Finding the right Laravel developer can be challenging, especially when you need someone who not only knows the framework but can also build scalable and maintainable applications. This curated list of interview questions will help you assess candidates effectively, ensuring you find someone who fits your team’s needs.
This blog post will cover a wide range of Laravel interview questions, from basic to expert levels, including multiple-choice questions (MCQs). We have structured the questions into five sections: Basic, Intermediate, Advanced, Expert, and MCQs, giving you a toolkit to assess candidates of varying skill levels.
By using these questions, you can streamline your interview process and identify top Laravel talent and before you interview, consider using a skills assessment test like our Laravel developer online test to filter candidates.
Table of contents
Basic Laravel interview questions
1. What is Laravel and why would you choose it over plain PHP?
Laravel is a PHP framework designed for building web applications following the Model-View-Controller (MVC) architectural pattern. It provides a robust set of tools and features that streamline development.
Choosing Laravel over plain PHP offers several advantages:
- Faster Development: Laravel offers pre-built components and features like routing, authentication, and templating, reducing the amount of code you need to write from scratch.
- Improved Security: Laravel provides built-in protection against common web vulnerabilities, such as cross-site scripting (XSS) and SQL injection.
- Maintainability: The MVC architecture promotes code organization and separation of concerns, making applications easier to maintain and scale. Code is more readable, testable and structured.
- Database Management: The eloquent ORM and schema builder simplifies database interactions and migrations.
php artisan migrate
automates DB changes. - Templating Engine: Blade templating engine provides simple ways to render dynamic data. For example:
@if(count($users) > 0) ... @endif
.
2. Explain the concept of 'artisan' in Laravel.
In Laravel, Artisan is the command-line interface (CLI) tool. It's based on Symfony's Console component and provides a number of helpful commands to assist in building and managing Laravel applications. Think of it as a swiss army knife for development tasks.
Artisan commands can perform tasks like creating controllers, models, migrations, seeding the database, clearing cache, managing routes, running tests, and even deploying your application. You can also create custom Artisan commands to automate repetitive tasks specific to your project. You run Artisan commands via the terminal using php artisan <command> [options] [arguments]
.
3. What are Laravel's key features?
Laravel boasts several key features that contribute to its popularity and developer-friendliness. These include a powerful routing system, an Eloquent ORM for database interaction, and the Blade templating engine for creating dynamic views. It also offers features like Artisan console for task automation, built-in authentication, and support for queues and scheduling.
Furthermore, Laravel emphasizes code organization and maintainability through its MVC architecture. It provides tools for testing, debugging, and security, making it a robust framework for building scalable web applications. It supports features like broadcasting and caching. Also, packages like Passport for API authentication and Sanctum for simple authentication are available. For example, defining a route in Laravel is very simple:
Route::get('/users', [UserController::class, 'index']);
4. Describe the purpose of a migration in Laravel.
In Laravel, migrations are like version control for your database schema. They allow you to modify and share the database schema definition for your application. Instead of manually creating tables or altering columns using SQL, you define these changes in migration files.
Migrations provide a structured and organized way to evolve your database over time. They are typically used to create tables, add or remove columns, create indexes, and perform other schema modifications. They ensure that all team members have the same database structure, making collaboration easier and preventing inconsistencies between environments (development, testing, production).
5. What is the role of a 'Model' in Laravel's MVC architecture?
In Laravel's MVC architecture, the Model represents the data structure and business logic of your application. It interacts with the database (or other data sources) to retrieve, store, update, and delete data. Essentially, the Model is responsible for managing the application's data.
More specifically, it typically defines:
- Database table: The table the model corresponds to.
- Relationships: How this model relates to other models.
- Attributes: Data fields representing columns in the database.
- Methods: Business logic related to the data, such as data validation or data transformation.
6. What is a 'Controller' in Laravel, and what does it do?
In Laravel, a Controller is a class that handles HTTP requests and orchestrates the application logic required to generate a response. It acts as an intermediary between the model (data layer) and the view (presentation layer), following the Model-View-Controller (MVC) architectural pattern.
Controllers contain methods (actions) that are executed when specific routes are matched. These actions typically retrieve data from the database (using Models), perform business logic, and then pass data to a View to be rendered. The controller then returns the rendered View (or other types of responses like JSON) back to the user. They help in organizing code and improving maintainability by separating concerns. Example:
class UserController extends Controller
{
public function show(int $id)
{
$user = User::find($id);
return view('user.profile', ['user' => $user]);
}
}
7. Explain the purpose of 'Routes' in a Laravel application.
In Laravel, routes define the endpoints of your application and dictate how the application responds to different HTTP requests (e.g., GET, POST, PUT, DELETE) made to specific URLs. They act as a central directory, mapping URLs to the appropriate controller methods or closures that will handle the request. Without routes, your application wouldn't know what code to execute when a user visits a particular URL.
Routes are typically defined in files like routes/web.php
(for web routes accessible via a browser) and routes/api.php
(for API routes). You can define a route using the Route
facade, specifying the HTTP verb, the URL, and the action to be taken:
Route::get('/users', [UserController::class, 'index']);
This example defines a GET route for the /users
URL, which will execute the index
method of the UserController
.
8. What are Laravel 'Views', and how are they used?
Laravel views are responsible for rendering the application's HTML. They separate the presentation logic from the application logic (controllers and models), promoting cleaner and more maintainable code. Views are typically simple HTML files with embedded PHP code, allowing dynamic content to be displayed. Views are usually stored in the resources/views
directory.
Views are used by controllers to display information to the user. A controller might fetch data from a database (using a Model) and then pass that data to a view. The view then uses this data to generate the HTML that is sent to the browser. The view()
helper function is used to load a view from within a controller action, for example:
return view('greeting', ['name' => 'Jessica']);
In the code above, the greeting
view (likely resources/views/greeting.blade.php
) is loaded, and the variable $name
is made available to the view with the value Jessica
.
9. Describe what 'Blade templating' is in Laravel.
Blade is Laravel's simple yet powerful templating engine. It allows you to use plain PHP within your views while also offering convenient shortcodes for common tasks like echoing data, looping, and conditional statements.
Instead of requiring you to use complex PHP code to generate HTML, Blade provides directives (like @if
, @foreach
, @extends
, @yield
, etc.) that are compiled into plain PHP code and cached until the view is modified. This compilation process speeds up rendering and makes views easier to read and maintain. Blade directives simplify common tasks and promote code reusability through features like template inheritance and sections.
10. How do you create a new Laravel project?
To create a new Laravel project, you can use Composer, the PHP dependency manager, or the Laravel installer.
- Using Composer: Open your terminal and run the command
composer create-project laravel/laravel your-project-name
. Replaceyour-project-name
with the desired name for your project. - Using the Laravel Installer: If you have the Laravel installer already, you can run
laravel new your-project-name
. If not, first install it globally usingcomposer global require laravel/installer
and ensure your system's PATH is correctly configured to execute thelaravel
command. Then you may runlaravel new your-project-name
.
11. What is Composer, and why is it important for Laravel?
Composer is a dependency management tool for PHP. It allows you to declare the libraries your project depends on, and it will manage (install and update) them for you. Think of it as npm
for Node.js or pip
for Python.
Composer is crucial for Laravel because Laravel relies heavily on third-party packages. Composer simplifies the process of including these packages in your project. Without Composer, manually downloading, managing, and autoloading these dependencies would be extremely cumbersome. It ensures that the correct versions of packages are installed and that dependencies between packages are resolved correctly, leading to a more stable and maintainable project. You can use it from the command line using composer install
or composer update
.
12. How do you configure a database connection in Laravel?
Database connections in Laravel are configured primarily through the .env
file and the config/database.php
file. The .env
file stores environment-specific settings like database host, port, database name, username, and password. These values are then accessed by the config/database.php
file.
To configure a database connection, you'll modify the .env
file with your database credentials:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name
DB_USERNAME=your_username
DB_PASSWORD=your_password
You can specify different database connections and their respective settings within the config/database.php
file. This file allows you to define multiple database connections and configure them based on the environment. The DB_CONNECTION
variable in the .env
file determines which connection Laravel will use by default.
13. Explain how to retrieve data from a database using Laravel.
Laravel provides several ways to retrieve data from a database. The most common methods involve using the Eloquent ORM or the Query Builder.
With Eloquent, you define a model representing a database table. You can then use methods like all()
, find()
, first()
, and get()
to retrieve records. For example, App\Models\User::all()
retrieves all users. The Query Builder offers a fluent interface for constructing SQL queries. You can use the DB
facade and chain methods like table()
, where()
, select()
, get()
, first()
etc. For example, DB::table('users')->where('id', 1)->first()
retrieves the first user with an ID of 1. Raw SQL queries can also be executed using DB::select('SELECT * FROM users WHERE id = ?', [1])
.
14. How do you pass data from a controller to a view?
There are several ways to pass data from a controller to a view, depending on the framework being used. A common method involves using a data structure like an array or object (often called a 'view model') to hold the data. This data is then passed as an argument to the view when it's rendered.
For example, in many MVC frameworks, you can pass an associative array to the view. Keys in the array become variables accessible within the view's template. Some frameworks also use dedicated 'view data' or 'view bag' properties to achieve this, providing a structured way to organize and pass data. return view('my_view', ['name' => $name, 'items' => $items]);
is a typical example.
15. What is the purpose of the `.env` file in Laravel?
The .env
file in Laravel stores environment-specific configuration settings. These settings are things that vary between different environments (development, testing, production), such as database credentials, API keys, debug mode, and application URL.
It allows you to keep sensitive information and environment-specific configurations out of your codebase, improving security and making it easier to manage different environments. Laravel uses the Dotenv
PHP library to load these variables into the $_ENV
and $_SERVER
superglobals, which you can then access using the env()
helper function.
16. How do you handle form submissions in Laravel?
In Laravel, form submissions are typically handled through routes, controllers, and request objects. First, a route is defined (often a POST
route) that corresponds to the form's action attribute. This route points to a controller method.
In the controller method, you can access the form data using Laravel's request object (Illuminate\Http\Request
). Laravel provides several ways to validate the incoming data, such as using request validation rules defined directly in the controller or by creating a separate form request class. After validation, you can process the data, typically storing it in the database using Eloquent ORM. Finally, you usually redirect the user to another page (e.g., a success page) or back to the form with a success/error message using Laravel's session flash messages.
17. Explain how to use Laravel's built-in authentication features.
Laravel provides a streamlined way to implement authentication using its built-in features. The quickest method is using the php artisan ui
command. For example, php artisan ui bootstrap --auth
scaffolds basic authentication views, routes, and controllers for login, registration, and password reset using Bootstrap. Alternatively, you can install Vue or React scaffolding too. After running the command, you'll need to run npm install && npm run dev
to compile the assets.
Behind the scenes, Laravel's authentication guard (configured in config/auth.php
) handles user authentication. The Auth
facade provides methods like Auth::attempt()
, Auth::check()
, and Auth::user()
to manage user sessions. Middleware such as auth
is used to protect routes, ensuring only authenticated users can access them. You can further customize the authentication process by modifying the generated controllers and views to suit your specific application needs, such as adding extra fields to the registration form or implementing different authentication methods.
18. What are 'seeders' in Laravel, and when would you use them?
Seeders in Laravel are classes that populate your database with initial data. They are typically used to pre-populate a database with default or testing data when you first set up your application, or when running tests.
You would use seeders in scenarios such as:
- Setting up initial user accounts (e.g., an administrator account).
- Populating lookup tables with predefined values (e.g., categories, statuses).
- Creating sample data for demonstration purposes.
- Creating data for running automated tests.
