Interviewing candidates for ExpressJS roles can be challenging, especially when trying to pinpoint essential skills and knowledge. To ease this process, we’ve compiled a comprehensive list of ExpressJS interview questions to help you identify top talent, similar to those used for back end developers.
In this blog post, you’ll discover a variety of ExpressJS interview questions categorized by experience level, including questions for basic, junior, intermediate, and senior developers. Additionally, we cover specialized topics like middleware and routing to give you a well-rounded assessment toolkit.
By using these targeted questions, you can accurately gauge your candidates’ expertise and make more informed hiring decisions. For further streamlining your hiring process, consider leveraging our ExpressJS online test prior to the interview stage.
Table of contents
15 basic ExpressJS interview questions and answers to assess candidates
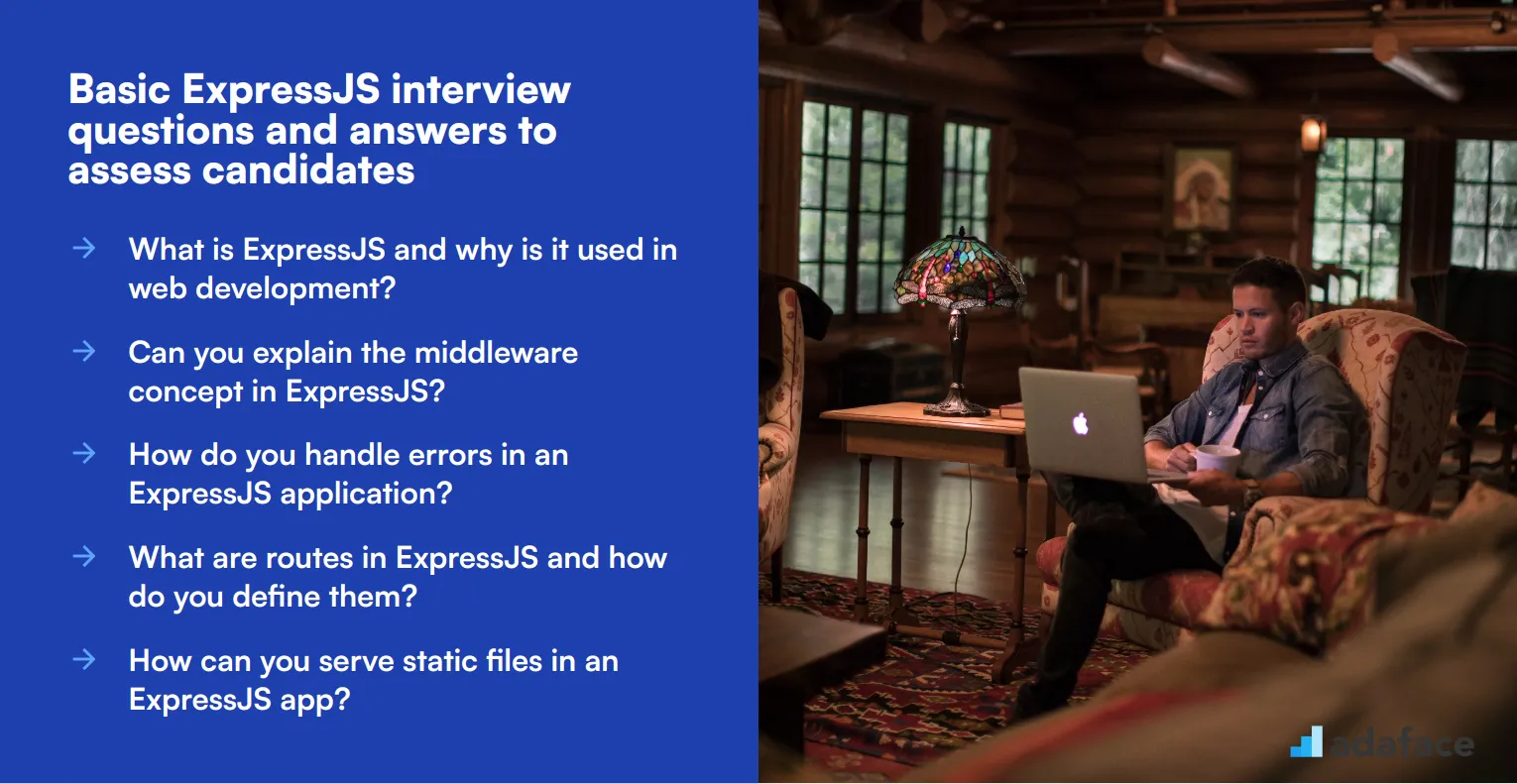
To evaluate whether candidates possess essential skills in ExpressJS, consider using this list of interview questions. These queries are designed to assess their foundational knowledge and practical understanding of ExpressJS, making them a valuable tool during the recruitment process. For specific job roles, you can refer to comprehensive descriptions like the JavaScript Developer Job Description.
- What is ExpressJS and why is it used in web development?
- Can you explain the middleware concept in ExpressJS?
- How do you handle errors in an ExpressJS application?
- What are routes in ExpressJS and how do you define them?
- How can you serve static files in an ExpressJS app?
- What is the purpose of the 'next' function in ExpressJS?
- How do you handle form data in ExpressJS?
- Can you explain the difference between req.params, req.query, and req.body?
- How do you implement authentication in an Express application?
- What are some common security practices you would implement in an ExpressJS application?
- How can you set up logging in an ExpressJS app?
- What is the role of the Express Router?
- How do you deploy an ExpressJS application?
- Can you explain the concept of template engines in ExpressJS?
- How would you optimize the performance of an ExpressJS application?
