Java 8 introduced significant features that revolutionized Java programming, making it crucial for interviewers to assess candidates' proficiency in these areas. Asking the right Java 8 interview questions can help you identify top talent and ensure they have the necessary skills to excel in your organization.
This blog post provides a comprehensive list of Java 8 interview questions tailored for different experience levels, from junior to senior developers. We've categorized the questions into general, junior, intermediate, advanced, technical definitions, and performance optimization sections to help you evaluate candidates thoroughly.
By using these questions, you can effectively gauge a candidate's Java 8 knowledge and make informed hiring decisions. Consider complementing your interview process with a pre-screening Java assessment to streamline your recruitment efforts and identify the most qualified candidates efficiently.
Table of contents
8 general Java 8 interview questions and answers to assess candidates
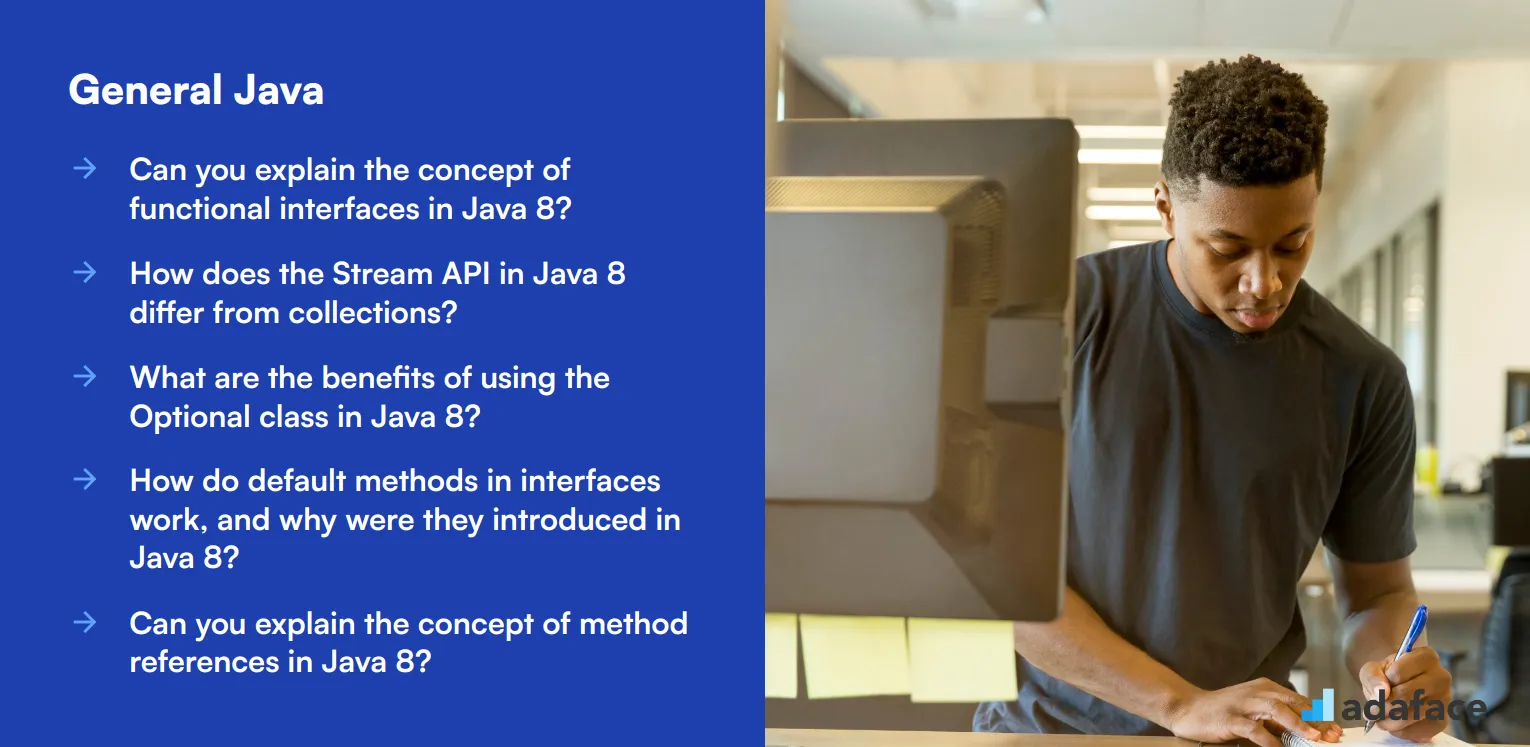
Ready to assess your Java 8 candidates? These 8 general questions will help you gauge a candidate's understanding of key Java 8 concepts. Use them to spark discussions, evaluate problem-solving skills, and uncover how well applicants can apply Java 8 features in real-world scenarios. Remember, the goal is to assess skills rather than stumping candidates with overly technical queries.
1. Can you explain the concept of functional interfaces in Java 8?
A functional interface in Java 8 is an interface that contains exactly one abstract method. These interfaces are the foundation for lambda expressions in Java.
Candidates should mention that functional interfaces can have multiple default or static methods, but only one abstract method. They might also give examples like Runnable, Callable, or Comparator.
Look for answers that demonstrate understanding of how functional interfaces enable more concise and readable code through lambda expressions and method references.
2. How does the Stream API in Java 8 differ from collections?
The Stream API in Java 8 provides a way to process sequences of elements. Unlike collections, streams don't store elements. They carry values from a source through a pipeline of computational operations.
Key differences candidates should mention include:
- Streams are functional in nature and designed for lambdas
- Streams can be infinite, whereas collections are finite
- Stream operations don't modify the source
- Streams are lazily evaluated and can be parallel
Look for answers that show an understanding of how streams can improve code readability and performance, especially when dealing with large data sets or complex operations.
3. What are the benefits of using the Optional class in Java 8?
The Optional class in Java 8 is a container object used to represent the presence or absence of a value. It's designed to reduce null pointer exceptions and improve code readability.
Candidates should mention benefits such as:
- Clearly communicating that a value might be absent
- Forcing developers to actively handle the case when a value is not present
- Providing useful methods to deal with possibly-null values without extensive null checks
Look for answers that demonstrate understanding of how Optional can lead to more robust and expressive code. Candidates who mention real-world scenarios where they've used Optional effectively are showing valuable practical experience.
4. How do default methods in interfaces work, and why were they introduced in Java 8?
Default methods in Java 8 allow developers to add new methods to interfaces without breaking existing implementations. These methods have a default implementation in the interface itself.
Candidates should explain that default methods were introduced to:
- Enable interface evolution without breaking existing code
- Provide a way to add methods to interfaces that all implementations would likely need
- Allow for multiple inheritance of behavior
Look for answers that show understanding of the implications of default methods, such as the potential for the diamond problem and how Java resolves it. Strong candidates might discuss scenarios where they've used or encountered default methods in real projects.
5. Can you explain the concept of method references in Java 8?
Method references in Java 8 provide a way to refer to methods or constructors without executing them. They're a shorthand notation of a lambda expression that executes just one method.
Candidates should be able to explain the four types of method references:
- Reference to a static method
- Reference to an instance method of a particular object
- Reference to an instance method of an arbitrary object of a particular type
- Reference to a constructor
Look for answers that demonstrate how method references can make code more readable and concise. Candidates who can provide examples of when to use each type of method reference are showing a deeper understanding of the concept.