To run a seeder, you would use the following artisan command:
php artisan db:seed --class=YourSeederClassName
19. Describe how you would implement basic input validation in Laravel.
Laravel provides a powerful and convenient way to implement input validation using validation rules. You can define these rules within your controller using the Validator
facade or by leveraging form request classes. Here's a basic example using the Validator
facade:
use Illuminate\Support\Facades\Validator;
$validator = Validator::make($request->all(), [
'name' => 'required|string|max:255',
'email' => 'required|email|unique:users',
'password' => 'required|min:8|confirmed',
]);
if ($validator->fails()) {
return redirect('post/create')
->withErrors($validator)
->withInput();
}
In this example, the Validator::make()
method takes the request data and an array of validation rules. If the validation fails ($validator->fails()
), it redirects back to the form with the error messages. Laravel's built-in validation rules cover common scenarios like required fields, email format, unique values, and password confirmation. Alternatively, Form Request classes can be used to encapsulate validation logic into a separate class, improving code organization and reusability. To use Form Request, you can use php artisan make:request StoreUserRequest
. In the rules
method, you can define the same rules as the example above.
20. What is the purpose of middleware in Laravel?
Middleware in Laravel provides a convenient mechanism for filtering HTTP requests entering your application. Think of it as a series of checkpoints a request must pass through before reaching your application's core logic (like controllers). Its primary purpose is to inspect and potentially modify the request or response.
Common uses include authentication (verifying if a user is logged in), logging (recording request details), CSRF protection (preventing cross-site request forgery), and modifying request headers. Middleware allows you to encapsulate these cross-cutting concerns in reusable classes, keeping your controllers clean and focused on handling business logic.
21. How do you define and use a custom middleware in Laravel?
In Laravel, custom middleware can be defined using the php artisan make:middleware <MiddlewareName>
command. This creates a new middleware class in the app/Http/Middleware
directory. Within this class, you define the handle
method, which receives the incoming request and a Closure
representing the next middleware in the pipeline.
To use the middleware, you must register it in either the $middleware
array in app/Http/Kernel.php
(global middleware) or the $routeMiddleware
array, assigning it a key. The key then can be applied to specific routes or route groups using the middleware()
method, e.g., Route::get('/profile', [UserController::class, 'show'])->middleware('auth');
. Here auth
is the key corresponding to the Authenticate
middleware that comes by default in laravel.
22. Explain the concept of dependency injection in Laravel.
Dependency injection (DI) in Laravel is a design pattern that allows you to remove hard-coded dependencies from your classes. Instead of a class creating its dependencies, these dependencies are "injected" into the class, usually through the constructor, setter methods, or sometimes even method parameters. This makes your code more modular, testable, and reusable.
Laravel's service container is a powerful tool for implementing DI. It automatically resolves dependencies by inspecting the class's constructor and injecting the required dependencies. This simplifies the process of managing dependencies and reduces boilerplate code. Example: public function __construct(UserRepositoryInterface $userRepository) { $this->userRepository = $userRepository; }
Laravel's container will resolve the UserRepositoryInterface
to its concrete implementation.
23. What are Service Providers in Laravel, and what do they do?
Service providers are the central point of bootstrapping a Laravel application. They are responsible for binding services into the service container, making them available for use throughout the application. Essentially, they're classes that configure your application with the things it needs, like database connections, mail configurations, and custom components.
Service providers typically define what services are available and how they should be instantiated. They have a register
method, where you bind things into Laravel's service container. A boot
method can also be used if you need to do anything after the services are registered, such as attaching a view composer or registering event listeners. For example, inside a service provider's register
method:
$this->app->singleton(MyService::class, function ($app) {
return new MyService($app->make(Dependency::class));
});
24. Describe how to use Laravel's Eloquent ORM to interact with a database.
Eloquent ORM in Laravel provides a simple ActiveRecord implementation for database interactions. Models are defined as classes extending Illuminate\Database\Eloquent\Model
. To retrieve data, you can use methods like all()
, find($id)
, where()
(with constraints), and first()
. For example:
$users = App\Models\User::all(); // Get all users
$user = App\Models\User::find(1); // Get user with ID 1
$admins = App\Models\User::where('role', 'admin')->get(); // Get all admins
To create, update, or delete records, you instantiate a model, set attributes, and then use save()
to persist the changes. For example:
$user = new App\Models\User;
$user->name = 'John Doe';
$user->email = 'john.doe@example.com';
$user->password = bcrypt('secret');
$user->save(); // Create a new user
$user = App\Models\User::find(1);
$user->email = 'new.email@example.com';
$user->save(); // Update user with ID 1
$user = App\Models\User::find(1);
$user->delete(); // Delete user with ID 1
Eloquent also supports relationships between models, enabling easy access to related data. Example: $user->posts
(assuming a hasMany
relationship defined in the User
model).
25. How do you define relationships between Eloquent models?
Eloquent provides several ways to define relationships between models, mirroring common database relationships. These relationships are defined as methods on your Eloquent model classes.
Common relationship types include:
- One To One:
hasOne
,belongsTo
- One To Many:
hasMany
- Many To Many:
belongsToMany
- Has One Through:
hasOneThrough
- Has Many Through:
hasManyThrough
- Polymorphic Relationships:
morphTo
,morphOne
,morphMany
,morphedByMany
For example, to define a one-to-many relationship between a User
model and a Post
model where a user can have many posts, you would define a posts()
method on the User
model like this:
public function posts()
{
return $this->hasMany(Post::class);
}
26. Explain how to use Laravel's built-in pagination.
Laravel's built-in pagination simplifies displaying large datasets in manageable chunks. To use it, first, in your controller, use the paginate()
method on your query builder or Eloquent model, specifying the number of items per page. For example: $users = User::paginate(15);
. This returns a LengthAwarePaginator
instance.
Then, in your Blade view, display the paginated data as you would normally with a foreach
loop. Finally, render the pagination links using {{ $users->links() }}
. This will generate HTML links for navigating between pages. You can customize the appearance of these links by publishing and modifying the pagination views. The paginator automatically detects the current page from the query string and applies appropriate 'active' classes. You can also use the simplePaginate()
method if you only need "Previous" and "Next" links for better performance.
27. Describe the purpose and benefits of using Laravel Mix.
Laravel Mix provides a fluent API for defining Webpack build steps for your Laravel applications. Its purpose is to simplify the often complex process of asset compilation, such as bundling JavaScript, compiling CSS with preprocessors like Sass or Less, and optimizing images.
The benefits include simplified configuration, a cleaner and more readable build process, and a unified way to manage assets across your project. It abstracts away much of the underlying Webpack configuration, allowing developers to focus on writing code rather than configuring build tools. mix.js('src/app.js', 'public/js')
, mix.sass('src/app.scss', 'public/css')
are some example usages.
Intermediate Laravel interview questions
1. Explain the concept of Service Containers in Laravel and how they facilitate dependency injection.
Laravel's service container is a powerful tool for managing class dependencies and performing dependency injection. It's essentially a central repository for binding interfaces to concrete implementations. When a class requires a dependency, the container can automatically resolve and inject that dependency, promoting loose coupling and testability.
It facilitates dependency injection through binding and resolving. You register (bind) interfaces or classes to the container, specifying how they should be instantiated. When a class hints at an interface or class as a dependency in its constructor, the container automatically resolves (injects) the appropriate instance based on the bindings. For example:
// Binding an interface to an implementation
$this->app->bind(MyInterface::class, MyImplementation::class);
// Resolving the dependency in a class constructor
public function __construct(MyInterface $myDependency) {
$this->myDependency = $myDependency;
}
2. Describe how you would implement a custom Artisan command that seeds data into the database based on a specific environment.
First, create a new Artisan command using php artisan make:command SeedEnvData
. Within the command's handle()
method, use App::environment()
to check the current environment. Based on the environment, call different seeders or run specific logic. For instance:
public function handle()
{
if (App::environment('local')) {
$this->call('db:seed', ['--class' => 'LocalSeeder']);
} elseif (App::environment('staging')) {
$this->call('db:seed', ['--class' => 'StagingSeeder']);
} else {
$this->info('No seeding for this environment.');
}
}
Remember to define your LocalSeeder
and StagingSeeder
classes. Finally, register the command in app/Console/Kernel.php
in the $commands
array, and you can then execute it with php artisan your:command
.
3. How would you go about optimizing database query performance in a Laravel application, considering eager loading and query caching?
To optimize database query performance in a Laravel application, I'd focus on eager loading and query caching. Eager loading prevents the N+1 problem by loading related models in a single query instead of multiple queries within a loop. Use with()
when querying your models to eagerly load relationships. For example, Model::with('relation')->get();
. This retrieves both the models and their related data efficiently. Query caching stores the results of expensive queries to avoid re-executing them repeatedly. You can use Laravel's Cache
facade, like so: Cache::remember('query_key', $ttl, function () { return DB::table('table')->get(); });
where $ttl
is the time-to-live in minutes. This significantly reduces database load for frequently accessed data.
4. What are the differences between `findOrFail()` and `firstOrFail()` methods in Eloquent, and when would you use each?
Both findOrFail()
and firstOrFail()
are Eloquent methods used to retrieve data from a database, but they differ in what they expect as a result and how they handle the case when no data is found.
findOrFail()
: This method is typically used with a primary key or unique identifier. It expects to find a single model instance. If a model matching the specified key isn't found, it throws aModelNotFoundException
. You'd use this when you're looking for a specific model by its ID. For example:$user = User::findOrFail(1);
firstOrFail()
: This method is used when you are applying conditions (e.g., usingwhere()
) and expect only one record to match those conditions. It returns the first model instance that matches the query's criteria. If no models match the criteria, it throws aModelNotFoundException
. Example:$product = Product::where('name', 'Example Product')->firstOrFail();
5. Explain the purpose and usage of Laravel's event system, and provide an example of how you might use events and listeners.
Laravel's event system provides a simple observer pattern implementation, allowing you to subscribe to and listen for events in your application. The purpose is to decouple components, enabling you to execute code when specific actions occur without directly modifying the code that triggers those actions. This improves maintainability and extensibility. For example, you might use events to send a welcome email when a new user registers. The registration process fires a UserRegistered
event, and a listener, SendWelcomeEmail
, handles the event and sends the email. This keeps the registration logic clean and focused on user creation, while the email sending is handled separately.
Usage involves defining events and listeners. Events are classes that represent something that happened in your application. Listeners are classes that handle the events when they are dispatched. Laravel's event service provider handles registration automatically. To define an event and listener, you can use artisan commands like php artisan make:event UserRegistered
and php artisan make:listener SendWelcomeEmail
. Here's an example of how you might dispatch an event:
event(new UserRegistered($user));
And in the listener, you'd handle the event like this:
public function handle(UserRegistered $event)
{
// Send welcome email to $event->user
}
6. How would you implement a middleware to check if a user has a specific permission before accessing a route?
To implement a middleware for permission checks, you'd typically: 1. Retrieve the user's roles or permissions (e.g., from a database or session). 2. Define a function that accepts the required permission as an argument. 3. Inside the function, check if the user has the specified permission. 4. If the user has the permission, call next()
to proceed to the next middleware or route handler. 5. If the user lacks the permission, return an appropriate error response (e.g., 403 Forbidden).
Here's a simplified example using JavaScript and Express.js:
const checkPermission = (permission) => {
return (req, res, next) => {
const userPermissions = req.user.permissions; // Assuming req.user is populated
if (userPermissions && userPermissions.includes(permission)) {
return next();
} else {
return res.status(403).json({ message: 'Forbidden' });
}
};
};
// Usage in a route:
app.get('/admin', checkPermission('admin.access'), (req, res) => {
res.send('Admin area');
});
7. Describe the process of creating and using custom validation rules in Laravel, including how to handle error messages.
In Laravel, you can create custom validation rules using the Validator
facade. First, define a new rule class implementing the Illuminate\Contracts\Validation\Rule
interface. This requires you to define two methods: passes($attribute, $value)
which contains the validation logic, and message()
which returns the error message when the validation fails. You register the custom rule using Validator::extend()
in a service provider's boot
method. Within your validator extend closure you would instantiate your new validation class. For example Validator::extend('custom_rule', CustomRule::class.'@passes', CustomRule::class.'@message');
To use the custom rule, simply apply it in your validation rules array like any other built-in rule, such as 'field' => 'required|custom_rule'
. To customize the error message, you can pass a third argument to Validator::extend()
or define the message()
method in your rule class to return a dynamic error message (e.g., based on the validated value). Error messages are typically stored in resources/lang/en/validation.php
or in your language files.
