As a recruiter or hiring manager, finding the right software developers for your team is a critical task that can significantly impact your project's success. Asking the right interview questions helps you evaluate candidates' technical skills, problem-solving abilities, and cultural fit effectively.
This comprehensive guide provides a curated list of software development interview questions tailored for different experience levels and areas of expertise. From common questions for all applicants to advanced queries for senior developers, we cover a wide range of topics including technical knowledge, processes, and situational scenarios.
By using these questions, you'll be better equipped to identify top talent and make informed hiring decisions. Consider complementing your interview process with pre-employment assessments to gain a more holistic view of candidates' capabilities.
Table of contents
10 common Software Development interview questions to ask your applicants
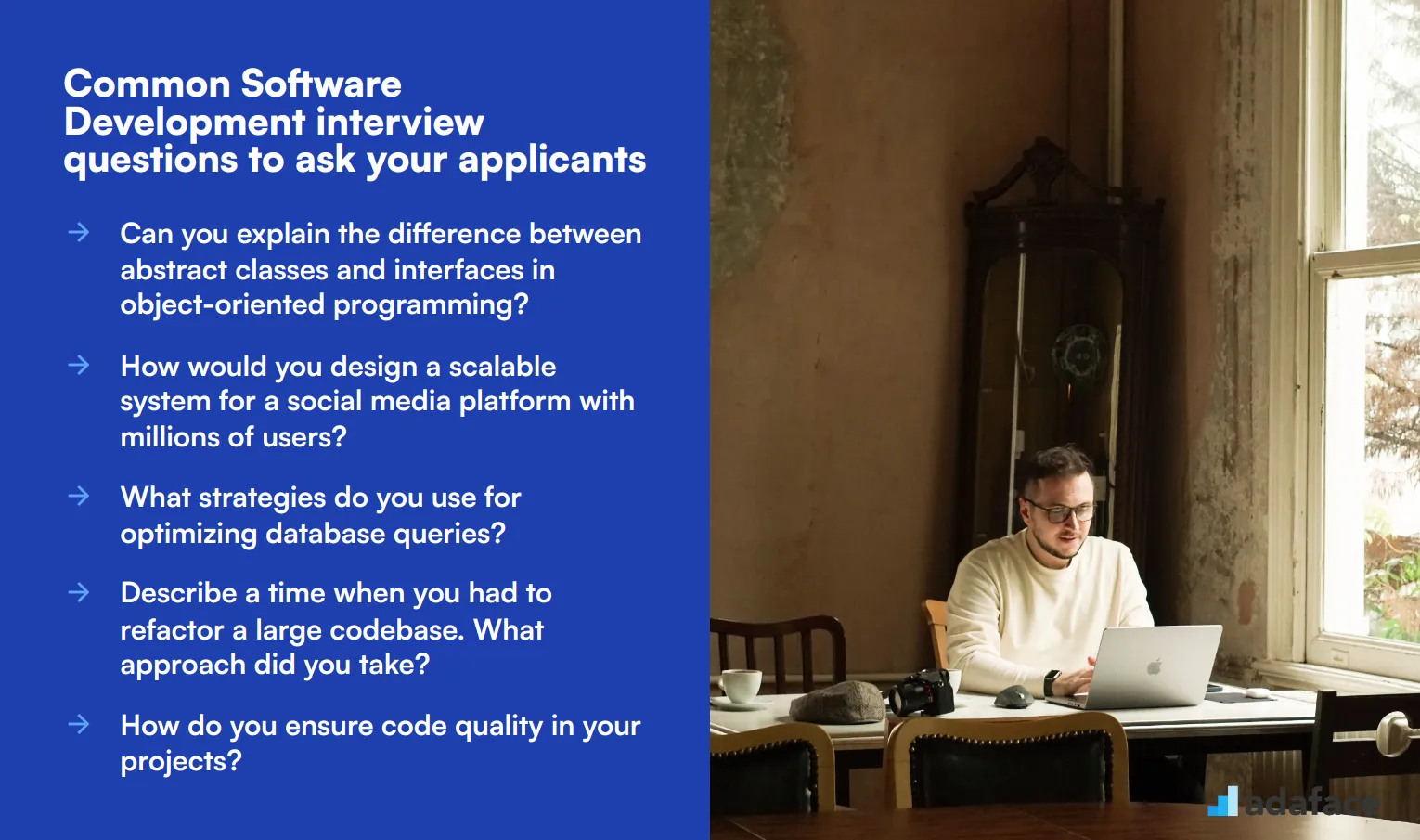
To assess the technical skills and problem-solving abilities of software developer candidates, use these carefully curated interview questions. These questions will help you evaluate a candidate's coding knowledge, system design understanding, and overall fit for your development team.
- Can you explain the difference between abstract classes and interfaces in object-oriented programming?
- How would you design a scalable system for a social media platform with millions of users?
- What strategies do you use for optimizing database queries?
- Describe a time when you had to refactor a large codebase. What approach did you take?
- How do you ensure code quality in your projects?
- Explain the concept of dependency injection and its benefits.
- What's your experience with version control systems? How do you handle merge conflicts?
- How would you implement a caching mechanism to improve application performance?
- Describe your approach to writing unit tests and ensuring code coverage.
- What security considerations do you keep in mind when developing web applications?