6. What is the significance of the @FunctionalInterface annotation?
The @FunctionalInterface annotation in Java 8 is used to indicate that an interface is intended to be a functional interface. While it's not required for functional interfaces, it helps with code clarity and compiler checks.
Candidates should mention that:
- It's informative, telling users of the interface that it's designed for a specific purpose
- It's checked by the compiler to ensure the interface meets the requirements of a functional interface
- It prevents accidental addition of abstract methods to the interface
Look for answers that show understanding of how this annotation contributes to code reliability and maintainability. Strong candidates might discuss scenarios where they've used or encountered this annotation in their software development projects.
7. How does the forEach method in Java 8 differ from a traditional for loop?
The forEach method in Java 8 is part of the Iterable interface and provides a more functional way to iterate over collections. It accepts a lambda expression or method reference as an argument.
Key differences candidates should mention include:
- forEach is more concise and often more readable
- It can be used with streams for parallel processing
- It doesn't allow for breaking out of the loop early or skipping elements
- It may be less flexible for complex iteration scenarios
Look for answers that demonstrate understanding of when to use forEach versus a traditional loop. Strong candidates will be able to discuss the trade-offs and provide examples of appropriate use cases for each.
8. What are some of the new Date and Time API improvements in Java 8?
Java 8 introduced a new Date and Time API (java.time package) to address the shortcomings of the older java.util.Date and java.util.Calendar classes. This new API is inspired by the Joda-Time library and provides a more intuitive and thread-safe approach to date and time manipulation.
Key improvements candidates should mention include:
- Immutability: The new classes like LocalDate, LocalTime, and LocalDateTime are immutable
- Separation of concerns: Clear separation between human-readable time and machine time
- Better time zone handling with ZonedDateTime
- Support for various calendaring systems
- Improved formatting and parsing capabilities
Look for answers that demonstrate understanding of how these improvements address real-world problems in date and time manipulation. Candidates who can provide examples of migrating from the old API to the new one are showing valuable practical experience.
20 Java 8 interview questions to ask junior developers
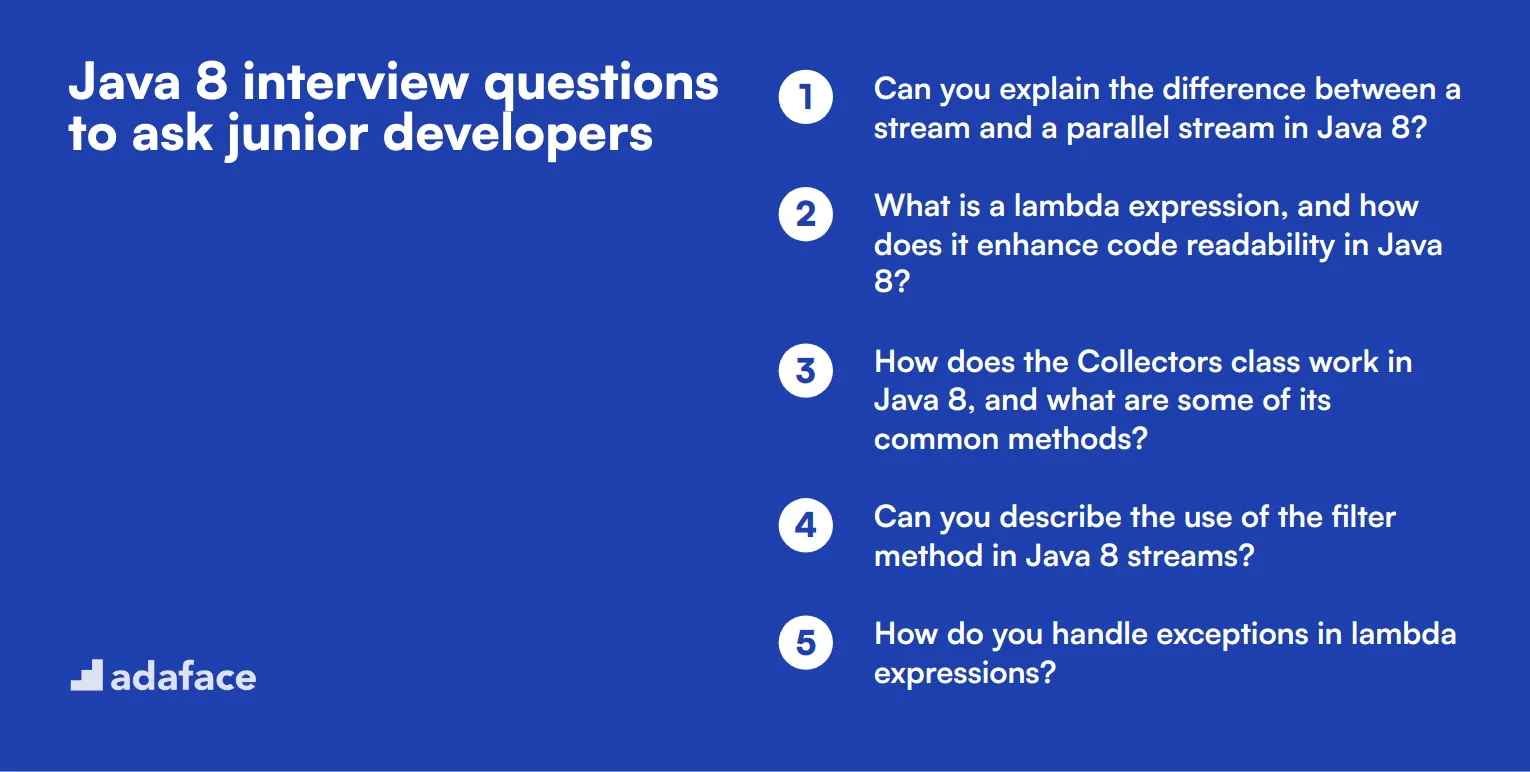
To assess whether your applicants have a strong grasp of Java 8 and can handle real-world coding challenges, use these 20 Java 8 interview questions. This list is tailored to help you gauge their understanding of key concepts and practical skills, ensuring you select the right candidate for your software engineer job description.
- Can you explain the difference between a stream and a parallel stream in Java 8?
- What is a lambda expression, and how does it enhance code readability in Java 8?
- How does the Collectors class work in Java 8, and what are some of its common methods?
- Can you describe the use of the filter method in Java 8 streams?
- How do you handle exceptions in lambda expressions?
- What is the purpose of the IntStream, LongStream, and DoubleStream classes?
- Can you explain how the map method works in Java 8 streams?
- What are the main differences between findFirst and findAny in Java 8 streams?
- How do you convert a stream back to a list or an array in Java 8?
- Explain the concept of stream pipelining in Java 8.
- What is the purpose of the flatMap method in Java 8 streams?
- Can you discuss the difference between intermediate and terminal operations in Java 8 streams?
- How does the reduce method work in Java 8 streams, and when would you use it?
- What are the benefits of using the @Repeatable annotation in Java 8?
- How do you use the Predicate functional interface in Java 8?
