Hiring skilled Scala developers is crucial for companies looking to leverage this powerful programming language. Conducting effective Scala interviews requires a well-prepared set of questions that can accurately assess a candidate's knowledge and expertise.
This blog post provides a comprehensive list of Scala interview questions, categorized for different experience levels and focusing on key concepts. From basic questions to evaluate junior developers to advanced queries on functional programming and situational scenarios, we've got you covered.
By using these questions, you can streamline your hiring process and identify top Scala talent. Consider complementing your interviews with a Scala skills assessment to get a more holistic view of candidates' abilities.
Table of contents
10 Scala interview questions to initiate the interview
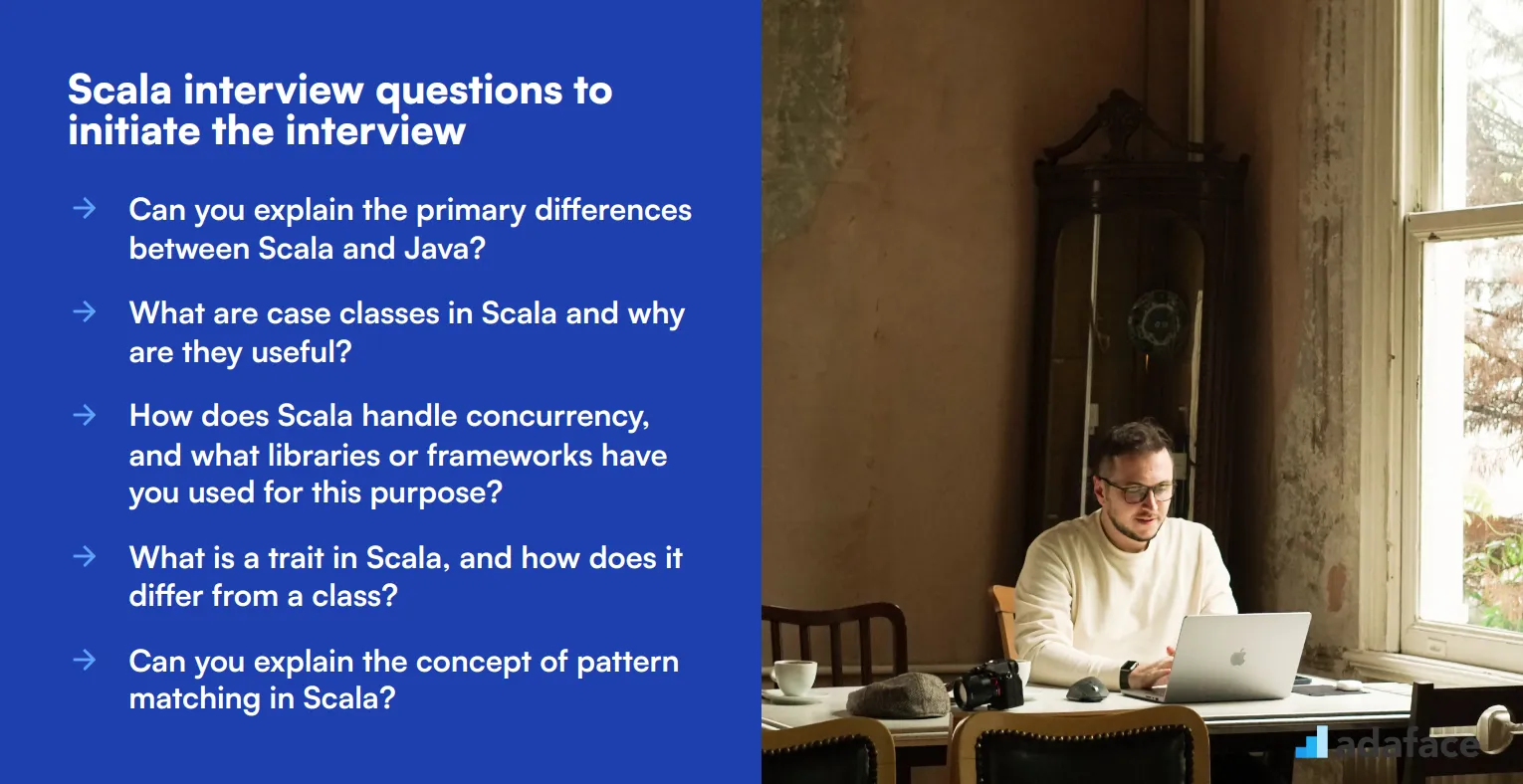
To determine if candidates have the necessary expertise in Scala, consider asking these 10 Scala interview questions. These questions can help you assess their technical skills and ensure they fit the Scala developer job description.
- Can you explain the primary differences between Scala and Java?
- What are case classes in Scala and why are they useful?
- How does Scala handle concurrency, and what libraries or frameworks have you used for this purpose?
- What is a trait in Scala, and how does it differ from a class?
- Can you explain the concept of pattern matching in Scala?
- How do you implement immutability in Scala, and why is it important?
- Describe how implicit classes and methods work in Scala.
- What are the key benefits of using Scala's functional programming features?
- How do you manage dependencies in a Scala project?
- Can you give an example of how you have used higher-order functions in Scala?