8 ExpressJS interview questions and answers to evaluate junior developers
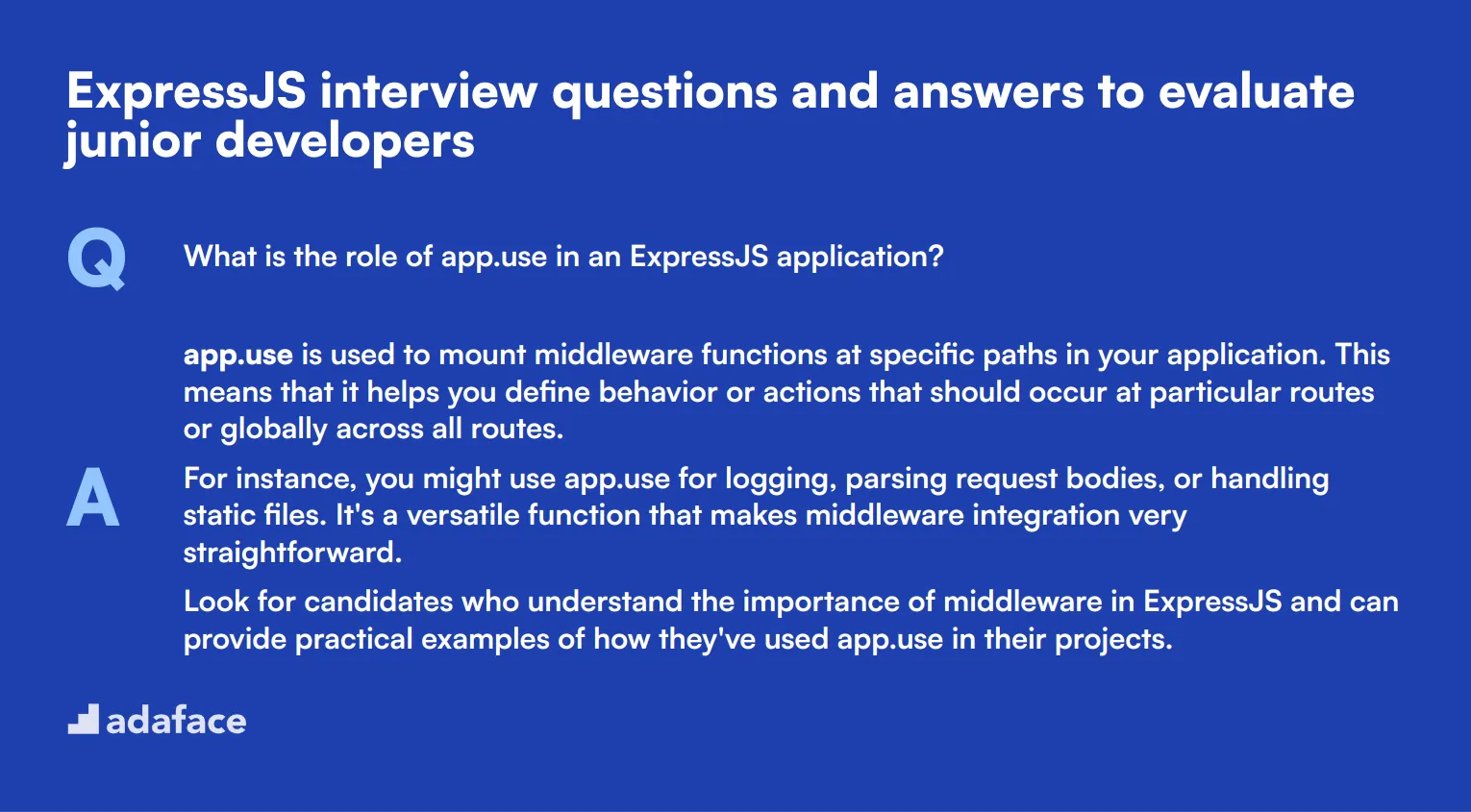
To determine whether your junior developer candidates have a solid grasp of ExpressJS basics, ask them some of these 8 interview questions. The answers will help you evaluate their understanding of fundamental concepts and their problem-solving approach.
1. What is the role of app.use in an ExpressJS application?
app.use is used to mount middleware functions at specific paths in your application. This means that it helps you define behavior or actions that should occur at particular routes or globally across all routes.
For instance, you might use app.use for logging, parsing request bodies, or handling static files. It's a versatile function that makes middleware integration very straightforward.
Look for candidates who understand the importance of middleware in ExpressJS and can provide practical examples of how they've used app.use in their projects.
2. How do you set up a basic ExpressJS server?
Setting up a basic ExpressJS server involves initializing an Express application instance and defining routes that respond to client requests. The server is then started by listening on a specific port.
A candidate might describe it like this: First, you create an Express app using express(). Next, you define routes using methods like app.get or app.post. Finally, you start the server with app.listen.
Look for candidates who can clearly outline these steps and demonstrate an understanding of how each part contributes to the overall server setup. They should also discuss any configurations or middleware they might use.
3. What are some ways to handle different HTTP methods in ExpressJS?
In ExpressJS, different HTTP methods such as GET, POST, PUT, DELETE, etc., can be handled using corresponding methods like app.get, app.post, app.put, and app.delete.
Each of these methods allows you to define a route and the specific logic that should be executed when a request with that HTTP method is made. For example, app.get('/users', callback) handles GET requests to the '/users' path.
Ideal candidates should mention the importance of using the appropriate method for the intended action and discuss scenarios where they have used various HTTP methods. Check for their understanding of RESTful principles.
4. How would you manage different environments in an ExpressJS application?
Managing different environments in an ExpressJS application typically involves setting environment variables and using configuration files to define settings specific to each environment (development, testing, production).
You can use tools like dotenv to load environment variables from a .env file. Then, within your app, you can access these variables using process.env.
Candidates should demonstrate an understanding of the importance of environment-specific configurations and provide examples of how they've managed settings like database connections, API keys, or logging levels in different environments.
5. What is the purpose of the 'next' function in middleware, and how do you use it?
The 'next' function in middleware is used to pass control to the next middleware function in the stack. Without calling 'next', the request-response cycle would be left hanging, and the request wouldn't progress to the next stage.
You use it by simply calling next() within your middleware function. This is typically done conditionally, based on some logic, to decide whether to proceed to the next middleware or send a response immediately.