8 Software Development interview questions and answers to evaluate junior developers
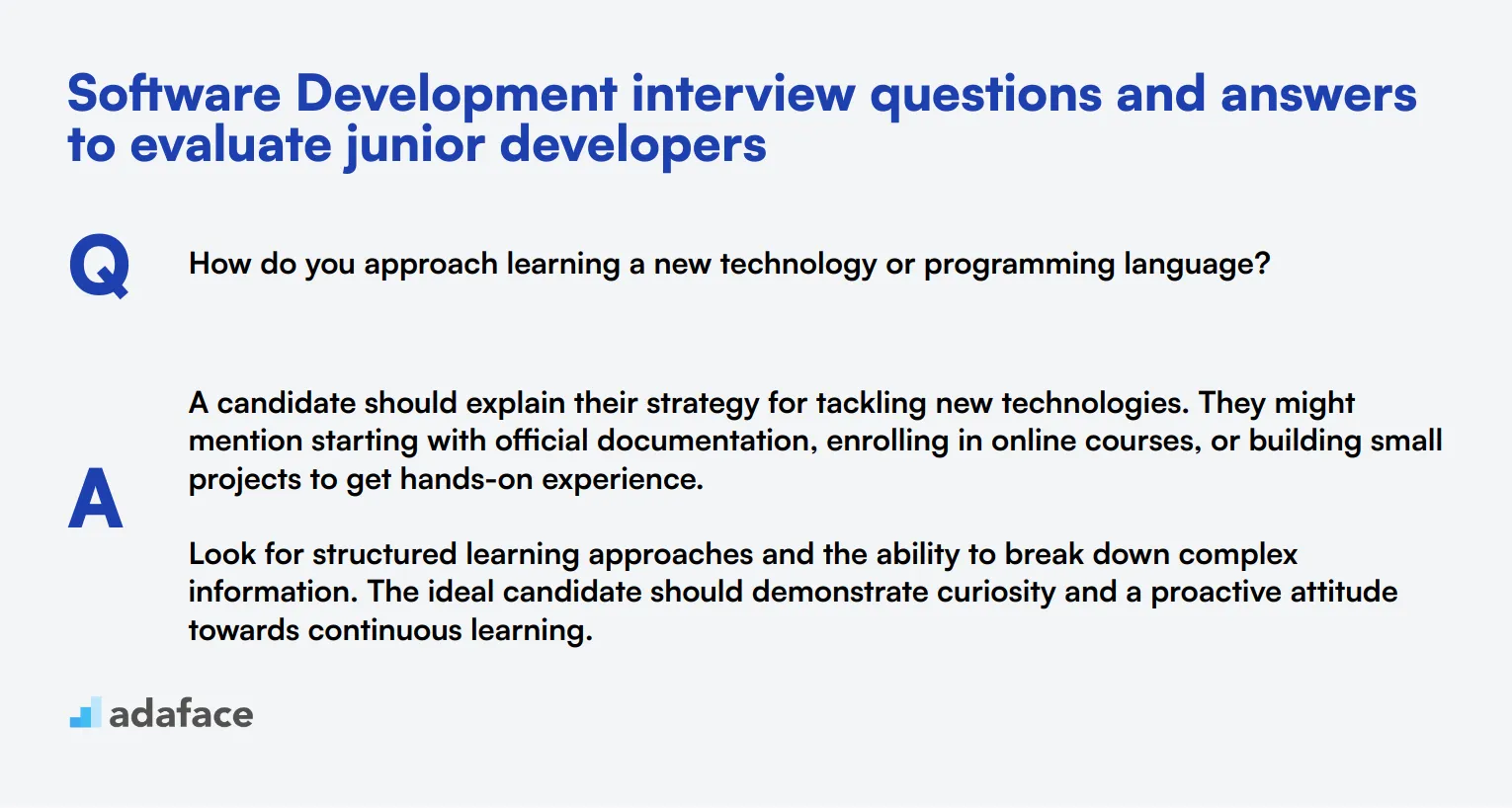
To evaluate whether junior software developers have the foundational skills and thought processes needed for your team, consider using these 8 interview questions. They are designed to help you gauge both technical understanding and problem-solving abilities, essential for any budding developer.
1. How do you approach learning a new technology or programming language?
A candidate should explain their strategy for tackling new technologies. They might mention starting with official documentation, enrolling in online courses, or building small projects to get hands-on experience.
Look for structured learning approaches and the ability to break down complex information. The ideal candidate should demonstrate curiosity and a proactive attitude towards continuous learning.
2. Can you describe a recent project you worked on? What challenges did you face and how did you overcome them?
The candidate should walk you through a specific project, detailing their role, the technologies used, and any hurdles they encountered. How they navigated these challenges will give you insight into their problem-solving abilities.
Look for clear communication and logical problem-solving steps. An ideal answer will reflect their resilience and ability to learn from difficult situations.
3. How do you prioritize tasks when working on a project?
Candidates should describe their method for managing tasks, such as using task management tools or following Agile methodologies. They might mention breaking down tasks into smaller, manageable parts and prioritizing based on deadlines or impact.
Look for organized and methodical approaches. The candidate should also demonstrate an understanding of balancing urgency with importance.
4. How do you handle constructive criticism on your code?
A strong candidate should be open to receiving feedback and view it as an opportunity to improve. They might describe how they actively seek feedback through code reviews or pair programming.
Look for a positive attitude towards feedback and examples of how they've implemented suggestions to enhance their code. This shows maturity and a growth mindset.
5. Can you explain the importance of version control in software development?
Version control systems, like Git, are essential for tracking changes, collaborating with team members, and maintaining a history of project development. It helps in managing codebase versions and resolving conflicts.
Candidates should demonstrate an understanding of version control's role in team collaboration and project management. They should ideally mention specific tools and practices they follow.
6. How do you stay updated with the latest trends and technologies in software development?
The candidate might mention following industry blogs, attending webinars, participating in developer communities, or contributing to open-source projects. Staying informed about skills required for Java developers can also be a part of their strategy.
Look for active engagement in the tech community and a genuine interest in continuous learning. This indicates they are proactive in keeping their skills relevant.
7. What is your process for debugging a complex issue in your code?
Candidates should explain their systematic approach to debugging, such as isolating the problem, using debugging tools, and testing potential solutions incrementally. They might also mention documenting the process for future reference.
Look for logical thinking and a clear, step-by-step debugging strategy. The ability to stay calm and focused under pressure is also a valuable trait.
8. How do you ensure your code is maintainable and scalable?
A candidate might discuss writing clean, modular code, following best practices, and conducting thorough code reviews. They should also mention the importance of documentation and tests.
Look for an understanding of writing code that others can easily read and modify. The ideal response should reflect a balance between immediate functionality and long-term maintainability.
15 intermediate Software Development interview questions and answers to ask mid-tier developers.
