When hiring developers with JUnit expertise, having a list of well-crafted interview questions is crucial. This helps identify candidates who not only have technical skills but also understand best practices in unit testing and Java development.
This blog post provides a structured set of questions to efficiently assess JUnit knowledge at various levels of experience, from junior to senior developers. Additionally, it includes specific queries related to unit testing techniques and test annotations.
Using this comprehensive list, you can effectively evaluate candidates and ensure you're making informed hiring decisions. To further streamline your assessment process, consider utilizing an online Java test before conducting interviews.
Table of contents
8 general Junit interview questions and answers to assess applicants
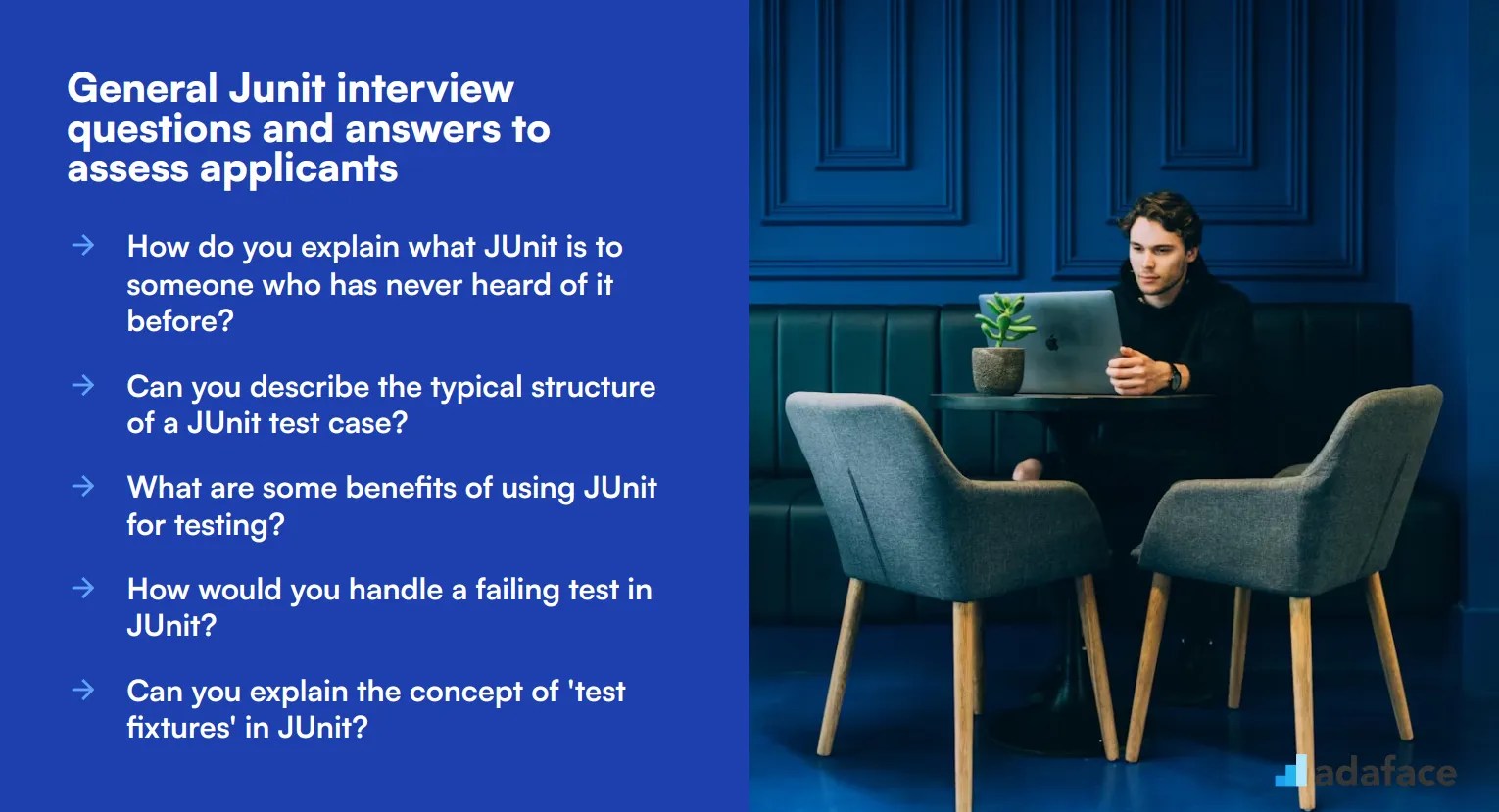
To determine if your candidates have a solid grasp of JUnit testing, use these eight interview questions and answers. This list will help you evaluate their practical knowledge and problem-solving skills, ensuring they can efficiently handle testing tasks in your projects.
1. How do you explain what JUnit is to someone who has never heard of it before?
JUnit is a popular framework used in Java for unit testing. It allows developers to write and run repeatable automated tests to ensure code works as expected. It simplifies the process of testing individual units of source code.
Look for candidates who can explain JUnit in simple terms, showing they understand its purpose and importance in software development. Strong answers will highlight the benefits of automated testing and its role in maintaining code quality.
2. Can you describe the typical structure of a JUnit test case?
A typical JUnit test case includes a setup method to prepare the test environment, one or more test methods to execute the code being tested, and a teardown method to clean up after tests. These methods are usually annotated with @Before, @Test, and @After respectively.
Candidates should demonstrate knowledge of the JUnit lifecycle and the importance of each phase in a test case. Ideal responses will also mention the use of assertions to verify test results.
3. What are some benefits of using JUnit for testing?
JUnit offers several benefits, including the ability to automate testing, which saves time and ensures consistency. It also provides immediate feedback on code quality, helps catch bugs early, and supports test-driven development (TDD).
Strong candidates will be able to articulate how JUnit fits into the larger software development lifecycle and its role in promoting good testing practices. Look for answers that mention specific advantages like reducing the risk of regression and improving code maintainability.
4. How would you handle a failing test in JUnit?
When a test fails, the first step is to review the test case and the code under test to identify the cause of failure. It could be due to a bug in the code, an issue with the test itself, or an external factor like incorrect test data. Debugging tools and logging can help pinpoint the problem.
Candidates should demonstrate a methodical approach to troubleshooting and fixing failing tests. Look for answers that emphasize the importance of understanding the root cause and ensuring the test accurately reflects the desired behavior.
5. Can you explain the concept of 'test fixtures' in JUnit?
A test fixture in JUnit refers to the setup of the testing environment, including the initialization of objects and resources needed for tests. This setup ensures that tests run in a consistent and controlled environment. The @Before annotation is commonly used to set up test fixtures.
Ideal candidates should understand the importance of test fixtures for creating reliable and repeatable tests. They should be able to explain how proper setup and teardown procedures contribute to accurate test results.
6. How do you decide what to test with JUnit?
The decision on what to test with JUnit generally involves identifying critical code paths, edge cases, and any areas prone to bugs or changes. Common targets for unit tests include individual methods and classes, especially those with complex logic or dependencies.
Look for responses that show a strategic approach to testing, prioritizing high-risk areas and ensuring comprehensive coverage. Strong answers will also mention the balance between testing enough to catch potential issues and maintaining efficient test suites.
7. What are some common pitfalls to avoid when writing JUnit tests?
Common pitfalls include writing tests that are too tightly coupled to the implementation, neglecting edge cases, and not cleaning up test data properly. Another issue is relying on external systems or resources that can make tests flaky and hard to reproduce.
Candidates should display awareness of best practices for writing robust and maintainable tests. Ideal answers will provide examples of potential pitfalls and explain how to avoid them, such as using mocks and stubs appropriately.