- Can you describe the use of the BiFunction interface in Java 8?
- How has the Java 8 Date and Time API improved over the older java.util.Date?
- What are the primary uses of the java.util.concurrent package in Java 8?
- Explain how you would utilize the Spliterator in Java 8.
- How does the CompletableFuture class in Java 8 help with asynchronous programming?
10 intermediate Java 8 interview questions and answers to ask mid-tier developers
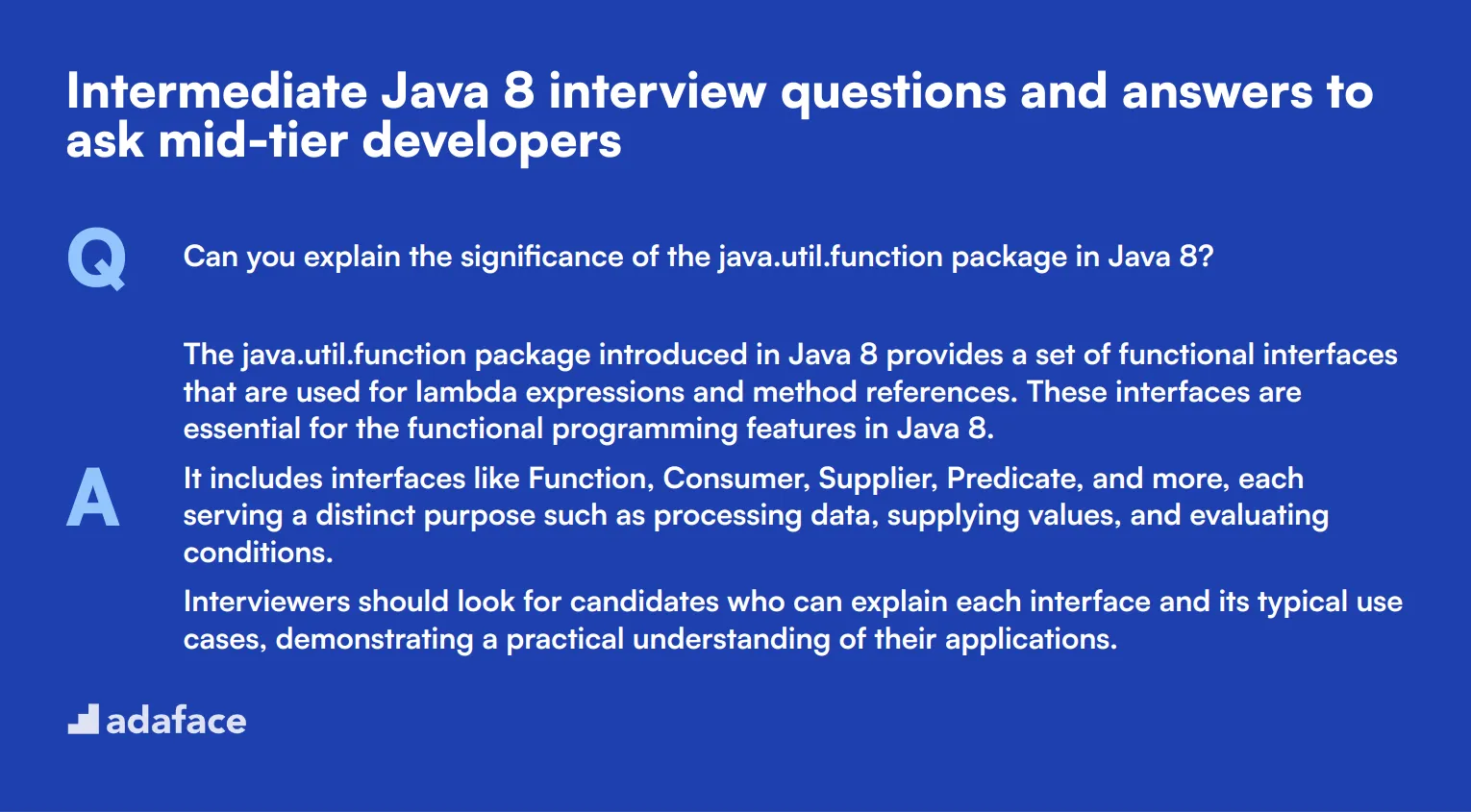
When you’re interviewing mid-tier developers, you need questions that go beyond the basics but aren't too advanced. This list of intermediate Java 8 interview questions will help you pinpoint candidates who not only understand Java 8 but can also apply its features to solve real-world problems effectively.
1. Can you explain the significance of the java.util.function package in Java 8?
The java.util.function package introduced in Java 8 provides a set of functional interfaces that are used for lambda expressions and method references. These interfaces are essential for the functional programming features in Java 8.
It includes interfaces like Function, Consumer, Supplier, Predicate, and more, each serving a distinct purpose such as processing data, supplying values, and evaluating conditions.
Interviewers should look for candidates who can explain each interface and its typical use cases, demonstrating a practical understanding of their applications.
2. What are the main advantages of using the new Java 8 features in terms of code readability and maintainability?
Java 8 introduced several features like lambda expressions, the Stream API, and default methods, which significantly improve code readability and maintainability.
Lambda expressions allow for more concise and readable code by eliminating boilerplate, while the Stream API provides a powerful way to process collections in a declarative manner. Default methods enable interface evolution without breaking existing implementations.
Candidates should illustrate how these features reduce code complexity and enhance clarity, making maintenance easier. Look for examples from their past experiences to validate their understanding.
3. How do you approach debugging streams in Java 8?
Debugging streams in Java 8 can be challenging due to their functional and declarative nature. Developers often rely on techniques like using peek operations to log intermediate states or breaking down complex stream operations into smaller, named methods.
Another approach is to convert the stream operations back into loops temporarily for more traditional debugging. Additionally, using tools like IDE debuggers that support lambda expressions can be helpful.
Candidates should mention these strategies and demonstrate a systematic approach to troubleshooting issues within stream pipelines.
4. What is a Spliterator and how does it differ from an Iterator in Java 8?
A Spliterator is an interface introduced in Java 8 for traversing and partitioning elements of a source, such as a collection. Unlike an Iterator, a Spliterator can split the data structure into multiple parts, which can be processed in parallel using the Stream API.
Spliterators offer additional methods like trySplit and estimateSize, enabling more efficient parallel processing. They are particularly useful for large data sets where parallelism can significantly improve performance.
Candidates should explain these differences and discuss scenarios where using a Spliterator would be advantageous over a traditional Iterator.
5. Can you discuss how the type inference in Java 8 has evolved compared to previous versions?
Java 8 introduced improved type inference capabilities, particularly with lambda expressions and the diamond operator. The compiler can infer parameter types in lambda expressions, reducing the need for explicit type declarations.
Additionally, the diamond operator allows for more concise generic instance creation, inferring types from the context. These enhancements make the code cleaner and easier to read.
Look for candidates who can provide examples demonstrating these improvements and explain how they contribute to more maintainable and readable code.
6. What role do functional interfaces play in enhancing the functional programming capabilities of Java 8?
Functional interfaces are central to Java 8's functional programming features. They enable the use of lambda expressions and method references by defining a single abstract method, which can be implemented concisely.
