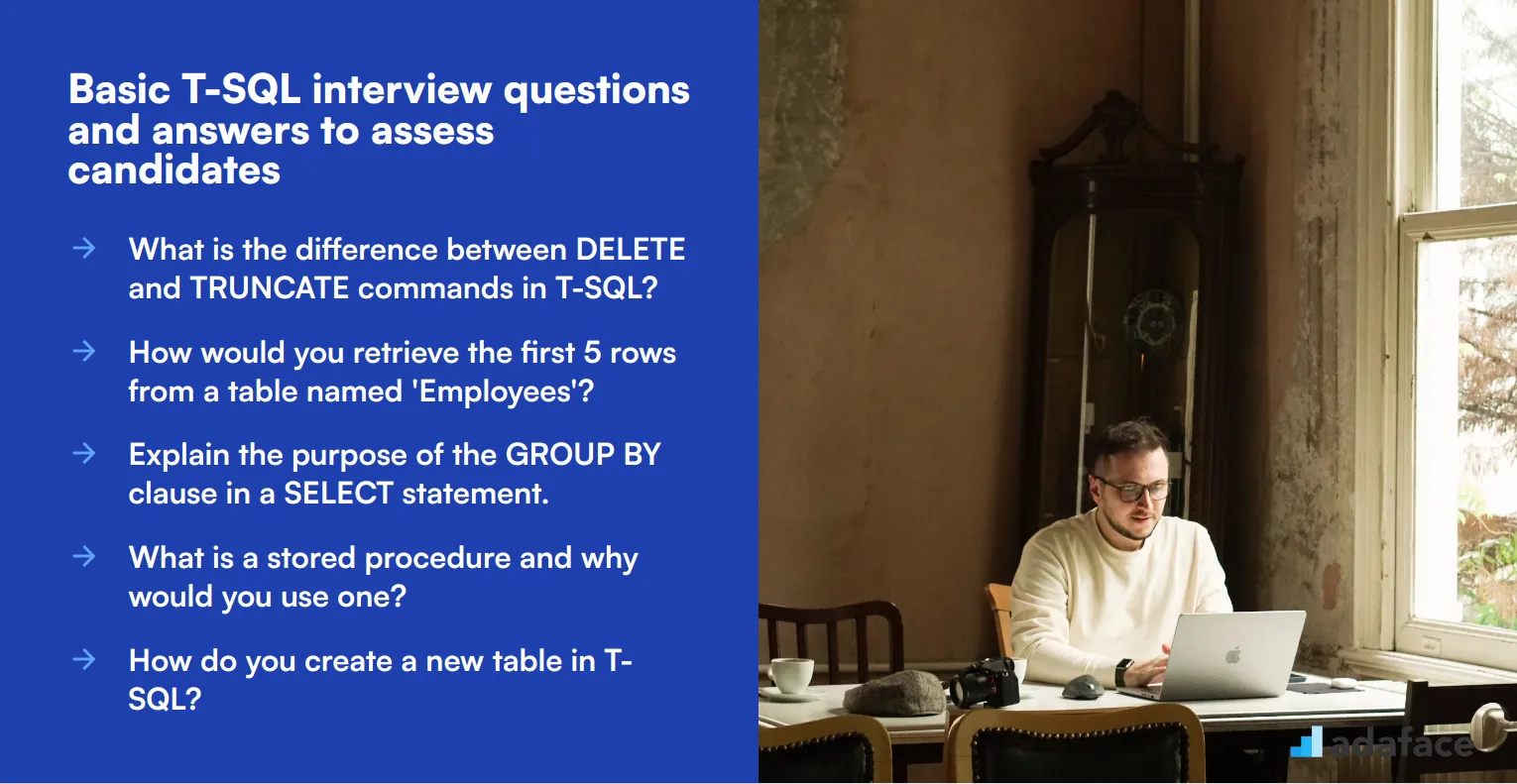
To assess candidates' foundational knowledge in T-SQL, use these 15 basic interview questions. They will help you gauge a potential SQL developer's understanding of core concepts and their ability to apply them in real-world scenarios. These questions are designed to be straightforward yet revealing.
- What is the difference between DELETE and TRUNCATE commands in T-SQL?
- How would you retrieve the first 5 rows from a table named 'Employees'?
- Explain the purpose of the GROUP BY clause in a SELECT statement.
- What is a stored procedure and why would you use one?
- How do you create a new table in T-SQL?
- What's the difference between UNION and UNION ALL?
- Explain the concept of a primary key in a database table.
- How would you find duplicate records in a table?
- What is the purpose of the HAVING clause in a SQL query?
- How do you join two tables in T-SQL?
- What is the difference between a clustered and non-clustered index?
- How would you convert a string to a date in T-SQL?
- Explain the concept of a subquery and provide a simple example.
- What is the purpose of the COALESCE function in T-SQL?
- How would you handle NULL values in a WHERE clause?