8. How do you integrate JUnit tests into a continuous integration (CI) pipeline?
Integrating JUnit tests into a CI pipeline involves configuring your build tool (e.g., Maven, Gradle) to run tests automatically whenever code is committed or merged. This setup ensures that tests are executed consistently and any issues are detected early in the development process.
Candidates should highlight their experience with CI tools like Jenkins, Travis CI, or CircleCI and explain how they have configured these tools to automate the testing process. Look for answers that show an understanding of the benefits of CI, such as early bug detection and improved code quality.
10 Junit interview questions to ask junior developers
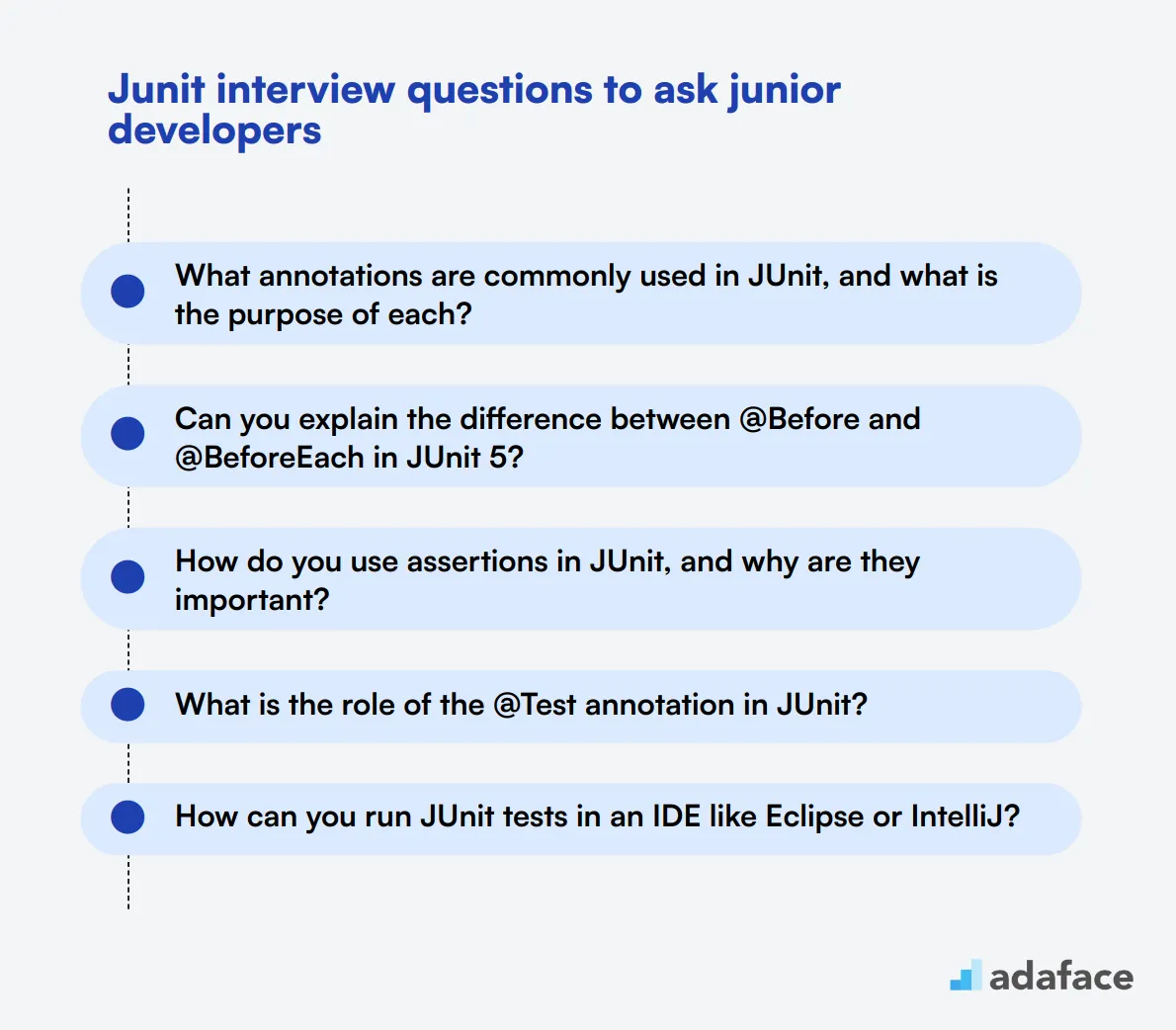
To assess if candidates possess the necessary skills for effective unit testing in Java, use these essential JUnit interview questions. Whether you're hiring for a role such as a software developer or a test engineer, these questions will help you gauge their technical understanding and practical application of JUnit.
- What annotations are commonly used in JUnit, and what is the purpose of each?
- Can you explain the difference between @Before and @BeforeEach in JUnit 5?
- How do you use assertions in JUnit, and why are they important?
- What is the role of the @Test annotation in JUnit?
- How can you run JUnit tests in an IDE like Eclipse or IntelliJ?
- What is a parameterized test in JUnit, and when would you use it?
- How do you test exceptions in JUnit?
- What is the difference between JUnit 4 and JUnit 5?
- Can you describe how to use JUnit with Mockito?
- What strategies do you use for organizing your test cases?
10 intermediate Junit interview questions and answers to ask mid-tier developers
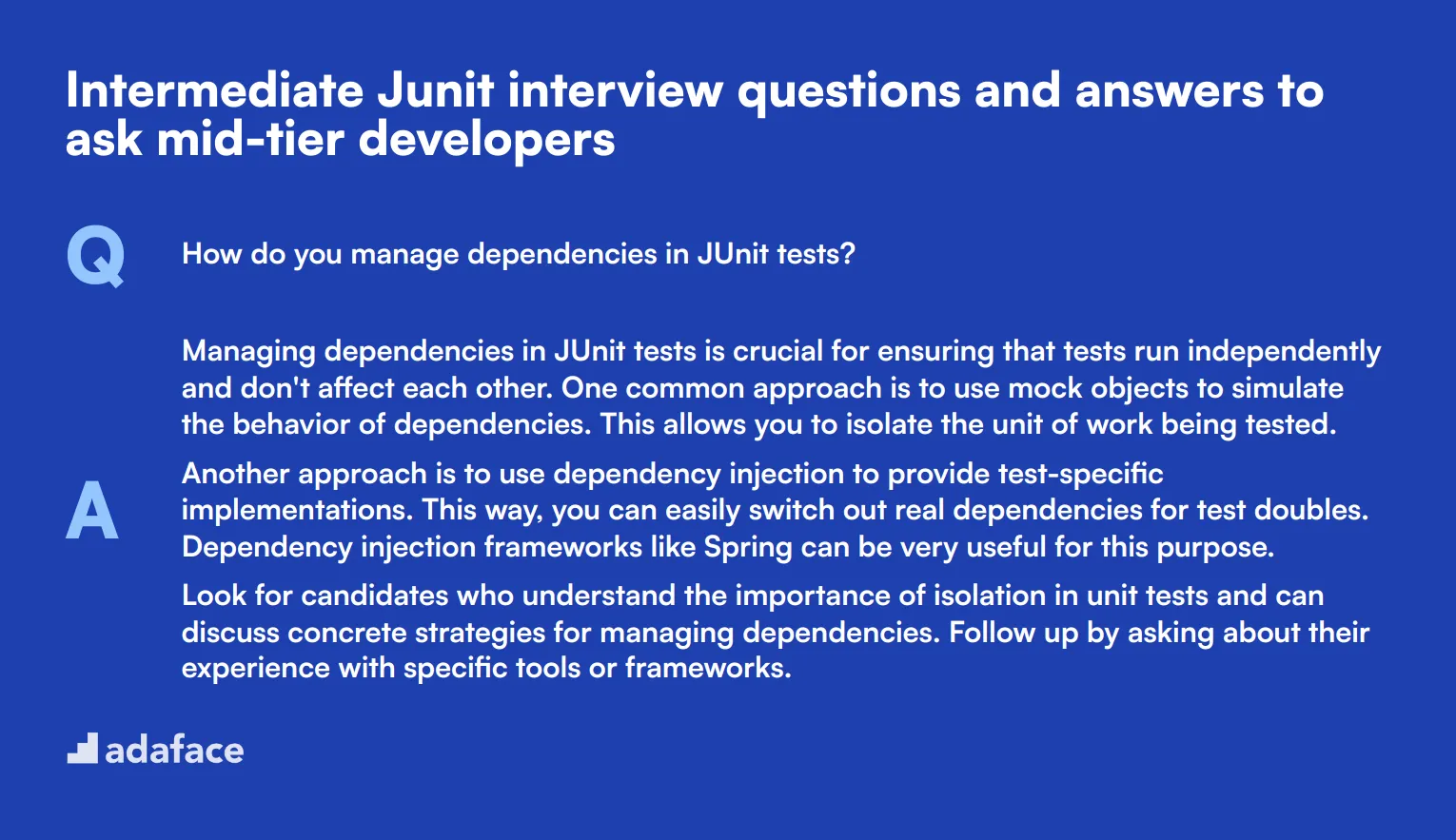
To gauge whether your mid-tier candidates have a deeper understanding of JUnit, consider these 10 intermediate interview questions. These questions will help you assess not just their technical know-how but also their problem-solving approach and ability to work within a team.