An ideal answer should include an understanding of middleware chaining and practical examples of situations where they've used 'next'. Look for candidates who can explain the flow of request handling in ExpressJS.
6. How can you structure an ExpressJS application for maintainability?
A well-structured ExpressJS application often follows a modular approach, segregating different parts of the application into separate files and folders. Common practices include separating routes, middleware, controllers, and configuration files.
You might organize your app by creating folders like routes/, controllers/, models/, and middleware/. Each folder contains relevant files that handle specific aspects of the application, making it easier to manage and scale.
Look for candidates who can explain the benefits of modularization, such as improved readability, easier maintenance, and better scalability. They should also provide examples of how they've structured their own projects.
7. What are some common security practices you would implement in an ExpressJS application?
Common security practices in ExpressJS include using middleware for input validation and sanitization, setting up HTTPS, implementing authentication and authorization mechanisms, and using security headers.
You might use packages like helmet for security headers and express-validator for input validation. Ensuring secure cookie handling and rate limiting to prevent DDoS attacks are also essential practices.
Candidates should mention specific tools and techniques they have used and explain the importance of each practice. Look for an understanding of common vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
8. How do you handle form data in ExpressJS?
Handling form data in ExpressJS typically involves using middleware to parse incoming request bodies. For URL-encoded data, you can use express.urlencoded and for JSON data, express.json.
You would set up these middlewares in your app configuration, allowing you to access form data via req.body. This is crucial for processing user inputs in forms.
Look for candidates who can explain the process clearly and provide examples of how they've handled form data in past projects. They should also discuss any validation or sanitization they perform on the input data.
10 intermediate ExpressJS interview questions and answers to ask mid-tier developers
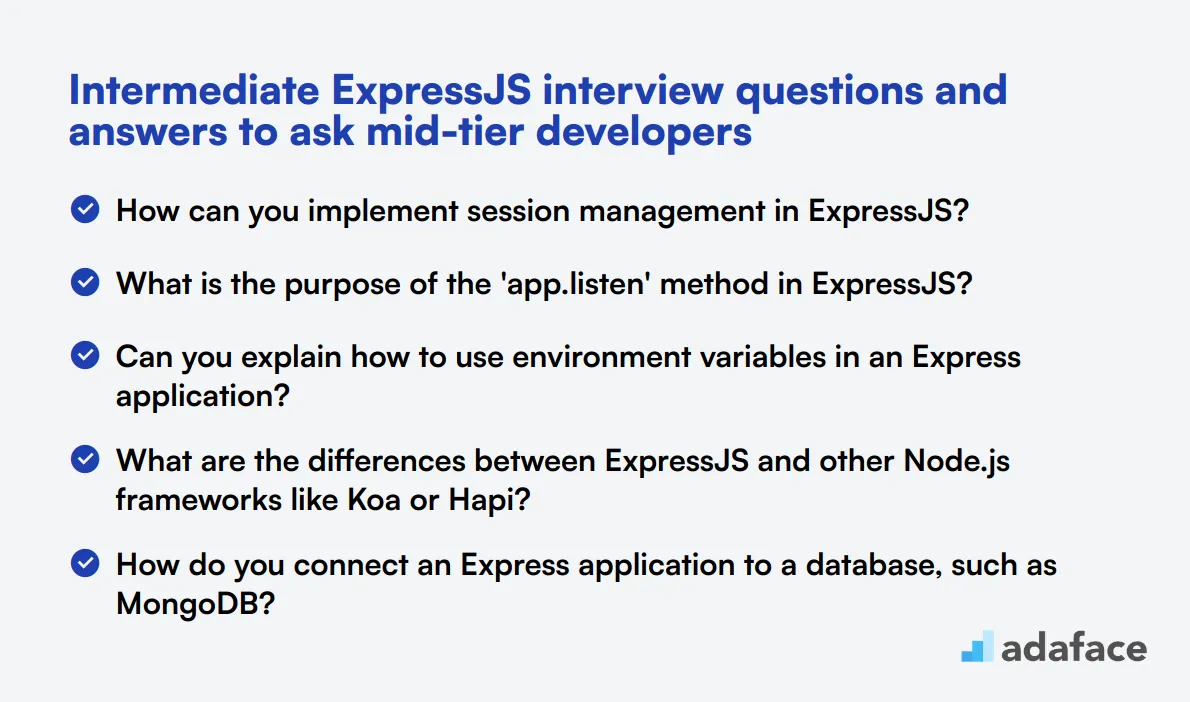
To assess the technical skills of mid-tier developers effectively, consider using this list of intermediate ExpressJS interview questions. These questions are designed to delve deeper into the candidate's knowledge and practical experience, ensuring they have the capabilities needed for a successful role in web development. For more information on related roles, check out our job descriptions.
- How can you implement session management in ExpressJS?
- What is the purpose of the 'app.listen' method in ExpressJS?
- Can you explain how to use environment variables in an Express application?
- What are the differences between ExpressJS and other Node.js frameworks like Koa or Hapi?
- How do you connect an Express application to a database, such as MongoDB?
- What are some strategies for handling CORS in an ExpressJS application?
- How can you use template engines like Pug or EJS with ExpressJS?
- What is the purpose of the 'body-parser' middleware in ExpressJS?
- How do you integrate third-party libraries into an Express application?
- Can you explain the process of setting up unit tests for an ExpressJS application?