8. Explain how you would use Laravel's queue system to handle long-running tasks, and the benefits of doing so.
Laravel's queue system allows offloading time-consuming tasks to be processed in the background, improving application responsiveness. I would use it by first configuring a queue connection (e.g., database, redis, sqs). Then, I'd dispatch jobs to the queue using Queue::push()
or dispatch()
. These jobs contain the code to execute the long-running task. A queue worker (using php artisan queue:work
) would then process these jobs asynchronously.
The benefits include:
- Improved user experience: The user doesn't have to wait for the task to complete.
- Scalability: Background processing can be scaled independently.
- Resilience: Failed jobs can be automatically retried.
- Reduced server load: Offloading tasks prevents overloading the web server.
9. What are the different types of relationships in Eloquent (one-to-one, one-to-many, many-to-many), and how do you define them?
Eloquent provides several types of relationships to help you manage how your database tables are related. These include one-to-one, one-to-many, and many-to-many. A one-to-one relationship connects one model to exactly one other model. For example, a User
model might have one Profile
model. You define this in the User
model using hasOne()
and in the Profile
model using belongsTo()
. A one-to-many relationship means one model can have multiple related models. For instance, a Post
model can have many Comment
models. This is defined using hasMany()
in the Post
model and belongsTo()
in the Comment
model.
A many-to-many relationship occurs when multiple models on both sides can be related to each other. Consider User
and Role
models, where a user can have multiple roles, and a role can be assigned to multiple users. A pivot table is typically used to manage the relationship. You define this using belongsToMany()
on both the User
and Role
models. Here's an example of defining a many-to-many relationship:
// User model
public function roles()
{
return $this->belongsToMany(Role::class);
}
// Role model
public function users()
{
return $this->belongsToMany(User::class);
}
10. Describe how you would implement API authentication using Laravel Passport.
To implement API authentication using Laravel Passport, I would first install the package via Composer: composer require laravel/passport
. Then, I'd run the Passport install command: php artisan passport:install
, which generates the encryption keys needed for secure token generation and sets up the necessary database migrations. Next, I would apply the migrations using php artisan migrate
.
After setup, I would configure the App\Models\User
model to use the Laravel\Passport\HasApiTokens
trait. Then, I'd define API routes and protect them using the auth:api
middleware. To issue access tokens, I'd typically use grant types like password grant (for trusted clients) or authorization code grant (for third-party applications), implementing the necessary logic in controllers to handle token requests and return the tokens to the client. Finally, on the client-side, I would store the access token securely and include it in the Authorization
header (as a Bearer
token) for every API request.
11. Explain the purpose of Laravel's service providers and how they are used to bootstrap application services.
Laravel service providers are central to bootstrapping a Laravel application. Their primary purpose is to register services within the application's service container, making them available for dependency injection. This includes things like database connections, mailers, view composers, and custom application components.
Service providers have two crucial methods: register()
and boot()
. The register()
method binds services to the container. The boot()
method is called after all service providers have been registered, allowing for any setup that depends on other services, such as registering routes, event listeners, or middleware. They help maintain a modular and organized codebase by encapsulating service registration logic.
12. How would you implement a feature that allows users to upload files to a specific storage location, including validation and security considerations?
To implement a file upload feature, I would start with a secure backend API endpoint. This endpoint would handle file reception, validation, and storage. Important validations include checking file size, type (using MIME types), and potentially scanning for malware. I'd use a library or service to handle the file type validation, ensuring that malicious files disguised with incorrect extensions are rejected. For storage, I'd opt for object storage services like AWS S3 or Azure Blob Storage for scalability and security. The backend would generate a unique, unpredictable filename to prevent overwrites and potential URL guessing attacks. Access control would be implemented at the storage level, ensuring that uploaded files are only accessible to authorized users or processes.
13. Explain the difference between the `get()` and `value()` methods when retrieving configuration values in Laravel.
In Laravel, both get()
and value()
are used to retrieve configuration values, but they behave differently when the configuration value is a Closure (anonymous function).
get()
will return the configuration value as is. If the value is a Closure, get()
will return the Closure itself (the function object) without executing it. value()
however, will execute the Closure if the configuration value is a Closure, returning the result of the Closure's execution. If the value is not a Closure, value()
will return the value directly, similar to get()
. In essence, value()
ensures that you always get the resolved value, whereas get()
gives you the raw value from the configuration, be it a simple string or a callable function. config('setting.key')
is equivalent to config()->get('setting.key')
, and config()->value('setting.key')
if the value is supposed to be resolved.
14. Describe how you would handle exceptions and errors in a Laravel application, including logging and custom error pages.
Laravel provides a robust error handling mechanism. For exceptions, I'd typically use the try-catch
block to gracefully handle them. Caught exceptions can be logged using Laravel's built-in logging facade: Log::error('My error message', ['context' => $exception]);
. Laravel automatically logs unhandled exceptions via the exception handler defined in app/Exceptions/Handler.php
. I'd customize the render
method in this handler to return custom error pages based on the exception type or HTTP status code. For instance, returning a 404.blade.php
view for NotFoundHttpException
.
15. How would you implement a search functionality in a Laravel application, considering performance and relevance?
To implement search functionality in Laravel with performance and relevance in mind, I'd use a combination of database indexing, full-text search capabilities, and potentially a dedicated search engine like Algolia or Elasticsearch. For simple searches on indexed columns, Laravel's Eloquent ORM with where
clauses and pagination would suffice. For more complex searches involving multiple columns and relevance ranking, I'd leverage MySQL's MATCH AGAINST
for full-text search after creating a FULLTEXT index on the relevant table columns or use PostgreSQL's full text search capabilities. Consider using database query optimization techniques, like EXPLAIN
to analyze query plans, and caching frequently accessed search results.
For high-performance and advanced relevance ranking, integrating a dedicated search engine like Algolia or Elasticsearch is ideal. These tools offer features like typo tolerance, stemming, synonyms, and faceted search. Libraries like Scout can simplify the integration with Laravel. Finally, it's important to implement proper pagination and limit the number of results returned to avoid performance bottlenecks. Regularly monitor query performance and optimize indexes as needed.
16. Explain the purpose and usage of route model binding in Laravel.
Route model binding in Laravel automatically injects model instances directly into your route closures or controller methods based on the route parameters. Instead of manually fetching a model using find()
or findOrFail()
within your controller, Laravel handles this for you.
To use it, you typically type-hint the model in your route closure or controller method and ensure the route parameter name matches the model's primary key column (usually id
). For example, Route::get('/users/{user}', function (App\Models\User $user) { return view('user.profile', ['user' => $user]); });
Here, $user
will automatically contain the User
model instance corresponding to the id
in the route. If no matching model is found, Laravel will automatically return a 404 HTTP response.
17. Describe how you would implement a multi-language (localization) feature in a Laravel application.
Laravel's localization features make it straightforward. First, create language files within the resources/lang
directory, organized by language code (e.g., en
, es
, fr
). Each file returns an array of key-value pairs representing the translations. Then, use the __()
helper function (or @lang
directive in Blade templates) to retrieve the appropriate translation based on the current locale. For instance, {{ __('messages.welcome') }}
will output the value associated with the welcome
key in the messages.php
file for the currently set language.
To manage the current locale, use App::setLocale($locale)
. You can detect the user's preferred language from their browser settings or allow them to select it through a UI element. Persist the selected locale using session storage or cookies so it's remembered across requests. Middleware can be used to automatically set the locale based on stored preference or user input parameters.
18. How do you use factories and seeders to create database records for testing or development purposes?
Factories define how to create a model instance with realistic, but potentially randomized, data. Seeders use factories to populate the database with multiple records, typically for initial setup, testing, or demo purposes. In Laravel, for example, you'd define a factory using $factory->define(Model::class, function (Faker $faker) { return [...]; });
specifying attributes and using Faker to generate data. Then, a seeder might look like factory(Model::class, 50)->create();
, which creates 50 instances of that model using the defined factory.
Seeders are called via the php artisan db:seed
command. You can target specific seeders or create a 'DatabaseSeeder' to run them sequentially. This provides a repeatable and controlled way to initialize your database. This is extremely useful for ensuring consistent data across different environments, or for quickly setting up a testing database with representative information.
19. Explain the concept of route groups and their benefits.
Route groups are a mechanism to organize and apply common configurations to a set of routes in web frameworks like Laravel, Express.js, or Flask. They allow you to group routes that share similar attributes, such as middleware, namespaces, prefixes, or rate limits. The primary benefit is reducing code duplication and improving maintainability. Instead of defining the same configurations for each route individually, you define them once for the group.
Benefits include:
- Reduced Code Duplication: Avoid repeating the same configurations for multiple routes.
- Improved Readability: Makes route definitions cleaner and easier to understand.
- Enhanced Maintainability: Changes to common configurations only need to be made in one place.
- Simplified Security: Easily apply middleware for authentication or authorization to a group of routes.
For example, in Laravel:
Route::middleware(['auth'])->prefix('admin')->group(function () {
Route::get('/users', [UserController::class, 'index']);
Route::post('/users', [UserController::class, 'store']);
});
This example applies the auth
middleware and the admin
prefix to both /admin/users
routes.
20. Describe how you would implement a role-based access control (RBAC) system in a Laravel application.
To implement RBAC in Laravel, I'd leverage a package like spatie/laravel-permission
. First, I'd install the package via composer: composer require spatie/laravel-permission
. Then, I would publish the migrations and configuration files using php artisan vendor:publish --provider="Spatie\Permission\PermissionServiceProvider"
. This creates tables for roles, permissions, and their relationships to users. I would define roles such as 'admin', 'editor', and 'viewer', and permissions like 'create-posts', 'edit-posts', and 'view-posts'.
Next, I would assign roles and permissions to users using the provided methods like $user->assignRole('admin')
and $role->givePermissionTo('create-posts')
. In my application's controllers and routes, I'd use middleware like role:admin
or permission:create-posts
to protect specific functionalities. Additionally, blade directives such as @role('admin')
could control view rendering based on the user's role. This provides a flexible and manageable RBAC system.
21. How can you prevent SQL injection vulnerabilities in your Laravel application when using raw queries?
To prevent SQL injection vulnerabilities in Laravel when using raw queries, you should always use parameter binding. Parameter binding ensures that user-supplied data is treated as data, not as part of the SQL query itself. This prevents malicious users from injecting arbitrary SQL code into your queries.
Instead of directly embedding user input into your raw SQL queries, use the ?
placeholder and pass an array of values as the second argument to the DB::raw()
or DB::statement()
methods. Laravel will then properly escape and quote these values, making SQL injection attempts ineffective. For example:
$userId = request('user_id');
$results = DB::select('SELECT * FROM users WHERE id = ?', [$userId]);
22. Explain how you would implement a real-time chat feature using Laravel and WebSockets.
To implement a real-time chat feature with Laravel and WebSockets, I'd use Laravel's broadcasting feature along with a WebSocket server like Pusher or Socket.IO. First, I would configure Laravel's broadcasting settings to use Pusher or Socket.IO. Then, when a new message is sent, a Laravel event would be dispatched. This event would be broadcasted using the configured broadcasting driver. On the client-side (e.g., using JavaScript in the browser), I would subscribe to the appropriate channel using a Pusher or Socket.IO client. When a new message event is received, the client-side code would update the chat interface in real-time.
For example, a new message event called NewChatMessage
would be created. This event would implement the ShouldBroadcast
interface. The broadcastOn
method would return the name of the channel to broadcast on (e.g., a public channel called chat
). Then, the front end uses a library like pusher-js
and subscribe to the chat
channel and listens for the NewChatMessage
event. Here's an example of broadcasting the event:
event(new NewChatMessage($message));
23. Describe the process of creating and using custom Eloquent accessors and mutators.
Eloquent accessors and mutators allow you to format attribute values when retrieving (accessors) or setting (mutators) them. To create an accessor, define a method in your model named get{AttributeName}Attribute
. This method will automatically be called when you access the {attribute_name}
property on your model. It should return the modified value. To create a mutator, define a method named set{AttributeName}Attribute
. This method will be called when you set the {attribute_name}
property on your model. It receives the value being set as an argument. Inside the mutator, you can modify the value and then assign it to the model's underlying attribute ( $this->attributes['attribute_name']
) or perform other actions.