8 Scala interview questions and answers to evaluate junior developers
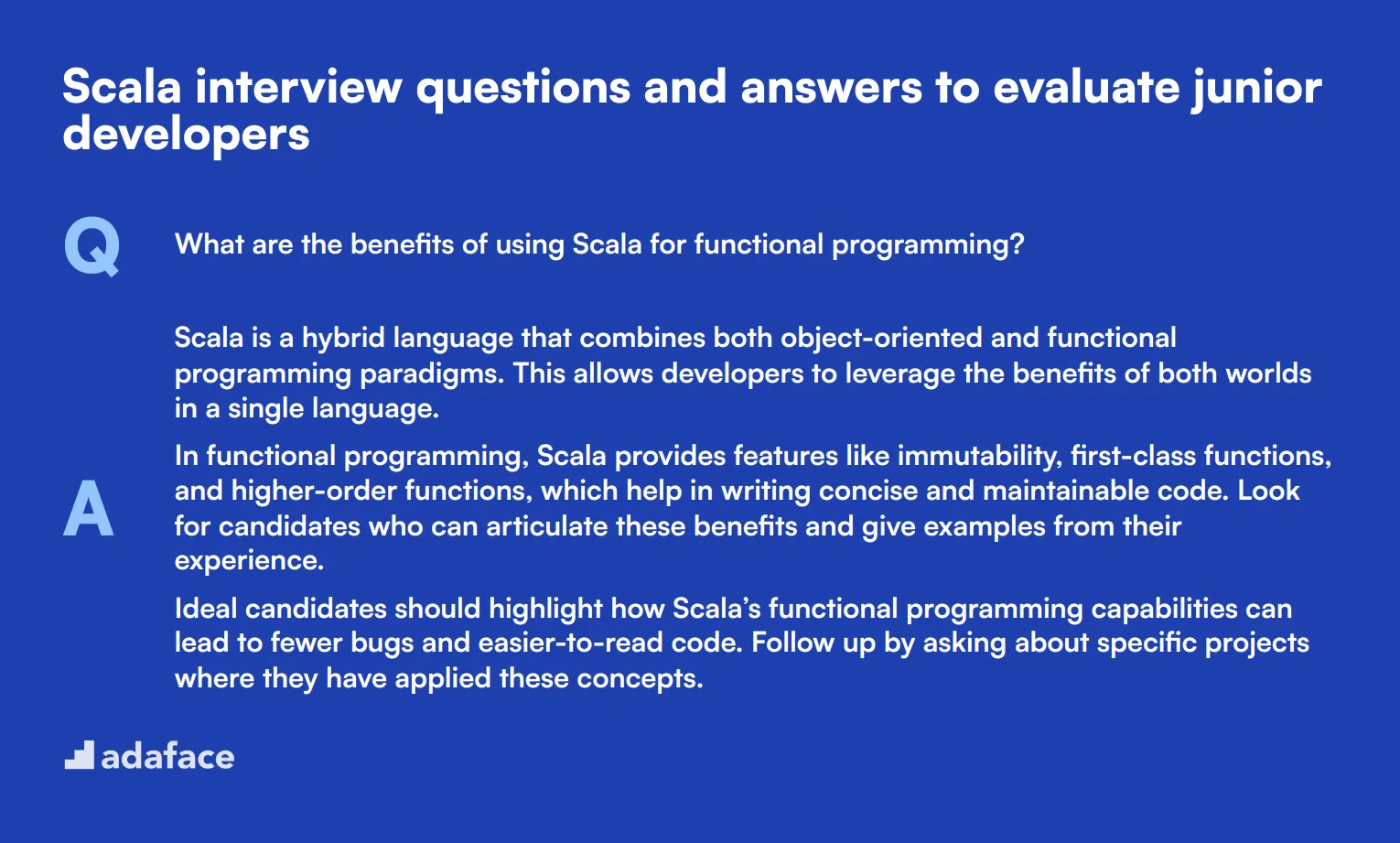
To gauge whether your junior Scala candidates have a solid grasp of fundamental concepts, use these eight interview questions. They are designed to help you assess their foundational knowledge and problem-solving abilities in a straightforward, effective way.
1. What are the benefits of using Scala for functional programming?
Scala is a hybrid language that combines both object-oriented and functional programming paradigms. This allows developers to leverage the benefits of both worlds in a single language.
In functional programming, Scala provides features like immutability, first-class functions, and higher-order functions, which help in writing concise and maintainable code. Look for candidates who can articulate these benefits and give examples from their experience.
Ideal candidates should highlight how Scala’s functional programming capabilities can lead to fewer bugs and easier-to-read code. Follow up by asking about specific projects where they have applied these concepts.
2. Can you describe the role of a companion object in Scala?
A companion object in Scala is an object that shares the same name as a class and is defined in the same file. It is used for defining methods and values that are related to the class but do not require an instance of the class to be accessed.
Companion objects are useful for implementing factory methods, utility functions, and can also be used to define apply and unapply methods for pattern matching. Candidates should mention that the companion object and the class can access each other's private members.
Check if the candidate understands the practical applications of companion objects and can provide scenarios where they have utilized this feature in their projects. Follow up with questions on how they manage companion object methods in large codebases.
3. How does Scala handle exceptions, and what is a 'Try' in Scala?
Scala handles exceptions using a similar approach to Java, with try-catch blocks. However, it also introduces the Try
class to handle exceptions in a more functional way.
The Try
class is a type that represents a computation that may either result in a value (Success
) or an exception (Failure
). This allows for chaining operations in a functional style while handling errors elegantly.
Look for candidates who can explain the advantages of using Try
over traditional try-catch blocks, such as better readability and easier handling of multiple operations that may fail. They should also be able to give examples of how they've used Try
in their projects.
4. What is a higher-order function in Scala and how is it useful?
A higher-order function is a function that takes other functions as parameters or returns a function as a result. This is a powerful feature in Scala that allows for flexible and reusable code.
Higher-order functions enable developers to create generic functions that can be customized with specific behavior by passing different functions as arguments. This leads to cleaner and more modular code.
Candidates should be able to discuss scenarios where they have used higher-order functions to simplify their code. Look for examples that demonstrate their understanding and ability to implement this concept effectively.
5. Can you explain the concept of lazy evaluation in Scala?
Lazy evaluation in Scala means that an expression is not evaluated until its value is actually needed. This can improve performance by avoiding unnecessary computations.
Scala provides the lazy
keyword to defer the evaluation of a variable until it is accessed for the first time. This can be particularly useful in scenarios where the initialization of a variable is resource-intensive.
Good candidates will mention that lazy evaluation can help with optimizing performance and reducing memory usage. They should also provide examples of how they have applied lazy evaluation in their projects.
6. What is an Option in Scala and how do you use it?
An Option
in Scala is a container that can either hold a value (Some
) or no value (None
). It is used to represent the presence or absence of a value, reducing the need for null checks.