7 ExpressJS interview questions and answers related to middleware
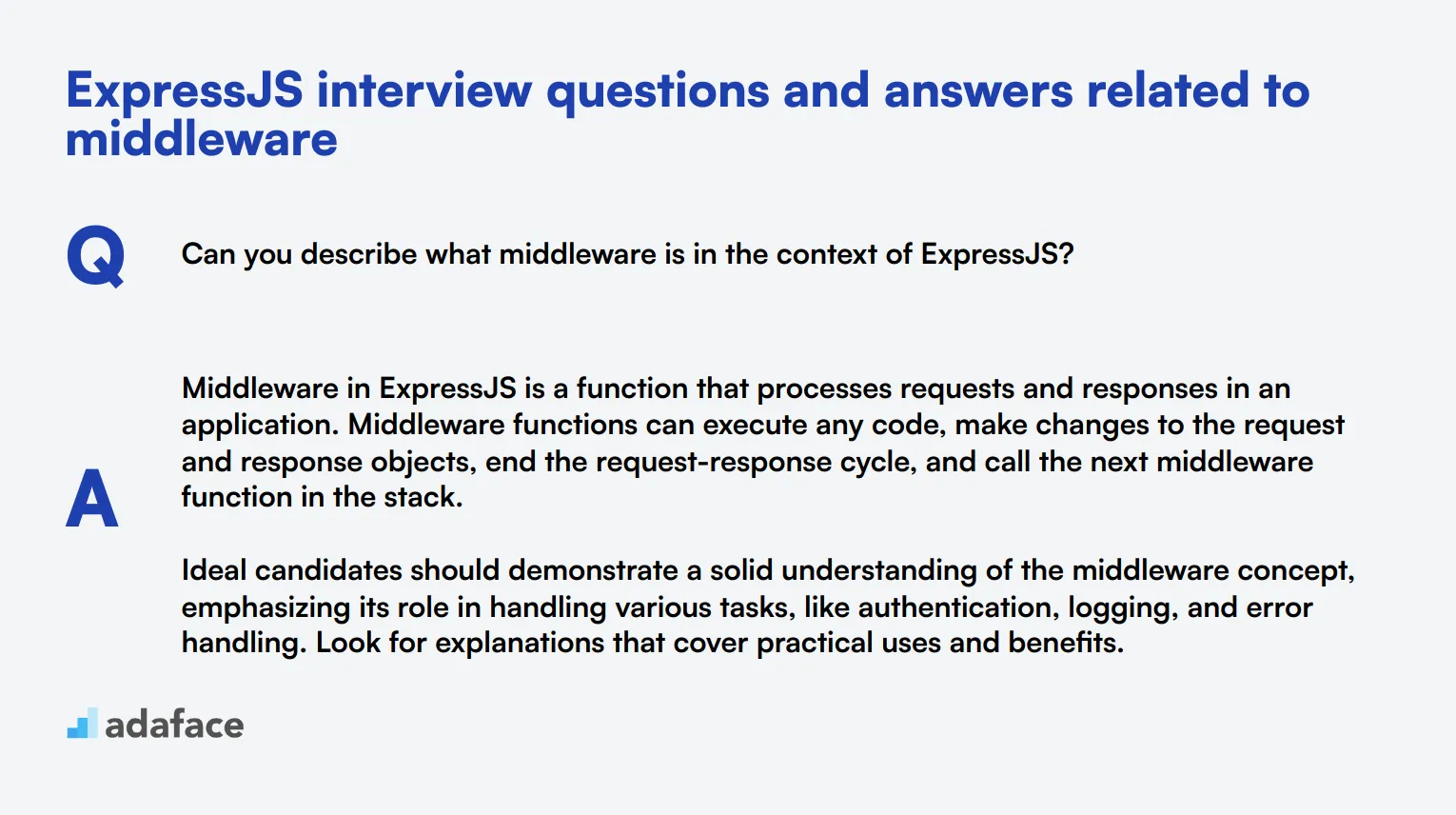
To ensure your candidates understand the essential role of middleware in ExpressJS, dive into these specific interview questions. These questions are designed to unveil their practical knowledge and problem-solving abilities, making your hiring process more effective.
1. Can you describe what middleware is in the context of ExpressJS?
Middleware in ExpressJS is a function that processes requests and responses in an application. Middleware functions can execute any code, make changes to the request and response objects, end the request-response cycle, and call the next middleware function in the stack.
Ideal candidates should demonstrate a solid understanding of the middleware concept, emphasizing its role in handling various tasks, like authentication, logging, and error handling. Look for explanations that cover practical uses and benefits.
2. How do you create custom middleware in ExpressJS?
Creating custom middleware in ExpressJS involves defining a function that takes three parameters: request, response, and next. This function can execute any necessary code and then call next()
to pass control to the next middleware.
Candidates should provide a clear explanation of the steps involved in creating custom middleware, focusing on how it fits into the request-response cycle. An ideal answer will include examples of when and why to use custom middleware.
3. What are some common types of middleware used in ExpressJS applications?
Common types of middleware in ExpressJS include built-in middleware like express.json()
for parsing JSON, third-party middleware like cors
for handling Cross-Origin Resource Sharing, and custom middleware for specific application needs.
A strong candidate response should list and briefly describe these types, providing examples of real-world applications. Look for understanding of how each type contributes to the overall functionality and security of the application.
4. How does middleware order affect an ExpressJS application?
Middleware order in ExpressJS is crucial because middleware functions are executed sequentially in the order they are defined. If middleware is placed incorrectly, it can lead to unexpected behaviors or security vulnerabilities.
Candidates should explain the importance of order and provide examples of potential issues that can arise from incorrect order. A good answer will also touch on strategies to ensure middleware is ordered correctly for optimal application performance.
5. Can you explain the difference between application-level and router-level middleware in ExpressJS?
Application-level middleware is bound to an instance of express()
and is used across the entire application. Router-level middleware is bound to an instance of express.Router()
and is used only for specific routes or groups of routes.
Look for candidates who can clearly differentiate between these two types and explain scenarios where each would be appropriate. This demonstrates their ability to organize middleware effectively within an application.
6. How can you handle errors with middleware in ExpressJS?
Error-handling middleware in ExpressJS is defined with four parameters: err
, req
, res
, and next
. It is used to catch and handle errors that occur during the request-response cycle.
A good candidate will describe how to set up error-handling middleware and provide examples of common errors it can catch, such as 404 errors or server errors. Look for an understanding of best practices in error handling.
7. Why is middleware important for handling asynchronous operations in ExpressJS?
Middleware is important for handling asynchronous operations in ExpressJS because it allows developers to manage async tasks, such as database queries or API calls, within the request-response cycle. This ensures smooth processing and error handling.