Common functional interfaces include Runnable, Callable, Comparator, and the new ones in the java.util.function package like Function, Consumer, and Predicate.
Candidates should highlight how these interfaces simplify code and allow for more flexible, higher-order function use. They should also discuss scenarios where functional interfaces provide significant advantages.
7. Explain how the new default methods in interfaces can help with API design and backward compatibility.
Default methods in interfaces allow developers to add new methods to interfaces without breaking existing implementations. This feature is particularly useful in API design, enabling the evolution of interfaces over time.
These methods provide a concrete implementation that existing classes can inherit, ensuring backward compatibility. This approach reduces the need for extensive refactoring and maintains the integrity of existing implementations.
Interviewers should look for candidates who understand the balance between interface evolution and backward compatibility, offering insights into practical scenarios where they have leveraged default methods.
8. What is the purpose of the java.util.Optional class, and how does it help in handling null values?
The java.util.Optional class in Java 8 is designed to handle null values more gracefully, reducing the risk of NullPointerExceptions. It is a container that may or may not hold a non-null value.
Optional provides methods like isPresent, ifPresent, orElse, and orElseGet to check and retrieve values safely. This approach encourages more expressive and safer code by explicitly handling the absence of values.
Candidates should discuss how Optional improves code robustness and clarity, potentially sharing examples from their experience where it has mitigated null-related issues.
9. How does the Collectors class enhance data collection and aggregation in Java 8?
The Collectors class in Java 8 provides a wide range of methods for data collection and aggregation, making it easier to transform streams into collections, sums, averages, and other summary statistics.
Some commonly used methods include toList, toSet, groupingBy, and joining. These methods enable developers to perform complex data manipulations in a concise and readable manner.
Candidates should be able to explain the utility of these methods and provide examples of how they have used Collectors to simplify data processing tasks in their projects.
10. Can you explain the use of the Predicate functional interface and provide a practical example?
The Predicate functional interface represents a single argument function that returns a boolean value. It is often used for filtering data in streams and other collections processing.
A typical use case is the filter method in streams, where Predicate is used to define the condition for elements to be included in the output stream.
Candidates should provide a practical example, such as filtering a list of employees based on a condition, demonstrating their understanding of Predicate's role in functional programming.
10 advanced Java 8 interview questions to ask senior developers
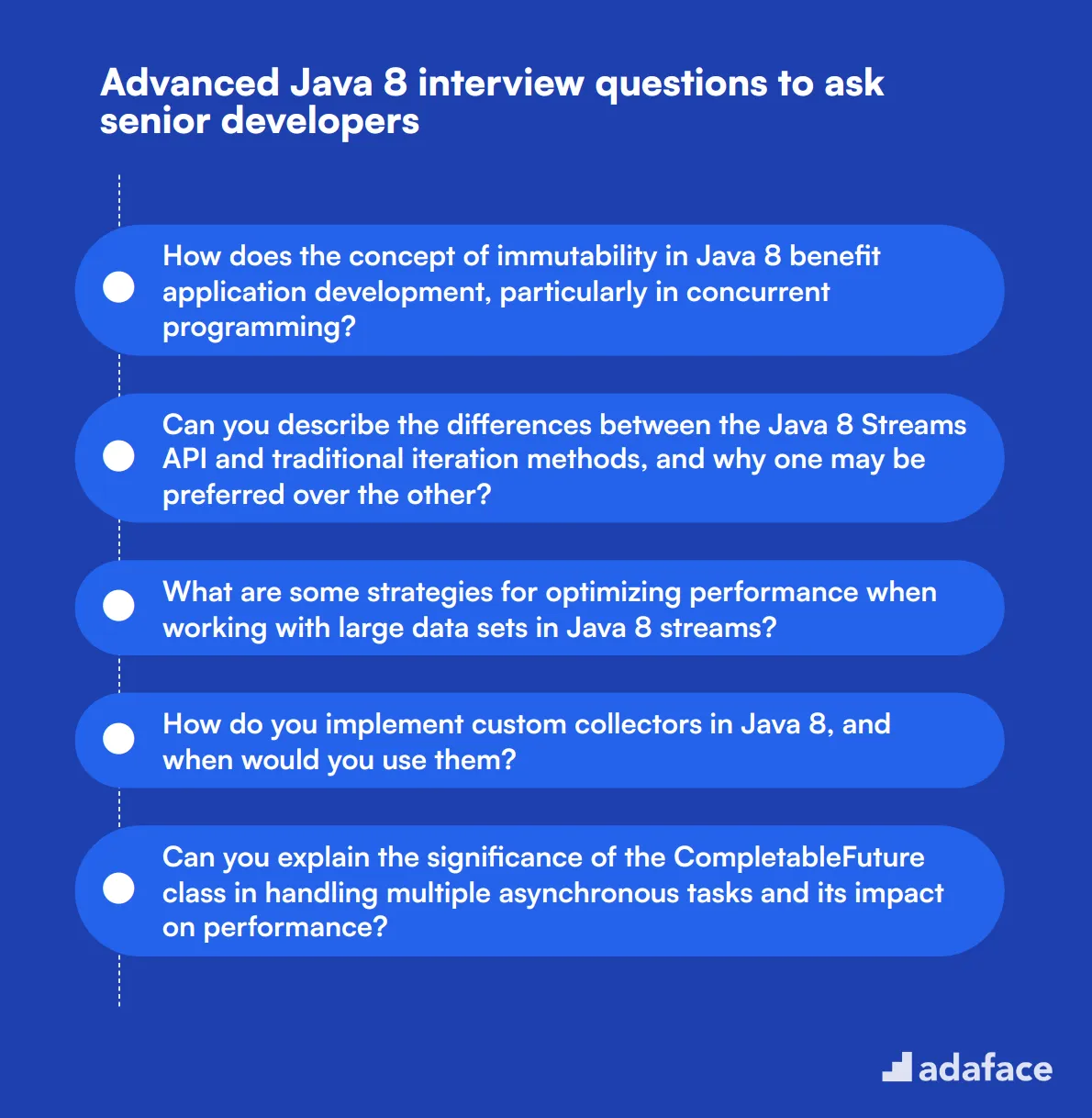
To assess whether candidates possess advanced knowledge of Java 8, consider using these targeted interview questions. They are designed to evaluate a developer's ability to leverage new features effectively, helping you identify top talent for your team. For more insights, refer to our Java Developer Job Description.
- How does the concept of immutability in Java 8 benefit application development, particularly in concurrent programming?
- Can you describe the differences between the Java 8 Streams API and traditional iteration methods, and why one may be preferred over the other?
- What are some strategies for optimizing performance when working with large data sets in Java 8 streams?
- How do you implement custom collectors in Java 8, and when would you use them?
- Can you explain the significance of the CompletableFuture class in handling multiple asynchronous tasks and its impact on performance?
- What is the role of the @FunctionalInterface annotation in Java 8, and how does it facilitate functional programming?
- How do you ensure thread safety when using mutable data structures in Java 8?
- Can you explain how the concept of 'Optional' can be integrated into a legacy codebase effectively?
- What are the advantages of using method references over lambda expressions in Java 8?
- How would you use the Stream API to perform a grouping operation on a collection of objects?