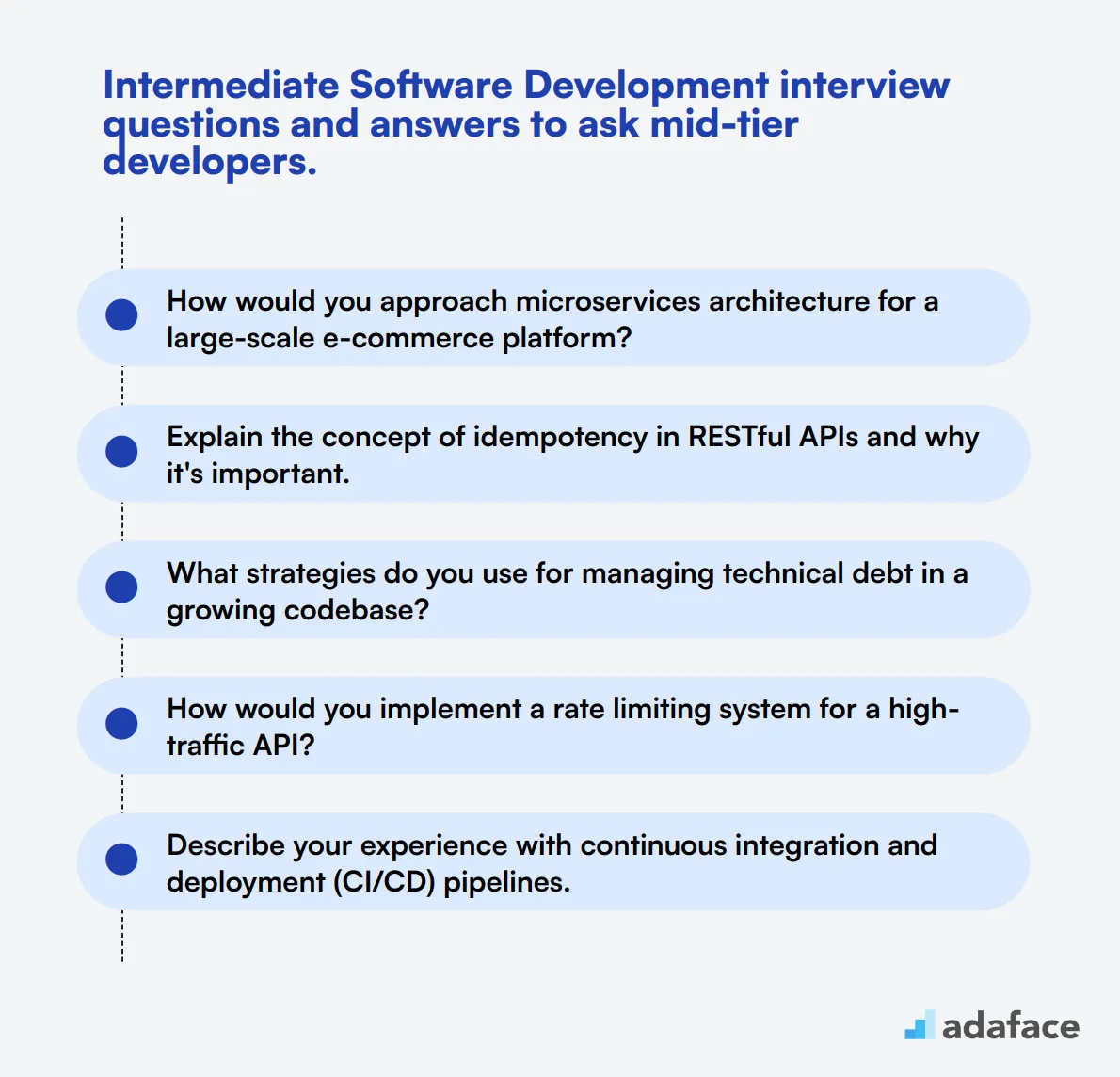
To effectively assess mid-tier software developers, use these 15 intermediate interview questions. These questions are designed to evaluate technical depth, problem-solving skills, and real-world application of software development concepts. Use them to gain insights into a candidate's experience and thought process.
- How would you approach microservices architecture for a large-scale e-commerce platform?
- Explain the concept of idempotency in RESTful APIs and why it's important.
- What strategies do you use for managing technical debt in a growing codebase?
- How would you implement a rate limiting system for a high-traffic API?
- Describe your experience with continuous integration and deployment (CI/CD) pipelines.
- How do you handle data consistency in a distributed system?
- Explain the differences between monolithic, microservices, and serverless architectures.
- What's your approach to implementing authentication and authorization in a web application?
- How would you design a system to handle real-time updates for a collaborative editing tool?
- Describe your experience with containerization technologies like Docker.
- How do you approach performance optimization in a web application?
- What strategies do you use for error handling and logging in a production environment?
- How would you design a scalable notification system for a mobile app with millions of users?
- Explain the concept of eventual consistency in distributed systems.
- How do you approach designing and implementing APIs for third-party integrations?