Candidates should explain how middleware helps manage asynchronous operations and provide examples of common async tasks. A strong answer will also cover how to handle errors in async middleware and the importance of using next()
properly.
12 ExpressJS interview questions about routing
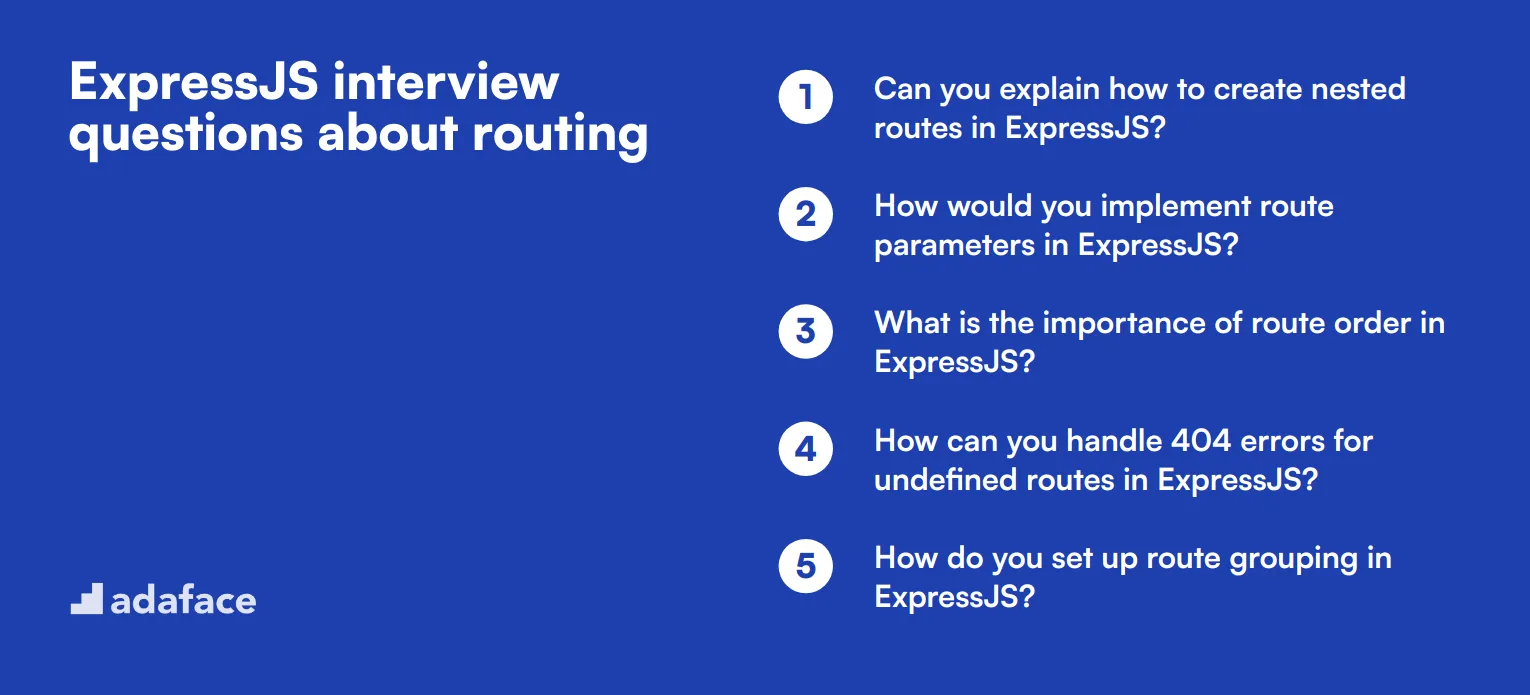
To determine whether your applicants have the right insights into ExpressJS routing, use these targeted interview questions. These questions help assess the candidate's practical knowledge, ensuring they are equipped to handle routing in a production environment. For a comprehensive understanding of other crucial skills, you can also refer to this guide.
- Can you explain how to create nested routes in ExpressJS?
- How would you implement route parameters in ExpressJS?
- What is the importance of route order in ExpressJS?
- How can you handle 404 errors for undefined routes in ExpressJS?
- How do you set up route grouping in ExpressJS?
- Can you explain the use of route-specific middleware in ExpressJS?
- How would you handle dynamic routes in ExpressJS?
- What are named routes and how can you use them in ExpressJS?
- How do you manage route constraints in ExpressJS?
- Can you describe how to set up a basic CRUD API with ExpressJS routes?
- How do you validate route parameters in ExpressJS?
- What strategies would you use to secure routes in an ExpressJS application?