Using Option
helps to make code more robust and less error-prone by explicitly handling cases where a value might be missing. This can lead to clearer and more maintainable code.
Look for candidates who can explain the practical benefits of using Option
and provide examples of how they have used it to handle optional values in their projects. Follow up by asking how they handle cases where an Option
might be empty.
7. Why is immutability important in Scala?
Immutability means that once an object is created, its state cannot be changed. In Scala, immutability is a core principle, especially in functional programming.
Immutability helps to avoid side effects and makes code easier to reason about and debug. It also leads to safer concurrent programming since immutable objects can be shared between threads without synchronization.
Candidates should highlight how immutability contributes to more reliable and maintainable code. They should provide examples of how they have designed immutable data structures in their projects and the benefits they have observed.
8. What are implicit parameters in Scala, and how are they useful?
Implicit parameters in Scala are parameters that are passed automatically by the compiler when a method is called, provided that the implicit values are in scope.
They are useful for reducing boilerplate code and can be used for dependency injection, type classes, and context passing. Implicit parameters allow for more concise and readable code.
Look for candidates who can explain the advantages and potential pitfalls of using implicit parameters. They should provide examples of how they have used implicit parameters in their projects and discuss scenarios where they are most beneficial.
14 Scala questions related to functional programming concepts
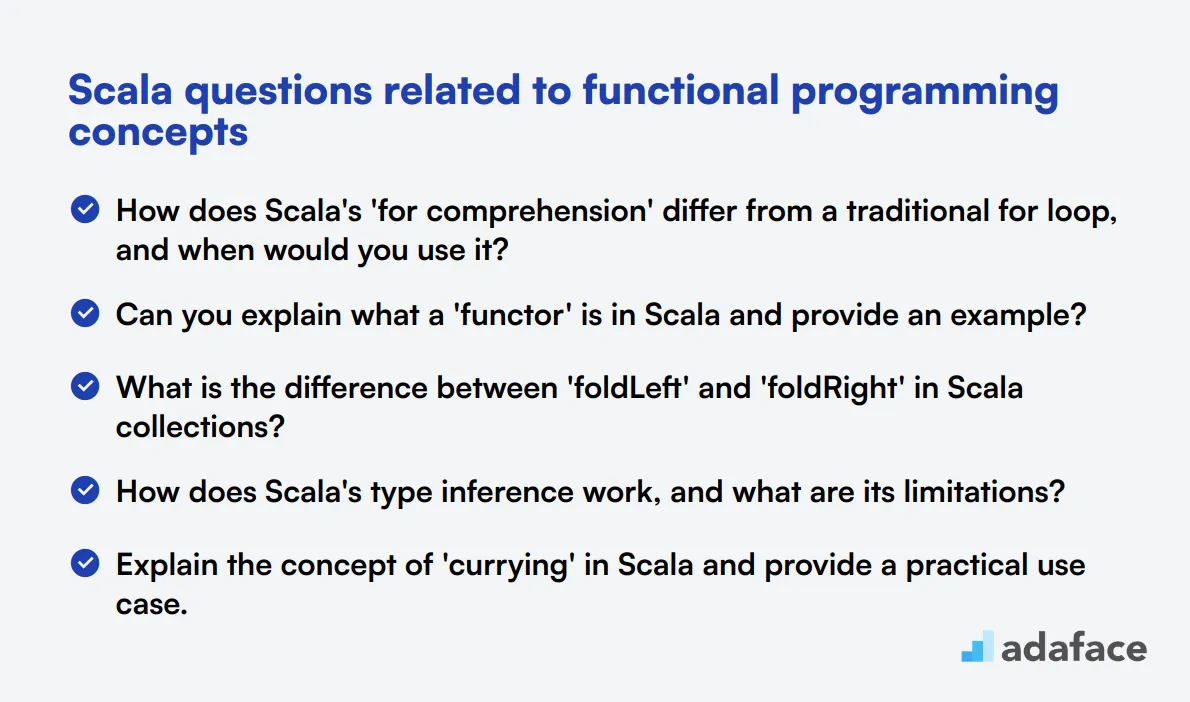
To assess a candidate's proficiency in Scala's functional programming concepts, use these targeted questions during your interview process. These questions will help you evaluate the software engineer's understanding of functional paradigms and their application in Scala development.
- How does Scala's 'for comprehension' differ from a traditional for loop, and when would you use it?
- Can you explain what a 'functor' is in Scala and provide an example?
- What is the difference between 'foldLeft' and 'foldRight' in Scala collections?
- How does Scala's type inference work, and what are its limitations?
- Explain the concept of 'currying' in Scala and provide a practical use case.
- What is the purpose of the 'apply' and 'unapply' methods in Scala?
- How do you implement and use partial functions in Scala?
- Can you explain what 'tail recursion' is and how Scala optimizes it?
- What are 'type classes' in Scala, and how do they support ad-hoc polymorphism?
- How does Scala's 'Future' work, and how does it differ from Java's CompletableFuture?
- Explain the concept of 'monads' in Scala and provide an example.
- What is the difference between 'val', 'lazy val', and 'def' in Scala?
- How do you use 'Either' for error handling in Scala?
- Can you explain what 'by-name parameters' are in Scala and when to use them?