7 advanced Software Development interview questions and answers to evaluate senior developers
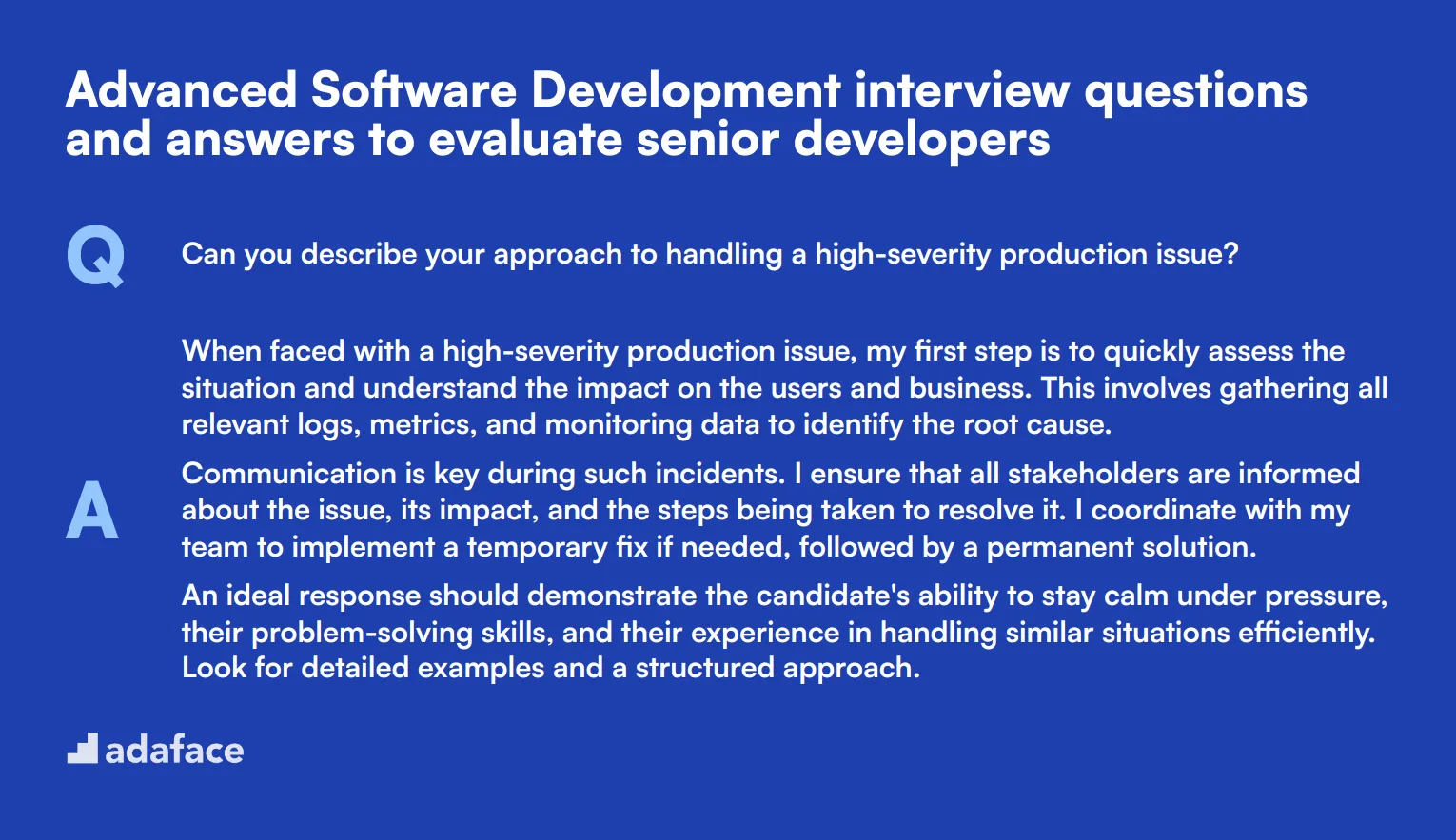
To evaluate senior developers effectively, it's crucial to ask the right advanced questions. This list will help you assess candidates' deeper understanding of software development principles, their problem-solving abilities, and their strategic thinking. Use these questions to uncover insights that go beyond technical skills and highlight their experience and thought processes.
1. Can you describe your approach to handling a high-severity production issue?
When faced with a high-severity production issue, my first step is to quickly assess the situation and understand the impact on the users and business. This involves gathering all relevant logs, metrics, and monitoring data to identify the root cause.
Communication is key during such incidents. I ensure that all stakeholders are informed about the issue, its impact, and the steps being taken to resolve it. I coordinate with my team to implement a temporary fix if needed, followed by a permanent solution.
An ideal response should demonstrate the candidate's ability to stay calm under pressure, their problem-solving skills, and their experience in handling similar situations efficiently. Look for detailed examples and a structured approach.
2. How do you approach designing a fault-tolerant system?
Designing a fault-tolerant system involves ensuring that the system can continue operating properly even when one or more of its components fail. I start by identifying potential points of failure and implementing redundancy for critical components.
I use techniques like load balancing, data replication, and automated failover mechanisms to ensure high availability. Monitoring and alerting systems are also crucial to detect issues early and respond promptly.
Look for candidates who emphasize proactive planning and robustness in their designs. They should mention specific strategies and tools they use to achieve fault tolerance and provide examples from their past experience.
3. What is your approach to mentoring junior developers?
Mentoring junior developers involves a balance of guidance, support, and challenge. I start by understanding their current skill level and career goals. From there, I provide them with learning resources, pair programming sessions, and code reviews.
I also encourage them to take ownership of small projects to build their confidence. Regular feedback and open lines of communication are crucial to address their questions and concerns promptly.
A strong candidate should demonstrate patience, empathy, and effective communication skills. They should provide examples of successful mentorship relationships and discuss how they tailor their approach to individual needs.
4. How do you handle disagreements with other team members on technical decisions?
Disagreements on technical decisions are common and can be healthy for the team's growth. My approach is to focus on the problem, not the person. I listen to the other person's perspective and ensure I understand their reasoning.
I present my viewpoint with supporting evidence and data. If we still disagree, I suggest a compromise or a proof-of-concept to test both approaches. The goal is to find the best solution for the project, not to win the argument.
Look for candidates who value collaboration and respect. They should demonstrate an ability to handle conflicts constructively and prioritize the team's success over their ego.
5. Can you describe a scenario where you had to balance technical debt with new feature development?
Balancing technical debt with new feature development is a common challenge. In one scenario, we had accumulated significant technical debt that was slowing down our development process. I proposed a plan to address the most critical issues while continuing to deliver new features.
We allocated a portion of each sprint to tackle technical debt and tracked our progress. This approach allowed us to improve the codebase's health without sacrificing the product roadmap.
An ideal candidate should showcase their ability to prioritize effectively and communicate the importance of technical debt management. They should provide specific examples and discuss the outcomes of their approach.
6. What strategies do you use for ensuring high availability in distributed systems?
Ensuring high availability in distributed systems involves multiple strategies. I use redundancy and replication to eliminate single points of failure. Load balancing helps distribute traffic evenly across servers, preventing any one server from becoming a bottleneck.
Automated failover mechanisms ensure that if one component fails, another can take its place seamlessly. Continuous monitoring and alerting systems are also crucial to detect issues early and respond promptly.
Candidates should demonstrate a deep understanding of high availability principles. Look for specific techniques and tools they have used and how they have implemented these strategies in past projects.
7. How do you stay motivated and productive during long projects with multiple challenges?
Staying motivated during long projects involves setting clear, achievable goals and celebrating small wins along the way. I break the project into smaller tasks and create a roadmap to track progress.
I also ensure regular communication with the team to share updates and address any challenges collectively. Maintaining a healthy work-life balance is crucial to avoid burnout and stay productive.