1. How do you manage dependencies in JUnit tests?
Managing dependencies in JUnit tests is crucial for ensuring that tests run independently and don't affect each other. One common approach is to use mock objects to simulate the behavior of dependencies. This allows you to isolate the unit of work being tested.
Another approach is to use dependency injection to provide test-specific implementations. This way, you can easily switch out real dependencies for test doubles. Dependency injection frameworks like Spring can be very useful for this purpose.
Look for candidates who understand the importance of isolation in unit tests and can discuss concrete strategies for managing dependencies. Follow up by asking about their experience with specific tools or frameworks.
2. What strategies do you use to ensure your JUnit tests are maintainable?
To ensure JUnit tests are maintainable, it's important to follow best practices such as keeping tests small and focused on a single functionality. This makes it easier to identify what went wrong when a test fails.
Another strategy is to use descriptive names for test methods and include clear assertions. Additionally, organizing tests into logical packages and classes helps in maintaining a clean and understandable test suite.
An ideal candidate should be able to discuss these strategies and possibly give examples from their own experience. You might want to ask them about a time when they refactored tests to improve maintainability.
3. How do you handle data setup and teardown in JUnit tests?
Data setup and teardown can be managed using JUnit annotations such as @BeforeEach and @AfterEach. These annotations allow you to run setup code before each test and teardown code after each test, ensuring a clean state for every test run.
For more complex scenarios, you can use @BeforeAll and @AfterAll annotations to run setup and teardown code once per test class. This is useful for expensive operations like initializing database connections.
Candidates should demonstrate an understanding of these annotations and discuss scenarios where they have used them. Follow up by asking about any challenges they faced in setting up test data.
4. What are some ways to improve the performance of your JUnit tests?
Improving the performance of JUnit tests often involves reducing the scope of what's being tested. This can be achieved by minimizing external dependencies and using mock objects instead of real ones.
Another way is to use parameterized tests to run multiple test cases with different inputs in a single test method, reducing the overhead of test initialization. Additionally, you can optimize setup and teardown operations to ensure they are as efficient as possible.
Strong candidates will discuss these strategies and may also reference specific tools or techniques they have used to improve test performance. Ask them for examples or case studies to assess their practical experience.
5. How do you approach testing private methods in JUnit?
Testing private methods directly is generally discouraged because it can lead to brittle tests that are tightly coupled to the implementation. Instead, focus on testing the public methods that use these private methods, ensuring they cover the necessary functionality.
In cases where testing private methods is unavoidable, you can use reflection to access and test them, although this should be a last resort. Another approach is to refactor the code to move the private method into a separate class where it can be tested directly.
Ideal candidates will understand the trade-offs involved in testing private methods and can discuss alternative approaches. Ask them about instances where they had to make such decisions and how they handled it.
6. Can you explain the concept of test-driven development (TDD) and how you apply it with JUnit?
Test-Driven Development (TDD) is a software development approach where you write tests before writing the actual code. In JUnit, this involves writing a failing test case first, then writing the minimum code required to pass the test, and finally refactoring the code while ensuring all tests still pass.
Applying TDD with JUnit encourages better design and helps catch issues early in the development process. It also ensures that your code is thoroughly tested from the start, reducing the risk of bugs.
Candidates should be able to discuss the TDD cycle and provide examples of how they've used TDD in their projects. Look for an understanding of the benefits and challenges associated with TDD.
7. How do you handle flaky tests in JUnit?
Flaky tests are tests that sometimes pass and sometimes fail without any changes to the code. To handle flaky tests, it's important to identify the root cause, which could be issues like timing problems, dependencies on external systems, or improper setup and teardown.
One approach is to isolate the flaky test and run it multiple times to gather more information. You can also improve test reliability by mocking external dependencies and ensuring that setup and teardown processes are idempotent.
Look for candidates who can discuss specific examples of flaky tests they've encountered and how they resolved them. This will give you insight into their problem-solving skills and attention to detail.
8. What is the importance of code coverage in JUnit testing, and how do you measure it?
Code coverage is a metric that indicates how much of your code is executed during testing. High code coverage generally means that more of your code is tested, reducing the risk of untested bugs.
To measure code coverage in JUnit, tools like JaCoCo or Cobertura can be used. These tools provide detailed reports showing which parts of the code were covered by tests and which were not. However, it's important to remember that high code coverage alone doesn't guarantee good tests; the quality and relevance of the tests are equally important.
Candidates should understand the balance between code coverage and test quality. Follow up by asking how they use code coverage reports to improve their test suites.
9. How do you ensure that your JUnit tests are readable and understandable by others?
Ensuring that JUnit tests are readable and understandable involves writing clear, descriptive test method names that indicate what the test is verifying. Additionally, using comments to explain the purpose of complex test cases can be helpful.
Organizing tests into logical packages and classes, and following consistent naming conventions, also contributes to readability. Using assertions effectively to make the test outcomes clear can further improve understanding.
Look for candidates who emphasize the importance of maintainability and clarity in their test code. Ask them for examples of how they've made their tests more readable in past projects.
10. Can you discuss a challenging bug you found using JUnit and how you resolved it?
Discussing a challenging bug helps assess a candidate's problem-solving and debugging skills. An ideal answer would detail the steps taken to identify the root cause, such as adding debug statements, isolating the problematic code, or writing additional tests to narrow down the issue.
Resolution might involve refactoring code, fixing logical errors, or improving test coverage. The candidate should also discuss any lessons learned from the experience and how it influenced their approach to testing in the future.
Strong candidates will provide a clear, structured narrative of the bug-finding process. Follow up by asking about specific tools or techniques they used, and how they ensured the bug wouldn't recur.