6 Scala interview questions and answers related to functional programming concepts
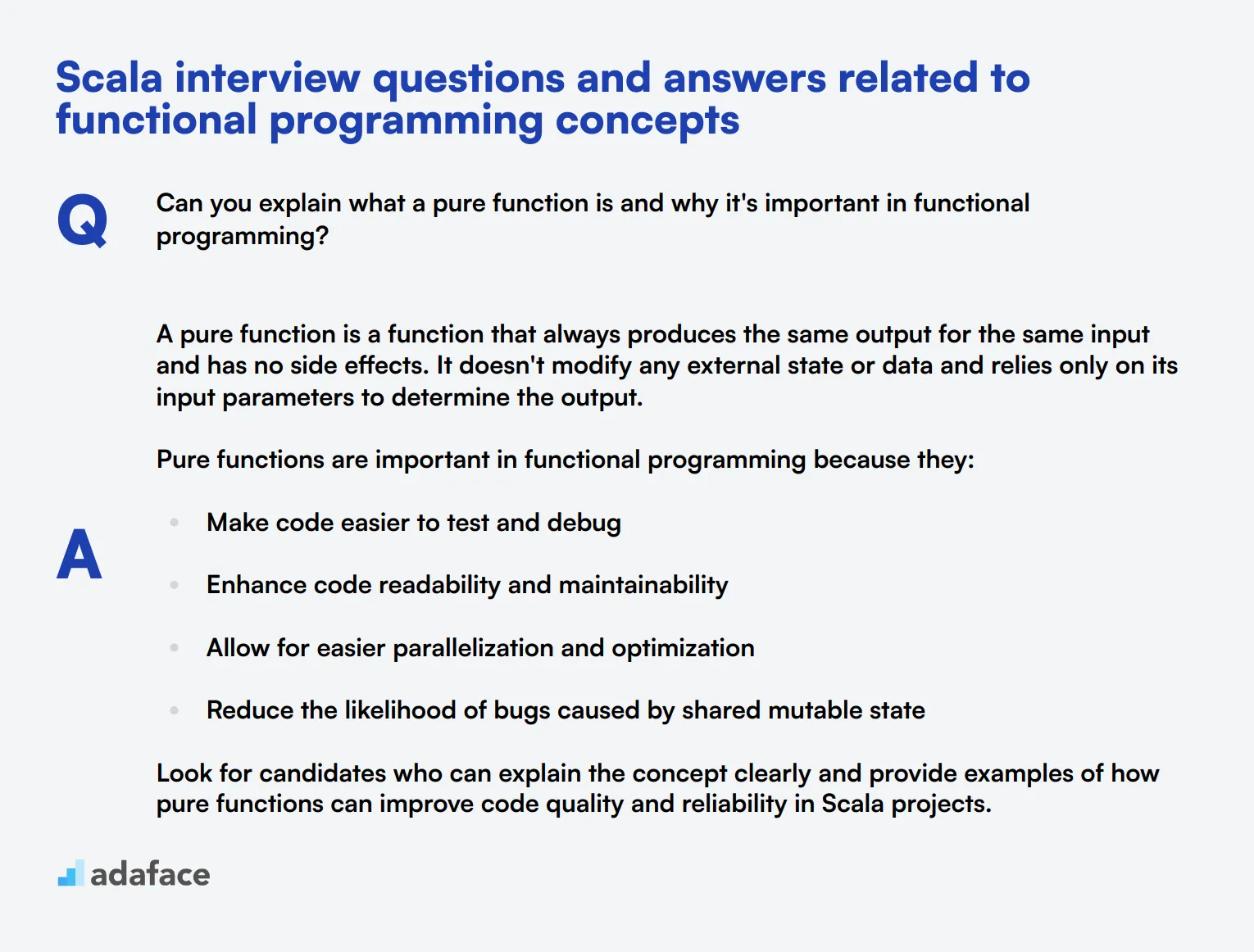
Functional programming concepts are crucial for Scala developers. These questions will help you gauge a candidate's understanding of functional paradigms and their application in Scala. Use this list to assess how well potential hires can leverage Scala's functional features to write clean, efficient code.
1. Can you explain what a pure function is and why it's important in functional programming?
A pure function is a function that always produces the same output for the same input and has no side effects. It doesn't modify any external state or data and relies only on its input parameters to determine the output.
Pure functions are important in functional programming because they:
- Make code easier to test and debug
- Enhance code readability and maintainability
- Allow for easier parallelization and optimization
- Reduce the likelihood of bugs caused by shared mutable state
Look for candidates who can explain the concept clearly and provide examples of how pure functions can improve code quality and reliability in Scala projects.
2. How does Scala support functional programming paradigms?
Scala supports functional programming paradigms through several key features:
- First-class functions: Functions can be assigned to variables, passed as arguments, and returned from other functions
- Immutable data structures: Scala encourages the use of immutable collections and variables
- Pattern matching: Allows for elegant and expressive code when working with different data types
- Lazy evaluation: Expressions are only evaluated when needed, improving performance in certain scenarios
- Tail recursion optimization: Enables efficient recursive functions without stack overflow risks
A strong candidate should be able to discuss how these features contribute to writing functional code in Scala and provide examples of when they've used them in real-world projects.
Pay attention to candidates who can explain the benefits of these features in terms of code maintainability, testability, and performance.
3. What is the difference between map and flatMap in Scala collections?
In Scala collections, map
and flatMap
are both higher-order functions used for transforming data, but they behave differently:
map
applies a given function to each element of a collection and returns a new collection of the same type with the transformed elements. It maintains the structure of the original collection.
flatMap
applies a given function that returns a collection for each element, and then flattens the resulting collections into a single collection. It's particularly useful when you want to transform elements and potentially change the structure of the collection.
Look for candidates who can provide clear examples of when to use map
vs flatMap
. They should understand that flatMap
is often used in scenarios involving nested collections or when working with monadic types like Option or Future.
4. Can you explain what a closure is in Scala and provide an example of its use?
A closure in Scala is a function that captures and carries with it the environment in which it was defined. This environment includes any variables that were in scope when the closure was created.
Closures are useful for:
- Creating functions with state
- Implementing callbacks
- Defining functions that depend on external context
An ideal candidate should be able to provide a simple example of a closure, such as a function that increments a counter defined outside its scope. They should also be able to explain how closures relate to Scala's lexical scoping rules and discuss potential pitfalls, like capturing mutable state.
5. How does Scala's for comprehension relate to functional programming concepts?
Scala's for comprehension is a syntactic sugar for working with monadic operations. It provides a more readable and expressive way to chain operations like map
, flatMap
, and filter
on collections or other monadic types.
For comprehensions relate to functional programming concepts in several ways:
- They encourage working with immutable data structures
- They promote composing functions and transformations
- They allow for expressive handling of operations that might fail or produce multiple results
A strong candidate should be able to explain how for comprehensions can be desugared into chains of map
, flatMap
, and filter
calls. They should also discuss scenarios where for comprehensions are particularly useful, such as working with Options, Futures, or complex data transformations.