10 situational ExpressJS interview questions for hiring top developers
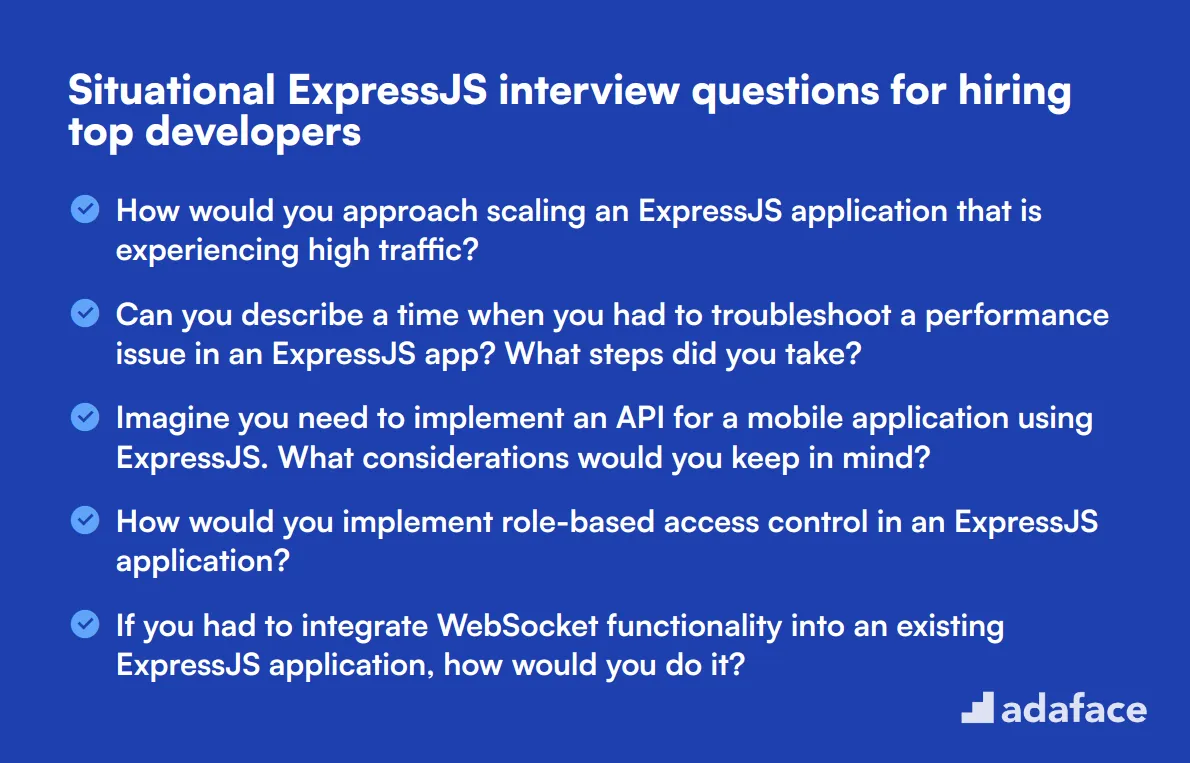
To gauge whether candidates possess the practical skills and problem-solving abilities essential for working with ExpressJS, utilize this list of situational interview questions. These queries are designed to explore their real-world experience and understanding of ExpressJS functionalities, ensuring you find the right fit for your development team in the context of a full-stack developer job description.
- How would you approach scaling an ExpressJS application that is experiencing high traffic?
- Can you describe a time when you had to troubleshoot a performance issue in an ExpressJS app? What steps did you take?
- Imagine you need to implement an API for a mobile application using ExpressJS. What considerations would you keep in mind?
- How would you implement role-based access control in an ExpressJS application?
- If you had to integrate WebSocket functionality into an existing ExpressJS application, how would you do it?
- Can you explain how you would manage asynchronous operations in your ExpressJS routes?
- How would you handle rate limiting in an ExpressJS application to protect against abuse?
- If your ExpressJS app needs to interact with a third-party API, how would you structure that integration?
- How do you ensure the security of sensitive data being transmitted in an ExpressJS application?
- What strategies would you employ to ensure unit tests in ExpressJS are effective and maintainable?
Which ExpressJS skills should you evaluate during the interview phase?
Evaluating every aspect of a candidate's skills in a single interview is challenging, but focusing on core ExpressJS skills can provide a solid foundation. These skills are crucial for assessing a candidate's proficiency with ExpressJS and will help ensure they are a good fit for your development team.
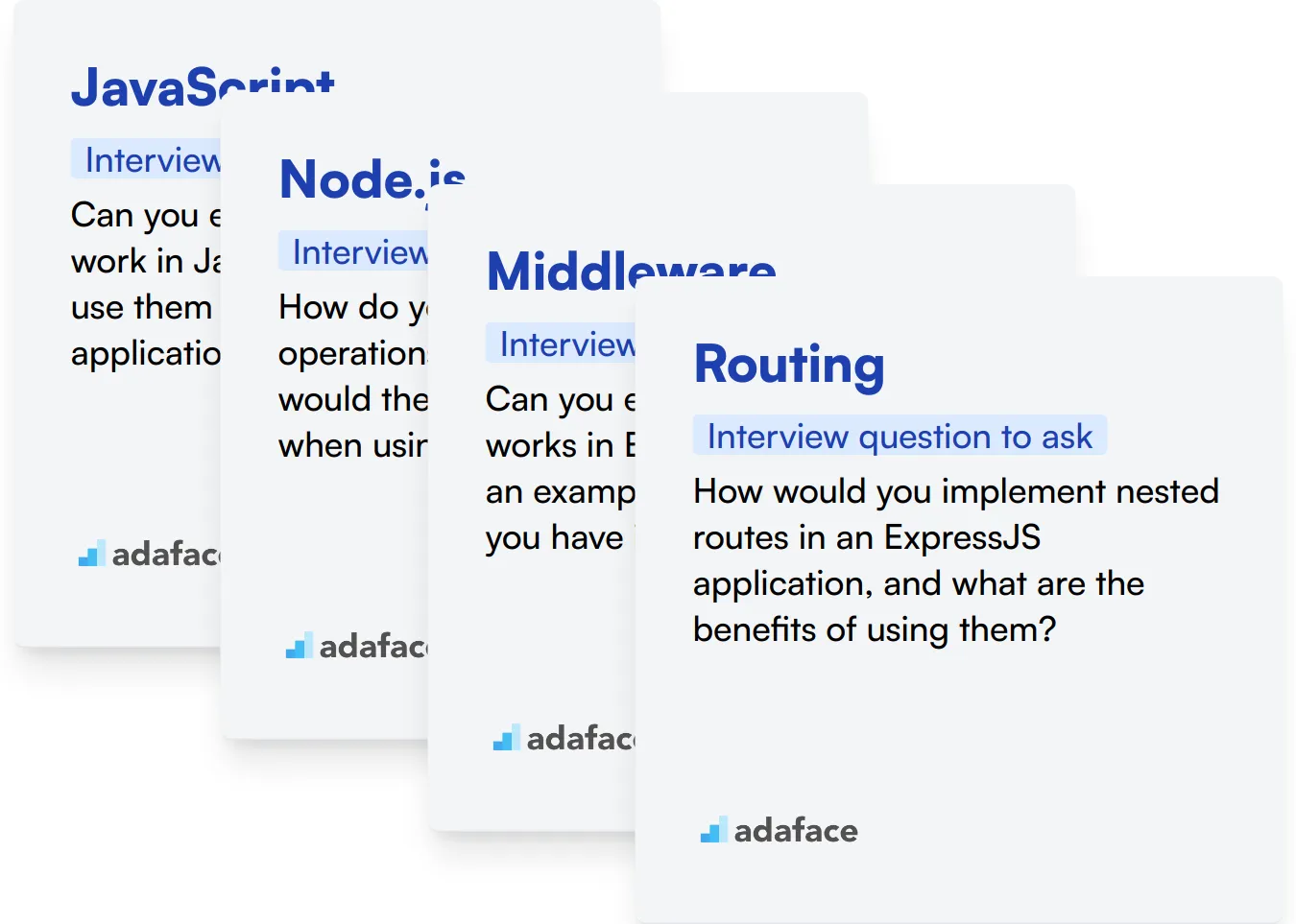
JavaScript
JavaScript is the fundamental language behind ExpressJS. A thorough understanding of JavaScript is necessary for developing, debugging, and optimizing ExpressJS applications.