6 Java 8 interview questions and answers related to technical definitions
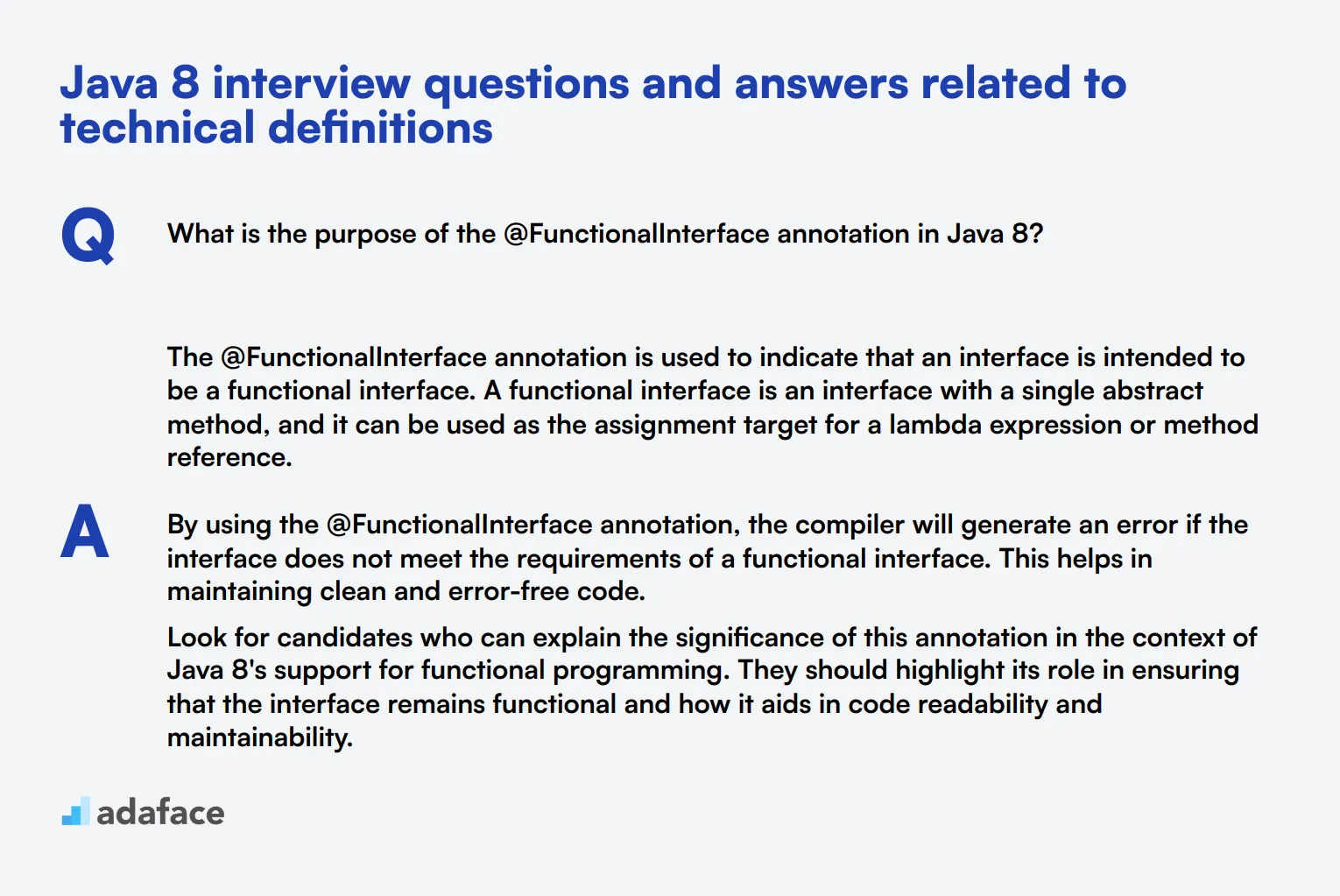
To assess whether your candidates have a solid understanding of Java 8's technical definitions, ask them some of these interview questions. These questions aim to uncover their grasp on key concepts and ensure they can apply them in practical scenarios.
1. What is the purpose of the @FunctionalInterface annotation in Java 8?
The @FunctionalInterface annotation is used to indicate that an interface is intended to be a functional interface. A functional interface is an interface with a single abstract method, and it can be used as the assignment target for a lambda expression or method reference.
By using the @FunctionalInterface annotation, the compiler will generate an error if the interface does not meet the requirements of a functional interface. This helps in maintaining clean and error-free code.
Look for candidates who can explain the significance of this annotation in the context of Java 8's support for functional programming. They should highlight its role in ensuring that the interface remains functional and how it aids in code readability and maintainability.
2. How does the Stream API in Java 8 help with processing collections of data?
The Stream API in Java 8 allows for processing collections of data in a declarative manner. It supports operations such as filtering, mapping, and reducing, which can be chained together to form a pipeline of transformations.
Streams provide a more efficient and readable way to process data compared to traditional loops by abstracting away the boilerplate code. They also support parallel processing, which can lead to performance improvements on multi-core systems.
Ideal answers should demonstrate an understanding of how streams can simplify code and improve performance. Candidates should highlight the benefits of using streams over traditional iteration methods, such as better readability and the potential for parallel execution.
3. What is the purpose of the Optional class in Java 8?
The Optional class in Java 8 is a container object which may or may not contain a non-null value. It is used to represent the presence or absence of a value, helping to avoid null pointer exceptions.
Optional provides methods to check for the presence of a value, retrieve the value if present, and provide a default value if the value is absent. This encourages a more functional and expressive approach to handling null values.
Candidates should be able to discuss how Optional can improve code safety and readability by making the handling of null values more explicit. Look for explanations that emphasize its role in reducing the likelihood of null pointer exceptions.
4. How do default methods in interfaces work in Java 8?
Default methods in interfaces allow the addition of new methods to interfaces without breaking existing implementations. A default method has a body and can be overridden by classes that implement the interface.
The main purpose of default methods is to enable interface evolution, allowing new methods to be added to interfaces without requiring all implementing classes to provide an implementation.
An ideal response should explain the concept clearly and mention scenarios where default methods can be particularly useful, such as in maintaining backward compatibility when updating an interface.
5. What is the significance of method references in Java 8?
Method references in Java 8 provide a shorthand syntax for calling a method. They are a type of lambda expression that refers to a method by name instead of invoking it directly.
Method references can improve code readability by reducing boilerplate code. They can be used to refer to static methods, instance methods, and constructors.
Look for answers that highlight how method references can make code more concise and readable. Candidates should provide examples of when method references might be preferred over lambda expressions.
6. Can you explain the concept of stream pipelining in Java 8?
Stream pipelining in Java 8 refers to the process of chaining multiple stream operations together to form a sequence of computational steps. Streams support both intermediate operations, which transform the stream, and terminal operations, which produce a result or side-effect.
Intermediate operations are lazy and are not executed until a terminal operation is invoked. This allows for optimizations such as short-circuiting and deferred execution.
Candidates should demonstrate an understanding of how stream pipelining allows for more efficient and readable data processing. They should be able to explain the difference between intermediate and terminal operations and give examples of each.