For example:
// Accessor
public function getFirstNameAttribute($value)
{
return ucfirst($value);
}
// Mutator
public function setFirstNameAttribute($value)
{
$this->attributes['first_name'] = strtolower($value);
}
With these defined, $model->first_name
will return the capitalized first name, and setting $model->first_name = 'JOHN'
will store 'john' in the database. No direct interaction with the accessor/mutator methods are required, Laravel automatically calls them when the appropriate attribute is accessed or mutated.
24. How would you implement a scheduled task (cron job) in Laravel?
Laravel provides a convenient way to implement scheduled tasks using the task scheduler. First, you define your scheduled tasks within the schedule
method of the app/Console/Kernel.php
file. You can use methods like call
, command
, or exec
to define what should be executed, and then chain methods like hourly
, daily
, weekly
, or use cron expressions via cron('* * * * *')
to specify the schedule.
Second, you need to add a single cron entry to your server that runs the Laravel scheduler every minute. This entry executes the schedule:run
Artisan command, which in turn checks the defined schedules and executes the tasks that are due. The cron entry would look something like this: * * * * * php /path/to/your/project/artisan schedule:run >> /dev/null 2>&1
Advanced Laravel interview questions
1. Explain Laravel's service container and how it facilitates dependency injection. Provide a practical example of its usage.
Laravel's service container is a powerful tool for managing class dependencies and performing dependency injection. It's essentially a central repository for resolving and injecting dependencies throughout your application. It allows you to bind interfaces to concrete implementations, making your code more modular, testable, and maintainable.
For example, consider an interface PaymentGatewayInterface
and two implementations, StripeGateway
and PayPalGateway
. Using the service container, you can bind the interface to a specific implementation in a service provider:
$this->app->bind(PaymentGatewayInterface::class, StripeGateway::class);
Then, anywhere you need a PaymentGatewayInterface
, Laravel will automatically inject an instance of StripeGateway
. In a controller:
public function __construct(PaymentGatewayInterface $paymentGateway)
{
$this->paymentGateway = $paymentGateway;
}
The $paymentGateway
property will automatically be an instance of StripeGateway
.
2. Describe the differences between Queues and Events in Laravel. When would you use one over the other?
Queues in Laravel provide a way to defer the processing of time-consuming tasks, like sending emails or processing large datasets. They allow your application to respond quickly to web requests while handling these tasks in the background. Events, on the other hand, are a way to broadcast that something has happened in your application. Listeners then react to these events and can perform actions based on them.
Use Queues when you need to process tasks asynchronously to improve response time. Use Events when you want to decouple different parts of your application, allowing multiple listeners to respond to a single action.
3. How can you optimize database queries in Laravel applications? Discuss techniques like eager loading, query caching, and indexing.
Optimizing database queries in Laravel involves several techniques. Eager loading prevents the N+1 query problem by loading related models in a single query using with()
. For example, $books = Book::with('author')->get();
retrieves all books and their authors efficiently. Query caching stores the results of frequently executed queries in the cache, reducing database load. You can use Cache::remember('users', 60, function () { return DB::table('users')->get(); });
. Finally, indexing adds indexes to database columns that are frequently used in WHERE
clauses to speed up data retrieval. Use migrations to define indexes, like $table->index('email');
.
4. Explain how Laravel's middleware works. Create a scenario where you'd implement custom middleware.
Laravel middleware provides a convenient mechanism for filtering HTTP requests entering your application. Think of them as a series of 'gatekeepers' that inspect and optionally modify the request before it reaches your application's core logic (like a controller). They can perform tasks such as authentication, logging, input validation, or even modifying response headers.
A common scenario for custom middleware is to check if a user's IP address is on a 'blacklist' of blocked IPs. The middleware would intercept the request, check the user's IP against a database or configuration file containing blacklisted IPs. If the IP is found on the blacklist, the middleware could redirect the user to an error page or return a 403 Forbidden response. Otherwise, the request is allowed to proceed to the intended route. An example code snippet inside the middleware handle method is shown below.
public function handle($request, Closure $next)
{
$ip = $request->ip();
if (in_array($ip, config('app.blacklist_ips'))){
abort(403, 'Your IP is blocked.');
}
return $next($request);
}
5. Describe the process of creating and using custom Artisan commands in Laravel. Give an example use case.
To create a custom Artisan command in Laravel, you use the make:command
Artisan command. For example, php artisan make:command SendEmails
will generate a new command class in the app/Console/Commands
directory. You then need to define the $signature
and $description
properties, and implement the handle()
method, which contains the logic to be executed when the command is run. Finally, register the command in the app/Console/Kernel.php
file within the $commands
array. To use the command, run php artisan your-command-name
in the terminal.
Example Use Case: Imagine you need to regularly clean up old log files. You could create an Artisan command named cleanup:logs
that handles this task. The command's handle()
method would contain the logic to delete files older than a certain date. This command can then be scheduled to run daily using Laravel's task scheduler.
6. How does Laravel's encryption work? What methods are available for securing sensitive data?
Laravel provides a powerful encryption system built on top of OpenSSL using AES-256-CBC. You can encrypt data using the encrypt
helper function or the Crypt
facade. The decryption is correspondingly done using decrypt
. Configuration is managed in config/app.php
, specifically the key
and cipher
settings. To secure sensitive data, Laravel offers:
- Encryption: Using the
encrypt
anddecrypt
methods or theCrypt
facade for encrypting/decrypting data in transit or at rest. Important when storing sensitive data in the database. Example:Crypt::encryptString('secret value')
- Hashing: Using
Hash::make
andHash::check
for one-way hashing of passwords. Bcrypt is the default algorithm. Always hash passwords instead of storing them in plain text.Hash::make('password')
- Token Generation: Utilizing
Str::random()
for generating secure API tokens or remember tokens. These tokens can be stored alongside user data. Example:Str::random(60)
- Environment Variables: Storing sensitive credentials (API keys, database passwords) in
.env
files and accessing them using theenv()
helper function instead of hardcoding them directly in the code. Make sure that .env file is not committed to git. - Rate Limiting: Protecting routes from brute-force attacks using middleware to limit the number of requests per IP address.
- Sanitization and Validation: Sanitizing user input to prevent XSS and SQL injection vulnerabilities and using Laravel's built-in validation features to ensure data integrity.
7. Explain the purpose and usage of Laravel's Policies for authorization. Provide a basic policy example.
Laravel Policies are used to encapsulate authorization logic for a particular model or resource. Instead of cluttering controllers with authorization checks, policies centralize this logic into dedicated classes. This promotes cleaner, more maintainable code by separating authorization concerns from business logic.
For example, a PostPolicy
might define methods like view
, update
, and delete
to determine if a user can perform these actions on a Post
model. Here's a basic example:
namespace App\Policies;
use App\Models\User;
use App\Models\Post;
use Illuminate\Auth\Access\HandlesAuthorization;
class PostPolicy
{
use HandlesAuthorization;
public function update(User $user, Post $post)
{
return $user->id === $post->user_id;
}
}
This policy's update
method checks if the user attempting to update the post is the owner of the post. You would then register this policy in your AuthServiceProvider
and use it in your controllers or views using methods like $this->authorize('update', $post);
.
8. Describe the different types of relationships available in Laravel Eloquent ORM. How do you use them effectively?
Eloquent ORM in Laravel provides several types of relationships to define how different models are related to each other. These include: One To One, One To Many, Many To Many, Has One Through, Has Many Through, One To Many (Polymorphic), Many To Many (Polymorphic).
To use them effectively, you define these relationships as methods on your Eloquent models. For example, in a User
model, you might define a hasOne
relationship to a Profile
model like this: public function profile() { return $this->hasOne(Profile::class); }
. Similarly, a hasMany
relationship to a Post
model would be: public function posts() { return $this->hasMany(Post::class); }
. Many-to-many relationships use belongsToMany
, requiring a pivot table. Polymorphic relationships are used when a model can belong to multiple types of models on a single association.
9. How do you implement API authentication in Laravel? Discuss different approaches like Sanctum, Passport, or JWT.
Laravel offers several options for API authentication. Sanctum is a lightweight package perfect for SPA and mobile applications authenticating with your Laravel backend. It uses API tokens and session cookies. To implement it, install the package via composer, publish the config, and then protect routes using the auth:sanctum
middleware.
Passport, on the other hand, provides a full OAuth2 server implementation. It's more complex but suitable for applications needing to offer API access to third-party developers. After installing it, you must run migrations, generate encryption keys, and configure your AuthServiceProvider
. JWT (JSON Web Tokens), often implemented using packages like tymon/jwt-auth
, offers a stateless approach. The client receives a token upon successful authentication and sends it with every subsequent request. The server verifies the token's signature to authenticate the user. Choose the method that best fits the complexity and security needs of your application.
10. Explain Laravel's broadcasting feature. How does it work, and what real-time functionalities does it enable?
Laravel's broadcasting feature allows you to emit server-side Laravel events to your client-side JavaScript application over a WebSocket connection. At a high level, when a Laravel event is triggered, it's broadcasted to a specific channel (e.g., a chat room). Clients subscribed to that channel receive the event data in real-time.
Here's how it works:
A Laravel event implements the
ShouldBroadcast
interface.The event specifies a broadcast channel (using channels like
public
,private
, orpresence
).Laravel dispatches the event data to a configured broadcasting driver (e.g., Pusher, Ably, Redis).
The broadcasting driver pushes the data to a WebSocket server.
The client-side JavaScript application, using a WebSocket client (like
laravel-echo
), subscribes to the channel and receives the event data. This enables real-time functionalities like live chat, real-time notifications, activity streams, collaborative editing, and live data dashboards.php artisan make:event
helps quickly set up events. Example setup:// Event class MessageSent implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct(Message $message) { $this->message = $message; } public function broadcastOn() { return new PrivateChannel('chat.' . $this->message->chat_id); } public function broadcastWith() { return ['message' => $this->message]; } }
11. What are Laravel's Service Providers and how are they used to bootstrap application components?
Laravel Service Providers are central to bootstrapping a Laravel application. They act as the entry point for registering application components and dependencies into the service container. Think of them as the place where you 'register' things your application needs to run, such as event listeners, routes, middleware, and even core service classes. They promote modularity, reusability, and maintainability by organizing application logic into discrete units.
Service providers typically have two key methods:
register()
: Binds services and classes into the service container, making them available for dependency injection.boot()
: Used to execute code after all service providers have been registered. This is where you might register routes, event listeners, or publish configuration files. Common use cases:- Registering custom validation rules.
- Registering view composers.
- Registering event listeners.
- Defining routes.
12. Describe the process of implementing localization in a Laravel application to support multiple languages.
Laravel localization allows you to support multiple languages in your application. First, you need to create language files within the resources/lang
directory. Each language has its own subdirectory (e.g., en
, es
, fr
). Within each subdirectory, create PHP files containing an array of key-value pairs, where the key is the identifier and the value is the translated string. For example:
// resources/lang/en/messages.php
return [
'welcome' => 'Welcome to our application!',
'goodbye' => 'Goodbye!',
];
// resources/lang/es/messages.php
return [
'welcome' => '¡Bienvenido a nuestra aplicación!',
'goodbye' => '¡Adiós!',
];
Then, you can use the trans()
helper function (or the @lang
Blade directive) in your views or code to retrieve the translated strings based on the current locale. To set the application's locale, use the App::setLocale()
method. This can be done based on user preferences, browser settings, or any other criteria. For instance: App::setLocale('es');
to set the locale to Spanish. You can then retrieve translations using trans('messages.welcome')
which will return '¡Bienvenido a nuestra aplicación!' if the locale is set to 'es'.
13. How can you implement custom validation rules in Laravel? Give an example of a complex validation scenario.
Laravel provides several ways to implement custom validation rules. You can use Closure-based rules, Rule objects, or extend the Validator class. For simple rules, Closure-based rules are quick and easy. For more complex or reusable rules, Rule objects are preferred. To create a Rule object, you can use the php artisan make:rule
command.