Look for candidates who demonstrate resilience and a proactive approach to maintaining motivation. They should provide examples of long projects they have completed and discuss how they managed to stay focused and productive.
12 Software Development interview questions about processes and tasks
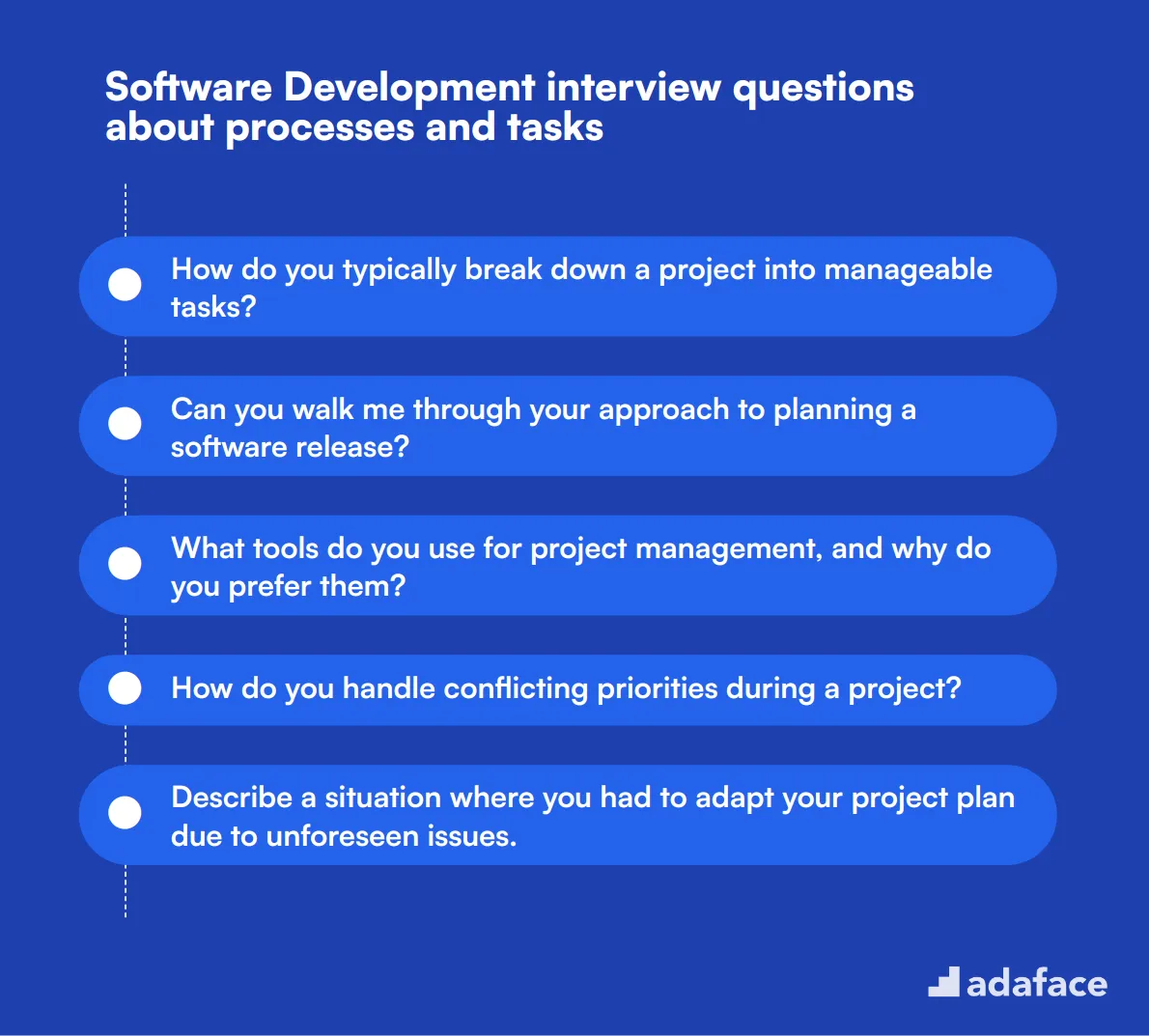
To effectively assess candidates' understanding of software development processes, use these tailored questions during interviews. They will help you gauge how well applicants can manage tasks in dynamic environments and ensure project success. For a comprehensive view of required skills, refer to our software developer job description.
- How do you typically break down a project into manageable tasks?
- Can you walk me through your approach to planning a software release?
- What tools do you use for project management, and why do you prefer them?
- How do you handle conflicting priorities during a project?
- Describe a situation where you had to adapt your project plan due to unforeseen issues.
- What is your process for gathering and incorporating feedback from stakeholders?
- How do you ensure effective communication within your development team during a project?
- What strategies do you employ to manage scope creep in software development?
- Can you explain your approach to estimating time and resources for a project?
- How do you balance quality assurance and timely delivery in software projects?
- What metrics do you use to measure the success of a development process?
- How do you foster collaboration among team members during a project?