8 T-SQL interview questions and answers to evaluate junior database developers
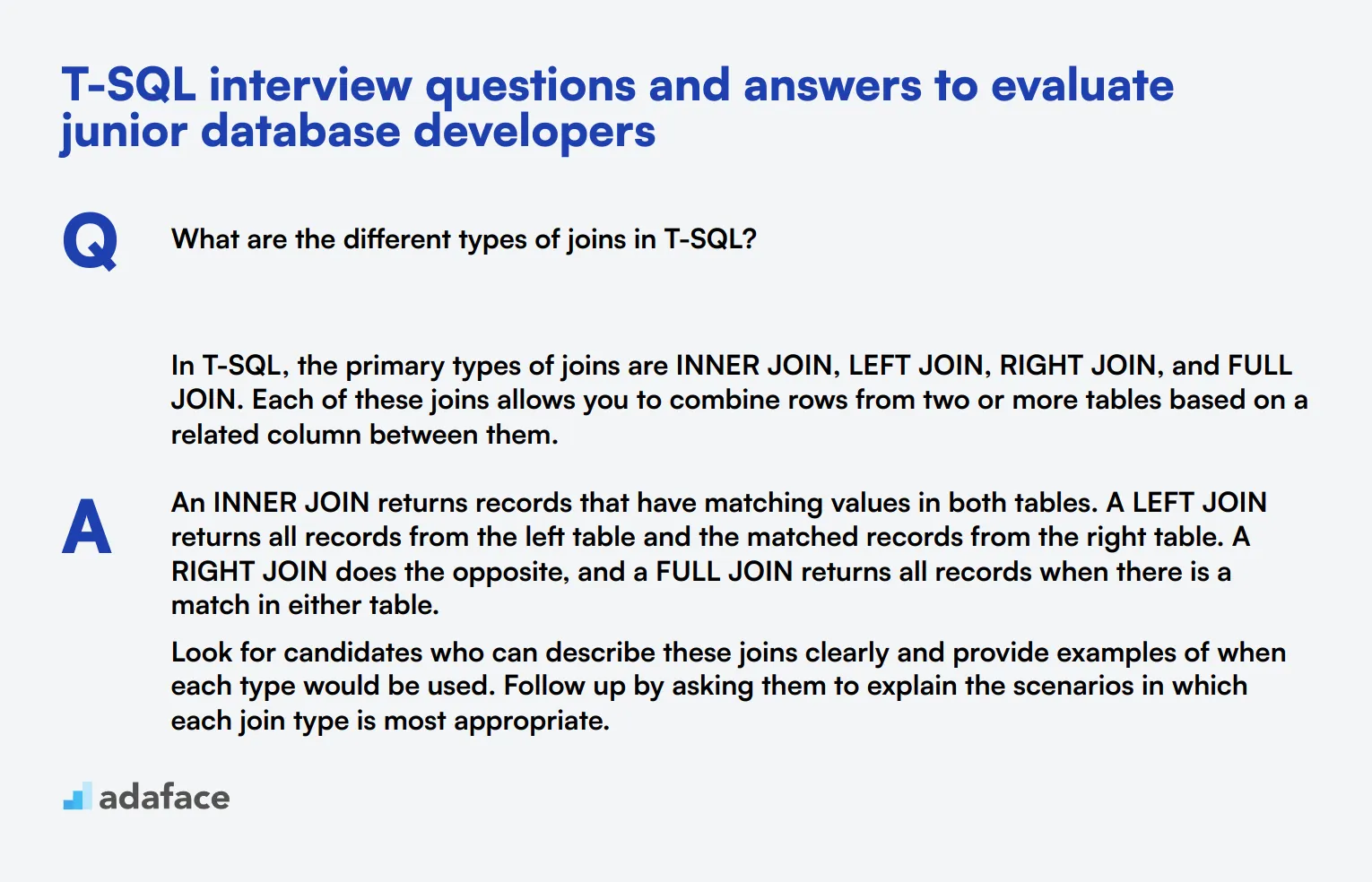
Looking to assess the T-SQL skills of your junior database developer applicants? These 8 interview questions will help you measure their foundational knowledge and problem-solving abilities, ensuring they have the right skills to contribute effectively to your team.
1. What are the different types of joins in T-SQL?
In T-SQL, the primary types of joins are INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN. Each of these joins allows you to combine rows from two or more tables based on a related column between them.
An INNER JOIN returns records that have matching values in both tables. A LEFT JOIN returns all records from the left table and the matched records from the right table. A RIGHT JOIN does the opposite, and a FULL JOIN returns all records when there is a match in either table.
Look for candidates who can describe these joins clearly and provide examples of when each type would be used. Follow up by asking them to explain the scenarios in which each join type is most appropriate.
2. Can you explain what a view is in T-SQL and why you might use one?
A view in T-SQL is a virtual table that is based on the result-set of an SQL statement. It can include data from one or more tables and is used to simplify complex queries, enhance security by restricting data access, and provide a consistent, abstracted view of the data.
You might use a view to encapsulate complex joins and subqueries, making it easier for users to query data without needing to know the underlying structure. Views also help in maintaining data security as they can expose only selected columns of the data.