Consider a scenario where you need to validate that a user's provided age is appropriate based on their selected country. For some countries, the minimum age might be 16, while for others, it's 18. Here's how you could implement this with a Rule object:
// app/Rules/AgeBasedOnCountry.php
namespace App\Rules;
use Illuminate\Contracts\Validation\Rule;
class AgeBasedOnCountry implements Rule
{
protected $country;
public function __construct($country)
{
$this->country = $country;
}
public function passes($attribute, $value)
{
if ($this->country == 'USA') {
return $value >= 21; // USA minimum age
} elseif ($this->country == 'Canada') {
return $value >= 19; // Canada minimum age
} else {
return $value >= 18; // Default minimum age
}
}
public function message()
{
return 'The age is not valid for the selected country.';
}
}
// In your controller
$request->validate([
'country' => 'required|string',
'age' => ['required', 'integer', new AgeBasedOnCountry($request->country)],
]);
14. Explain how to handle file uploads in Laravel, including validation, storage, and retrieval.
To handle file uploads in Laravel, you typically use the store
or storeAs
methods on an uploaded file instance obtained from the request. First, access the file using $request->file('your_file_input_name')
. For validation, you can use Laravel's validation rules within your request validation, such as file
, required
, mimes:jpeg,png
, and max:2048
(kilobytes). To store the file, use $request->file('your_file_input_name')->store('uploads')
which stores the file in the storage/app/uploads
directory with an auto-generated name. Alternatively, storeAs('uploads', 'filename.jpg')
lets you specify the filename. Configuration for the filesystem (e.g., local, s3) resides in config/filesystems.php
.
Retrieval involves creating a symbolic link from public/storage
to storage/app/public
using the php artisan storage:link
command if you're storing files in the public
disk. After that, you can access the file using asset('storage/uploads/filename.jpg')
in your Blade templates. If storing the files on s3, use the Storage::url('uploads/filename.jpg')
helper to generate the public URL.
15. Describe the different methods for testing Laravel applications (unit, feature, integration). Give examples of each.
Laravel provides several methods for testing applications: unit, feature, and integration tests. Unit tests focus on testing individual units of code, like methods, in isolation. For example, testing that a sum
function correctly adds two numbers would be a unit test. This usually involves mocking dependencies. A simple example:
use PHPUnit\Framework\TestCase;
class ExampleTest extends TestCase
{
public function testBasicTest()
{
$result = 1 + 1;
$this->assertEquals(2, $result);
}
}
Feature tests (previously known as 'functional tests') test a larger portion of the application, often involving multiple components interacting. They simulate user requests and verify the application's response. For example, testing that a user can register via a form and is redirected to the dashboard is a feature test. Feature tests interact with your actual routes, controllers, and views. An example might be:
public function testUserCanRegister()
{
$response = $this->post('/register', [
'name' => 'John Doe',
'email' => 'john@example.com',
'password' => 'password',
'password_confirmation' => 'password',
]);
$response->assertRedirect('/dashboard');
$this->assertAuthenticated();
}
Integration tests verify that different parts of the application work together correctly. They sit between unit and feature tests in terms of scope. For example, testing that the database properly saves the user data after registration (verifying correct model behavior interacting with the database) is an integration test. Unlike unit tests, mocks are generally avoided in integration tests, instead using a test database for realistic interactions.
16. How can you use Laravel's events and listeners to decouple application components and handle side effects?
Laravel's events and listeners provide a powerful mechanism for decoupling application components. An event signifies that something has occurred in the application, and listeners react to those events. Instead of directly implementing side effects within the code that triggers the event, you can define listeners to handle these actions. This isolates the core logic from secondary concerns.
For example, instead of sending a welcome email directly after user registration, you can fire a UserRegistered
event. A SendWelcomeEmail
listener can then be configured to listen for that event and handle the email sending process. This approach enhances maintainability, testability, and scalability by preventing tight coupling between different parts of the application. Here's how events and listeners work together:
- Events: Act as triggers.
- Listeners: React to specific events and execute code.
- Decoupling: Events don't know about listeners, and listeners don't directly influence events.
- The
Event::dispatch()
method is used to fire events. Listeners use thehandle()
method to define how to react to events.
17. Explain the process of deploying a Laravel application to a production environment. What are some key considerations?
Deploying a Laravel application involves several steps: First, prepare the application for production by setting APP_ENV=production
in your .env
file and running php artisan config:cache
and php artisan route:cache
to optimize performance. Then, upload your application files to the server, excluding development-related files like .env
and node_modules
. Install dependencies using composer install --no-dev
. Configure your web server (Nginx or Apache) to point to the public
directory as the document root.
Key considerations include database configuration (ensuring you're using the production database), setting up proper file permissions for storage and bootstrap/cache directories, configuring queue workers (using php artisan queue:work
), and implementing a robust deployment strategy like zero-downtime deployment. Additionally, use a process manager like Supervisor to keep queue workers running, and ensure you have a mechanism for handling errors and logging. Configure SSL (HTTPS) for security.
18. How do you handle exceptions and errors in Laravel? Discuss custom error pages and logging.
Laravel provides robust exception handling through its exception handler. By default, Laravel logs exceptions using a Monolog-based logger, configured in config/logging.php
. You can customize exception handling by modifying the app/Exceptions/Handler.php
file. The render
method allows you to customize the response returned for a given exception type. For example, you can return a custom error view for specific exceptions. report
method for logging.
Custom error pages can be created by placing Blade templates in the resources/views/errors
directory. Laravel will automatically render these views based on the HTTP status code of the error (e.g., 404.blade.php
, 500.blade.php
). You can also define custom error pages for specific exception types in the render
method of the exception handler. Laravel's logging can be configured to use various channels (e.g., single file, daily files, syslog) and log levels (e.g., debug, info, error) as needed. Log::channel('slack')->error('Something Happened');
is an example of using a custom logging channel.
19. Describe how you can use Laravel's task scheduling feature to automate recurring tasks.
Laravel's task scheduling allows you to define commands that run on a schedule. You define these schedules within the app/Console/Kernel.php
file, specifically in the schedule
method. Inside this method, you use the $schedule
object to define what command to run and how often using methods like daily()
, hourly()
, weekly()
, cron()
and many others. For example:
$schedule->command('emails:send')->dailyAt('08:00');
This line schedules the emails:send
artisan command to run every day at 8:00 AM. To make it work, you need to add a single cron entry to your server that calls the Laravel scheduler every minute:
* * * * * php /path-to-your-project/artisan schedule:run >> /dev/null 2>&1
20. How can you use Laravel's Telescope to debug and monitor your application's performance?
Laravel Telescope provides a dashboard to gain insights into your application's requests, exceptions, database queries, logs, and more. To debug, first ensure Telescope is installed and configured. Then, access the Telescope dashboard via its route (usually /telescope
).
You can then monitor various aspects. For example:
- Requests: Inspect request details, including headers, payloads, and response data to identify slow endpoints or unexpected inputs.
- Queries: Analyze database queries, including execution time and SQL, to find performance bottlenecks or inefficient queries. Use Telescope's query analysis to identify N+1 issues.
- Exceptions: Review exceptions and stack traces to understand and fix errors quickly. Telescope shows the exact point of failure.
- Logs: Examine application logs for debugging information or unusual behavior. Telescope provides a centralized view of your logs.
- Cache: Monitor cache hits and misses to optimize cache usage.
- Redis: Inspect Redis commands to identify performance issues related to Redis.
Telescope allows filtering and searching within each category to quickly find specific issues or patterns, enabling efficient debugging and performance monitoring.
Expert Laravel interview questions
1. How would you optimize a Laravel application dealing with millions of database records to ensure quick response times?
Optimizing a Laravel application with millions of database records involves several strategies. Firstly, focus on database optimization:
- Indexing: Ensure appropriate indexes are in place for frequently queried columns. Use tools like
php artisan optimize:index
to analyze and suggest missing indexes. - Query optimization: Use
EXPLAIN
to analyze slow queries and rewrite them for better performance. AvoidSELECT *
, and only retrieve necessary columns. Consider usingchunk()
orlazy()
for processing large datasets. - Caching: Implement caching at various levels: database query caching, route caching, and view caching.
- Database connection pooling: Use connection pooling to reduce the overhead of establishing new connections for each request.
- Consider using read replicas to distribute read load.
Secondly, optimize the application code:
- Eager loading: Prevent N+1 query problems by using eager loading (
with()
) to retrieve related data in a single query. - Queueing: Offload long-running tasks (e.g., sending emails, processing data) to queues for background processing.
- Code profiling: Use tools like Laravel Telescope or Xdebug to identify performance bottlenecks in your code.
- Optimize auto-loading: Run
composer dump-autoload --optimize
to optimize the autoloader.
Finally, server optimization is also very important. Ensure sufficient memory, CPU, and use opcode caching like OPCache. Consider a CDN to serve static assets.
2. Describe your approach to building a highly scalable API using Laravel, considering rate limiting, versioning, and security.
To build a highly scalable API with Laravel, I'd prioritize several key aspects. First, rate limiting can be implemented using Laravel's built-in throttle middleware or packages like thomasjohnkane/laravel-throttle
. This prevents abuse and protects resources. Versioning is crucial, employing URI versioning (e.g., /api/v1/resource
) or header-based versioning. Laravel's middleware can route requests to the appropriate controller version. Security involves authentication (using Laravel Passport or Sanctum for API tokens, or JWTs), authorization (using policies), and input validation to prevent common vulnerabilities. Always use HTTPS and configure CORS properly. Consider using tools like PHPStan
and Psalm
for static analysis to catch potential issues early.
For scalability, optimize database queries using eager loading and caching (Redis or Memcached). Queue asynchronous tasks to offload heavy processing. Load balancing across multiple servers and a CDN for static assets significantly improves performance. Furthermore, monitor API performance using tools like New Relic or DataDog to identify bottlenecks and areas for improvement. Code should be stateless where applicable.
3. Explain how you would implement a custom authentication guard in Laravel, detailing the steps and considerations involved.
To implement a custom authentication guard in Laravel, you'd start by creating a custom guard class that implements the Illuminate\Contracts\Auth\Guard
and Illuminate\Contracts\Auth\UserProvider
interfaces. The Guard
handles authentication logic (e.g., attempt
, user
, validate
) and the UserProvider
retrieves user data from your data source. You would then register the custom guard in the config/auth.php
file, within the guards
array, specifying the driver (pointing to your custom guard) and the provider (specifying which UserProvider to use).
Key considerations include securely handling user credentials, implementing robust session management or token-based authentication as appropriate, and ensuring your UserProvider
is correctly configured to interact with your user data store. For example the provider might use Eloquent or a custom database query. Here's a snippet showing how to define it in config/auth.php
:
'guards' => [
'custom' => [
'driver' => 'custom_driver',
'provider' => 'users',
],
],
'providers' => [
'users' => [
'driver' => 'eloquent',
'model' => App\Models\User::class,
],
],
4. How would you design and implement a complex queuing system in Laravel, capable of handling different types of jobs with varying priorities and retry strategies?
To design a complex queuing system in Laravel, I'd leverage Laravel's built-in queue system and customize it. First, define different queue connections in config/queue.php
for different job types (e.g., 'high_priority', 'low_priority'). Each connection can use different drivers (database, Redis, SQS, etc.). Then, implement custom job classes for each type of task. These classes should implement the ShouldQueue
interface. Implement specific retry strategies by overriding the retryAfter()
and tries
properties or methods in the job class. For priority, push jobs to different queues based on priority levels. Use queue workers dedicated to specific queues to process jobs based on their assigned priority.
For more advanced control, consider using a package like supervisord
or Laravel's Horizon to manage queue workers. Implement rate limiting if needed to prevent overwhelming resources. Utilize middleware to tag the job to track the progress. Use the Bus::dispatch()
method to dispatch jobs and optionally chain them together. Finally, monitor queue health and performance using tools like Laravel Telescope or custom monitoring dashboards.
5. Discuss your experience with integrating Laravel with different front-end frameworks like React, Vue.js, or Angular, and how you managed the API interactions and state management.