9 Java 8 interview questions and answers related to performance optimization
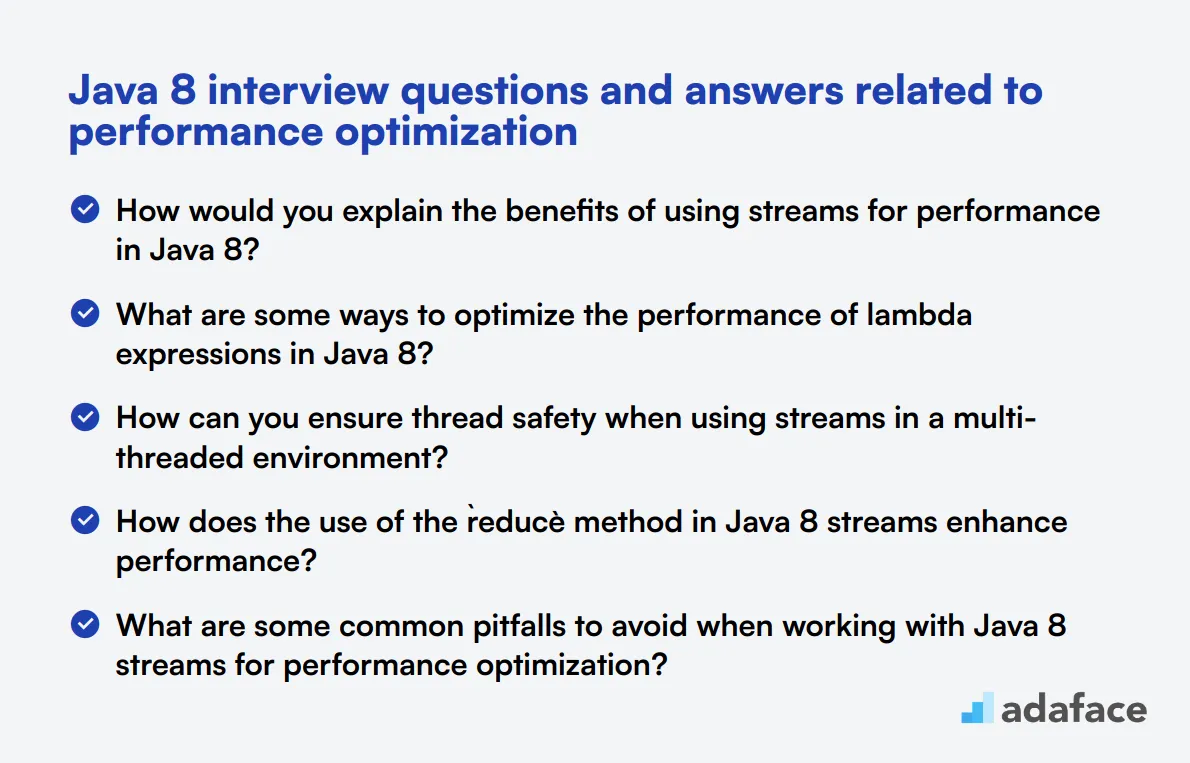
To ensure your candidates can optimize performance with Java 8, ask them these targeted interview questions. These questions are designed to reveal their understanding of key concepts and best practices for performance enhancement.
1. How would you explain the benefits of using streams for performance in Java 8?
Using streams in Java 8 can significantly improve performance by enabling parallel processing. Streams are designed to support operations such as map-reduce transformations, which can be easily parallelized across multiple CPU cores.
Streams also offer a high level of abstraction, reducing the complexity of code and making it more readable and maintainable. This helps in identifying performance bottlenecks more easily.
Look for candidates who can articulate the advantages of streams in terms of parallel processing and code simplicity. They should also mention the potential trade-offs, such as the overhead of thread management.
2. What are some ways to optimize the performance of lambda expressions in Java 8?
To optimize lambda expressions, one can use them judiciously to avoid unnecessary object creation, which can lead to increased garbage collection. Another approach is to leverage method references where applicable, as they can be more efficient.
Inlining small lambda expressions directly within the code can also help reduce overhead. Additionally, ensure that lambda expressions do not capture any variables that may lead to unintended object retention.
Good answers should demonstrate an understanding of how to use lambda expressions efficiently and recognize situations where method references might be more suitable. They should also be aware of potential pitfalls like unintended object retention.
3. How can you ensure thread safety when using streams in a multi-threaded environment?
Ensuring thread safety with streams in a multi-threaded environment involves a few strategies. One key approach is to use concurrent collections like ConcurrentHashMap
and CopyOnWriteArrayList
when collecting results from parallel streams.
Another important consideration is to avoid shared mutable state within the stream operations. Immutable data structures and stateless operations can help maintain thread safety.
Candidates should be able to explain these concepts and discuss specific practices like using concurrent collections or avoiding shared mutable state. Their answers should reflect an understanding of the complexities involved in multi-threaded environments.
4. How does the use of the `reduce` method in Java 8 streams enhance performance?
The reduce
method in Java 8 streams is a powerful tool for performing aggregation operations. It allows for efficient reduction of data sets into a single result by using associative and commutative operations, which can be parallelized for better performance.
By providing a single point of aggregation, the reduce
method reduces the need for intermediate data structures, thus optimizing memory usage and processing time.
Look for candidates who can explain the benefits of using reduce
for aggregation and how it can improve performance through parallel execution. They should also be aware of when and how to use the method effectively.
5. What are some common pitfalls to avoid when working with Java 8 streams for performance optimization?
One common pitfall is the misuse of parallel streams, which can lead to performance degradation rather than improvement if not used correctly. It's important to assess the nature of the task and the underlying hardware before deciding to use parallel streams.
Another pitfall is excessive use of intermediate operations that can lead to unnecessary computation. It's crucial to design stream pipelines carefully to minimize redundant operations.
Strong answers should highlight these pitfalls and provide examples or scenarios where they might occur. Candidates should also suggest best practices for avoiding these issues, such as proper assessment before using parallel streams and careful pipeline design.
6. How can the `Collectors` class be used to enhance performance in Java 8 streams?
The Collectors
class in Java 8 provides a variety of methods for efficient data collection and aggregation. For instance, using toList
, toMap
, and groupingBy
can help efficiently gather and organize data from streams.
Custom collectors can also be created to perform specialized collection tasks, allowing for more optimized and context-specific performance enhancements.
Candidates should demonstrate familiarity with common collector methods and the ability to create custom collectors when needed. They should be able to explain how these can be used to optimize performance in specific scenarios.
7. In what scenarios would you prefer using parallel streams over sequential streams in Java 8?
Parallel streams are beneficial when dealing with large data sets that can be divided into independent tasks. They leverage multiple CPU cores to process data in parallel, leading to significant performance improvements.
However, parallel streams may not be suitable for all scenarios, especially those involving I/O operations or tasks that are not easily parallelizable. They also introduce overhead due to thread management, which should be considered.
An ideal candidate should be able to identify scenarios where parallel processing can offer performance gains and discuss the trade-offs involved. They should also know when to avoid parallel streams to prevent unnecessary overhead.
8. What strategies can you use to handle large data sets efficiently in Java 8?
Handling large data sets efficiently in Java 8 can be achieved through strategies like using streams for lazy evaluation, which processes elements on-demand and helps reduce memory consumption.