12 Junit questions related to unit testing
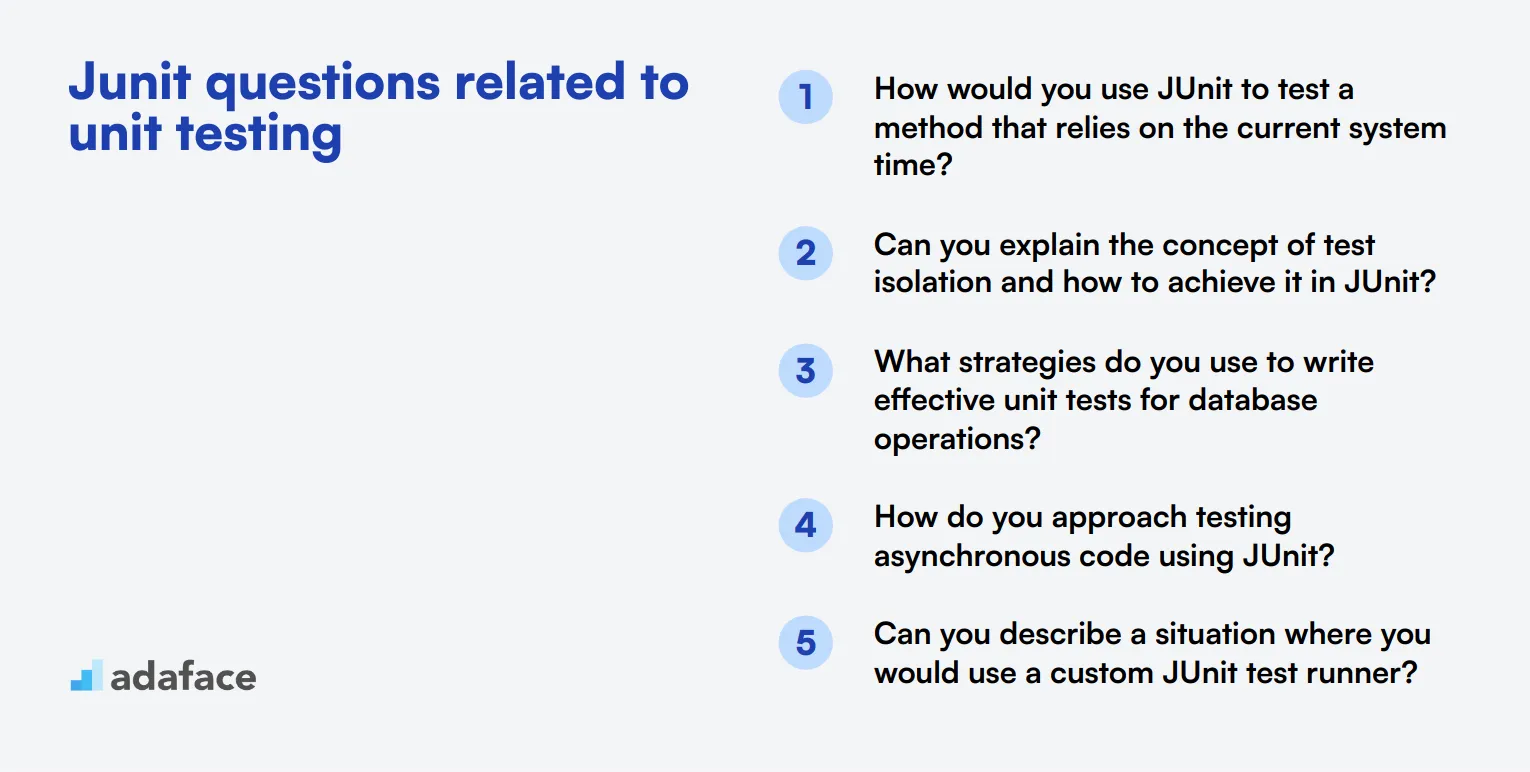
To assess a candidate's proficiency in JUnit and unit testing practices, consider asking some of these 12 JUnit questions. These questions are designed to evaluate practical knowledge and problem-solving skills, helping you identify top-tier software testing talent.
- How would you use JUnit to test a method that relies on the current system time?
- Can you explain the concept of test isolation and how to achieve it in JUnit?
- What strategies do you use to write effective unit tests for database operations?
- How do you approach testing asynchronous code using JUnit?
- Can you describe a situation where you would use a custom JUnit test runner?
- How do you handle testing of legacy code that wasn't designed with testability in mind?
- What techniques do you use to create meaningful test data for your JUnit tests?
- How would you test a method that makes HTTP requests to an external API?
- Can you explain the concept of test doubles and when you would use them in JUnit tests?
- How do you ensure that your JUnit tests are not only passing but also meaningful?
- What approach do you take when writing tests for code with complex dependencies?
- How do you use JUnit to test multi-threaded code?
8 Junit interview questions and answers related to test annotations
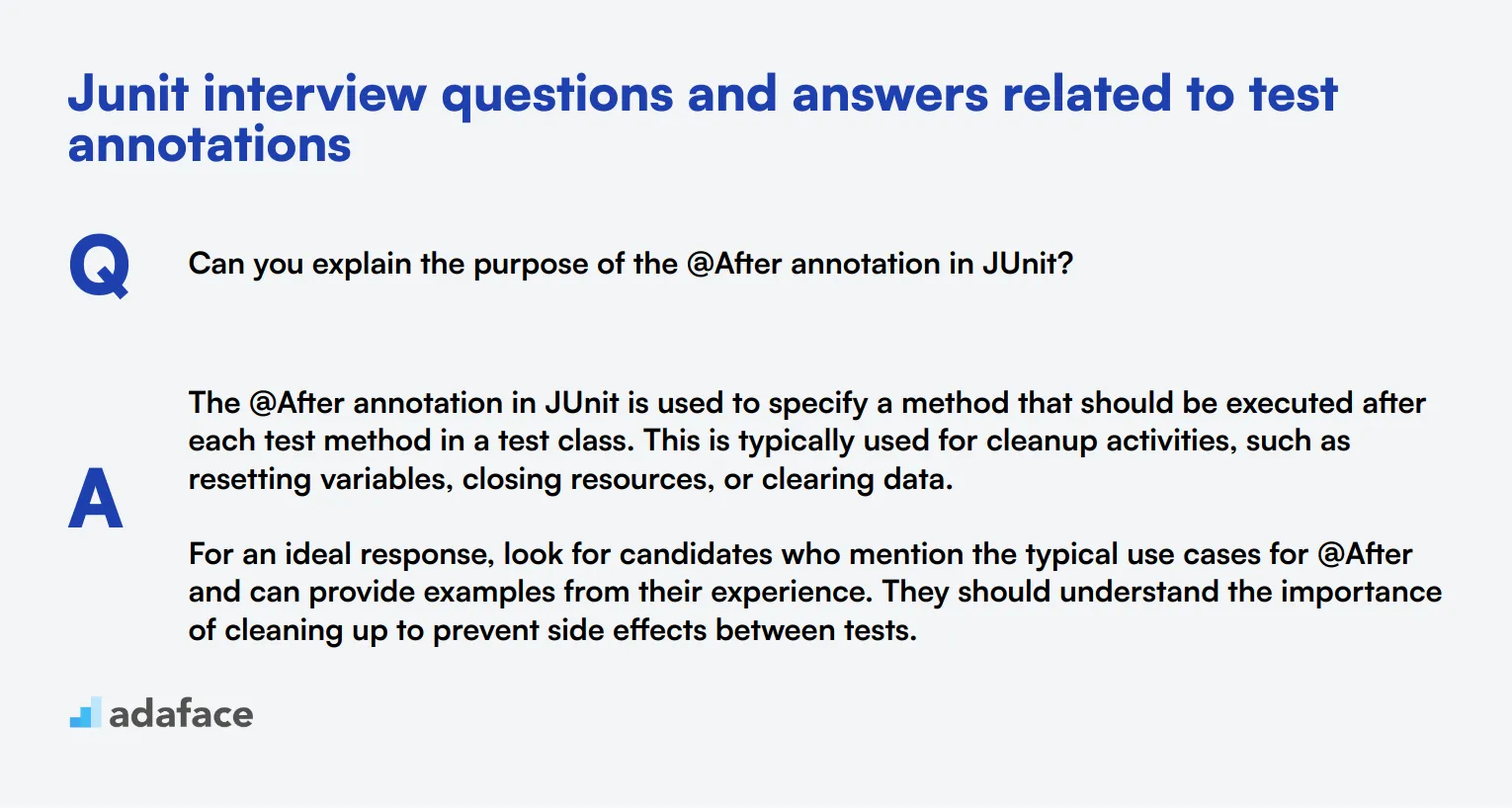
To assess whether your candidates are well-versed in JUnit test annotations, ask them some of these insightful questions. These queries will help you gauge their practical understanding and ensure they can leverage JUnit effectively in your testing environment.