14 Software Development interview questions about technical knowledge and definitions
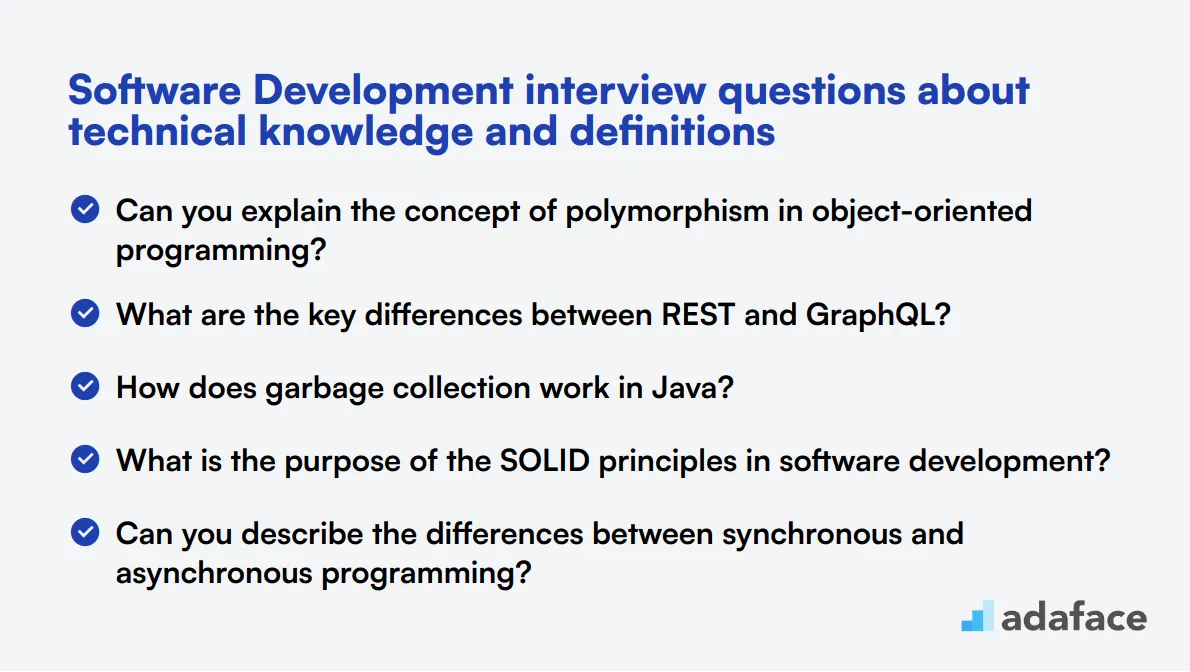
To assess the depth of your candidates' technical knowledge, these 14 software development interview questions will help you identify their understanding of key concepts and definitions. Use these questions to gauge their expertise and ensure they are well-versed in the fundamentals of software development. For more details, you can refer to this software developer job description.
- Can you explain the concept of polymorphism in object-oriented programming?
- What are the key differences between REST and GraphQL?
- How does garbage collection work in Java?
- What is the purpose of the SOLID principles in software development?
- Can you describe the differences between synchronous and asynchronous programming?
- How does a blockchain work, and what are its primary components?
- What is the difference between statically typed and dynamically typed languages?
- Can you explain what a 'race condition' is and how to prevent it?
- What are design patterns and why are they important in software development?
- How does a load balancer work in a web application architecture?
- Can you explain the concept of 'big O notation' in algorithm analysis?
- What is the difference between continuous integration and continuous deployment?
- Can you describe how a distributed system achieves consensus?
- What is the purpose of a message queue and how is it used in software systems?
10 situational Software Development interview questions for hiring top developers
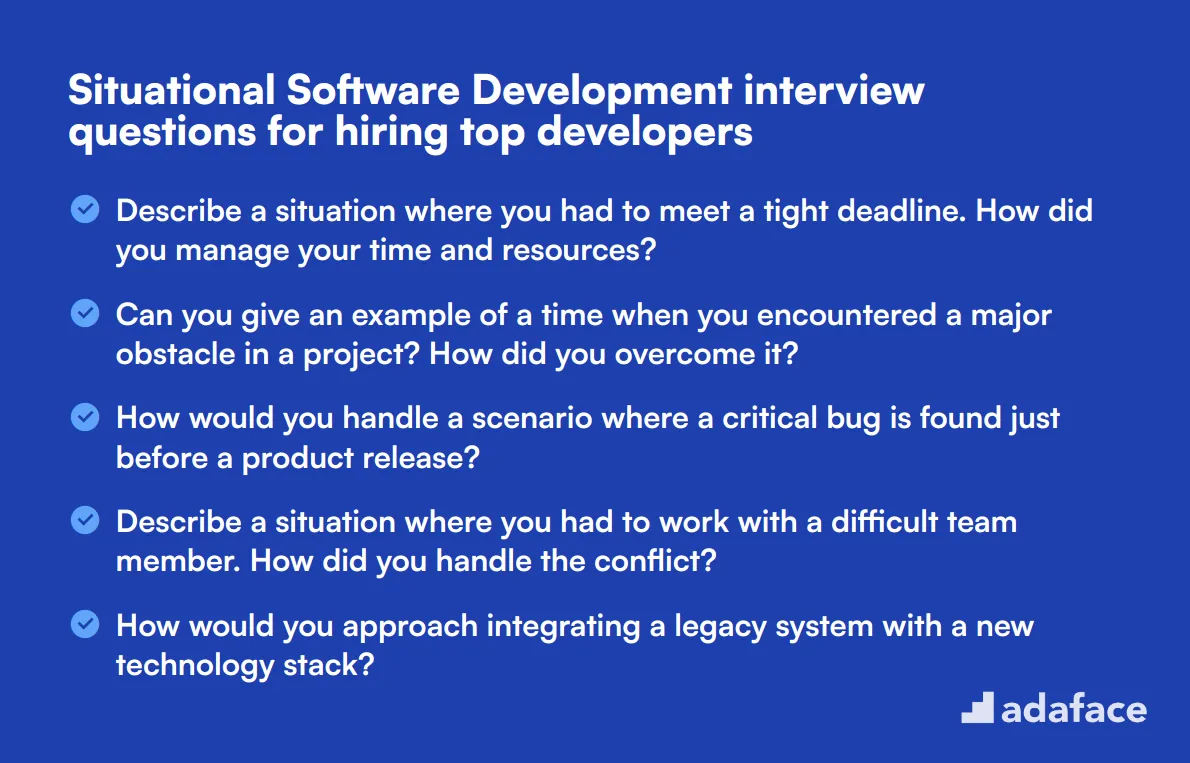
To identify top-performing developers, situational questions can provide valuable insights into their problem-solving and decision-making abilities. Use this list of carefully curated situational software development interview questions to evaluate how candidates handle real-world challenges and ensure they align with your team's needs. For more details, refer to this software developer job description.
- Describe a situation where you had to meet a tight deadline. How did you manage your time and resources?
- Can you give an example of a time when you encountered a major obstacle in a project? How did you overcome it?
- How would you handle a scenario where a critical bug is found just before a product release?
- Describe a situation where you had to work with a difficult team member. How did you handle the conflict?
- How would you approach integrating a legacy system with a new technology stack?
- Tell me about a time when you had to quickly learn a new technology or tool to complete a project. How did you go about it?
- How would you handle a situation where a stakeholder requests a significant change late in the development cycle?
- Describe a time when you had to make a critical technical decision with incomplete information. How did you proceed?