6. What is function composition in Scala, and why is it useful?
Function composition in Scala is the process of combining two or more functions to create a new function. The output of one function becomes the input of the next function in the composition.
Function composition is useful because it:
- Allows for building complex operations from simpler, reusable functions
- Promotes code modularity and reusability
- Enables point-free style programming, which can lead to more concise and readable code
- Facilitates the creation of data processing pipelines
Look for candidates who can explain how to compose functions using the andThen
or compose
methods in Scala. They should also be able to discuss the benefits of function composition in terms of code organization and maintainability in larger Scala projects.
9 situational Scala interview questions with answers for hiring top developers
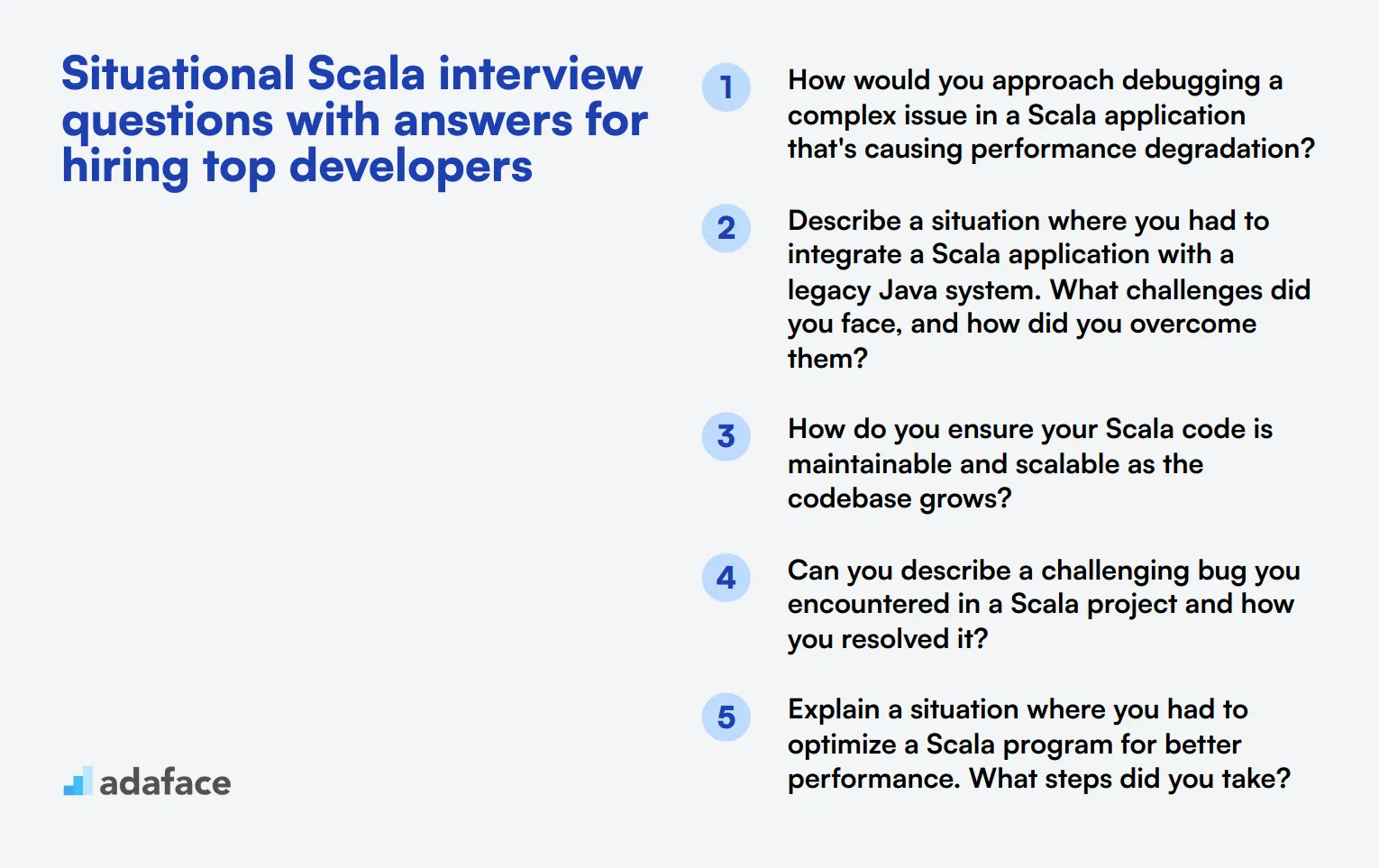
To hire top Scala developers, it's crucial to assess how they handle real-world scenarios and challenges. This list of situational Scala interview questions will help you evaluate candidates' problem-solving skills, practical experience, and ability to apply Scala concepts in various contexts.
1. How would you approach debugging a complex issue in a Scala application that's causing performance degradation?
A strong candidate will begin by identifying the issue through profiling tools and logs to understand where the performance hit is occurring. They will likely mention using tools such as JVisualVM or YourKit for profiling.
From there, they should discuss analyzing the hot spots in the code, looking for inefficient algorithms, excessive logging, or poorly optimized operations. They might also talk about reviewing memory usage and garbage collection logs.
Look for candidates who emphasize a systematic approach to debugging, including isolating the problem, reproducing it consistently, and applying incremental fixes. An ideal response will include a focus on both short-term fixes and long-term improvements.
2. Describe a situation where you had to integrate a Scala application with a legacy Java system. What challenges did you face, and how did you overcome them?
An ideal candidate should describe their experience with interoperability between Scala and Java, emphasizing the use of Scala’s ability to seamlessly call Java code.
They might mention challenges such as handling Java’s null values safely in Scala or dealing with differences in the type systems. They should discuss strategies such as using Option to wrap potential null values or using implicit conversions where necessary.
Strong answers will highlight the candidate’s ability to bridge the gap between the two languages by leveraging Scala's features to write clean, maintainable code while still interacting effectively with the existing Java codebase.