Consider using an assessment test with relevant JavaScript MCQs to filter out candidates who lack basic proficiency in JavaScript. Adaface's JavaScript online test can be a useful tool in this regard.
You can also ask targeted interview questions to assess JavaScript knowledge specifically in the context of ExpressJS.
Can you explain how closures work in JavaScript and how you'd use them in an ExpressJS application?
Look for candidates who can provide a clear explanation of closures, including how they capture lexical scope. They should also be able to give practical examples of using closures in ExpressJS, such as managing middleware or routes.
Node.js
ExpressJS is built on top of Node.js, so understanding Node.js is critical for any ExpressJS developer. Proficiency in Node.js enables developers to manage server-side functionality effectively.
Implementing an assessment test with Node.js-related MCQs can help gauge a candidate's familiarity with Node.js concepts. Adaface's Node.js online test can assist in this evaluation.
Ask specific interview questions to evaluate Node.js knowledge and its application in ExpressJS projects.
How do you handle asynchronous operations in Node.js, and how would these techniques apply when using ExpressJS?
Candidates should demonstrate a good grasp of asynchronous programming in Node.js, including callbacks, promises, and async/await. They should explain how these concepts are applied within ExpressJS to handle asynchronous tasks such as API requests or database operations.
Middleware
Middleware is a core concept in ExpressJS for handling requests and responses. Understanding how to implement and use middleware effectively is essential for building scalable and maintainable applications.
Targeted questions about middleware can reveal a candidate's ability to use this feature efficiently in ExpressJS applications.
Can you explain how middleware works in ExpressJS and provide an example of custom middleware you have implemented?
Look for candidates who can clearly describe the middleware function signature (req, res, next) and its flow in request handling. They should also offer a real-world example of custom middleware, such as logging requests or handling authentication.
Routing
Routing is a fundamental aspect of ExpressJS applications, determining how an application responds to client requests. Proficiency in defining and managing routes is crucial for creating robust ExpressJS applications.
Inquire about routing techniques to understand a candidate's ability to structure and manage routes effectively in an ExpressJS application.
How would you implement nested routes in an ExpressJS application, and what are the benefits of using them?
Candidates should demonstrate an understanding of route handling, including the creation of nested routes and their benefits, such as modularity and cleaner code structure. They should also be able to provide a practical example of nested routes in use.
Hire Top ExpressJS Developers with Skills Tests and Targeted Interviews
Looking to hire someone with ExpressJS skills? It's important to verify their abilities accurately. This ensures you bring on board developers who can contribute effectively to your projects.
The most efficient way to assess ExpressJS skills is through skills tests. These tests provide an objective measure of a candidate's proficiency in ExpressJS and related technologies.
After using skills tests to shortlist top applicants, you can invite them for interviews. This two-step process helps you focus on candidates who have already demonstrated their technical abilities.
Ready to streamline your hiring process for ExpressJS developers? Sign up to access our ExpressJS skills tests and start identifying top talent for your team.
ExpressJS Test
Download ExpressJS interview questions template in multiple formats
ExpressJS Interview Questions FAQs
Key topics include basic concepts, middleware, routing, and situational questions to gauge problem-solving skills.
These questions help assess a candidate's technical knowledge, practical experience, and problem-solving approach.
No, choose questions relevant to the candidate's experience level and the specific requirements of the job role.
Focus on basic concepts, practical coding tasks, and their understanding of middleware and routing.
Use situational questions that require the candidate to explain their approach to real-world problems.
Look for clarity, depth of knowledge, practical experience, and a logical approach to problem-solving.
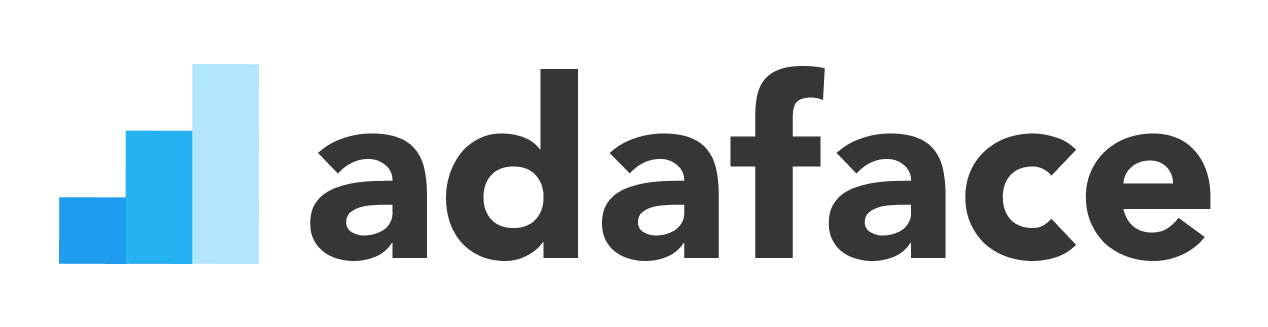
40 min skill tests.
No trick questions.