- Can you provide an example of how you ensured effective communication within a dispersed (remote) team during a project?
- How would you handle a situation where your project is falling behind schedule due to unforeseen technical challenges?
Which Software Development skills should you evaluate during the interview phase?
Evaluating a candidate's skills in software development during an interview can be challenging, as it is impossible to cover every facet of their proficiency in one sitting. However, focusing on a few core skills can provide significant insights into their capability to succeed in the role.
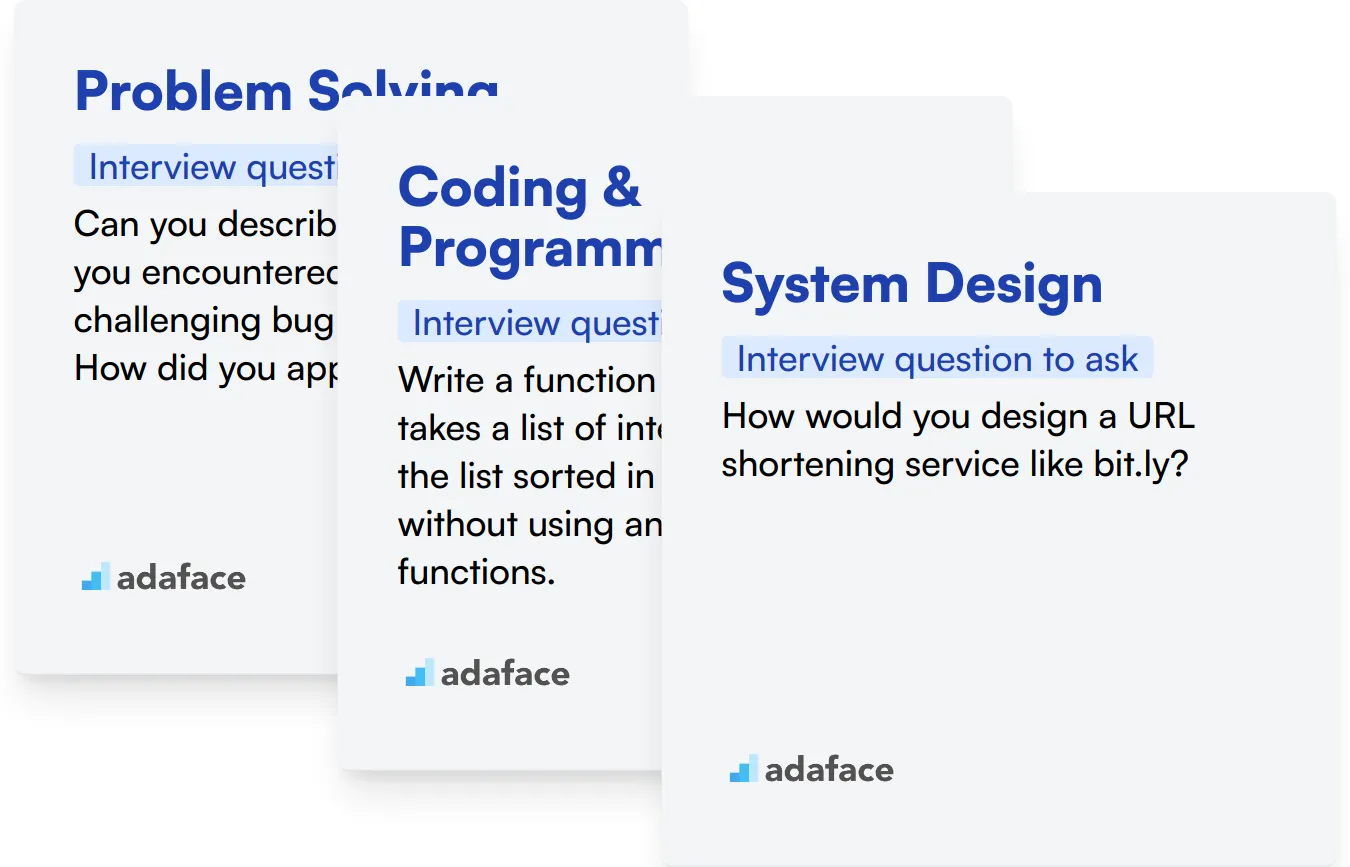
Problem Solving
Problem-solving is essential in software development as it directly impacts how developers approach, diagnose, and resolve issues within the code. It reflects their analytical thinking and ability to overcome challenges.
You can use an assessment test that asks relevant MCQs to filter out this skill. For instance, our technical-quantitative-aptitude-assessment-test includes problem-solving questions.
You can also try asking targeted interview questions specifically to judge this subskill. One useful question is:
Can you describe a time when you encountered a particularly challenging bug in your code? How did you approach solving it?
When asking this question, look out for how the candidate breaks down the problem, their logical approach to troubleshooting, and the methods they used to implement a solution.
Coding & Programming
Proficiency in coding and programming is fundamental for any software developer. It determines their ability to write efficient, clean, and maintainable code, which is crucial for project success.
To evaluate this skill, you can use our computer-programmer-aptitude-test that covers various coding challenges and questions.
You can also assess this skill by asking questions that require coding solutions. An example question is:
Write a function in Python that takes a list of integers and returns the list sorted in ascending order without using any built-in sort functions.
Focus on the candidate’s understanding of algorithms, their ability to write syntactically correct code, and how they optimize their solution for performance and readability.
System Design
System design skills are important for developers, particularly those at senior levels, as they need to architect scalable, efficient, and reliable systems. It demonstrates their ability to think at a high level and design comprehensive solutions.
System design can be evaluated using our software-system-design-online-test, which includes relevant MCQs.
To further assess this skill, ask questions that require them to design a system. A good question is:
How would you design a URL shortening service like bit.ly?
Look for the candidate’s ability to discuss different components of the system, their approach to handling scalability, potential bottlenecks, and how they ensure reliability and performance.
Streamline Your Hiring with Adaface Skill Tests and Targeted Interview Questions
When seeking to hire professionals with specific skills, verifying their proficiency accurately is key. This ensures you bring on board candidates who can genuinely meet the technical demands of the role.
The most direct method to assess these skills is through specialized tests. Adaface offers a variety of skill assessments tailored to gauge expertise in areas such as JavaScript, Python, and Java, ensuring you measure what matters.