I've worked with Laravel as a backend API provider for React and Vue.js frontends. My approach generally involves creating RESTful APIs using Laravel's resource controllers. For API interactions, I typically use axios
in React and Vue, handling HTTP requests (GET, POST, PUT, DELETE) and managing responses. On the Laravel side, I use Eloquent ORM to interact with the database and return data in JSON format using Laravel's built-in response helpers (response()->json()
).
For state management, in React, I've used Context API for simpler applications and Redux or Zustand for more complex state requirements. In Vue.js, I've used Vuex. I ensure the frontend framework holds the application state fetched from the API, and mutations to the state trigger updates in the UI. I have also used Laravel Sanctum for API authentication, securing the API endpoints and managing user sessions using tokens.
6. Describe your strategy for handling transactions across multiple database connections in Laravel to ensure data consistency.
To ensure data consistency across multiple database connections in Laravel transactions, I would utilize Laravel's DB::transaction()
method, but specify the connection for each database operation. For instance, I would define connections in config/database.php
such as mysql_one
and mysql_two
. I would then use DB::connection('mysql_one')->transaction(function () { ... });
and DB::connection('mysql_two')->transaction(function () { ... });
where necessary. However, transactions are typically connection-specific.
For truly distributed transactions across different database systems (e.g., MySQL and PostgreSQL), a more advanced approach using two-phase commit (2PC) might be required via libraries such as Jenssegers/Laravel-Transactions
. This helps coordinate commits across multiple connections, ensuring either all operations succeed or all are rolled back. If 2PC is not feasible, implementing compensating transactions (manually undoing changes if one transaction fails) is another possibility, although more complex to manage.
7. How would you implement a robust role-based access control (RBAC) system in Laravel, allowing fine-grained control over user permissions and resources?
To implement RBAC in Laravel, I'd leverage packages like spatie/laravel-permission
. First, I'd define roles (e.g., 'admin', 'editor') and permissions (e.g., 'create-post', 'edit-post'). Then, I'd assign permissions to roles and roles to users. The spatie/laravel-permission
package provides handy methods and traits for this, such as assignRole
, hasPermissionTo
, and the @can
Blade directive. For fine-grained control, resource-based permissions are crucial. This means defining permissions not just on actions but also on specific resources. For instance, an 'editor' role might have 'edit' permission only on posts they created. Policy classes are key here; Laravel policies allow you to specify authorization logic for each model (resource) by checking against the current user and any relevant data. For instance:
//In PostPolicy.php
public function update(User $user, Post $post)
{
return $user->hasRole('admin') || ($user->id === $post->user_id && $user->hasPermissionTo('edit-post'));
}
Finally, middleware can be used to protect routes based on roles or permissions, adding an extra layer of security.
8. Explain how you would approach testing a complex Laravel application, including unit, integration, and end-to-end tests, and the tools you would use.
When testing a complex Laravel application, I'd start with unit tests to isolate and verify individual components like models, services, and controllers. PHPUnit is my go-to tool for this. For example:
class ExampleTest extends TestCase
{
public function test_example(): void
{
$this->assertTrue(true);
}
}
Next, integration tests ensure that different parts of the application work together correctly. This might involve testing interactions between controllers and models, or how events are handled. The built-in Laravel testing helpers would be used. Finally, end-to-end (E2E) tests simulate real user scenarios, validating the entire application flow. I'd use tools like Laravel Dusk or Cypress for E2E tests. Mocking external services would be crucial in both integration and E2E tests for isolation and speed.
9. How do you handle different environments such as development, staging, and production in a Laravel project and ensure consistency across them?
I typically use Laravel's .env
files in conjunction with configuration caching to manage environment-specific settings. Each environment (development, staging, production) has its own .env
file that defines variables like database credentials, API keys, and debugging settings. These .env
variables are then accessed in Laravel's configuration files (config/*
) using the env()
helper function. This allows you to easily change settings without modifying the core codebase. I would use tools like laravel-env-sync to keep environments in sync.
To ensure consistency, I leverage configuration caching (php artisan config:cache
). This caches all configuration values into a single file for faster loading in production. It's crucial to run php artisan config:clear
whenever you modify .env
variables or configuration files to prevent stale values. I also use database seeders and migrations to manage database schema and initial data, ensuring that the database structure is consistent across all environments. These are essential parts of my CI/CD pipeline.
10. Describe your experience with debugging and profiling Laravel applications to identify and resolve performance bottlenecks.
I have experience using Laravel's built-in debugging tools like debugbar and clockwork
to inspect queries, routes, views, and request/response data. These tools help me quickly identify slow queries, redundant operations, or inefficient data loading (N+1 problem). I've also utilized Laravel's logging capabilities and tools like Telescope for more in-depth monitoring and analysis of application performance in production environments.
For resolving performance bottlenecks, I've employed techniques like query optimization (using indexes, eager loading), caching (using Redis or Memcached for frequently accessed data), code profiling with tools like Xdebug and Blackfire, and optimizing image/asset delivery through CDNs. I also focus on refactoring code for efficiency, minimizing database interactions, and implementing queue systems for long-running tasks to improve overall application responsiveness.
11. How would you implement a feature flag system in Laravel to enable or disable features dynamically without deploying new code?
A feature flag system in Laravel can be implemented using a database table to store flags and their states (enabled/disabled). A configuration file can also be used, but a database allows for runtime changes. A middleware or a simple helper function checks the flag status before allowing access to a feature. For example, a middleware could read the flag value from the database or cache using a key like feature.new_feature_x.enabled
. If the flag is enabled, the request proceeds; otherwise, a redirect or error message is shown.
To make this dynamic, an admin panel or API endpoint can be created to toggle the feature flags in the database. When a flag is changed, the cache (if used) should be cleared to reflect the new state immediately. The helper function or middleware will then use the updated values. Using a package like Laravel Feature Flags
simplifies the process.
12. Explain how you would approach building a multi-tenant application using Laravel, considering data isolation and shared resources.
To build a multi-tenant application with Laravel, I'd focus on database isolation and resource sharing. For database isolation, I'd choose either separate databases or a shared database with tenant identifiers. Separate databases offer strong isolation but increase management overhead. A shared database uses a tenant_id
column in each table to isolate data. This approach requires careful query scoping using global scopes or middleware.
For shared resources like code and assets, I'd leverage Laravel's features. Route-model binding can automatically filter queries based on the current tenant. Service providers and middleware can handle tenant context detection (e.g., from subdomain, URL path, or custom header) and apply the appropriate database connection or query scope. Caching strategies would need to consider tenant-specific keys to avoid data leaks. Finally, I would implement proper authentication and authorization to prevent cross-tenant access, using Laravel's built-in features with modifications to account for tenancy.
13. How would you secure a Laravel application against common web vulnerabilities like SQL injection, XSS, and CSRF attacks?
To secure a Laravel application, use Eloquent ORM with parameterized queries to prevent SQL injection. Always escape output data using {{ }}
Blade syntax (which defaults to escaping) or the e()
helper function to mitigate XSS. Laravel provides built-in CSRF protection; enable it by including the @csrf
directive in your forms and using the VerifyCsrfToken
middleware. Also, utilize features like input validation, proper authentication and authorization, and keep your dependencies updated.
14. Describe your experience with using Laravel's event system to decouple components and improve the maintainability of a large application.
In a large application, I used Laravel's event system to decouple several components, specifically around user registration and order processing. For example, after a user registered, instead of directly triggering welcome emails and analytics updates within the registration controller, I dispatched a UserRegistered
event. Listeners then handled tasks like sending emails and updating analytics. This significantly reduced dependencies and made the registration controller much cleaner. Similarly, for order processing, events like OrderCreated
, OrderShipped
, and OrderDelivered
were used to trigger various actions such as updating inventory, sending notifications, and generating reports. Each listener could focus on its specific task without needing to know about other parts of the system.
This approach greatly improved maintainability. When we needed to add a new action after an order was shipped (e.g., sending a feedback request), we simply created a new listener and registered it with the OrderShipped
event, without modifying the core order processing logic. This loose coupling made it much easier to add new features and modify existing ones with minimal risk of introducing regressions. Also, testing become simpler. Individual listeners could be easily tested in isolation. I often used queued listeners for tasks that didn't require immediate execution, further improving performance and resilience.
15. How do you implement and manage API versioning in a Laravel application to ensure backward compatibility?
API versioning in Laravel can be implemented through various strategies. A common approach is using URI versioning (e.g., api/v1/resource
, api/v2/resource
). Middleware can then be used to route requests based on the version specified in the URI or a custom request header. Controllers are namespaced according to their version (e.g., App\Http\Controllers\Api\V1\ResourceController
). This allows maintaining multiple versions of the same resource concurrently. For example:
Route::prefix('api/v1')->group(function () {
Route::get('/resource', 'App\Http\Controllers\Api\V1\ResourceController@index');
});
Route::prefix('api/v2')->group(function () {
Route::get('/resource', 'App\Http\Controllers\Api\V2\ResourceController@index');
});
Backward compatibility can be ensured by:
- Maintaining older versions: Continue supporting older API versions for a defined period.
- Deprecation warnings: Include deprecation warnings in responses from older versions, informing clients about the upcoming removal.
- Transformers: Use transformers to manage data output consistently across versions, adapting older data structures for newer API formats.
- Versioning Routes: Route groups are used to organize routes based on versions. This allows you to maintain distinct route definitions for each API version. It avoids disrupting existing clients reliant on older API versions by preventing any abrupt changes to the API's structure or behavior.
16. Explain your approach to handling file uploads and storage in Laravel, considering different storage providers and security implications.
In Laravel, I typically use the built-in filesystem abstraction for handling file uploads and storage. I configure the config/filesystems.php
file to define different storage disks like local
, s3
, or public
. For uploads, I use the Storage
facade, which provides methods like putFile
, putFileAs
, and disk()
to interact with these storage disks. For example: Storage::disk('s3')->putFile('uploads', $request->file('photo'));
.
Security is paramount. I validate file uploads rigorously using Laravel's validation rules, checking MIME types, file sizes, and extensions. For public storage, I generate unique filenames to prevent unauthorized access and directory listing. When working with sensitive data, I prefer using signed URLs for temporary access or encrypting the files before storing them. Also, ensuring that the storage directories are not publicly accessible by configuring web server rules is crucial.
17. How would you implement a real-time notification system in Laravel using WebSockets or server-sent events (SSE)?
To implement a real-time notification system in Laravel, I'd leverage WebSockets or SSE. Using WebSockets (e.g., with Laravel Echo and Pusher/Ably/Redis), I'd create an event that's broadcast when a notification occurs. A WebSocket listener on the client-side, set up via Laravel Echo, would then receive this event and display the notification to the user. Alternatively, with SSE, the server pushes updates to the client. A Laravel controller would handle the event, format the notification data, and send it as an SSE stream. On the client-side, an EventSource
object would listen for these streams and update the UI accordingly.
Key steps would involve:
- Choosing a broadcasting driver: (Pusher, Ably, Redis, etc.)
- Defining an event:
php artisan make:event NotificationSent
- Broadcasting the event:
broadcast(new NotificationSent($notification));
- Setting up Laravel Echo: configure in
bootstrap.js
. - Listening for the event:
Echo.channel('notifications').listen('NotificationSent', (e) => { /* update UI */ });
- For SSE, configure your web server to handle SSE correctly, ensure the Content-Type header is set to
text/event-stream
, and useyield
in the controller to send chunks of data.
18. Describe your experience with contributing to open-source Laravel packages or creating your own, and the challenges you faced.
I've contributed to a few open-source Laravel packages, primarily focusing on documentation improvements and bug fixes. For example, I submitted a pull request to correct a typo in the documentation of the laravel-debugbar
package. Also, I reported an issue regarding localization in spatie/laravel-medialibrary
and offered a potential solution, which was helpful for other contributors. While the scope was limited, it provided valuable experience in understanding contribution workflows.