Ideal candidates should be able to explain the practical uses of views, focusing on scenarios where they simplify query management and improve data security. They should also mention how views can contribute to efficiency in database management.
3. What is the purpose of the DISTINCT keyword in T-SQL?
The DISTINCT keyword is used in T-SQL to remove duplicate records from the result set of a query. It ensures that the results returned are unique.
For instance, if you have a list of names with duplicates, using DISTINCT will give you a list with only unique names. This is useful for reporting purposes where you need to ensure that the data presented is unique and not repeated.
Look for candidates who can explain when and why they would use DISTINCT. Follow up by asking about its impact on performance and whether there are any potential downsides to using it in large datasets.
4. How can you improve the performance of a slow-running query in T-SQL?
Improving the performance of a slow-running query can be done through several methods, such as optimizing the query structure, creating indexes, avoiding unnecessary columns in SELECT statements, and using joins efficiently.
Other strategies include analyzing query execution plans to identify bottlenecks, updating statistics, and ensuring proper indexing. Writing more specific WHERE clauses to reduce the number of rows processed can also help improve performance.
An ideal candidate should mention these strategies and possibly share examples of how they've optimized queries in the past. Look for their understanding of the trade-offs involved and how they balance performance with other factors like maintainability.
5. Explain what a transaction is in T-SQL and why it is important.
A transaction in T-SQL is a sequence of one or more SQL operations executed as a single unit of work. Transactions ensure that all operations within that sequence either complete successfully or none of them do, maintaining data integrity.
Transactions are important for consistency and reliability, especially in environments where multiple users are accessing and modifying the database simultaneously. They help prevent data corruption and ensure that the database remains in a consistent state.
Candidates should be able to explain the ACID properties (Atomicity, Consistency, Isolation, Durability) of transactions and why these properties are crucial for database operations. Follow up by asking about how they manage transactions in real-world scenarios.
6. What is normalization, and why is it important in database design?
Normalization is the process of organizing data in a database to reduce redundancy and improve data integrity. It involves dividing large tables into smaller, related tables and defining relationships between them.
The primary goal of normalization is to eliminate duplicate data and ensure that data dependencies make sense. This helps in maintaining consistency and avoiding anomalies during data operations such as insertions, updates, and deletions.
Look for candidates who can explain the different normal forms (1NF, 2NF, 3NF, etc.) and why each form is important. Follow up by asking for examples of how they've implemented normalization in their previous projects.
7. How would you handle error handling in T-SQL?
Error handling in T-SQL can be managed using TRY...CATCH blocks. Within the TRY block, you place the SQL statements that may cause errors, and within the CATCH block, you handle any errors that occur.
In the CATCH block, you can use functions like ERROR_MESSAGE(), ERROR_NUMBER(), and ERROR_LINE() to get details about the error and take appropriate actions such as logging the error or rolling back transactions.
Candidates should be able to discuss the importance of error handling for maintaining robust and reliable database applications. They should mention best practices and how they've implemented error handling in their own projects.
8. What do you understand by data integrity, and how do you enforce it in T-SQL?
Data integrity refers to the accuracy and consistency of data stored in a database. It ensures that the data remains correct and reliable throughout its lifecycle.
In T-SQL, data integrity can be enforced using constraints such as PRIMARY KEY, FOREIGN KEY, UNIQUE, CHECK, and DEFAULT constraints. These constraints help in maintaining valid data by restricting what can be entered into the database tables.
An ideal candidate should explain how they use these constraints to enforce data integrity and provide examples of how they've dealt with data integrity issues in past projects. Follow up by asking about any challenges they've faced and how they overcame them.