Accurate shortlisting.
We make it easy for you to find the best candidates in your pipeline with a 40 min skills test.
Try for freeRelated posts
Free resources
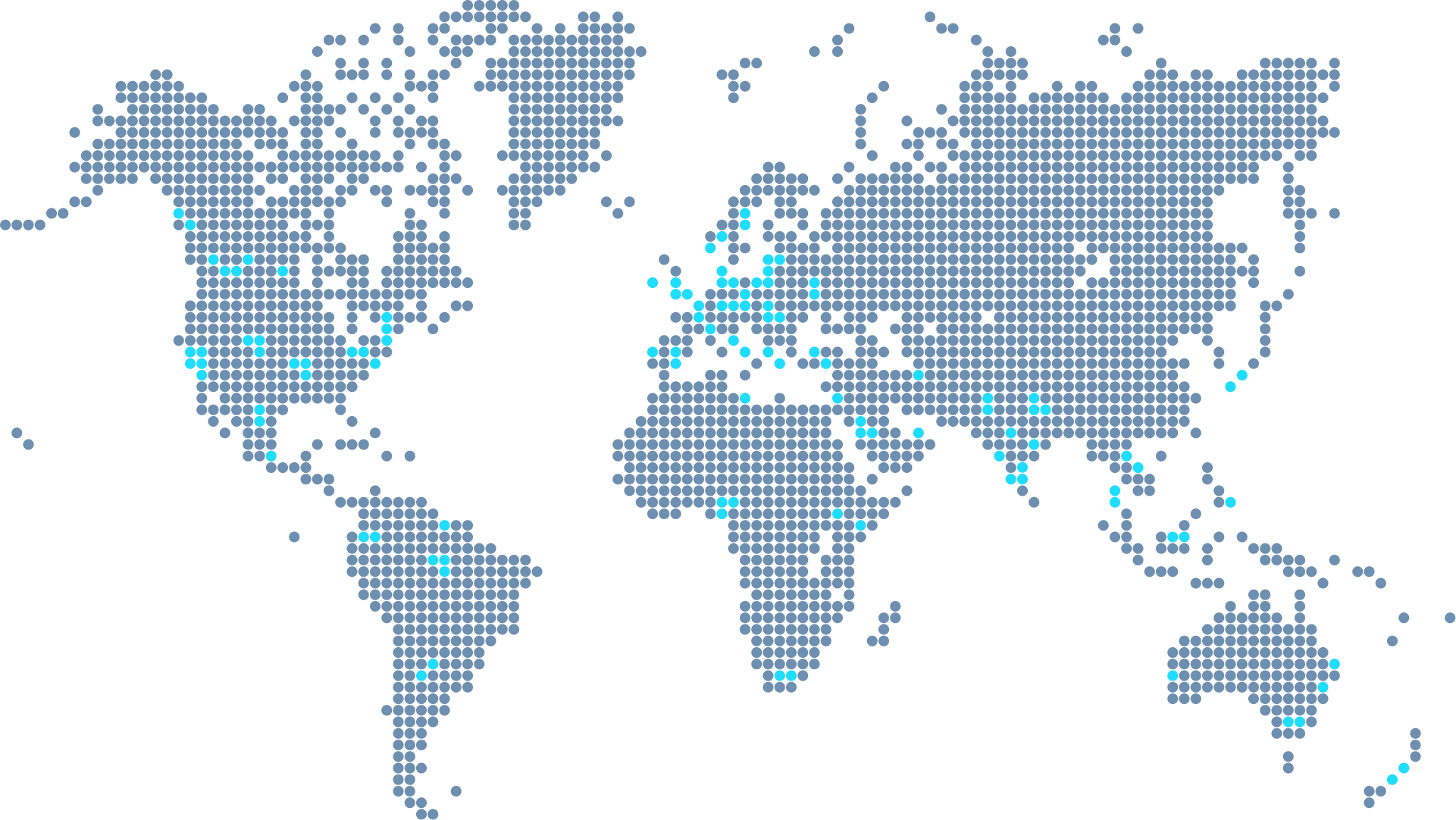
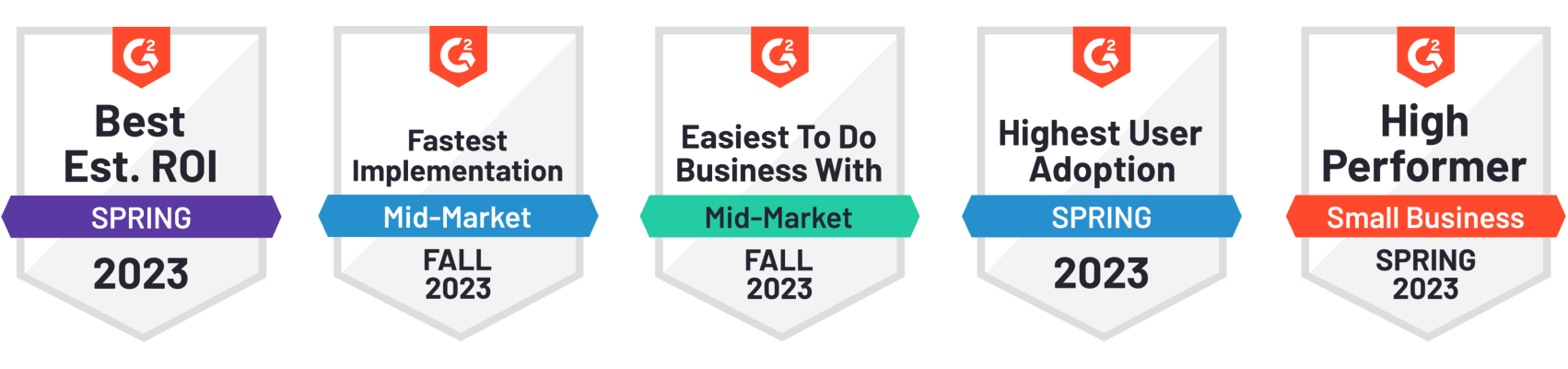