Utilizing these tests allows you to efficiently screen and shortlist the top candidates. This preliminary filtering ensures that only the most promising applicants are invited for in-depth interviews, optimizing the hiring process.
To initiate the screening process and find the best matches for your team, consider signing up on Adaface. Start by exploring our assessments library or learn more about our hiring solutions.
Data Structures Test
Download Software Development interview questions template in multiple formats
Software Development Interview Questions FAQs
This post covers common, junior, intermediate, advanced, process-related, technical, and situational Software Development interview questions.
The post includes 8 interview questions and answers specifically designed to evaluate junior developers.
Yes, the post provides 7 advanced Software Development interview questions and answers to evaluate senior developers.
Absolutely. There are 14 Software Development interview questions focusing on technical knowledge and definitions.
These questions can help assess candidates' skills, knowledge, and problem-solving abilities across various levels of experience in Software Development.
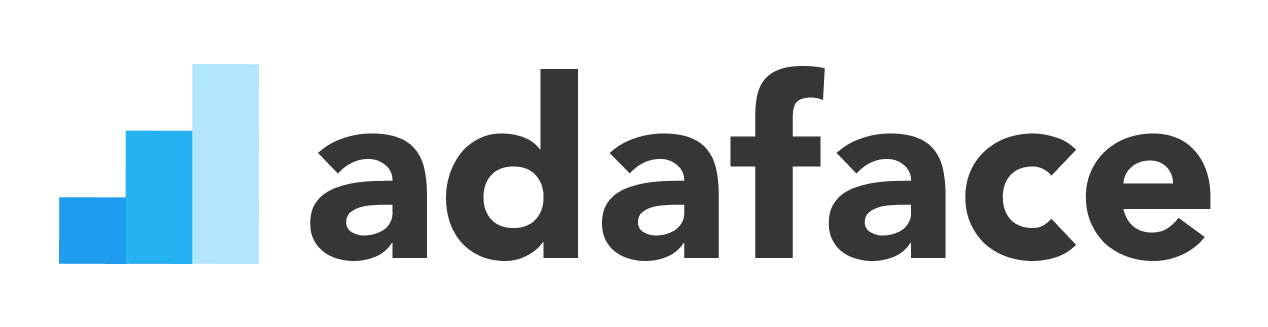
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
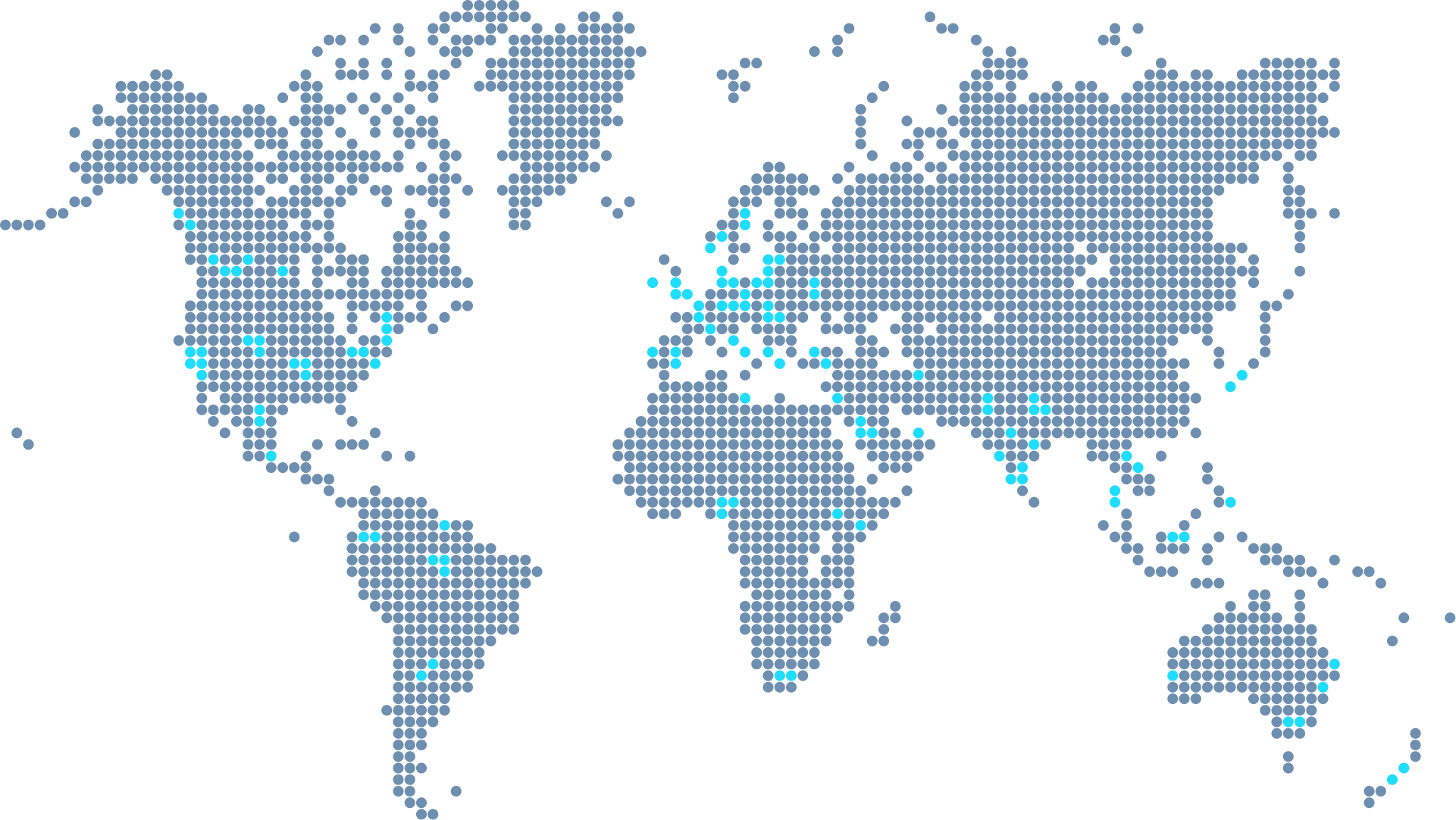