I also developed a small Laravel package for simplifying API versioning, although it hasn't reached widespread adoption. The main challenge was maintaining momentum and dedicating time to properly document and test the package while balancing other commitments. Additionally, ensuring the package adhered to best practices and kept up with Laravel updates required continuous learning. Another challenge was writing comprehensive tests. I relied heavily on PHPUnit and Mockery, aiming for high test coverage but also striving for tests that truly reflected the package's intended behavior.
19. How would you implement a search functionality in Laravel that can handle large datasets and provide relevant results quickly?
For large datasets, using Laravel's Eloquent directly can be slow. I'd leverage a dedicated search engine like Elasticsearch or Algolia. First, I'd configure a scout driver for either of these. Then, I'd index the relevant model data to the search engine, which provides optimized text-based search and filtering. When a user performs a search, the Laravel application would query the search engine via scout, get the relevant IDs and hydrate the models for presentation, and display them efficiently.
Alternatively, for simpler needs or where external services aren't desired, consider database optimizations. Using fulltext indexes on relevant columns in MySQL or PostgreSQL could significantly improve search performance. Then, utilize raw queries for search and employ pagination with eager loading of relationships to keep the response times fast.
20. Explain how you would handle localization (i18n) and internationalization (l10n) in a Laravel application to support multiple languages and regions.
Laravel provides robust features for localization and internationalization. To support multiple languages, I would first create language files for each supported locale in the resources/lang
directory. These files contain key-value pairs of translated strings. For example, resources/lang/en/messages.php
and resources/lang/fr/messages.php
. Laravel uses a 'locale' configuration to determine the current language. This can be set in the config/app.php
file or dynamically using App::setLocale($locale)
.
To display translated text in views, I would use the @lang('messages.welcome')
Blade directive or the __('messages.welcome')
helper function. Additionally, I would configure middleware to detect the user's preferred language based on headers or session variables and set the application locale accordingly. For date and number formatting, Laravel's Intl
extension can be used along with Carbon
for date manipulation, ensuring culturally appropriate display. For more complex projects, consider using packages like mcamara/laravel-localization
for URL-based locale detection and management.
21. How do you monitor the performance and health of a live Laravel application and respond to issues proactively?
To monitor a live Laravel application, I'd use a combination of tools and techniques. For performance monitoring, I'd leverage tools like Laravel Telescope (for local debugging and insight), New Relic, or Blackfire.io to identify slow queries, bottlenecks in the code, and memory usage issues. For server health, I'd use tools like Prometheus with Grafana or simple monitoring scripts that check CPU usage, disk space, and memory consumption. Laravel's built-in logging capabilities, along with a centralized logging system like ELK stack (Elasticsearch, Logstash, Kibana) or Sentry, help track errors and exceptions.
Proactive issue response involves setting up alerts based on predefined thresholds for metrics like response time, error rates, and server resource utilization. These alerts can be configured within the monitoring tools mentioned above, sending notifications via email, Slack, or other channels. Furthermore, I'd implement automated health checks within the application to detect and potentially resolve issues before they impact users. Scheduled tasks can run diagnostics and self-healing processes. Regularly reviewing logs and monitoring dashboards is also crucial for identifying potential problems early on.
22. Explain how you would implement two-factor authentication (2FA) in a Laravel application.
Implementing 2FA in Laravel typically involves these steps: First, install a 2FA package like laravel-google-authenticator
. Next, add a two_factor_secret
column to your users table to store the secret key. When a user enables 2FA, generate a unique secret key using the package, store it securely (encrypted) in the database, and display a QR code or the secret key itself for the user to set up in their authenticator app (like Google Authenticator or Authy).
During login, after the user provides their username/password, check if 2FA is enabled for their account. If so, prompt them for the verification code from their authenticator app. Validate this code against the stored secret using the package's verification methods. If the code is valid, grant access; otherwise, deny login. You might also want to provide a 'remember me' functionality that leverages browser cookies to bypass 2FA on trusted devices.
23. How would you design a system for handling scheduled tasks in Laravel, considering concurrency and error handling?
In Laravel, I'd use the built-in scheduler along with Redis for managing scheduled tasks, focusing on concurrency and error handling. For concurrency, I'd leverage Redis-based locks to ensure only one instance of a task runs at a time, preventing race conditions. Specifically, I'd use Cache::lock('task_name', $seconds)
before executing the task logic and release it afterward.
For error handling, each scheduled task would be wrapped in a try-catch
block. Errors would be logged using Laravel's logging facade for debugging. Additionally, I'd configure the scheduler to send email notifications on task failure using Laravel's built-in mail functionality. For more complex scenarios, I might consider using a dedicated queue system like Horizon to offload scheduled tasks to background workers, further improving reliability and performance.
24. Describe your experience with using Laravel's collection pipeline to perform complex data transformations.
I've used Laravel's collection pipeline extensively for complex data transformations. I appreciate how it allows me to chain methods for data manipulation in a readable and fluent way, replacing verbose loops with more concise code. For example, I've used it to filter data based on multiple criteria, group related items, map values to new formats, and then sort the results, all within a single collection pipeline.
Specifically, I've used methods like map
, filter
, groupBy
, sortBy
, and reduce
to perform complex operations. Consider a scenario where I needed to transform a list of user objects to include only their names and email addresses, sorted by name, and grouped by the first letter of their last name. This could be achieved with a clean and manageable collection pipeline rather than nested loops and conditional statements. collect($users)->map(function ($user) { return ['name' => $user->name, 'email' => $user->email]; })->sortBy('name')->groupBy(function ($user) { return substr($user['name'], 0, 1); });
25. How do you ensure the quality of your Laravel code through code reviews, static analysis, and automated testing?
I ensure Laravel code quality through a combination of code reviews, static analysis, and automated testing.
For code reviews, I focus on code clarity, adherence to coding standards (PSR-12), proper use of Laravel features, security vulnerabilities, and performance bottlenecks. We use pull requests and GitLab/GitHub's built-in review tools. For static analysis, I use tools like PHPStan and Psalm to detect potential errors, type inconsistencies, and dead code. These are integrated into our CI/CD pipeline. Automated testing is crucial; I write unit tests for individual components and features, feature tests for user interactions, and integration tests to ensure different parts of the application work together correctly. We use PHPUnit and Pest. Code coverage is monitored to ensure a sufficient percentage of the code is tested. These automated tests are run on every commit to prevent regressions.
Example using PHPUnit:
public function test_example(): void
{
$response = $this->get('/');
$response->assertStatus(200);
}
26. Explain how you would integrate a third-party payment gateway (e.g., Stripe, PayPal) into a Laravel application.
Integrating a third-party payment gateway like Stripe or PayPal in Laravel typically involves these steps: First, choose a suitable PHP package (e.g., Stripe's official PHP library or Omnipay for a more generic approach). Install the package via Composer: composer require stripe/stripe-php
(for Stripe). Next, configure the package with your API keys (obtained from the payment gateway's dashboard) in your .env
file and create a config file to access them. Then, implement the payment flow in your Laravel application. This usually includes creating forms to collect payment information, using the payment gateway's API to process the payment (e.g., creating a charge or customer), and handling success and error responses. Finally, store transaction details in your database and update order status accordingly.
27. How would you optimize the loading speed of a Laravel application, focusing on both front-end and back-end techniques?
To optimize a Laravel application's loading speed, several front-end and back-end techniques can be employed. On the front-end, minify CSS, JavaScript, and HTML files to reduce their size. Implement browser caching to store static assets locally. Optimize images by compressing them and using appropriate formats (e.g., WebP). Use a Content Delivery Network (CDN) to distribute assets globally. Utilize lazy loading for images and other content below the fold.
On the back-end, optimize database queries by using indexes and eager loading relationships (with()
in Eloquent). Implement caching strategies using Laravel's caching system (e.g., Redis or Memcached) to store frequently accessed data. Use a performant web server like Nginx or Apache with appropriate configurations. Ensure you are using the latest stable version of PHP and have enabled opcode caching (e.g., OPcache). Profile your application to identify bottlenecks using tools like Laravel Telescope or Xdebug, and optimize slow-running code. Consider using queues to offload time-consuming tasks like sending emails or processing large datasets.
Laravel MCQ
In Laravel Eloquent, what relationship should you define on the Country
model to access all of its Post
models, assuming each post belongs to exactly one country, and the posts
table has a country_id
column?
Which of the following represents the most secure way to prevent mass assignment vulnerabilities in a Laravel model?
Which of the following statements best describes the primary purpose of Model Factories in Laravel?
In Laravel, what is the primary purpose of binding a class or interface to the service container?
options:
In Laravel, what is the primary difference between a Gate and a Policy?
In the Laravel request lifecycle, which of the following components is responsible for filtering HTTP requests entering your application?
What is the primary role of Service Providers in a Laravel application?
Options:
In Laravel, what is the primary difference between using the app()
and resolve()
helper functions to retrieve a service from the service container?
options:
Which of the following statements best describes the primary purpose of Laravel Queues?
In Laravel, middleware is executed in the order it is defined. Where should you typically register global middleware to ensure it's applied to every request?
What is the primary benefit of using implicit route model binding in Laravel?
In Laravel's event system, what is the primary purpose of an event listener?
Which statement best describes the primary purpose of Form Request validation in Laravel?
options:
In Laravel, what is the primary purpose of Facades?
Which of the following best describes the primary purpose of Laravel's Dependency Injection Container?
Which Blade directive in Laravel is used to check if the user is authenticated and display content accordingly?
Which of the following methods is the most secure and recommended way to store session data in a production Laravel application?
options:
What is the primary benefit of using Laravel Contracts over traditional class dependencies?
In Laravel, what is the primary purpose of defining a scope in a Policy?
In Laravel's Eloquent ORM, what is the primary purpose of accessors and mutators?
What is the primary purpose of database migrations in Laravel?
What is the primary purpose of Global Scopes in Laravel's Eloquent ORM?
Which of the following Eloquent relationship types allows a model to belong to more than one other model on a single association?
Which of the following is the correct way to define a scheduled task to run every day at 7:00 AM in Laravel's Console/Kernel.php
file?
Which of the following validation rules in Laravel is used to ensure that the field under validation is formatted as a valid URL?
Which Laravel skills should you evaluate during the interview phase?
It's impossible to assess every aspect of a candidate's abilities in a single interview. However, when it comes to Laravel skills, focusing on core areas is key. Evaluating these skills will help you determine if the candidate has the right foundation for your team.