12 T-SQL questions related to query optimization
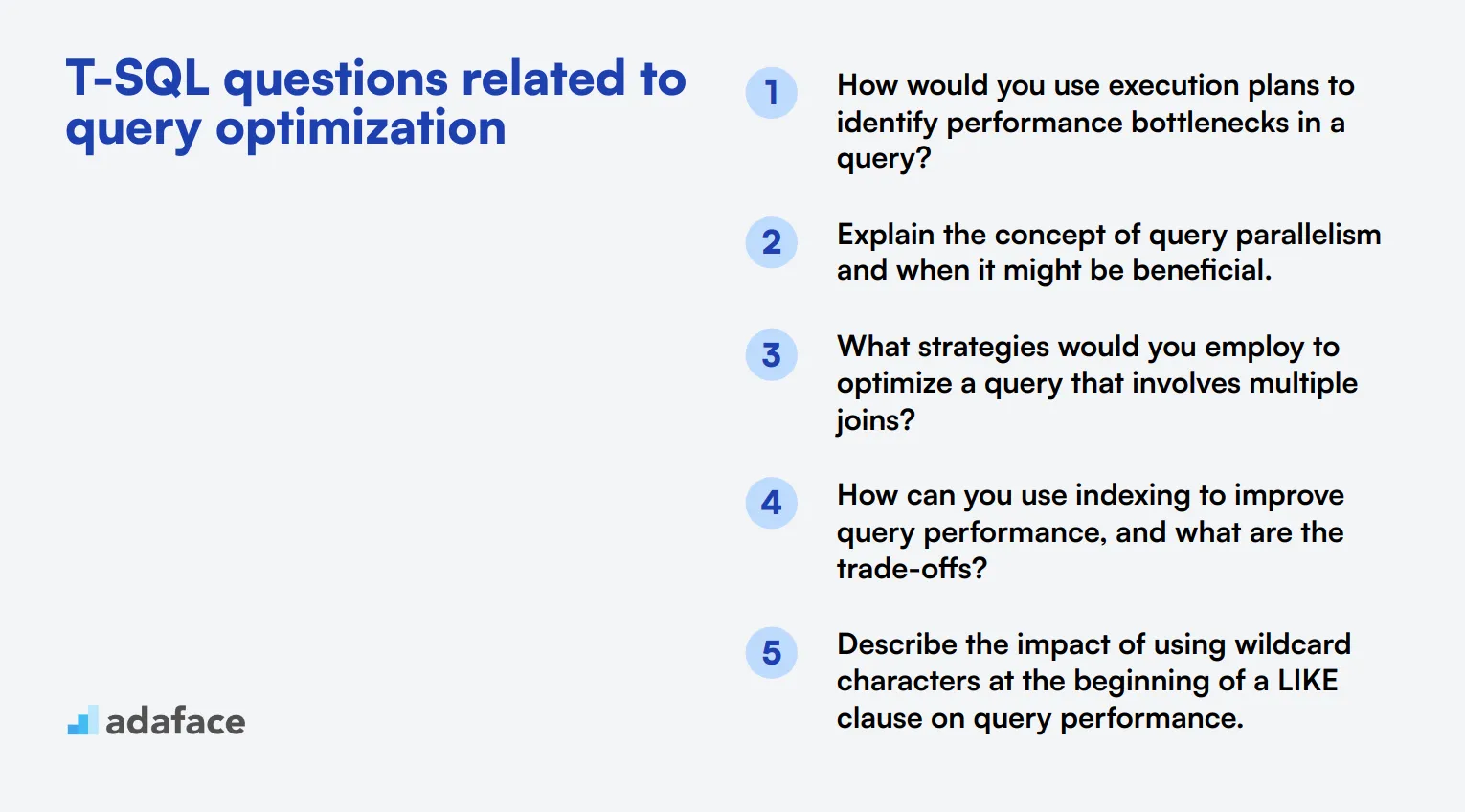
To assess a candidate's ability to optimize SQL queries, use these 12 T-SQL questions focused on query performance. These questions will help you evaluate an applicant's understanding of efficiency techniques and their capacity to improve database operations.
- How would you use execution plans to identify performance bottlenecks in a query?
- Explain the concept of query parallelism and when it might be beneficial.
- What strategies would you employ to optimize a query that involves multiple joins?
- How can you use indexing to improve query performance, and what are the trade-offs?
- Describe the impact of using wildcard characters at the beginning of a LIKE clause on query performance.
- How does the NOLOCK hint affect query execution, and when should it be used?
- Explain the benefits and potential drawbacks of using temporary tables in complex queries.
- How can you use the OPTION (RECOMPILE) query hint to potentially improve performance?
- What is parameter sniffing, and how can it affect query performance?
- How would you approach optimizing a query that uses a scalar user-defined function?
- Explain the concept of statistics in SQL Server and how they impact query performance.
- How can you use the MERGE statement to improve the performance of UPSERT operations?