Another strategy is to leverage parallel streams for concurrent processing, provided that the tasks can be parallelized without introducing significant overhead. Additionally, using efficient data structures and avoiding unnecessary data copying can enhance performance.
Candidates should discuss these strategies and demonstrate an understanding of how to apply them effectively. They should also be aware of the potential limitations and trade-offs involved in each approach.
9. How can the use of the `Optional` class in Java 8 contribute to performance optimization?
The Optional
class in Java 8 helps avoid null checks and reduce the likelihood of NullPointerException
, leading to cleaner and more readable code. This can indirectly contribute to performance optimization by eliminating defensive programming practices.
Using Optional
can also improve method chaining and functional programming patterns, making the code more efficient and easier to optimize.
Look for candidates who can explain how Optional
enhances code quality and reduces the need for defensive checks. They should also discuss its role in improving overall code performance through better design patterns.
Which Java 8 skills should you evaluate during the interview phase?
While one interview can't cover every aspect of a candidate's skills, focusing on core Java 8 competencies can provide a robust assessment. These key skills will help you gauge a candidate's proficiency and readiness for your specific needs.
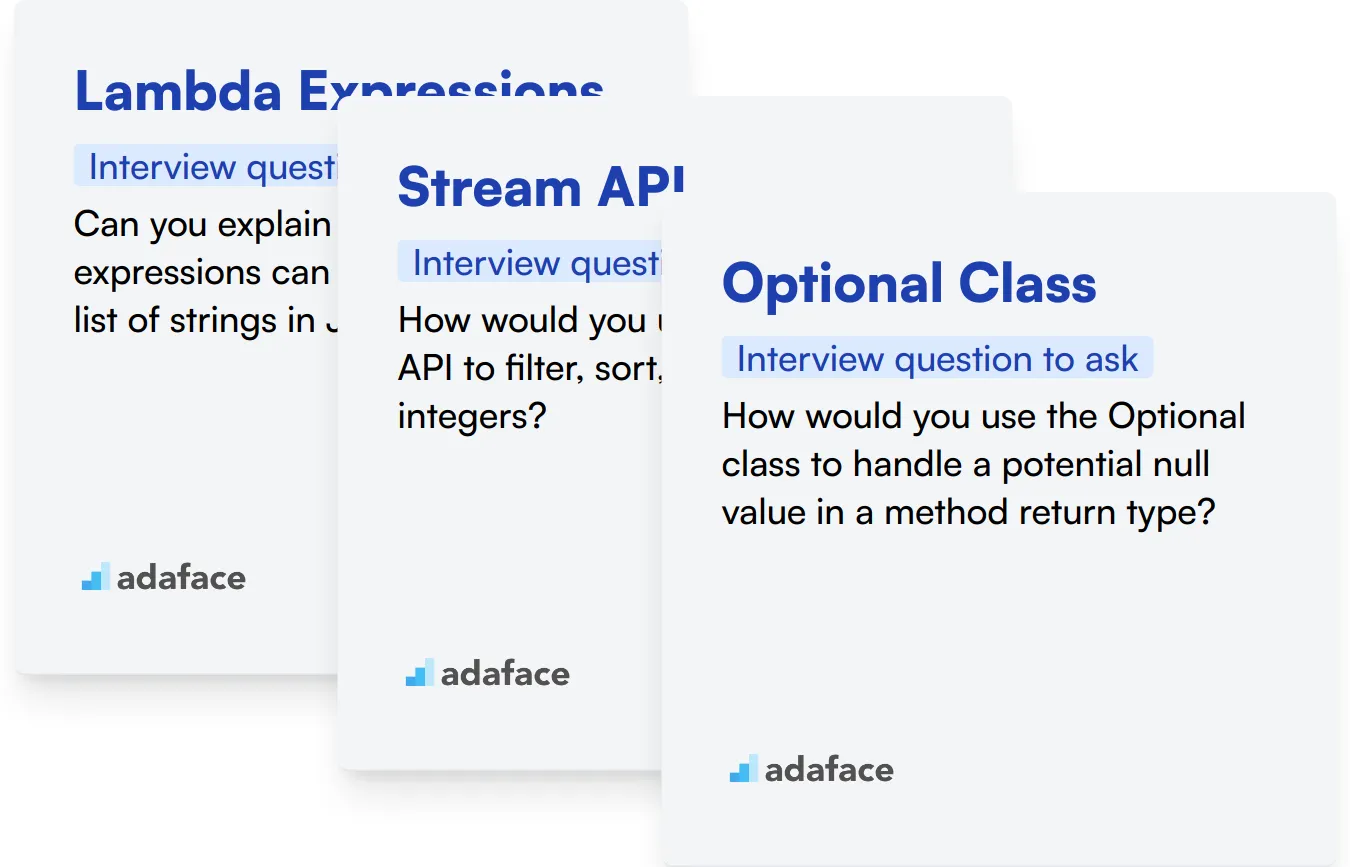
Lambda Expressions
Lambda expressions are a major addition in Java 8, allowing for more readable and concise code. They enable functional programming in Java, making it easier to write and maintain code.
You can use an assessment test that asks relevant MCQs to filter out this skill, like the Java online test.
To assess this skill during the interview, you can ask a targeted question about lambda expressions.
Can you explain how lambda expressions can be used to sort a list of strings in Java 8?
Look for candidates who can clearly explain the syntax and usage of lambda expressions in sorting operations. They should be able to demonstrate knowledge of Java 8's Stream API and functional interfaces.
Stream API
The Stream API in Java 8 introduces a new abstraction to process sequences of elements in a declarative way. It makes data processing easy and efficient.
You can use an assessment test that asks relevant MCQs to filter out this skill, like the Java online test.
To evaluate a candidate's understanding of the Stream API, consider asking specific usage questions.
How would you use the Stream API to filter, sort, and print a list of integers?
Look for candidates who can articulate the use of stream operations like filter, sorted, and forEach, demonstrating an understanding of intermediate and terminal operations in streams.
Optional Class
The Optional class in Java 8 is used to represent optional values that can either contain a value or be empty. It helps in avoiding null pointer exceptions.
During the interview, you can ask a question to explore the candidate's grasp of the Optional class.
How would you use the Optional class to handle a potential null value in a method return type?
Candidates should explain how to create, check, and retrieve values from an Optional instance, highlighting methods like of(), isPresent(), and ifPresent(). This demonstrates their understanding of handling null values effectively.
3 Tips for Effectively Using Java 8 Interview Questions
Before you put your newfound knowledge to use, here are some tips to enhance your interview process and ensure you select the best candidates.
1. Implement Skills Tests Before Interviews
Utilizing skills tests before interviews helps filter candidates based on their actual abilities rather than their resumes. This ensures that only those with the necessary competencies make it to the interview stage.
For Java 8, consider using assessments like the Java Online Test to evaluate programming skills. This can be followed by targeted tests for Java Spring or Hibernate to further assess proficiency in relevant frameworks.
By implementing these tests early, you streamline the interview process, allowing you to focus on candidates who have demonstrated their skills. This also helps to align your interview questions with the skills that matter most for the role.