3. How do you ensure your Scala code is maintainable and scalable as the codebase grows?
Candidates should emphasize writing clean, modular code with a clear separation of concerns. They might mention using design patterns and principles like SOLID to improve maintainability.
They may also discuss the importance of writing tests, including unit and integration tests, to ensure code reliability and ease future changes. Using tools like ScalaTest or Specs2 might be mentioned.
Look for candidates who stress the importance of code reviews, continuous refactoring, and documentation. An ideal candidate will talk about proactive measures they take to ensure the code remains scalable and maintainable over time.
4. Can you describe a challenging bug you encountered in a Scala project and how you resolved it?
An effective response will detail a specific bug, how they identified it, and the steps they took to resolve it. They should mention tools and techniques used, such as logging, debugging, or profiling.
They might discuss working with colleagues to brainstorm solutions or using version control to track changes. Emphasis on root cause analysis and preventing future occurrences would be beneficial.
The candidate’s ability to communicate the problem clearly and logically walk through their resolution process is key. Look for problem-solving skills and an understanding of the importance of collaboration and thorough analysis.
5. Explain a situation where you had to optimize a Scala program for better performance. What steps did you take?
Candidates should describe the initial performance issues and how they measured the performance before and after the optimization. They might talk about using profiling tools to identify bottlenecks.
They should mention specific optimizations, such as improving algorithm efficiency, reducing memory usage, or leveraging parallelism and concurrency where appropriate.
Look for candidates who can articulate the impact of their changes and how they verified the improvements. An ideal response will show a good balance between theoretical knowledge and practical application.
6. How do you handle dependencies and library management in a Scala project, especially when working in a team?
Candidates should mention using build tools like sbt or Maven to manage dependencies. They might discuss the importance of maintaining a shared build configuration to ensure consistency across the team.
They should talk about versioning strategies, such as semantic versioning, and how they handle potential conflicts or updates to dependencies.
An ideal candidate will emphasize the importance of clear communication and documentation within the team to manage dependencies effectively. They might also discuss using tools like Nexus or Artifactory for managing internal repositories.
7. Describe a time when you had to refactor a large Scala codebase. What challenges did you face, and how did you handle them?
An effective response will outline the reasons for the refactor, such as improving code maintainability or performance. They should describe their approach, such as starting with a thorough code review and identifying areas for improvement.
They might mention using automated tests to ensure functionality is preserved and breaking the refactor into manageable pieces to reduce risk.
Look for candidates who can articulate the benefits of the refactor and how they managed the challenges, such as coordinating with team members and maintaining code stability throughout the process.
8. How do you handle data serialization and deserialization in Scala, especially when dealing with multiple data formats?
Candidates should talk about libraries and frameworks they have used, such as JSON4S, Circe, or Play JSON for JSON data, and Apache Avro or Protobuf for other data formats.
They should discuss their approach to ensuring data compatibility and integrity, such as using schema validation or versioning strategies.
An ideal candidate will emphasize the importance of thorough testing and proper error handling during serialization and deserialization processes to prevent data corruption and ensure smooth data interchange.
9. Can you describe a situation where you had to write a highly concurrent or parallel program in Scala? What were the key considerations?
Candidates should describe the specific problem and why concurrency or parallelism was necessary. They might mention using Scala’s built-in concurrency constructs such as Futures and Promises or libraries like Akka.
They should discuss key considerations such as avoiding shared mutable state, ensuring thread safety, and minimizing contention. They might talk about techniques like immutable data structures or actor-based concurrency.
Look for candidates who demonstrate a good understanding of the complexities and potential pitfalls of concurrent programming, as well as strategies for writing efficient, safe concurrent code.
Which Scala skills should you evaluate during the interview phase?
While it's impossible to gauge every aspect of a candidate's abilities in a single interview, focusing on key Scala skills can help you make a well-rounded assessment. Here, we'll outline the core competencies that interviewers should evaluate to ensure they are selecting the right talent for Scala development roles.
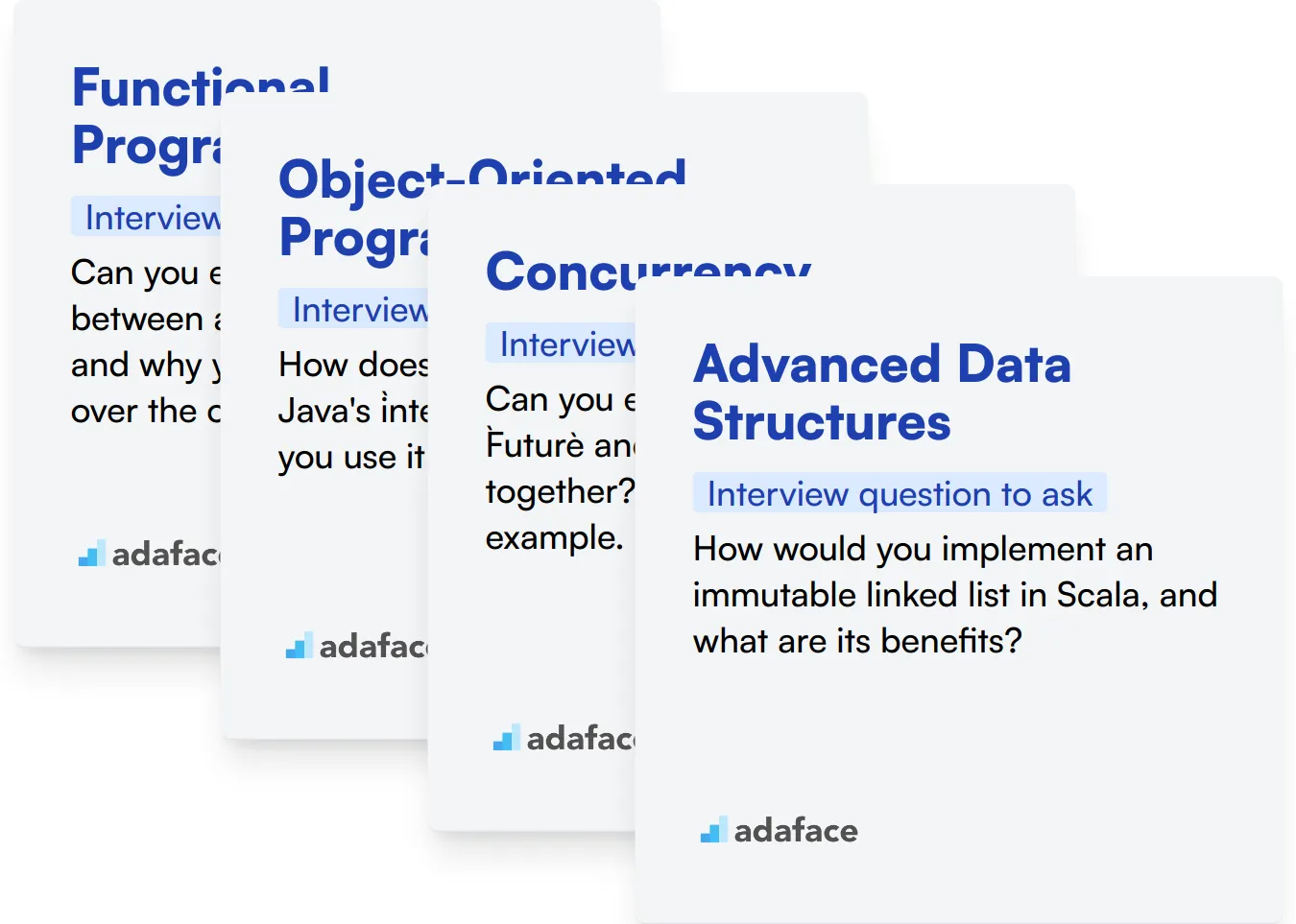
Functional Programming