7 T-SQL interview questions and answers related to transactions
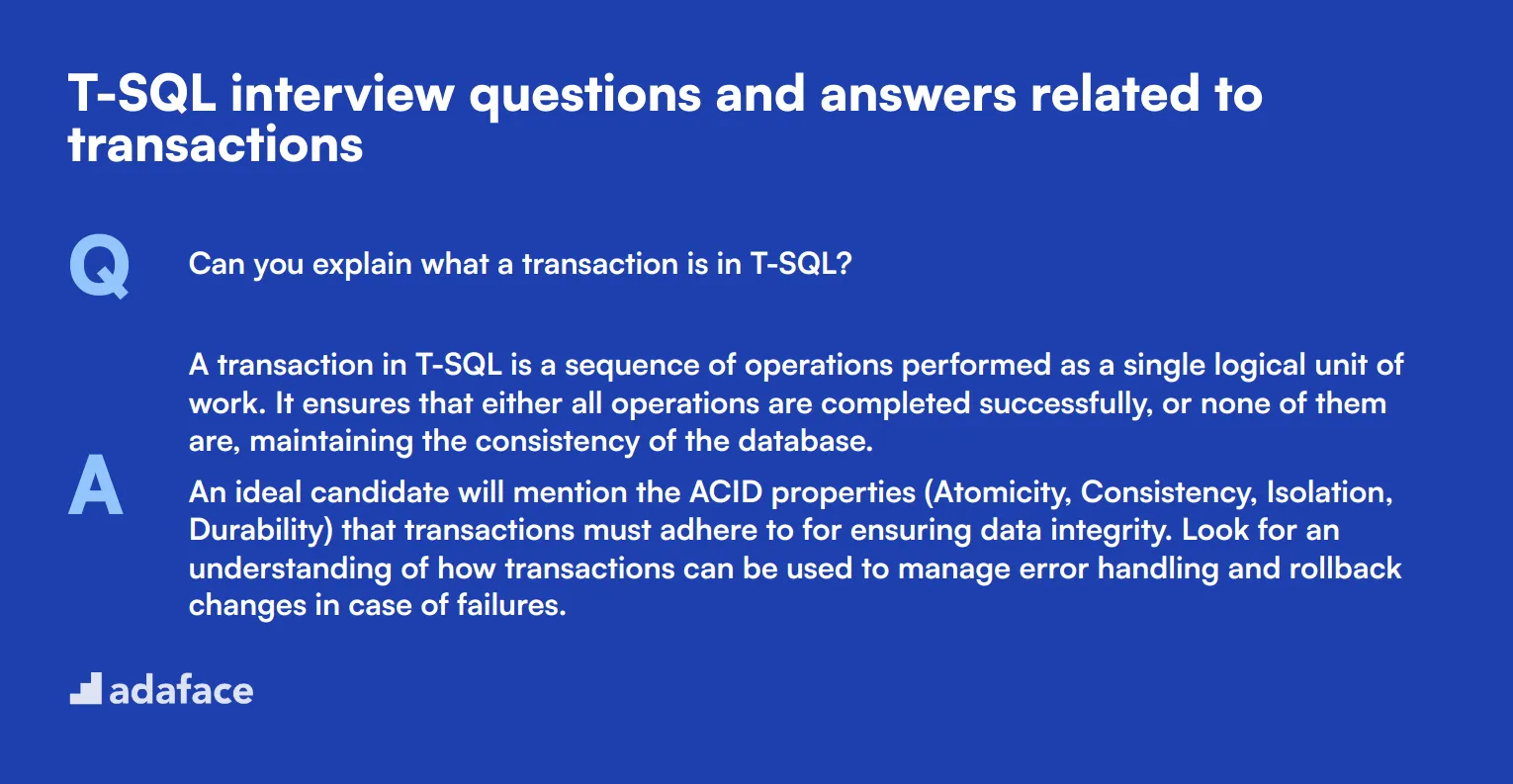
To determine whether your applicants have the right understanding of T-SQL transactions, ask them some of these 7 interview questions. These questions are designed to uncover their knowledge and practical experience with transactions, which are crucial for maintaining data integrity and consistency.
1. Can you explain what a transaction is in T-SQL?
A transaction in T-SQL is a sequence of operations performed as a single logical unit of work. It ensures that either all operations are completed successfully, or none of them are, maintaining the consistency of the database.
An ideal candidate will mention the ACID properties (Atomicity, Consistency, Isolation, Durability) that transactions must adhere to for ensuring data integrity. Look for an understanding of how transactions can be used to manage error handling and rollback changes in case of failures.
2. What is the difference between COMMIT and ROLLBACK in T-SQL transactions?
COMMIT and ROLLBACK are commands used to manage transactions. COMMIT makes all the changes made in the transaction permanent, while ROLLBACK undoes all the changes made during the transaction.
Strong candidates should illustrate scenarios where each command would be appropriately used. Look for an understanding of how these commands help maintain data integrity in different situations.
3. How would you handle errors within a transaction in T-SQL?
Handling errors within a transaction typically involves using TRY...CATCH blocks. The TRY block contains the code that might throw an error, while the CATCH block contains the code to handle the error, such as rolling back the transaction.
Candidates should mention the importance of error handling to ensure data consistency and should be able to describe how to use TRY...CATCH blocks effectively. Look for an understanding of how to log errors and perform cleanup operations within the CATCH block.
4. Why is it important to use transactions in database operations?
Transactions are important because they ensure data integrity by making sure that a series of database operations are either all completed successfully or none at all. This helps prevent data corruption and maintains consistency.
An ideal candidate will mention the ACID properties and provide examples of scenarios where transactions are crucial, such as multi-step updates or financial transactions. Look for an understanding of how transactions help in maintaining a reliable and consistent database state.
5. What is a nested transaction, and how does it behave in T-SQL?
A nested transaction occurs when a new transaction is started within the scope of an already active transaction. In T-SQL, only the outermost transaction can be committed or rolled back, while inner transactions rely on the outer transaction's success or failure.
Candidates should explain how nested transactions can be useful for modularizing code but also highlight the potential pitfalls, such as increased complexity and potential deadlocks. Look for an understanding of the COMMIT and ROLLBACK behavior in nested transactions.
6. What are savepoints and how are they used in T-SQL transactions?
Savepoints are markers within a transaction that allow for partial rollbacks. By setting savepoints, you can rollback to a specific point within the transaction without rolling back the entire transaction.