1. Can you explain the purpose of the @After annotation in JUnit?
The @After annotation in JUnit is used to specify a method that should be executed after each test method in a test class. This is typically used for cleanup activities, such as resetting variables, closing resources, or clearing data.
For an ideal response, look for candidates who mention the typical use cases for @After and can provide examples from their experience. They should understand the importance of cleaning up to prevent side effects between tests.
2. What is the difference between @BeforeAll and @BeforeEach annotations in JUnit?
The @BeforeAll annotation is used to indicate that the annotated method should run once before any of the test methods in the class. It is typically used for setting up expensive resources that can be shared across all tests, such as database connections.
On the other hand, @BeforeEach is used to specify a method that should run before each test method. This is useful for setting up test-specific resources or initializing variables to a known state.
An ideal candidate should explain these differences clearly and give examples of when to use each annotation. Look for an understanding of resource management and test isolation.
3. How does the @AfterAll annotation differ from the @AfterEach annotation in JUnit?
The @AfterAll annotation signifies that the annotated method should run once after all the test methods in the current class have been executed. This is often used to release expensive resources like database connections or network sockets.
In contrast, @AfterEach is used to denote a method that should run after each individual test method. It's typically used for cleaning up test-specific resources or resetting states to avoid side effects.
Candidates should highlight these distinctions and provide contextual examples. Look for practical knowledge of managing resources efficiently and preventing test contamination.
4. Can you describe the use of @Disabled annotation in JUnit?
The @Disabled annotation in JUnit is used to disable a test method or an entire test class. This means that the annotated test method or test class will not be executed during the test run. It is useful when you have tests that are not ready to run or need to be temporarily excluded from the test suite.
Look for candidates who understand when and why to use the @Disabled annotation. They should mention scenarios such as ongoing development, known bugs, or tests that are being refactored.
5. What is the role of the @Tag annotation in JUnit?
The @Tag annotation in JUnit is used to categorize tests. By tagging tests, you can organize and filter them based on specific criteria, such as 'slow', 'fast', 'integration', or 'unit'. This allows you to run subsets of tests based on the tags assigned.
Candidates should explain how @Tag helps in managing large test suites and provide examples of how they have used it in their projects. Look for an understanding of test organization and the ability to run targeted test cases efficiently.
6. How does the @RepeatedTest annotation work in JUnit?
The @RepeatedTest annotation is used to run a test multiple times. This can be useful for identifying flaky tests or ensuring that a test consistently passes across multiple runs. You specify the number of repetitions as an argument to the annotation.
An ideal candidate should describe scenarios where repeated testing is beneficial and provide examples of how they have used @RepeatedTest. They should demonstrate an understanding of test reliability and robustness.
7. Can you explain the @Nested annotation in JUnit and its benefits?
The @Nested annotation in JUnit allows you to create nested test classes within a test class. This helps in logically grouping test cases and improving the readability and structure of your test code. Each nested class can have its own setup and teardown methods, providing better test isolation and organization.
Look for candidates who can articulate the benefits of using @Nested annotation, such as improved test maintainability and the ability to group related tests together. They should provide examples of how they have used nested classes in their testing practices.
8. What is the purpose of the @Timeout annotation in JUnit?
The @Timeout annotation is used to specify a maximum duration for a test method to run. If the test method exceeds the specified time limit, it will fail. This is useful for identifying and handling long-running or potentially hanging tests.
Candidates should explain how @Timeout helps in ensuring that tests complete in a reasonable time frame and provide examples of its use. Look for an understanding of test performance and the ability to manage test execution time effectively.
7 situational Junit interview questions with answers for hiring top developers
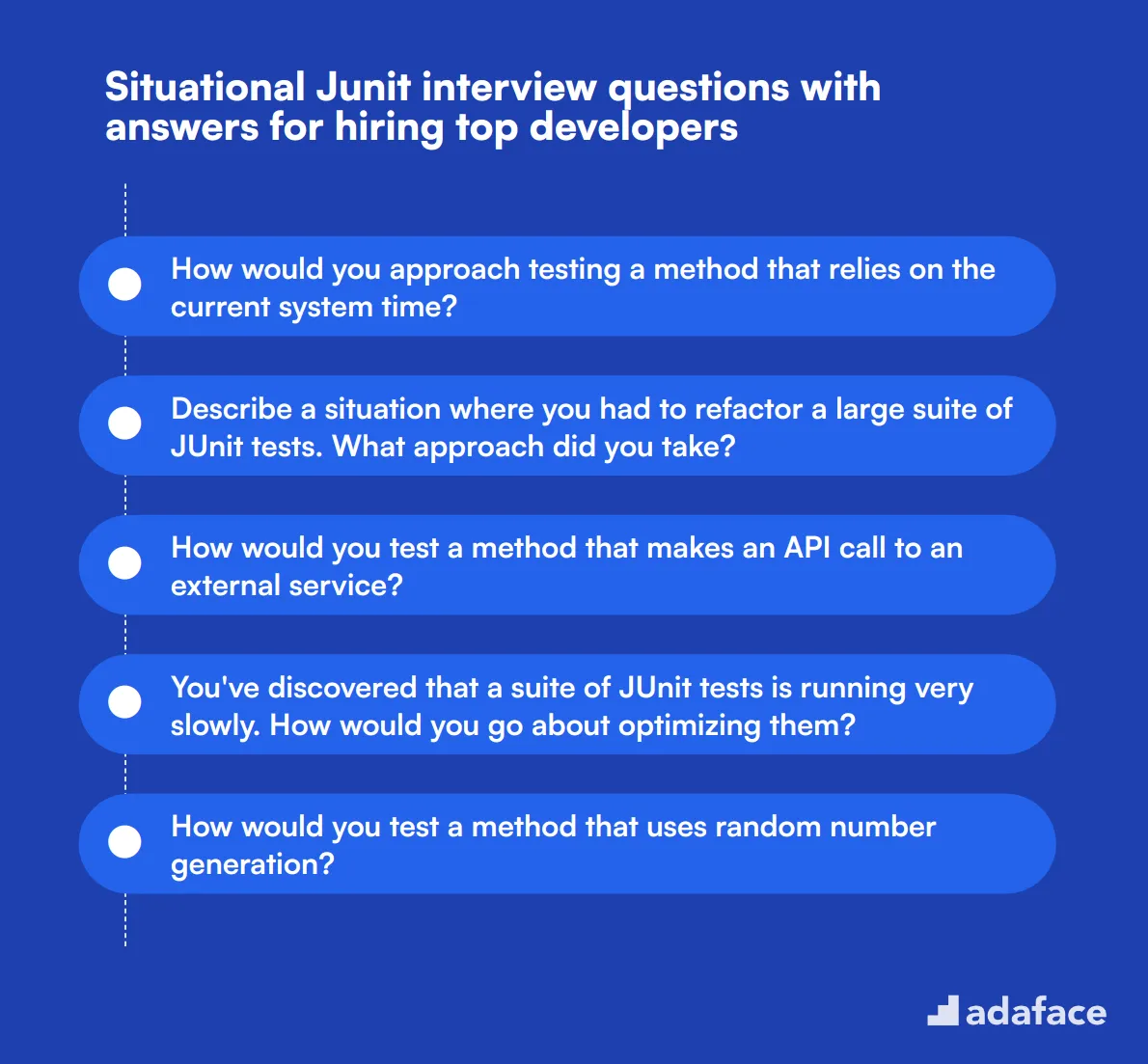
Ready to uncover the true JUnit wizards among your candidates? These 7 situational JUnit interview questions will help you identify top developers who can write robust, efficient tests. Use these questions to gauge not just technical knowledge, but also problem-solving skills and best practices in real-world scenarios.