2. Select and Compile Relevant Interview Questions
With limited time during interviews, prioritize selecting a small number of focused questions. This approach maximizes the effectiveness of your evaluations and allows for deeper discussions.
Consider incorporating questions from related areas such as Java Spring or SQL to gauge candidates' broader technical capabilities. You might also want to address soft skills like communication and culture fit, which are critical for team dynamics.
By carefully curating your questions, you ensure that you cover essential aspects without overwhelming the candidate, leading to more productive interviews.
3. Ask Follow-Up Questions
Simply asking interview questions is not enough; follow-up questions are key to uncovering a candidate’s true understanding and experience. This helps you gauge whether they can apply concepts in real-world situations.
For example, if a candidate explains the use of streams in Java 8, a good follow-up question might be, 'Can you give an example of how you've implemented streams to optimize a process?' This encourages candidates to demonstrate their depth of knowledge and practical experience.
Use Java 8 interview questions and skill tests to hire talented developers
If you are looking to hire someone with Java 8 skills, you need to ensure they possess those skills accurately. The best way to achieve this is to use skill tests such as the Java Online Test or the Java Spring Test.
Once you have used these tests, you can shortlist the best applicants and call them for interviews. You can sign up for our platform here or explore our online assessment platform for more options.
Java Online Test
Download Java 8 interview questions template in multiple formats
Java 8 Interview Questions FAQs
Java 8 interview questions help assess the candidate's proficiency with new features introduced in Java 8, such as streams, lambdas, and more.
Interviewers should look for clear understanding, practical examples, and the ability to explain Java 8 features and their benefits.
Candidates can prepare by understanding Java 8 features, practicing coding problems, and familiarizing themselves with common interview questions.
Some key features of Java 8 include lambda expressions, the Stream API, the new date and time API, and default methods in interfaces.
Java 8 features such as the Stream API and lambda expressions can improve performance by enabling more efficient data processing and cleaner, more maintainable code.
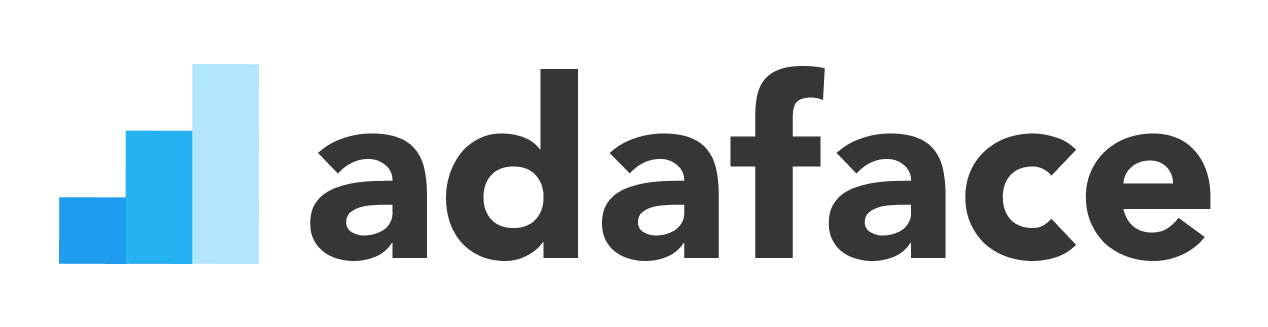
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
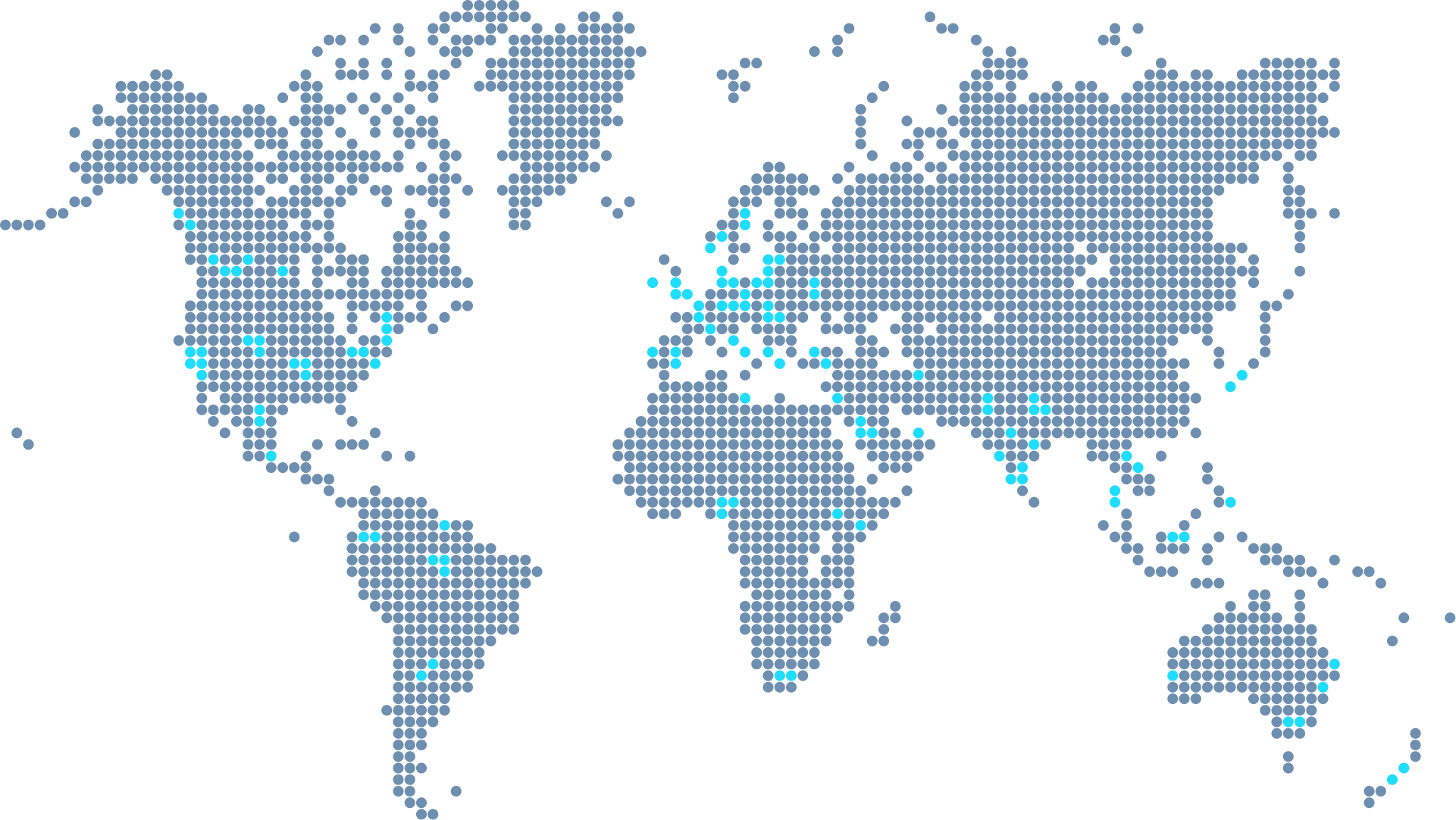