Candidates should describe how savepoints can be useful for complex transactions where partial rollbacks are required. Look for an understanding of how to create, use, and release savepoints effectively in T-SQL.
7. How do isolation levels affect transactions in T-SQL?
Isolation levels determine the degree to which the operations in one transaction are isolated from those in other transactions. T-SQL supports several isolation levels, including Read Uncommitted, Read Committed, Repeatable Read, and Serializable.
Strong answers will explain how each isolation level affects concurrency and data consistency. Look for an understanding of the trade-offs between higher isolation and performance, and how to choose the appropriate isolation level for different scenarios.
Which T-SQL skills should you evaluate during the interview phase?
It’s impossible to assess every facet of a candidate’s T-SQL expertise in a single interview. However, focusing on a few core skills can give you valuable insights into their capabilities and fit for the role. Here are the essential T-SQL skills you should evaluate during the interview phase:
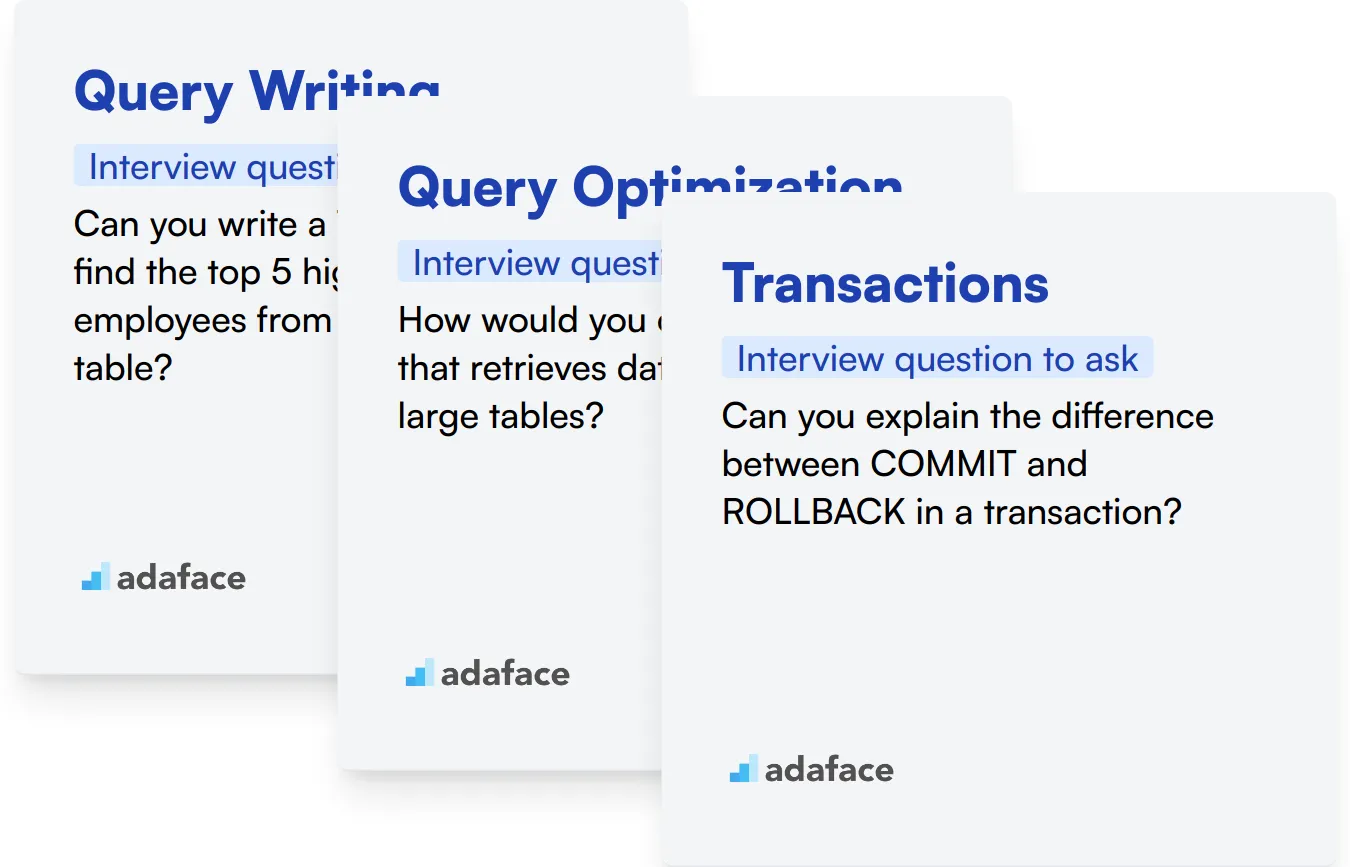
Query Writing
Query writing is the backbone of T-SQL. It demonstrates a candidate’s ability to retrieve and manipulate data, which is essential for any database-related role. Skilled query writing ensures efficient and accurate data retrieval, enabling better decision-making and reporting.