1. How would you approach testing a method that relies on the current system time?
When testing a method that relies on the current system time, I would use dependency injection to inject a clock or time provider into the method. This allows us to control the time during tests, making them deterministic and repeatable.
Here's how I would approach it:
- Create an interface (e.g.,
TimeProvider
) with a method to get the current time
- Implement this interface with a real implementation for production code
- Create a mock implementation for testing, allowing us to set specific times
- Inject the
TimeProvider
into the class or method being tested
- In tests, use the mock implementation to set the desired time
A strong candidate should demonstrate understanding of dependency injection, mocking, and the importance of deterministic tests. Look for candidates who mention potential pitfalls of relying directly on system time in tests and show awareness of test isolation principles.
2. Describe a situation where you had to refactor a large suite of JUnit tests. What approach did you take?
When refactoring a large suite of JUnit tests, I would follow these steps:
- Analyze the existing tests to identify common patterns and redundancies
- Create a plan for refactoring, focusing on improving maintainability and reducing duplication
- Start with small, incremental changes, ensuring all tests still pass after each modification
- Introduce shared setup methods or test fixtures for common test scenarios
- Extract reusable assertion methods for complex verifications
- Use parameterized tests to consolidate similar test cases
- Organize tests into logical groupings or nested classes
- Continuously run the entire test suite to catch any regressions
A strong candidate should emphasize the importance of maintaining test coverage throughout the refactoring process. Look for mentions of version control, code reviews, and collaboration with team members during the refactoring effort. The ideal response should balance improving test structure with preserving the tests' ability to catch bugs effectively.
3. How would you test a method that makes an API call to an external service?
To test a method that makes an API call to an external service, I would use the following approach:
- Use a mocking framework (like Mockito) to create a mock of the external service client
- Set up the mock to return predefined responses for different scenarios
- Inject the mock into the class under test
- Write tests that cover various scenarios: successful responses, error responses, timeouts, etc.
- Verify that the method handles these scenarios correctly
Additionally, I would consider writing integration tests that use a test double (like WireMock) to simulate the external service. This allows testing the actual HTTP interactions without depending on the real service.
Look for candidates who mention the importance of not relying on external services in unit tests, as it can make tests slow, flaky, and dependent on external factors. They should also discuss error handling, timeout scenarios, and how to test different response types. A strong answer might also touch on contract testing or the use of test doubles for more comprehensive testing strategies.
4. You've discovered that a suite of JUnit tests is running very slowly. How would you go about optimizing them?
To optimize a slow-running suite of JUnit tests, I would take the following steps:
- Profile the tests to identify the slowest ones and understand where the time is being spent
- Look for and eliminate unnecessary setup and teardown operations
- Use @BeforeAll and @AfterAll for one-time setup and teardown instead of @BeforeEach and @AfterEach where possible
- Minimize database operations by using in-memory databases or mocking database calls
- Replace slow I/O operations with mocks or stubs
- Utilize parallel test execution if the tests are independent
- Group related tests to reduce redundant setup
- Use parameterized tests to combine similar test cases
- Optimize any custom test data generation or complex object creation
A strong candidate should emphasize the importance of maintaining test quality and coverage while improving performance. Look for mentions of tools like JUnit's built-in performance testing features or external profiling tools. The ideal response should also include considerations about the trade-offs between test speed and thoroughness, and possibly suggest strategies for running a subset of tests more frequently while keeping full runs for CI/CD pipelines.
5. How would you test a method that uses random number generation?
Testing a method that uses random number generation requires a strategy to make the tests deterministic and repeatable. Here's how I would approach it:
- Use dependency injection to inject a random number generator into the method or class
- Create an interface (e.g.,
RandomGenerator
) with methods for generating random numbers
- Implement this interface with a real random number generator for production code
- Create a mock implementation for testing, allowing us to control the 'random' numbers
- In tests, use the mock implementation to set specific sequences of numbers
- Write tests for different scenarios, including edge cases
This approach allows us to test both the logic of the method and how it handles different random inputs.
Look for candidates who understand the importance of making tests repeatable when dealing with randomness. They should mention strategies for testing edge cases and boundary conditions. A strong answer might also discuss the use of seed values for reproducibility in production code, and how to test that the method behaves correctly across a range of random inputs without making the tests themselves non-deterministic.
6. Describe how you would test a complex algorithm with multiple edge cases using JUnit.
To test a complex algorithm with multiple edge cases using JUnit, I would follow these steps:
- Break down the algorithm into smaller, testable units if possible
- Identify all edge cases and boundary conditions
- Create a comprehensive set of test cases covering:
- Normal inputs
- Edge cases (e.g., empty inputs, maximum/minimum values)
- Boundary conditions
- Invalid inputs
- Use parameterized tests to run multiple scenarios efficiently
- Implement helper methods for complex input generation or result validation
- Use assertAll() to check multiple conditions in a single test
- Consider property-based testing for generating a wide range of inputs
- Use @DisplayName and clear test method names to document what each test covers
A strong candidate should emphasize the importance of thorough testing and clear test organization. Look for mentions of test data preparation, possibly using test factories or builders. The ideal response might also include strategies for dealing with performance considerations when testing complex algorithms, such as using timeout annotations or separating long-running tests. Candidates should demonstrate an understanding of how to balance comprehensive testing with maintainable and readable test code.
7. How would you use JUnit to test a method that interacts with a database?
To test a method that interacts with a database using JUnit, I would employ the following strategies:
- Use an in-memory database (like H2) for testing to avoid affecting the production database
- Set up the database schema and initial data in @BeforeEach or @BeforeAll methods
- Use @AfterEach or @AfterAll to clean up the database after tests
- Utilize database transactions to roll back changes after each test
- Create helper methods for common database operations in tests
- Use a database migration tool (like Flyway or Liquibase) to manage test database schema
- Consider using a separate test configuration for database connection details
- Test different scenarios: successful operations, constraint violations, concurrent access, etc.
- Use mocking for external services that the database-interacting method might call
A strong candidate should discuss the trade-offs between using a real database vs. an in-memory one for tests. They should emphasize the importance of test isolation and repeatable results. Look for mentions of how to handle database-specific features or limitations in the testing environment. The ideal response might also touch on strategies for testing database performance or handling large datasets in tests without slowing down the test suite excessively.
Which Junit skills should you evaluate during the interview phase?
While it's challenging to fully gauge a candidate's capabilities in a single interview, focusing on key JUnit skills can provide significant insights. These core skills are crucial for determining a candidate's proficiency with JUnit, which is a fundamental tool for any Java developer involved in writing and testing code.