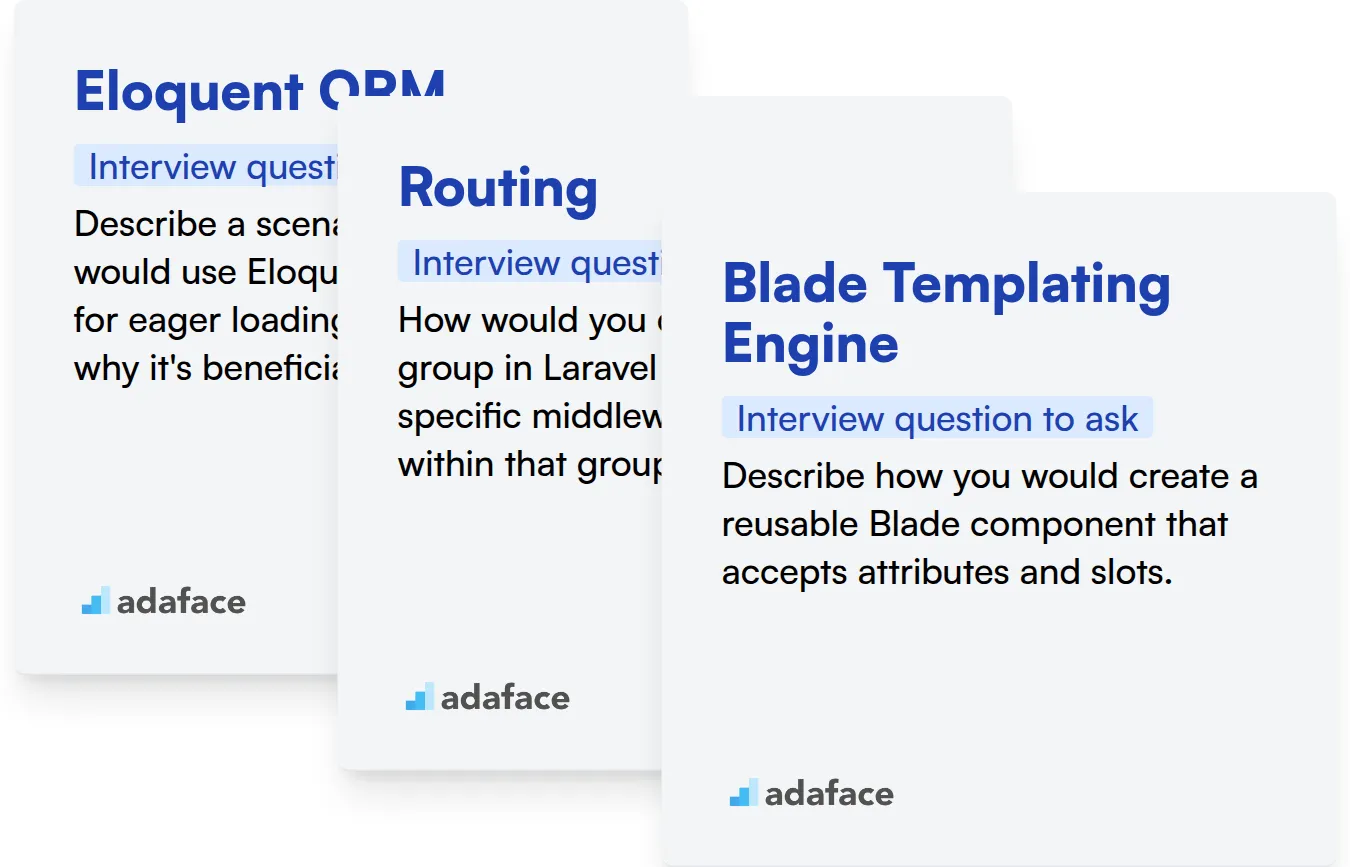
Eloquent ORM
Assess a candidate's Eloquent ORM skills by using targeted MCQs. Our Laravel developer assessment includes questions on Eloquent relationships, query scopes, and model events.
To gauge a candidate's understanding of Eloquent, ask targeted questions. Here's an example question to get you started.
Describe a scenario where you would use Eloquent's with
method for eager loading, and explain why it's beneficial.
Look for the candidate to explain how with
prevents the N+1 query problem, thus optimizing database queries. The best answers will provide practical examples from their own experience.
Routing
Evaluate the candidate's knowledge on Laravel's routing system using MCQs. You can test on topics like route parameters, middleware, and route groups.
To assess a candidate's routing expertise, present a practical problem. Here's a question to try.
How would you create a route group in Laravel that applies a specific middleware to all routes within that group?
The candidate should demonstrate knowledge of route groups and middleware assignment. Good answers will show an understanding of how to use the middleware
method within the Route::group
to apply middleware globally.
Blade Templating Engine
An assessment can help you screen for familiarity with Blade directives, template inheritance, and component usage. Our Laravel developer assessment covers questions on Blade components and slots.
Test a candidate's proficiency with Blade by posing a scenario. Try this question:
Describe how you would create a reusable Blade component that accepts attributes and slots.
Look for understanding of component syntax (@component
), attribute passing, and slot usage ({{ $slot }}
). The best answers will also highlight the benefits of using components for code reuse.
Streamline Your Laravel Hiring with Skills Tests and Targeted Questions
Hiring developers with strong Laravel skills requires verifying their abilities accurately. You need to ensure they possess the practical knowledge to contribute effectively to your projects.
Skills tests provide the most straightforward method for assessing candidates' Laravel expertise. Explore Adaface's range of assessments, including the Laravel Developer Online Test and the PHP Laravel SQL Test, to identify top talent.
After using skills tests to identify the best candidates, you can confidently move forward with interviews. This allows you to focus your time on candidates who have demonstrated a solid understanding of Laravel.
Ready to simplify your Laravel hiring process? Visit our Online Assessment Platform to learn more and sign up for a free trial, or check out our Coding Tests page.
Laravel Online Test
Download Laravel interview questions template in multiple formats
Laravel Interview Questions FAQs
Basic Laravel interview questions cover topics such as routing, controllers, models, and views. They assess a candidate's understanding of the core principles of Laravel.
Intermediate questions explore more advanced topics like middleware, database migrations, Eloquent ORM, and form requests. These determine if a candidate can apply their knowledge to real-world scenarios.
Advanced questions often cover topics like queues, events, caching, testing, and package development. These help determine a candidate's expertise and ability to contribute to complex projects.
Expert level Laravel interview questions might explore topics such as architectural patterns, performance optimization, security best practices, and contribution to the Laravel community itself. The goal is to see how much they know about the Laravel framework and how they can improve it with their extensive knowledge.
Combining skills tests with targeted interview questions is ideal. Skills tests offer quantitative insights into a candidate's practical abilities, while interview questions allow for a more in-depth exploration of their understanding and problem-solving approach.
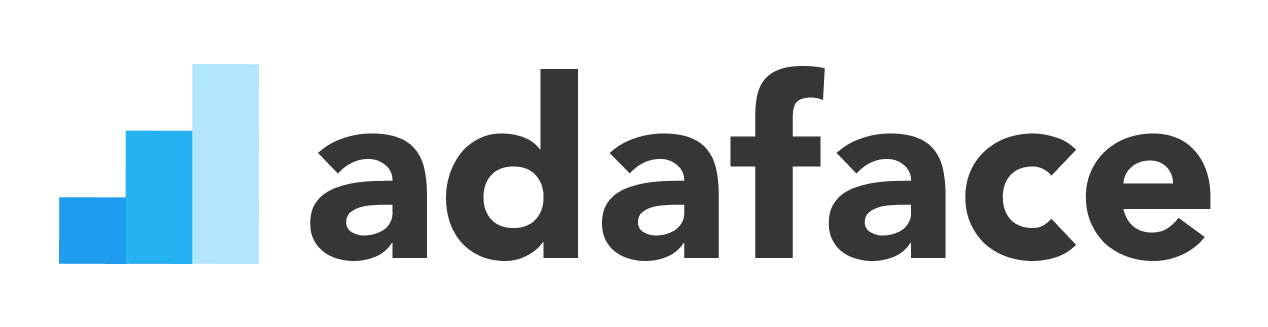
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
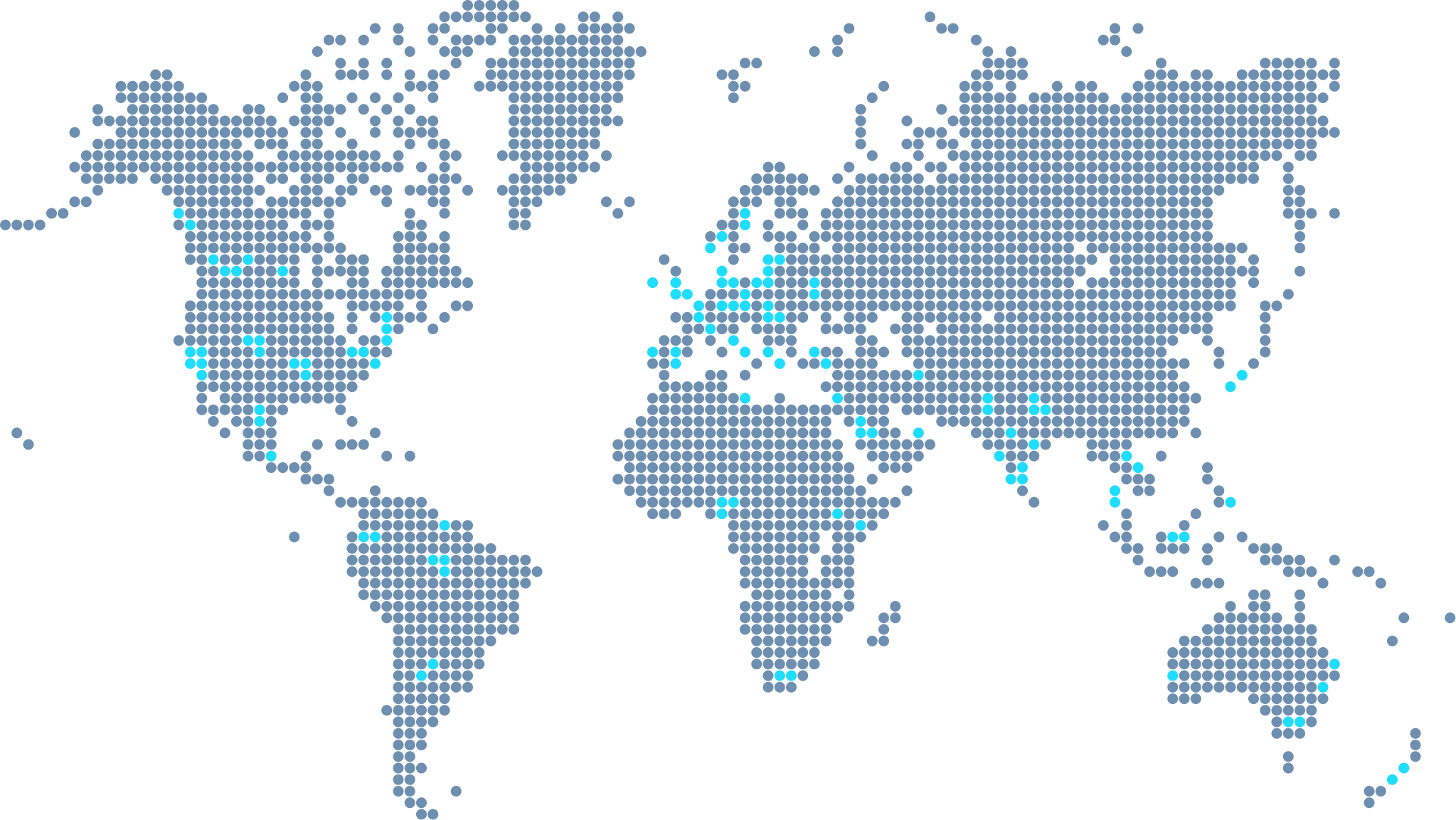
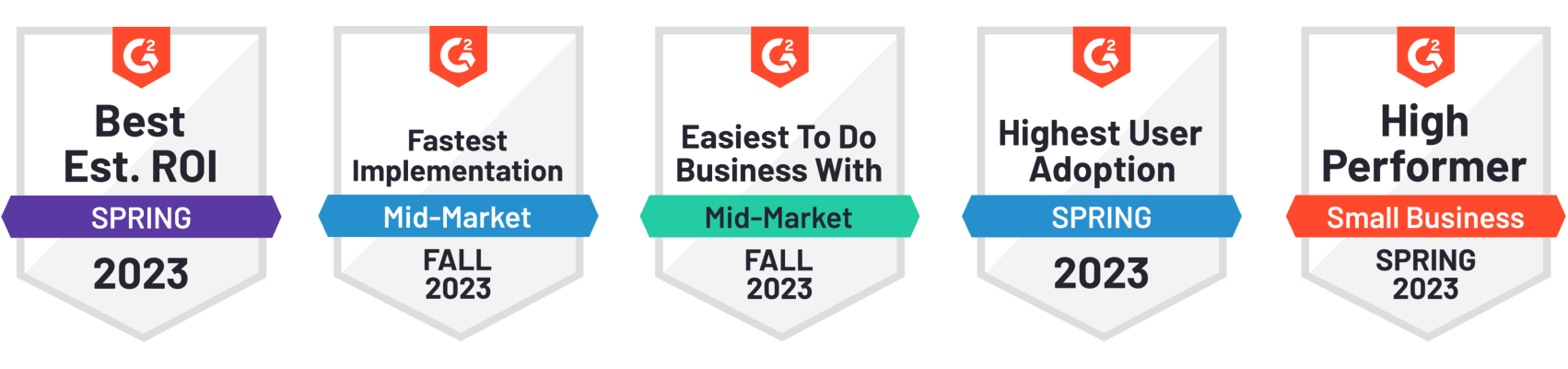