Scala is renowned for its functional programming features which enable developers to write concise and expressive code. Understanding functional programming concepts like immutability, first-class functions, and higher-order functions is essential for writing effective Scala code.
To assess this skill, consider using a Scala functional programming test that includes multiple-choice questions tailored to gauge the candidate's understanding of these concepts.
You can also ask targeted interview questions to evaluate this skill in-depth.
Can you explain the difference between a val
and a var
in Scala and why you might choose one over the other?
Look for candidates who not only know that val
is immutable (cannot be changed) while var
is mutable (can be changed) but also understand the implications of immutability for functional programming and concurrency.
Object-Oriented Programming (OOP)
Scala seamlessly integrates object-oriented programming principles with functional programming. Mastery of OOP concepts like inheritance, polymorphism, and encapsulation is vital for designing robust Scala applications.
An Object Oriented Programming test can help identify candidates who have a solid grasp of these foundational concepts.
To dig deeper, you might ask specific questions related to OOP in Scala.
How does Scala's trait
differ from Java's interface
, and how would you use it in a practical scenario?
Candidates should demonstrate an understanding that traits
in Scala can contain both abstract and concrete methods, unlike Java interfaces, and discuss practical use cases for traits in code reuse and mixin composition.
Concurrency
Concurrency is crucial for developing high-performance Scala applications, especially in a multi-threaded environment. Scala's advanced concurrency tools like futures, promises, and actors are essential for building responsive and scalable systems.
Consider utilizing a Scala concurrency test that includes relevant multiple-choice questions to screen for this skill.
You can further examine this skill through well-crafted interview questions.
Can you explain how Scala's Future
and Promise
work together? Provide a simple example.
Look for explanations that cover the basics of asynchronous computation, showing a clear understanding of how Future
represents a value that may not be available yet, while Promise
is used to complete a Future
.
Advanced Data Structures
Knowledge of advanced data structures is pivotal for optimizing performance and solving complex problems efficiently in Scala. Understanding collections like lists, sets, maps, and their immutable counterparts can significantly impact the quality of code.
A Data Structures test can help you filter candidates with strong proficiency in this area.
Assessing this skill can also be done through pointed interview questions.
How would you implement an immutable linked list in Scala, and what are its benefits?
Candidates should articulate the construction of an immutable linked list, emphasizing benefits like thread-safety and historical data preservation, which are hallmarks of functional programming.
3 Tips for Effectively Using Scala Interview Questions
Before you start applying what you've learned about Scala interview questions, here are some tips to enhance your candidate evaluation process.
1. Incorporate Skills Tests Prior to Interviews
To accurately assess a candidate's technical abilities, using skills tests before interviews is essential. This approach helps identify candidates who possess the necessary programming skills for Scala, allowing you to focus on those who are best suited for the role.
Consider using the Scala online test for evaluating coding skills, or even tests related to functional programming concepts. These tests reveal candidates' practical abilities and problem-solving skills in realistic scenarios.
Implementing these tests in your hiring process not only saves time but also strengthens your interview by ensuring you engage with well-qualified candidates. This sets a solid foundation for the next step: preparing targeted interview questions.
2. Compile Relevant Interview Questions
When conducting interviews, time is limited. Therefore, it's critical to select a concise set of questions that effectively evaluate the most relevant skills and attributes.
Beyond Scala-specific questions, consider integrating inquiries related to soft skills like communication and teamwork. Questions about react or data structures can also provide valuable insights into a candidate's fit for your organization.
Focus on quality over quantity when compiling your list of questions. Prioritizing key areas increases your chances of identifying the best candidates who match the role.
3. Ask Follow-Up Questions
Relying solely on initial interview questions may not provide a complete picture of a candidate's capabilities. Follow-up questions are essential for uncovering deeper insights and addressing potential inconsistencies or shallow responses.
For instance, if a candidate states they have experience with Scala's pattern matching, a suitable follow-up could be, "Can you provide an example where you utilized pattern matching to solve a problem?" This not only probes their understanding but also allows you to gauge their practical experience.
Hire top Scala developers with targeted interview questions and skills tests
Looking to hire someone with Scala skills? Make sure you assess their abilities accurately. The most effective way to do this is by using skill tests. Consider using our Scala online test to evaluate candidates' proficiency.