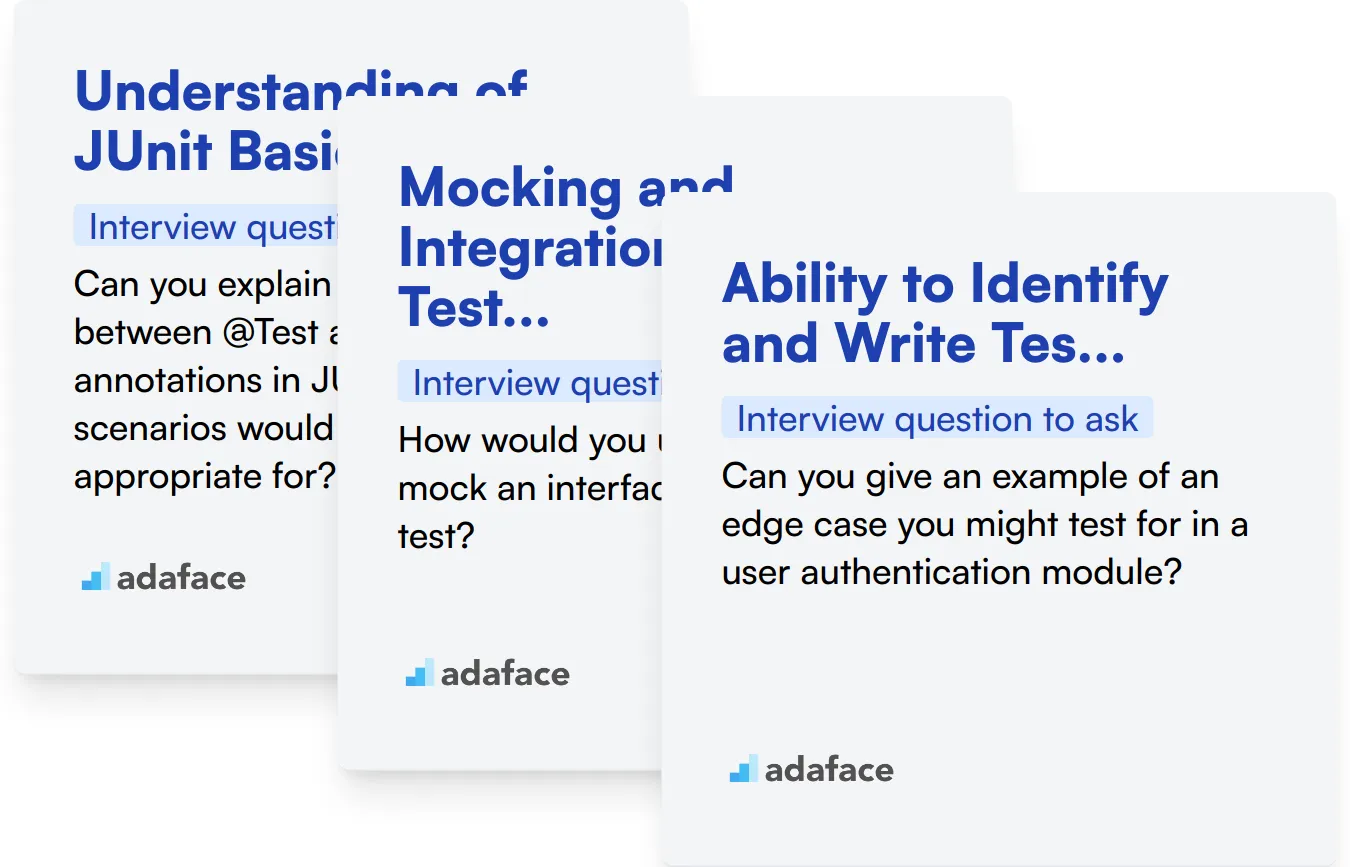
Understanding of JUnit Basics and Test Design
A deep understanding of JUnit basics and the ability to design effective tests are essential. This skill ensures that the candidate can write tests that are not only functional but also maintainable and scalable, which is critical for ongoing project health.
Consider utilizing a JUnit assessment test that features relevant multiple-choice questions to efficiently evaluate this skill.
To further assess this skill, you could ask the candidate a targeted interview question that focuses on their practical understanding of JUnit.
Can you explain the difference between @Test and @Before annotations in JUnit? Which scenarios would each be appropriate for?
Look for detailed explanations that show a clear understanding of each annotation's purpose and use cases. The ability to contextualize their use demonstrates deep knowledge of test structuring.
Mocking and Integration with Testing Frameworks
Proficiency in mocking and understanding integration with other testing frameworks like Mockito shows the candidate's ability to test Java applications more comprehensively. This skill is vital for testing in isolation and ensuring unit tests are reliable and independent of external dependencies.
To assess the candidate's capability in this area, present an interview question that tests their practical knowledge.
How would you use Mockito to mock an interface for a JUnit test?
Expect answers that demonstrate a clear process for using Mockito, including setting up the mock, defining behaviors, and integrating it within a JUnit test case. This reflects a practical application of both tools in unit testing.
Ability to Identify and Write Test Cases for Edge Cases
The ability to identify and write test cases for edge cases is indicative of a candidate's meticulousness and foresight in testing. Handling edge cases well can prevent future bugs and is a mark of a thoughtful programmer.
To evaluate this skill during the interview, pose a question that challenges the candidate to think critically about potential system limitations.
Can you give an example of an edge case you might test for in a user authentication module?
Good responses will detail specific scenarios such as testing limits on input lengths or unusual input characters. This indicates a candidate's ability to anticipate and mitigate potential issues that could arise from uncommon user behavior.
Best Practices for Using JUnit Interview Questions
Before putting your JUnit interview questions to use, consider these tips to maximize their effectiveness in evaluating candidates.
1. Combine Skills Tests with Interview Questions
Start by using skills tests to screen candidates before the interview stage. This approach helps you focus on the most promising applicants and saves time in the long run.
For JUnit-related roles, consider using a Java online test or a Java Spring test to assess candidates' technical skills. These tests can evaluate a candidate's proficiency in Java and related frameworks, which are crucial for JUnit testing.
By using skills tests, you can ensure that candidates have the necessary technical background before diving into more specific JUnit questions during the interview. This process allows you to make data-driven decisions and streamline your hiring workflow.
2. Curate a Balanced Set of Interview Questions
When preparing for the interview, select a mix of questions that cover different aspects of JUnit and related skills. This approach helps you assess candidates' knowledge comprehensively in the limited time available.
Include questions about Java programming, software testing principles, and specific JUnit features. You might also want to incorporate questions about related topics like REST API or Spring Framework to gauge the candidate's broader understanding of the testing ecosystem.
Remember to tailor your question set based on the specific role and seniority level you're hiring for. This customization ensures that you're evaluating candidates on the most relevant skills for the position.
3. Ask Insightful Follow-up Questions
Don't rely solely on prepared questions. Be ready to ask follow-up questions to dig deeper into a candidate's knowledge and experience. This approach helps you distinguish between candidates who have memorized answers and those with genuine understanding.
For example, if a candidate correctly answers a question about JUnit annotations, you might follow up by asking how they've used these annotations in real-world projects. This type of question reveals practical experience and problem-solving skills, giving you a more complete picture of the candidate's capabilities.
Hire Talented Developers Using JUnit Interview Questions and Skills Tests
If you are looking to hire someone with JUnit skills, you need to ensure they possess the required expertise. The best way to do this is to use skills tests. You can explore our relevant tests such as the Java Online Test or Java Spring Test.
Once you use these tests, you can shortlist the best applicants and call them for interviews. To get started, sign up on our dashboard or check out our online assessment platform for more information.
Java Online Test
Download Junit interview questions template in multiple formats
Junit Interview Questions FAQs
JUnit is a popular Java framework used to write and run repeatable automated tests.