Consider using an assessment test that includes multiple-choice questions tailored to evaluate query writing skills. This can help you filter out candidates who possess the necessary foundational knowledge. Our T-SQL test includes relevant MCQs on this topic.
You can also ask targeted questions during the interview to evaluate the candidate’s query writing skills.
Can you write a T-SQL query to find the top 5 highest-paid employees from the Employees table?
Look for candidates who can structure their query correctly, utilize appropriate SQL functions, and demonstrate an understanding of sorting and filtering data efficiently.
Query Optimization
Query optimization is critical for ensuring that the database performs efficiently. A candidate who understands query optimization can help minimize the load on the database and reduce response times, thereby improving overall system performance.
Using an assessment test with MCQs on query optimization can help you identify candidates familiar with best practices in writing efficient T-SQL queries. Our T-SQL test offers questions on this subject.
Ask specific questions to gauge the candidate’s expertise in optimizing T-SQL queries.
How would you optimize a query that retrieves data from multiple large tables?
Effective answers will discuss indexing, query refactoring, and other optimization techniques. This will help you understand the candidate’s depth of knowledge in improving query performance.
Transactions
Understanding transactions is essential for maintaining data integrity and consistency. A candidate proficient in T-SQL transactions can ensure that database operations are performed reliably and can handle potential issues like deadlocks and rollbacks.
You can use an assessment test with specific questions on transactions to identify candidates who are knowledgeable in this area. Our T-SQL test includes relevant questions on transactions.
In the interview, ask questions that will reveal the candidate’s understanding and practical experience with T-SQL transactions.
Can you explain the difference between COMMIT and ROLLBACK in a transaction?
Good answers will clearly describe the purpose of each command, when to use them, and how they help maintain data integrity, reflecting the candidate’s practical knowledge of T-SQL transactions.
Maximizing the Effectiveness of T-SQL Interview Questions
Before putting your newfound knowledge to use, consider these tips to enhance your T-SQL interview process. These strategies will help you make the most of your candidate evaluations.
1. Implement Skills Tests Before Interviews
Skills tests provide an objective measure of a candidate's T-SQL proficiency before the interview stage. This approach saves time and ensures you're interviewing candidates with the necessary technical skills.
For T-SQL roles, consider using a T-SQL test or an SQL Server test to evaluate candidates' database skills. These tests can assess query writing, database design, and performance optimization abilities.
By using skills tests, you can create a shortlist of qualified candidates and tailor your interview questions to each candidate's strengths and weaknesses. This method allows for more focused and productive interviews.
2. Prepare a Balanced Set of Interview Questions
When compiling your interview questions, aim for a mix that covers various aspects of T-SQL and related skills. Include questions on query optimization, transaction management, and database design to get a well-rounded view of the candidate's expertise.
Consider incorporating questions from related areas such as database administration or data modeling. This approach helps assess the candidate's broader understanding of database systems and their ability to work in a larger tech ecosystem.
Don't forget to include questions that evaluate soft skills like problem-solving and communication. These are crucial for database developers who often need to collaborate with other team members and explain complex concepts to non-technical stakeholders.
3. Master the Art of Follow-up Questions
Asking follow-up questions is key to understanding a candidate's depth of knowledge and problem-solving approach. It helps you distinguish between candidates who have memorized answers and those who truly understand T-SQL concepts.
For example, if you ask about indexing in T-SQL, a follow-up question could be, 'Can you describe a situation where adding an index might not improve query performance?' This probes the candidate's practical experience and understanding of database optimization nuances.
Hire top T-SQL talent with targeted interview questions and skill tests
To hire someone with strong T-SQL skills, you need to assess their abilities accurately. The most effective way to do this is by using skill tests. Consider using our T-SQL test or SQL Server online test to evaluate candidates' proficiency.
After using these tests to shortlist the best applicants, you can invite them for interviews. To streamline your hiring process and find the right talent, explore our online assessment platform or sign up to get started with skill assessments today.
T SQL Online Test
Download T-SQL interview questions template in multiple formats
T-SQL Interview Questions FAQs
Some basic T-SQL questions include asking about the differences between SQL and T-SQL, understanding of joins, and basic CRUD operations.
Focus on questions related to basic SQL commands, understanding of primary keys and foreign keys, and simple query writing to assess a junior developer's skills.
Ask about indexing strategies, query execution plans, and how to optimize slow queries to evaluate a candidate's query optimization skills.
Questions about transaction control commands, understanding of ACID properties, and how to handle deadlocks are useful in assessing knowledge of transactions.
Using a variety of questions helps in assessing the candidate's overall expertise, problem-solving abilities, and their understanding of different aspects of T-SQL.
Make sure your questions are relevant to the job role, cover different difficulty levels, and are designed to gauge both theoretical understanding and practical skills.