After using the test to shortlist the best applicants, you can invite them for interviews. To streamline your hiring process and find the most qualified Scala developers, check out our online assessment platform for a comprehensive solution.
Scala Online Test
Download Scala interview questions template in multiple formats
Scala Interview Questions FAQs
Include a mix of basic concepts, functional programming, and situational questions to assess both theoretical knowledge and practical skills.
Focus on fundamental Scala concepts and simple coding problems to gauge their understanding of the language basics.
Cover topics like immutability, higher-order functions, pattern matching, and recursion to assess functional programming knowledge.
Present real-world scenarios to evaluate problem-solving skills and how candidates apply Scala concepts in practical situations.
Yes, combining coding tests with interview questions provides a more complete assessment of a candidate's Scala skills.
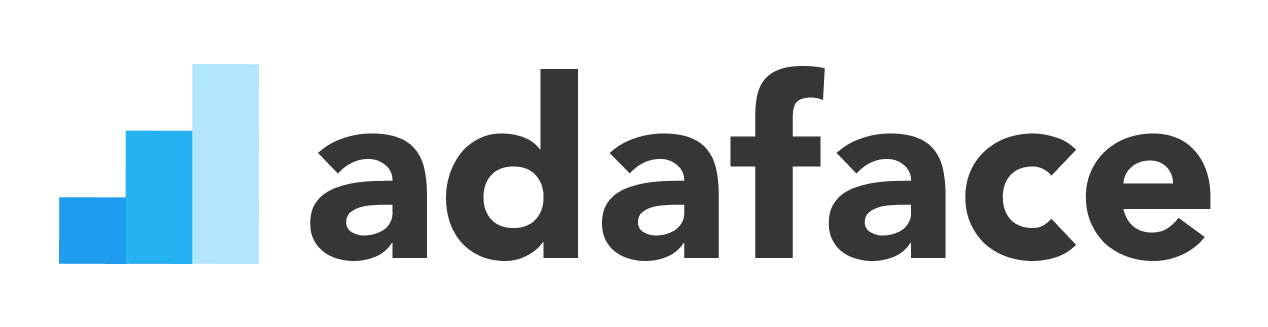
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
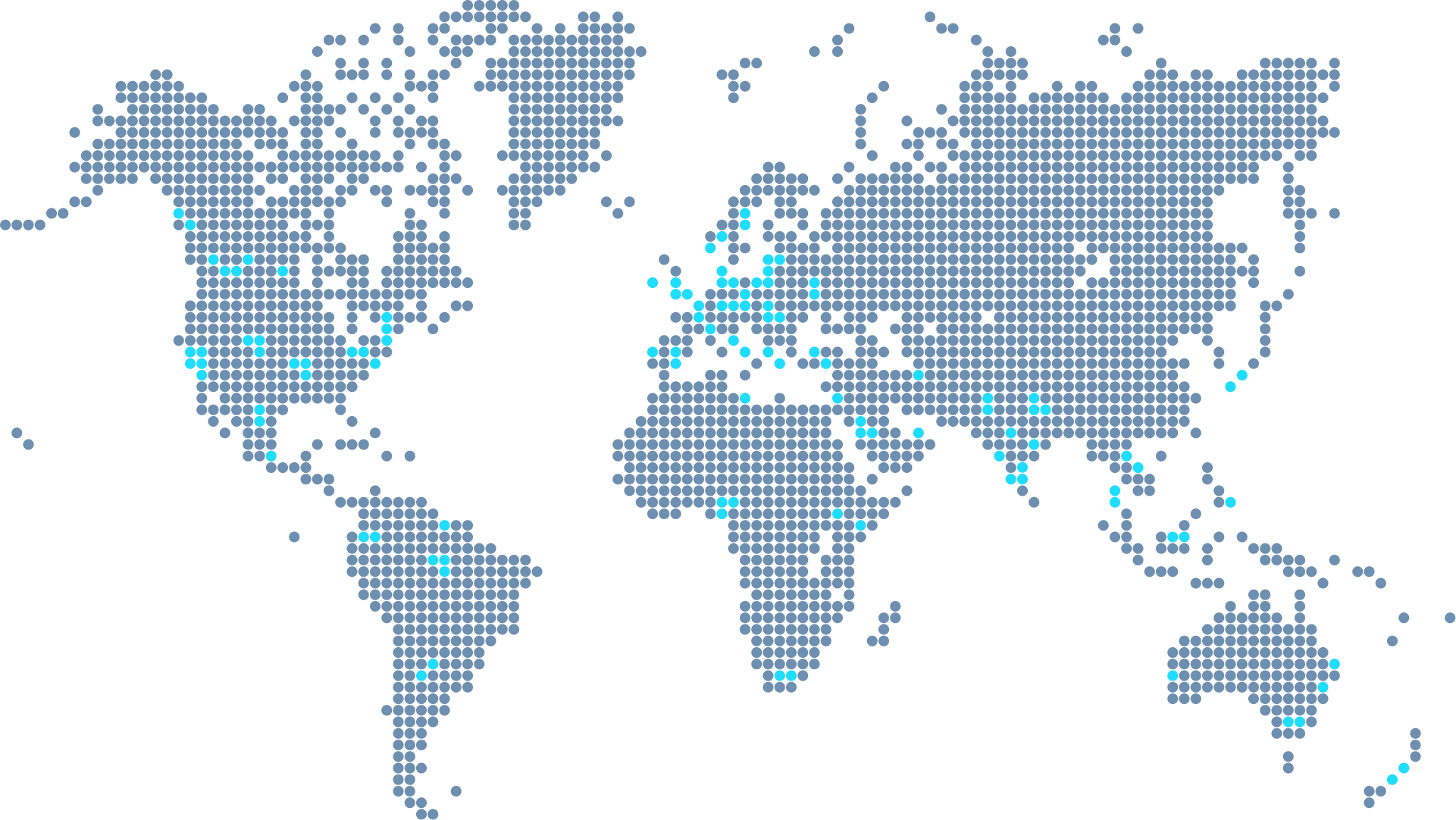