JUnit questions help assess a candidate’s ability to write and execute unit tests, ensuring code quality and reliability.
Common topics include JUnit annotations, test lifecycle, test-driven development, and handling edge cases.
They can identify candidates with strong testing skills who can contribute to maintaining high code quality.
Test annotations facilitate the creation, execution, and organization of tests, making code more maintainable and readable.
Review key concepts, practice writing tests, and understand the typical scenarios and problems you might encounter.
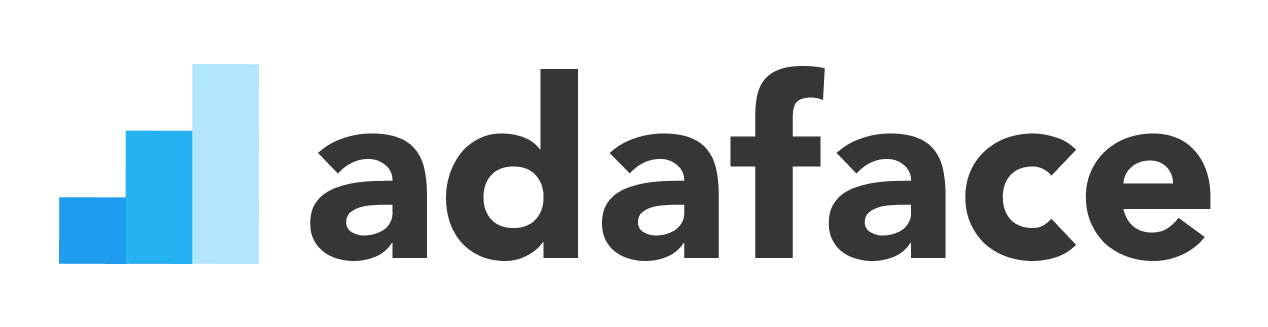
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
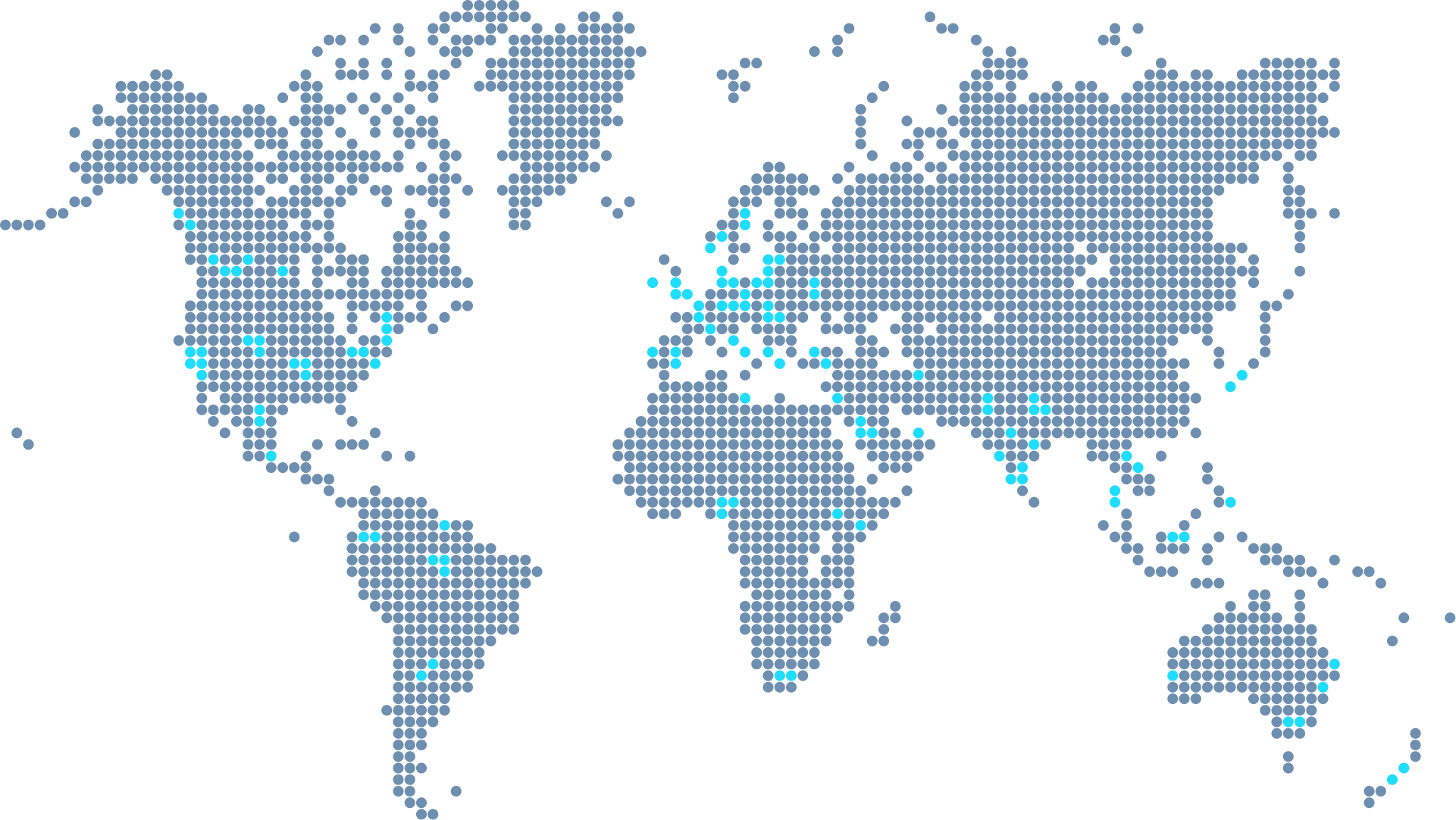
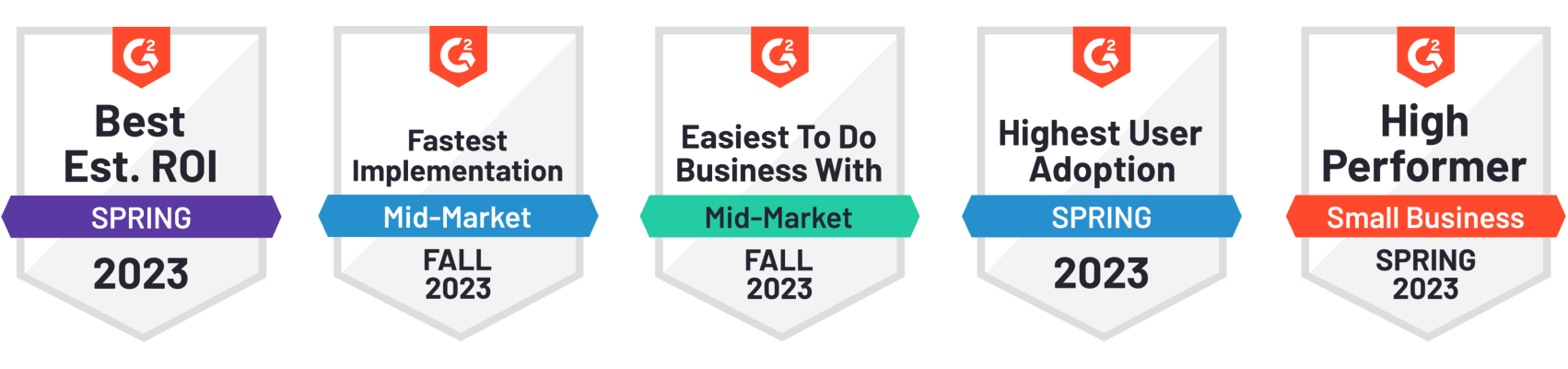