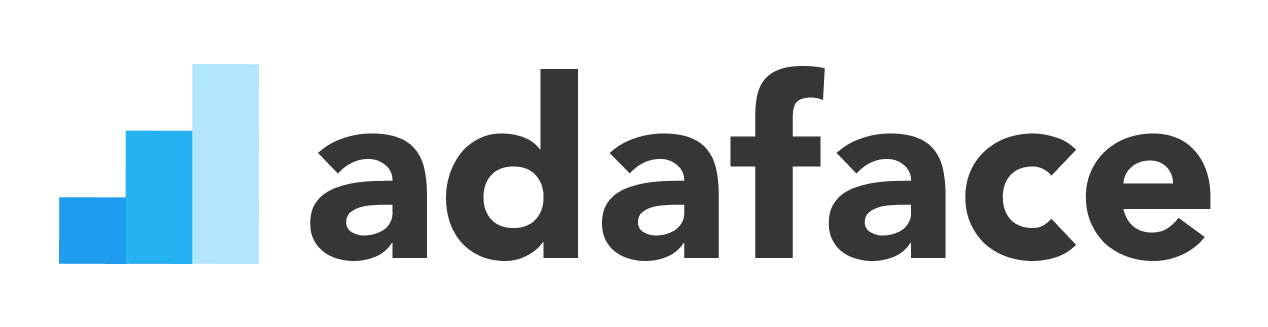
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
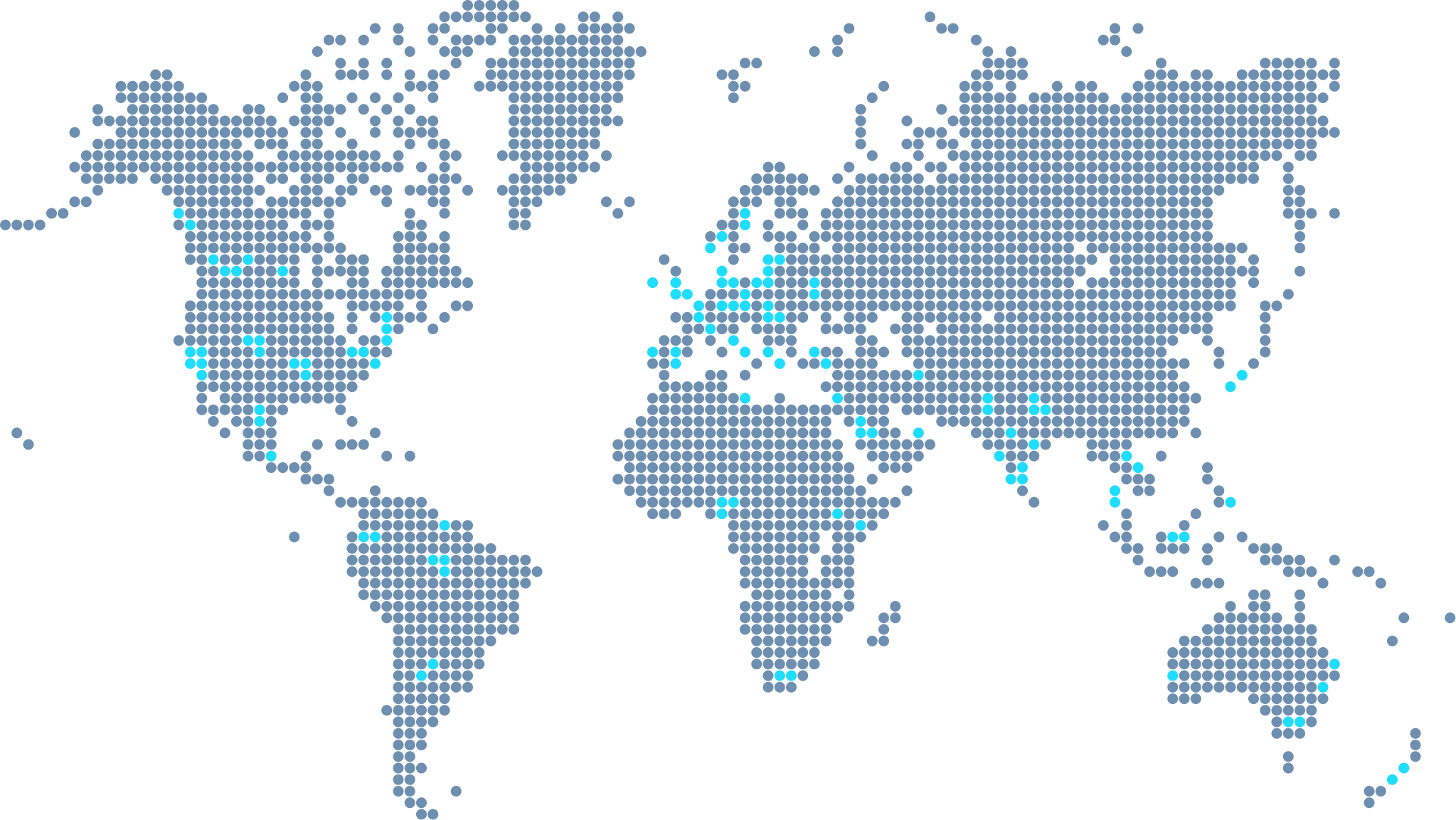
